Maybe someone remembers the wonderful old-school space game
Star Control 2. At one time, I was struck by a huge star map with unexplored planets that were to be investigated against the background of an unfolding global catastrophe. Since the authors have published the source codes, the game has been ported under the new name of
The Ur-Quan Masters to most modern platforms.
Having rummaged in source codes, I found the simple algorithm generating textures of planets, and wrote the program in Python allowing to generate similar textures.
Algorithm
- We form a height map using the Fault Formation algorithm ( “fault formation” )
- We color the height map using the RGB reference colors and the gradient between them.
Formation of a height map
- Create a matrix of heights of the same dimension as the generated texture of the planet. North is above, south is below, and the horizontal of the matrix is a circular sweep of the planet along the parallel. The height can only vary from 0 to 255, so it is convenient to display them in shades of gray
- Fill the matrix with the base value of the height ( base elevation ), for example, 128:
')
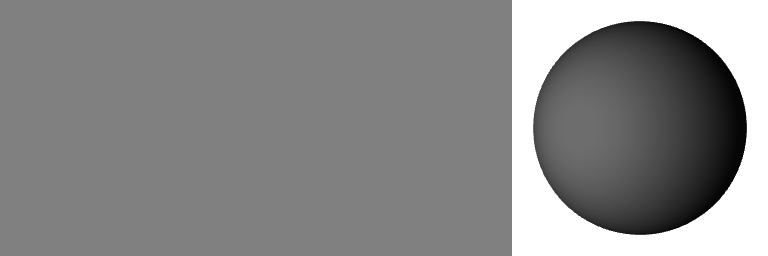
- We generate two random non-intersecting lines from north to south. These lines "form a rift" - they divide the surface of the planet into two parts, one of which we lift by a fixed constant ( elevation delta ), and the other is dropped by the same constant. For clarity, let the constant be 10:

- Repeat the previous item a specified number of times ( iterations num ).
In the figures below: 10 iterations, 100 iterations, 1000 iterations:
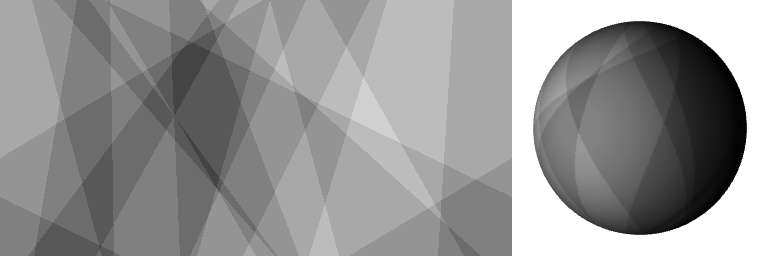


Coloring the height map with RGB colors
- We divide the entire range of heights (from 0 to 255) into N more or less equal parts with N + 1 reference points on the borders of these parts.
- Set the RGB color for each range reference point.
- We calculate the RGB color for all intermediate points of the range, linearly interpolating the color components between the reference points. In other words, we fill the height values with a gradient between the reference colors.
- We generate the texture of the planet, replacing each cell of the DEM with the RGB color calculated in the previous paragraph.
Here are a few options for coloring the same height map:
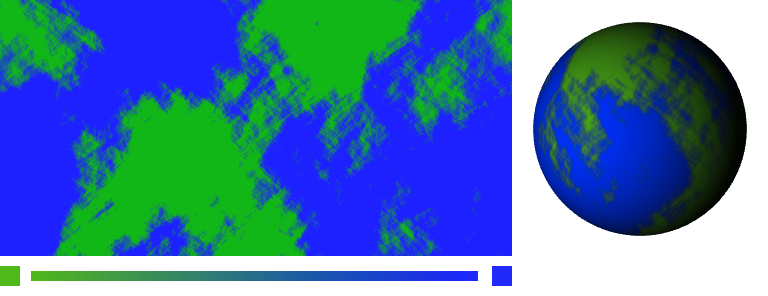


Program
To run the program, you need to install
Python 2.7 and several libraries to it:
NumPy ,
PIL and
PyOpenGL.The program can be downloaded from the git repository:
https://github.com/barabanus/starcontrolThe program consists of two independent scripts:
planet.py (generates a texture), and
space.py (draws a rotating planet with a superimposed texture).
Some management features:
- To change the reference color, left-click on the box with the color. In MacOS, in the pop-up dialog you can sample color from the screen.
- To create a new reference color, left-click on the gradient.
- To remove the reference color, right-click on the box with the color
- To save a texture, click on it with the left mouse button. This launches a script that draws a rotating planet with this texture.
The program was created on MacOS, tested on WinXP. If you found and were able to correct the error in the code or decorated the render - write and suggest changes.