Good day.
My friend and I have been developing for Android for several years. Created toys on pure Android, OpenGL, and also Unity3d. The main problem of the first two technologies is intolerance to different mobile platforms. Actually, this is why we started using Unity3d.
This is quite an interesting thing. It combines great programming possibilities with the mouse, as well as programming in a scripting language that looks like C #. Since we are Java developers, I wanted to write something cross-platform in Java. The choice fell on LibGDX. Having opened the manual and downloaded a couple of special skeleton collectors of the project on LibGDX, they started working.
')
As a result, we spent most of the time not on programming, but on setting up a project, setting up a gradle, and adding Google Play Services. As a result, having filled the bumps, we decided to write this article.
Preparing the development environment
First you need to download JDK 1.7 . In our project, we used Intellij Idea 14+ Community Edition . Well, for the mobile development of Android SDK . It should also be noted that it is recommended to use the Android 4.4W.2 SDK (API 20). Next you need to download the libgdx project collector gdx-setup.jar .
Deploying the project
In order to create a project, the libGDX developers have provided a very convenient utility in which you can select a set of libraries that you will use in your project. In general, run gdx-setup.jar and fill in all the fields:

After pressing generate a ready test project is created on libGDX. Via Intellij Idea, we call import and select gradle.build in our project directory. Further through the gradle task, you can run Android, Desktop, as well as web applications. But only for further development this project is not quite suitable. For some unknown reason, the Android module in Idea was left without dependences and, accordingly, writing some code in this module is not very convenient. Firstly, if you write something on Android, then Idea does not see dependencies and does not suggest anything, and secondly, if you compile it not through a gradle, then the absence of these dependencies will sprinkle. We dug for a long time and eventually found the right sequence of actions to build the project as it should.
Build project
First, create a project as described earlier. Then in the project directory run the commandgradlew idea
This command will create the correct project files. Then open the * .ipr project and look at the list of project modules. An indicator that all dependencies are applied will be a bold selection of each module:

In principle, it is already possible to work with the project. In order to run the Android application, you need to add the Debug / Run Configuration Andoid application with the following settings:

Similarly, we create a configuration for a desktop application:

