If you need to upload images and / or send http requests in your project, perform any other long-term operation that can block the UI stream, no matter how hard you need to use the solution to perform asynchronous requests.
From the very beginning, in the old-fashioned way, I’ll tell you about the standard AsyncTask / Loaders methods and explain why it’s better not to use them. Then I will tell you about advanced methods for solving this problem.
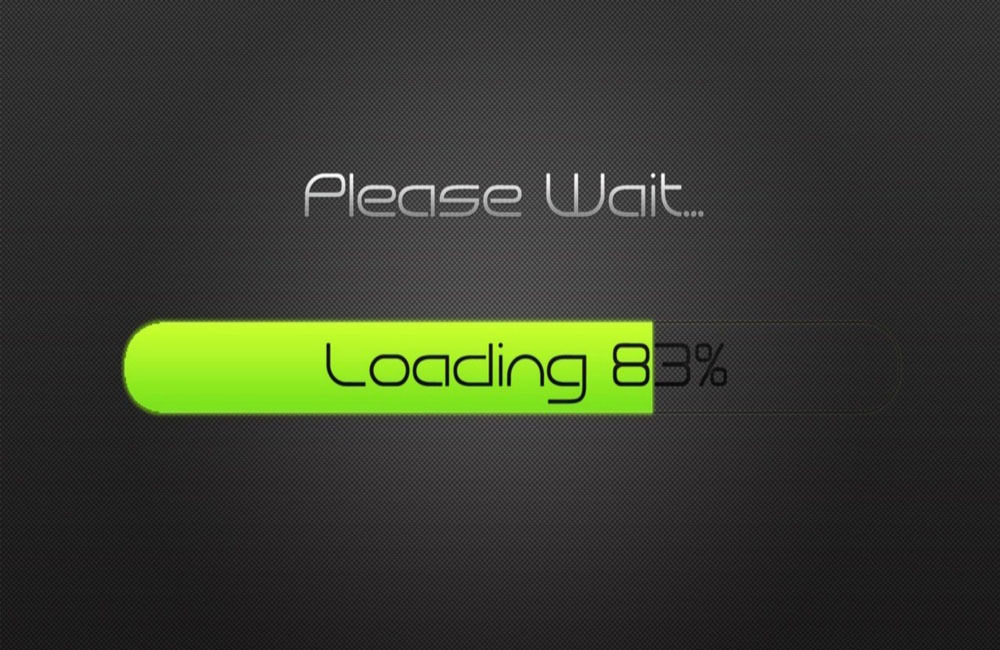
The most trivial solution is AsyncTask
Pros:
1. Simple to understand.
2. Solution out of the box.
')
Minuses:
1. Memory leak at screen flipping - the old activity will not be destroyed until the task is completed, and the result will come to the old activity, and, most likely, you will see an exception.
2. No error handling.
3. Long operations will be interrupted as soon as the system decides to destroy your application (if you have minimized it, for example).
Code example:
requestDataTask = new AsyncTask<Void, Void, JSONObject>() { @Override protected JSONObject doInBackground(Void... params) {
More modern solution - Loaders
The same as AsyncTask, except for one thing: when you flip the screen, you can get the result in a new activity. But the 2nd and 3rd problems are still there. And although Google recommends using loaders, I do not recommend it. You will have to write a lot of boilerplate code.
You can see an example
here , or you can just believe me and not use Loaders. I do not copy the code here, since these are very verbose loaders.
And now the best solution in my opinion - Robospice
Pros:
1. Robospice performs all operations in the service. This means that if the activity is destroyed, the task will continue to run anyway. The best solution for long tasks.
2. There are methods for handling errors.
3. Request caching, integration with
Retrofit .
But not everything is so rosy ... There are also disadvantages:
1. There is no method for checking whether the task is executed or not, and this is a very important thing for me. On github there is a pool request with a solution to this problem -
# 383 . Who needs it - use this fork.
2. Not the most convenient api in the world, but it will come down.
Code example:
spiceManager.execute(new SpiceRequest<String>(String.class) { @Override public String loadDataFromNetwork() throws Exception { Thread.sleep(1000);
Worth noting solution - RxJava
A good solution with a bunch of different features, you can read more on Habré in the publication
“Reactive Programming for Android” .
In short, from me: there is error handling, support for the activity / fragment life cycle. But it is not suitable for long operations (unlike Robospice), plus it was hard for me personally to deal with it, and it still seems that I do not understand the whole idea.
Pros:
1. Simple to understand. based on AsyncTask.
2. There is error handling.
3. Support life cycle activity.
4. Api is a bit more comfortable.
5. It is easy to check whether the task is being executed or not.
Minuses:
1. Poor functionality, unlike RxJava.
2. Not suitable for long tasks (see Robospice).
Code example:
new SligeTask<String,Integer,String>((notifier, strings) -> { try { Thread.sleep(1000); return "executed successfully!!!"; } catch (InterruptedException e) { notifier.publishError(e);
Conclusion
In conclusion, I would like to summarize. Personally, I will use in my projects fork Robospice and SligeTask, if the tasks are not long and do not require caching. You are free to choose whatever you want.
Yes, libraries are considered, of course, not all (there are a lot of them). I reviewed the most popular solutions.
Here you can find a bunch of others.
Write in the comments what libraries you use and why.
If someone noticed the lambda in the code examples, but does not know what it is, how and why - pay attention to
this project .