In the
previous article, we connected the OpenHAB open-source home automation platform with the Arduino controller using a very simple text protocol. But this decision will stump us, if we want to connect our controller to another system, what should we do?
Modbus is the most famous and widespread standard in industrial automation, it is supported by millions of devices around the world, these devices are easily integrated into a single network and interconnect with a huge amount of off-the-shelf software. Let's try to use it in our project?
What do we need to know about this standard?
The Modbus protocol uses serial communication lines (for example, RS232, RS485), and the Modbus TCP protocol is designed for data transmission over TCP / IP networks.
Modbus protocol has two modes of transmitting RTU and ASCII, in ASCII mode, each byte is transmitted as two ASCII characters of its hexadecimal representation.
There is only one master in the Modbus network, which polls several slave devices at a given interval, each of which has its own unique address from 1 to 254, the address 0 is broadcast and all devices respond to it, since it has one address on the network master. .
The Modbus specification defines two types of data, one bit and 16 bit word. The data is organized into four tables with 16-bit addressing cells, addressing in the tables starts from 0. Separate commands are used to access data from different tables.
Discrete inputs | 1 bit | only reading |
Coils | 1 bit | read and write |
Input Registers | 16 bits | only reading |
Holding registers | 16 bits | read and write |
How do we connect a Modbus device to OpenHAB?
The
Modbus Tcp Binding module is responsible for this, this module operates in the master mode and provides the connection of several slave devices via a serial port or TCP / IP network.
In order to connect Arduino with it, we need to implement a Modbus slave device in the controller, let's use the
Modbus-Master-Slave-for-Arduino library for this.
')
Download the library and create a sketch by copying the following code into the program editor. If you wish, you can simply download the project with the necessary files from the
repository .
Consider the example of our sketch, the basic steps necessary to work with this library.
All library functions are implemented in a single ModbusRtu.h file.
To interact with it, you need to create an object in the program by specifying the address, serial port number, output number controlling the transmission in the Modbus constructor (for RS485)
Modbus slave(ID, 0, 0);
Then define an array of Modbus registers.
uint16_t au16data[11];
After that, when starting the program, configure the serial port of the slave
slave.begin(9600);
In the main program loop, call the Modbus message handling function.
state = slave.poll(au16data, 11);
And after that, you can process the data and save the necessary variables in the Modbus registers.
#include "ModbusRtu.h" #define ID 1
The standard provides a separate table for each data type, but the feature of the applied library is that all registers are stored in one array as overlapping tables, so the controller register structure will look like this:
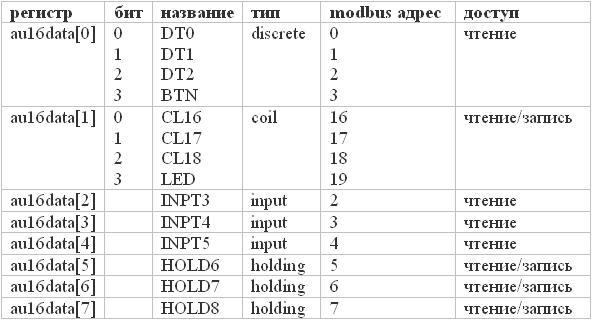
To demonstrate the work with different registers, in the course of program operation, data from a register with a coil type will be copied into a register with a discrete type, and from registers with a holding type into registers with an input type. In addition, the button state will be saved to the third bit of the register au16data [0] (discrete), and the value of the third bit of the register au16data [1] (coil) is output to the LED.
We will refine the layout of the controller, which was assembled for previous experiments, switch the LED from 13 to 12 output. Usually the Arduino board itself already has an LED connected to pin 13, in our program it will become an indicator of the status of work. Now we connect the USB cable to the computer, compile and download the program to the controller.