To start the web application, run the command gradlew html:superDev
then follow the instructions in the log.
Everything would be fine if we didn’t want to add new modules to the gradle project. I didn’t want to add my dependencies to the project settings, because I must do it myself. In general, we have also been busy with this question for a long time, because gradle has long refused to see the Google Play Services libraries.
Install and configure Google Play Services
First of all, we began to follow the Setting Up Google Play Services instruction, which states the following:
Add to Android module build.gradle configuration:
dependencies { compile 'com.android.support:appcompat-v7:21.0.3' compile 'com.google.android.gms:play-services:6.5.87' }
And also in AndroidManifest.xml the following:
<meta-data android:name="com.google.android.gms.version" android:value="@integer/google_play_services_version" />
As a result, after synchronization of Gradle, we observe the following error:
Could not find any version that matches com.google.android.gms:play-services:6+. Searched in the following locations: https:
It turns out that this library was not found either in the maven repositories or in others. In order to correct the situation, you need to install additional libraries from the extras package in the Android SDK Manager:
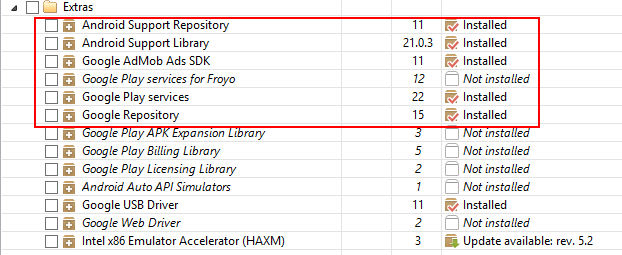
After installing all the libraries the project is assembled.
Further, the installation instructions indicated links to the BaseGameUtils project, which implements the Helper classes for working with Google Play Services. It's all pretty simple. We add the entire package to the Android module, and in the AndroidLauncher.java class, we add GameHelper initialization and inherit it from the GameHelperListener interface.
public class AndroidLauncher extends AndroidApplication implements GameHelper.GameHelperListener { private GameHelper gameHelper; @Override protected void onCreate (Bundle savedInstanceState) { super.onCreate(savedInstanceState); gameHelper = new GameHelper(this, GameHelper.CLIENT_ALL); gameHelper.setup(this); } ...
Here comes another problem. GameHelper was instantiated by us in the Android module, and all development on libGDX takes place in the Core module. Accordingly, it is necessary to come up with a mechanism by which one could call GameHelper methods in Core. For this, the IServices interface was invented, which describes all the work with the Google Play Service calls.
public interface IServices { public void signIn(); public void showAchievements(); public void showLeaderboard(); public void addScore(final String scoreName, final Integer value); public Integer getScore(final String scoreName); public void unlockAchievement(final String achievementID); public boolean isAchievementUnlocked(final String achievementID); }
We inherit from this interface our AndroidLauncher class, implement all the methods, and also save the link to it in a static manager, which is accessible throughout the project.
public class AndroidLauncher extends AndroidApplication implements IServices, GameHelper.GameHelperListener { private GameHelper gameHelper; @Override protected void onCreate (Bundle savedInstanceState) { super.onCreate(savedInstanceState); gameHelper = new GameHelper(this, GameHelper.CLIENT_ALL); AchievementsManager.setPlayService(this); gameHelper.setup(this); } @Override public final void signIn() { try { runOnUiThread(new Runnable() { public final void run() { gameHelper.beginUserInitiatedSignIn(); } }); } catch (final Exception ignored) {} } @Override public final void showAchievements() { try { runOnUiThread(new Runnable() { public final void run() { startActivityForResult(Games.Achievements.getAchievementsIntent(gameHelper.mGoogleApiClient), REQUEST_ACHIEVEMENTS); } }); } catch (final Exception ignored) {} } @Override public final void showLeaderboard() { try { runOnUiThread(new Runnable() { public final void run() { startActivityForResult(Games.Leaderboards.getLeaderboardIntent(gameHelper.mGoogleApiClient, AchievementsManager.c_ScoreID), REQUEST_ACHIEVEMENTS); } }); } catch (final Exception ignored) {} } @Override public final void addScore(final String scoreName, final Integer value) { try { Games.Leaderboards.submitScore(gameHelper.getApiClient(), scoreName, value); } catch (final Exception e) { e.printStackTrace(); } } @Override public final void unlockAchievement(final String achievementID) { try { Games.Achievements.unlock(gameHelper.getApiClient(), achievementID); } catch (final Exception e) { e.printStackTrace(); } } @Override protected final void onActivityResult(final int requestCode, final int resultCode, final Intent intent) { super.onActivityResult(requestCode, resultCode, intent); gameHelper.onActivityResult(requestCode, resultCode, intent); } }
The next step is to create Google Play Services for your project in the Google Developer Console. All we need is to create a project of services, create leaderboards and achievments in it, and then add their id to the project constants. Id services themselves need to add to AndroidManifest:
<?xml version="1.0" encoding="utf-8"?> <manifest xmlns:android="http://schemas.android.com/apk/res/android" package="com.habrahabr.test" android:versionCode="5" android:versionName="1.0" > <uses-sdk android:minSdkVersion="9" android:targetSdkVersion="20" /> <uses-permission android:name="android.permission.ACCESS_NETWORK_STATE" /> <uses-permission android:name="android.permission.INTERNET" /> <application android:allowBackup="true" android:icon="@drawable/icon" android:label="@string/app_name" android:theme="@style/GdxTheme" > <meta-data android:name="com.google.android.gms.version" android:value="@integer/google_play_services_version" /> <meta-data android:name="com.google.android.gms.games.APP_ID" android:value="ID " /> <meta-data android:name="com.google.android.gms.appstate.APP_ID" android:value="ID " /> <activity android:name="com.gesoftware.figures.android.AndroidLauncher" android:label="@string/app_name" android:screenOrientation="portrait" android:configChanges="keyboard|keyboardHidden|orientation|screenSize"> <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> </activity> </application> </manifest>
Next, do not forget to sign the project with the key and put the alpha release in the Developer Console.
After that, the services still did not work. The reason for this was the lack of permissions on the API in the console . And the Google Play Game Management, Play Game Services and Google+ API were included, but they were not enough. I had to add more Drive API, Drive SDK and Google Cloud Storage JSON API.
As a result, we were able to get the skeleton of the project on LibGDX with Google Play Services embedded in it. In the next article I would like to talk about the project itself and how to build an application architecture using the libGDX libraries.