When developing a cross-platform mobile application that has in its functionality the work with files, there is a question about the organization of the processes of working with files on each platform. We encountered this issue while developing a new version of
Edusty , which allows sharing files with our classmates. In this article, we will describe how the files are imported and exported in applications running on iOS, Android, and Windows Phone operating systems.

iOS
In the iOS operating system, the concept of a file system is hidden for the user and interaction with files is carried out by the means of the application itself and only with files located in the application directory. You can import a file into the application directory in several ways — using iTunes File Sharing or registering File Types for the application.
')
When using iTunes File Sharing, the application will be displayed in iTunes in the Shared Files section, where you can add files to the application from your computer. Files added in this way fall into the
/ Documents directory of the application.
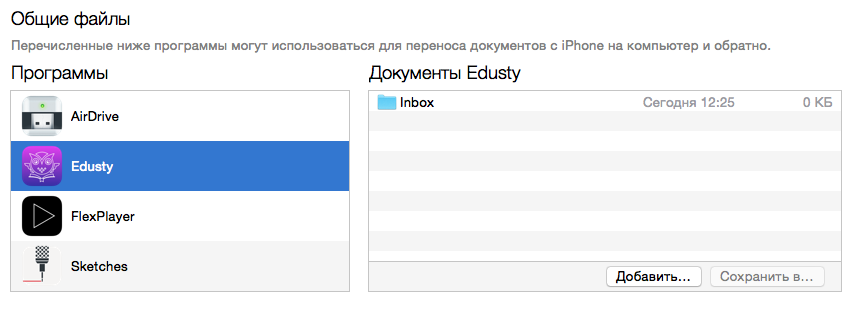
The application should monitor this directory for new files to appear. You also need to keep in mind that iTunes File Sharing actually opens up direct access to documents for the user, which means that files in this directory can be renamed, deleted, etc. at any time.
To use iTunes File Sharing, you need to add the
UIFileSharingEnabled (Application supports iTunes file sharing) flag to the
application's info.plist file.
<key>UIFileSharingEnabled</key> <true/>
When you register File Types, the application will appear in the selection list for opening the file, when you click on the standard “open with” dialog box.

When opening a file in this way, a copy of it is placed in the
/ Documents / Inbox directory, and the application method is called
: openURL: sourceApplication: annotation: of the
UIApplicationDelegate protocol, in which the url of the file being opened is transmitted.
Files in the
/ Documents / Inbox directory can be read and deleted, but cannot be changed. To change the file, you must transfer it to another directory, for example, in
/ Documents .
To be able to import files in this way, you need to add the
CFBundleDocumentTypes (Document types) key to the
info.plist file of the application. Its value is an array, each element of which is a dictionary used to describe each type of document supported by the application. A full description of possible keys and their values ​​can be found in the documentation:
developer.apple.com/library/ios/documentation/General/Reference/InfoPlistKeyReference/Articles/CoreFoundationKeys.htmlWe did not make restrictions on file types, but made the ability to import any files. Info.plist looks like this:
<key>CFBundleDocumentTypes</key> <array> <dict> <key>CFBundleTypeName</key> <string>All files</string> <key>CFBundleTypeRole</key> <string>Viewer</string> <key>LSHandlerRank</key> <string>Alternate</string> <key>LSItemContentTypes</key> <array> <string>public.data</string> </array> </dict> </array>
To export files from the application, use the same “open with” dialog box that initializes the
UIDocumentInteractionController . It is possible to immediately open a list of applications for opening a file, open a list of applications for opening a file along with standard services, such as printing a file, sending by mail, etc. It is also possible to open the file with the built-in previewer, from where a button is also available to open the file with another application.

Android
In Android, applications can get access to files, except for those that are in the private application directories.
To perform such operations as selecting a file, sending an email or opening a link in a browser, use Intents.
To select a file from the file system, you must use the
ACTION_GET_CONTENT action.
Using the
setType () method, you can specify which file types will be available for selection. For example, if you specify
setType (“audio / mp3”) , then in the file viewer we will only see files with the extension .mp3, or specify “* / *” to display all files. Then we call the
startActivityForResult () method, where we pass the
Intent.createChooser () as a parameter, which creates an application selection dialog.
Also in the manifest file you need to add the necessary permissions:
<uses-permission android:name="android.permission.READ_EXTERNAL_STORAGE" />

After we select a file in the file manager, we get to the
onActivityResult method
(int requestCode, int resultCode, Intent data) , where
Uri of our file will be in
data .
To open files from the service, use the
ACTION_VIEW action. The choice of an application for opening a file is done using the
FileOpen class (found in the vast StackOverFlow and slightly modified), in which we check what type of file we are trying to open, according to its potentially possible extension.
FileOpen.class public class FileOpen { public static void openFile(Context context, File url) throws IOException { File file = url; Uri uri = Uri.fromFile(file); Intent intent = new Intent(Intent.ACTION_VIEW); if (url.toString().contains(".doc") || url.toString().contains(".docx") || url.toString().contains(".odt")) {
When finding a match, use the
setDataAndType () method to specify
Uri and the MIME type for the data being transferred.