It's time to start testing. It greatly facilitates the work at this stage of the Modbus master device emulator, the network has some good, while free programs, here are some of them:
www.focus-sw.com/fieldtalk/modpoll.htmlqmodbus.sourceforge.netwww.mikont.com/products/EAT-Console.htmlAmong them, the EAT-Console utility allows not only to control and poll Modbus devices, but also displays data in graphical form, which is very convenient when debugging work with various sensors, such as humidity, pressure and temperature sensors. Before you start working with the program and its configurator, I recommend that you familiarize yourself with the
documentation .
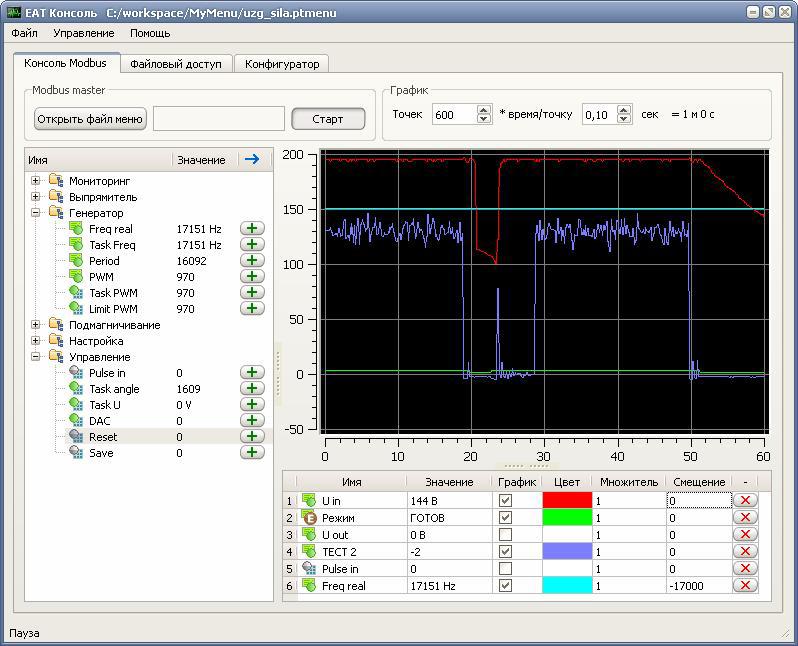
To install the emulator, you need to download the
archive and unpack it to the C: \ arduino \ EATConsole folder, then open the
Eclipse download page , download the Eclipse IDE for Java Developers and unpack it to the C: \ arduino \ eclipse folder, then copy the files from the C folder: \ arduino \ EATConsole \ eclipse \ plugins in the C: \ arduino \ eclipse \ plugins folder.
To create a configuration, you must run C: \ arduino \ eclipse \ eclipse.exe, create an empty project, copy an empty file C: \ arduino \ EATConsole \ menu.ptmenu into it and add items in the editor according to the following table. If you downloaded the project from the
repository , then there is already a prepared menu.ptmenu file in the EATConsole folder.
Type | Address | Bit | Name | Point | Slave |
Display boolean | 0 | 0 | DT0 | | one |
Display boolean | 0 | one | DT1 | | one |
Display boolean | 0 | 2 | DT2 | | one |
Display boolean | 0 | 3 | Btn | | one |
Input boolean | one | 0 | CL16 | | one |
Input boolean | one | one | CL17 | | one |
Input boolean | one | 2 | CL18 | | one |
Input boolean | one | 3 | LED | | one |
Display integer | 2 | | INPT3 | 0 | one |
Display integer | 3 | | INPT4 | 0 | one |
Display integer | four | | INPT5 | 0 | one |
Display integer | five | | HOLD6 | 0 | one |
Display integer | 6 | | HOLD7 | 0 | one |
Display integer | 7 | | HOLD8 | 0 | one |
Type - the type of the EATConsole menu item.
Address - the address of the data register.
Bit - the number of bits in the register.
Name - the name of the menu item.
Point - the number of decimal places after a point.
Slave - Modbus controller address.

Now, save and copy the menu.ptmenu file to the C: \ arduino \ EATConsole directory. To do this, right-click on the file directly in Eclipse, select the “Copy” item in the context menu, and then paste into the C: \ arduino folder in the explorer \ EATConsole.
