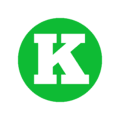
Greetings. Introducing the community project
Konva.js .
Konva.js is a framework that allows you to work on canvas 2d in object style with event support.
')
Briefly, the list of features looks like this:
- Object API
- Nested objects and “ascent” of events
- Support for multiple layers (multiple canvas elements)
- Object caching
- Animation support
- Customizable drag & drop
- Filters
- Ready-to-use objects, including rectangle, circle, image, text, line, SVG path, ..
- Easy creation of your own shapes
- Event architecture that allows developers to subscribe to event changes in attributes, drawing, and so on
- Serialization and deserialization
- Advanced search using stage.get ('# foo') and layer.get ('. Bar') selectors
- Desktop and Mobile Events
- Built-in support for HDPI devices
- and many more
Next, we will take a closer look at the capabilities of the framework with code examples.
Introduction
It all starts with a Stage, which combines custom layers (Layer).
Each layer (Layer) is one canvas element on a page and can contain shapes, groups of shapes, or groups of groups.
Each element can be stylized and transformed.
Once you have configured the Stage and layers, added shapes, you can subscribe to events, change the properties of the elements, start the animation, create filters.
Minimum code example
[Result] :

Basic shapes
Konva.js supports the following shapes: rectangle (Rect), circle (Circle), oval (Ellipse), line (Line), image (Image), text (Text), text path (TextPath), star (Star), label ( Label), svg path (Path), regular polygon (RegularPolygon). You can also create
your own shape :
var triangle = new Konva.Shape({ drawFunc: function(context) { context.beginPath(); context.moveTo(20, 50); context.lineTo(220, 80); context.quadraticCurveTo(150, 100, 260, 170); context.closePath();

Styles
Each shape supports the following style properties:
- Fill (fill) - supports solid color, gradients and images
- Contour (stroke, strokeWidth)
- Shadow (shadowColor, shadowOffset, shadowOpacity, shadowBlur)
- Transparency (opacity)
var pentagon = new Konva.RegularPolygon({ x: stage.getWidth() / 2, y: stage.getHeight() / 2, sides: 5, radius: 70, fill: 'red', stroke: 'black', strokeWidth: 4, shadowOffsetX : 20, shadowOffsetY : 25, shadowBlur : 40, opacity : 0.5 });
Result:
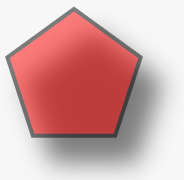
Developments
Using Konva.js, you can easily subscribe to input events (click, dblclick, mouseover, tap, dbltap, touchstart, and so on), attribute change events (scaleXChange, fillChange), and drag & drop events (dragstart, dragmove, dragend) .
circle.on('mouseout touchend', function() { console.log('user input'); }); circle.on('xChange', function() { console.log('position change'); }); circle.on('dragend', function() { console.log('drag stopped'); });
Work code + demoDRAG AND DROP
Frameworks has built-in drag support. At the moment there is no support for drop events (drop, dragenter, dragleave, dragover), but they are quite simply implemented
by the framework .
In order to drag an element, it is enough to set the properties draggable = true.
shape.draggable('true');
At the same time, you can subscribe to drag & drop events and set movement restrictions.
[Demo] .
Filters
Konva.js includes many filters: blur, inversion, sepia, noise and so on. A complete list of available filters can be found in the
filters documentation API .
Filter example:
image.cache(); image.filters([Konva.Filters.Invert]);
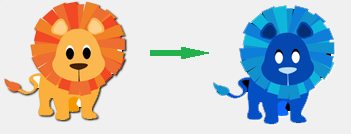
Animation
You can create an animation in two ways:
1. Through the object "Animation":
var anim = new Konva.Animation(function(frame) { var time = frame.time, timeDiff = frame.timeDiff, frameRate = frame.frameRate;
[Demo]2. Through the “Tween” object:
var tween = new Konva.Tween({ node: rect, duration: 1, x: 140, rotation: Math.PI * 2, opacity: 1, strokeWidth: 6 }); tween.play();
[Demo]Selectors
When building a large application, it is extremely convenient to use the search by created elements. Konva.js allows you to search for objects using selectors using the methods find (returns a collection) and findOne (returns the first element of a collection):
var circle = new Konva.Circle({ radius: 10, fill: 'red', id : 'face', name : 'red circle' }); layer.add(circle);
Serialization and deserialization
You can save all created objects in JSON format, for example, to save to a server or local storage:
var json = stage.toJSON();
And also create elements from JSON:
var json = '{"attrs":{"width":578,"height":200},"className":"Stage","children":[{"attrs":{},"className":"Layer","children":[{"attrs":{"x":100,"y":100,"sides":6,"radius":70,"fill":"red","stroke":"black","strokeWidth":4},"className":"RegularPolygon"}]}]}'; var stage = Konva.Node.create(json, 'container');
Performance
Konva.js has a lot of tools to significantly improve performance.
1. Caching allows you to draw some element in the buffer canvas and then draw it from there. This can significantly improve the rendering performance of complex objects, such as text or objects with shadows and outlines.
shape.cache();
[Demo]2. Work with layers. Since the framework supports several canvas elements, you can distribute objects at your discretion. Suppose an application consists of a complex background with a text and several moveable shapes. It will be logical to transfer the background and text to one layer, and the shapes to another. In this case, when updating the position of the figures, the background layer can be not redrawn.
[Demo]A more comprehensive list of performance tips is available here:
http://konvajs.imtqy.com/docs/performance/All_Performance_Tips.htmlConclusion
GitHub:
https://github.com/konvajs/konvaHomepage:
http://konvajs.imtqy.com/Documentation with examples:
http://konvajs.imtqy.com/docs/Full API:
http://konvajs.imtqy.com/api/Konva.htmlThis project is a fork of the famous
KineticJS library, which is no longer supported by the author.
The list of changes from the latest official version of KineticJS can be seen
here .