If the file contains a format that was not considered by us, then in
setDataAndType () we specify “* / *” as the type. Thus, the system will show us all applications installed on the device, so that we can choose by ourselves what to open the file with.
Windows phone
The universal system for Windows Store / Windows Phone Store is almost completely open file system, at least not system folders. The OpenFilePicker class allows you to open files from any available source on both platforms: device memory, external media and devices, clouds (if the appropriate applications are installed). In this regard, there are no problems with opening files on these devices, the standard class even allows you to take a photo through the file selection menu, which is very convenient.
In order to open files through the standard file selection dialog, you must create an instance of the OpenFilePicker class and initiate it with several parameters:
List<string> .FileTypeFilter
- a collection of allowed file extensions (to allow selecting any files, you need to add the entry "*").
PickerLocationId .SuggestedStartLocation
- this enumeration type allows you to specify the location that opens first in the dialog (for example: PickerLocationId.ComputerFolder).
PickerViewMode .ViewMode
- allows you to select the type of display files (list or grid).
Calling the file selection dialog on Windows 8.1 and Windows Phone 8.1 is different, despite the universal API (as you know, it is not 100% universal).
For Windows 8.1 applications, you must call the
.PickMultipleFilesAsync () or
.PickSingleFileAsync () method depending on whether you need to select one file or several. These methods return IReadOnlyList <StorageFile> and StorageFile respectively (strictly speaking, they return a Task, so you need to use async / await).
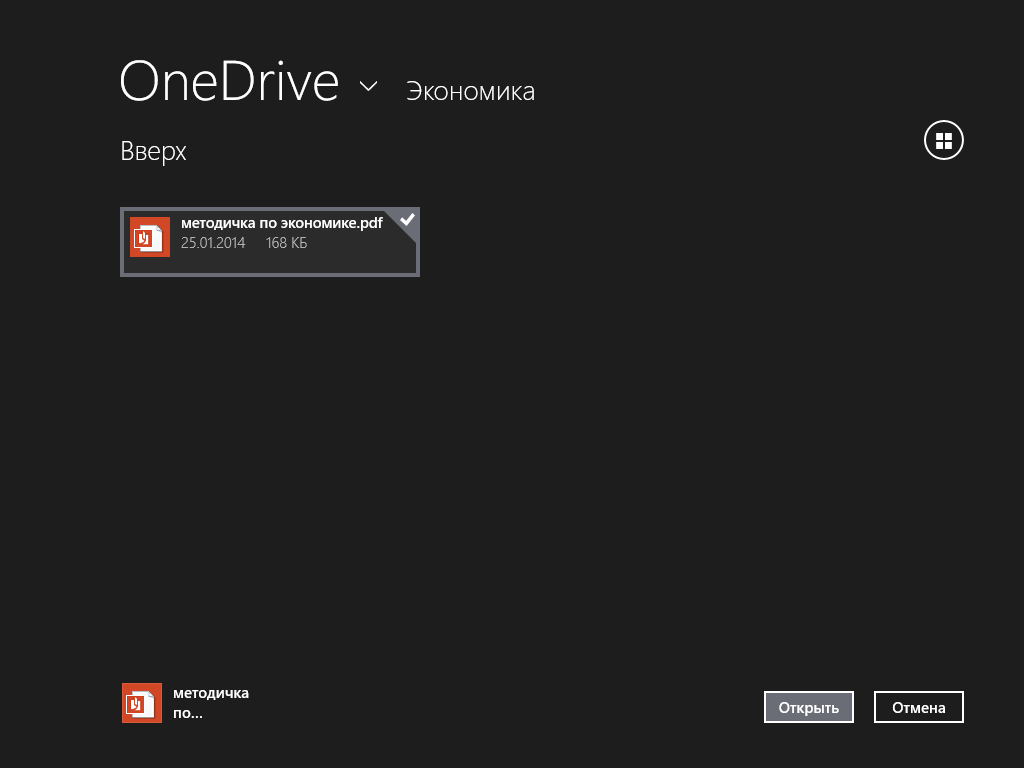
For Windows Phone 8.1, everything is somewhat more complicated. Similar methods are called
.PickMultipleFilesAndContinue () and
.PickSingleFileAndContinue () . Both methods do not return anything (void), but after selecting the files in the dialog, the
ContinueFileOpenPicker () method is called, which is a member of the
IFileOpenPickerContinuable interface. This interface is not built into the standard API, but you can take it in the
ContinuationManager class. Learn more about how to continue working after calling the * AndContinue () methods, as well as download the
ContinuationManager class
here . And so, after the
ContnuationManager class
is added to the project according to the instructions, you need a class from which the
.PickMultipleFilesAsync () or
.PickSingleFileAsync () method is called, inherit from the
IFileOpenPickerContinuable interface. Then you need to implement the
void method
ContinueFileOpenPicker (FileOpenPickerContinuationEventArgs args) , where args.Files contains a collection of StorageFile objects.

Opening files from the service is done by saving the file to disk in the internal folder of the application and then specifying it in the
Launchher method of
LaunchFileAsync () . After that, the system either opens the file as the default application for this type of file, either offers to select an application from the installed ones, or offers to find it in the store.