After that, run C: \ arduino \ EATConsole \ EATConsole.exe, configure the serial connection by selecting the File \ Settings menu item, specify the port number, 9600 speed, 8 data bits, 1 stop bit in the dialog box.
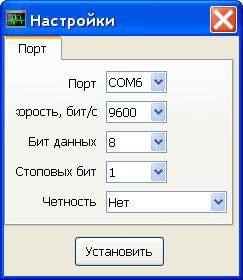
* The program works with ports 1 through 8 and if the Arduino USB adapter is on the port with a larger number, you will have to open the Windows Device Manager and change the port number for it.
When all the settings have been entered, click the “Install” button, immediately after this the program will start polling the device and if something went wrong the message “NO COMMUNICATION” will appear. If everything has been done correctly and there is a connection in what can be seen by the flashing status indicator (LED on pin 13), then it's time to start testing our controller.
Let's try to change the value in the registers HLD0 ... HLD2 and L0 ... L2, the value in the corresponding register IN0..IN2 and DT0..DT2 should change, then click on the layout button, the value in the BTN field should change, click on the LED field , at the same time the LED should light up or go out.
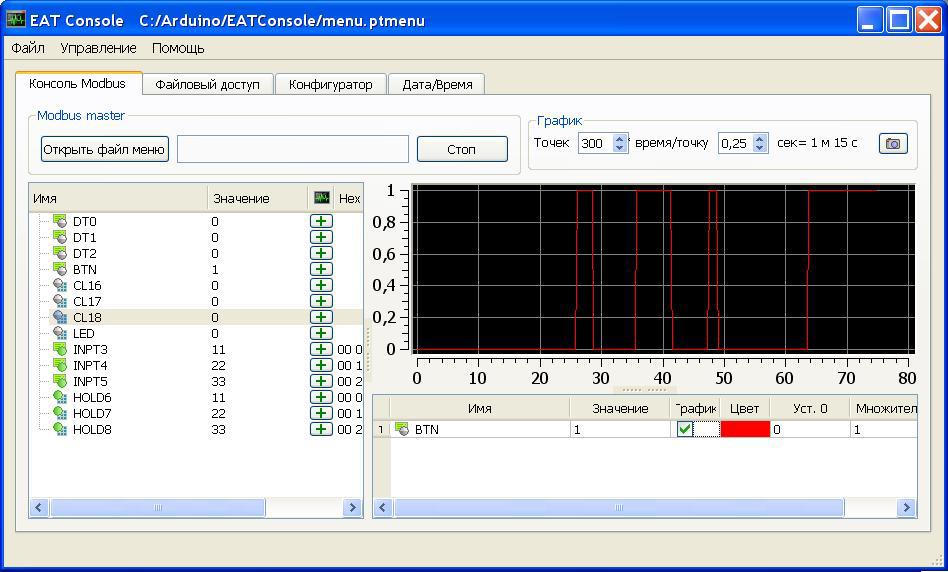
What we received as a result of our work:
1 got acquainted with the basics of the Modbus protocol;
2 created a sketch that turns the Arduino into a Modbus slave device and allows you to read and write several Modbus registers with different data types;
3 tested the exchange with the controller using the emulator of functions of the Modbus master device, for which they created a configuration corresponding to the register structure of the controller.
findings
The
Modbus-Master-Slave-for-Arduino library is easy to use, it allows you to create a Modbus slave device that works correctly with a software emulator. The compiled example takes a little more than 5kb of program memory, so there is enough space in the controller to add the necessary functionality.
The Modbus standard is open and popular, but there are a number of flaws in it - the standard defines only two types of data,
The protocol requires constant exchange between the master and the slave devices; it is necessary to configure the system manually.
With some drawbacks, the protocol is quite suitable for use in the controller of home automation systems, especially if docking with various software is necessary.
Next time, let's connect the controller to the OpenHAB platform.