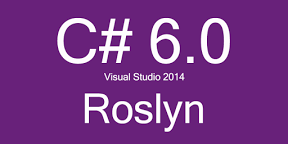
Microsoft has released a preliminary version of Visual studio 2015 and .Net 4.6 for developers. In the new C # 6.0, several new features that can facilitate coding.
This article describes the new features of the language C # 6.0. Download the new VS by the link:
Microsoft Visual Studio Ultimate 2015 Preview')
Initializing properties with values
In C # 6.0, we can initialize properties with values by writing their value to the right of them. This helps to avoid errors with null and empty property values.
Earlier:
public int Id { get; set; } public string FirstName { get; set; }
Now:
public int Id { get; set; } = 1001; public string FirstName { get; set; } = "Srinivas";
String interpolation
Every day we have to deal with concatenation of strings. Someone basically uses the “+” operator, someone - the string.Format () method. I personally prefer string.Format (). But the problems with it are known to all: with too many parameters it is hard to understand what each number means - {1}, {2}, {3}. In C # 6.0, a new feature was invented, which should combine the merits of both methods.
Earlier:
name = string.Format("Employee name is {0}, located at {1}", emp.FirstName, emp.Location);
Now:
name = $"Employee name is {emp.FirstName}, located at {emp.Location}";
At the request of workers IL code
IL_0000: nop IL_0001: ldstr "Ivan" IL_0006: stloc.0 IL_0007: ldstr "Moscow" IL_000c: stloc.1 IL_000d: ldstr "Employee name is {0}, located at {1}" IL_0012: ldloc.0 IL_0013: ldloc.1 IL_0014: call string [mscorlib]System.String::Format(string, object, object) IL_0019: stloc.2 IL_001a: ret
You can also use the conditions:
name = $"Employee name is {emp.FirstName}, located at {emp.Location}. Age of employee is {(emp.Age > 0) ? emp.Age.ToString() : "N/A"}";
Using lambda expressions
In C # 6.0, properties and methods can be defined via lambda expressions. This greatly reduces the amount of code.
Earlier:
public string[] GetCountryList() { return new string[] { "Russia", "USA", "UK" }; }
Now:
public string[] GetCountryList() => new string[] { "Russia", "USA", "UK" };
Import static classes
All static class members can be defined using another static class. But we have to constantly repeat the name of this static class. With a large number of properties, you have to repeat the same thing many times.
C # 6.0 introduced the ability to import using the keyword using static classes. Let's look at everything using the Math library as an example:
Earlier
double powerValue = Math.Pow(2, 3); double roundedValue = Math.Round(10.6);
Now:
using System.Math; double powerValue = Pow(2, 3); double roundedValue = Round(10.6);
This can be used not only inside the class, but also when executing the method:
Earlier:
var employees = listEmployees.Where(i => i.Location == "Bangalore");
Now:
using System.Linq.Enumerable; var employees = Where(listEmployees, i => i.Location == "Bangalore");
Null conditional statement
C # 6.0 introduces a new so-called Null-conditional operator (?.), Which will work on top of the conditional operator (? :). It is designed to facilitate checking for null values.
It returns null values if the class object to which the statement is applied is null:
var emp = new Employee() { Id = 1, Age = 30, Location = "Bangalore", Department = new Department() { Id = 1, Name = "IT" } };
Earlier:
string location = emp == null ? null : emp.Location; string departmentName = emp == null ? null : emp.Department == null ? null : emp.Department.Name;
Now:
string location = emp?.Location; string departmentName = emp?.Department?.Name;
nameof operator
In C # 6.0, the nameof operator will be used to avoid the appearance of string literals of properties in code. This operator returns the string literal of the element passed to it. As a parameter, you can pass any member of the class or the class itself.
var emp = new Employee() { Id = 1, Age = 30, Location = "Moscow", Department = new Department() { Id = 1, Name = "IT" } }; Response.Write(emp.Location); //result: Moscow Response.Write(nameof(Employee.Location)); //result: Location
Await in catch and finally blocks
Prior to C # 6.0, it was not possible to use the await statement in the catch and final blocks. Now this opportunity has appeared. It can be used to free up resources or to log errors.
public async Task StartAnalyzingData() { try {
Exception filters
The exception filters were in the CLR, and they are available in VB, but they were not in C #. Now this feature has appeared, and you can impose an additional filter on exceptions:
try { // } catch (ArgumentNullException ex) if (ex.Source == "EmployeeCreation") { // } catch (InvalidOperationException ex) if (ex.InnerException != null) { // } catch (Exception ex) if (ex.InnerException != null) { // }
Dictionary initialization
In C # 6.0, the ability to initialize Dictionary by key value is added. This should simplify the initialization of dictionaries.
For example, for JSON objects:
var country = new Dictionary<int, string> { [0] = "Russia", [1] = "USA", [2] = "UK", [3] = "Japan" };
In C # 6.0, there are a lot of syntax changes and new features. Microsoft also improves the new compiler in terms of performance.
PS New features are described for the current version of the compiler, the syntax may be changed by the final version.