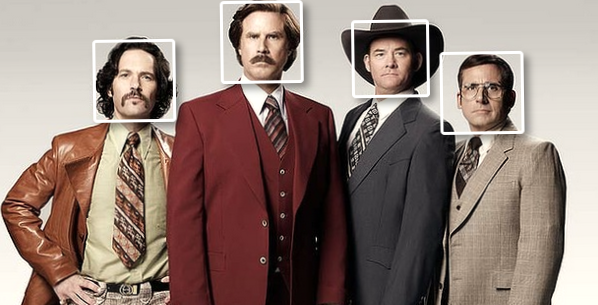
Face recognition can be useful in many situations. For example, if you need to trim the avatar and not touch the face of the user, or you want to give users a convenient way to mark their friends in the photo and video.
I will talk about how to implement the recognition of any number of faces on any photo or video in a browser using JavaScript and jQuery of the FaceDetection plugin in a few lines.
')
Who cares, I ask under the cat.
First you need to install the latest version of the FaceDetection plugin. You can do this by downloading the archive on the
official website or on
GitHub .
Installation via Bower and NPM is also available.Bower:
bower install jquery.facedetection
NPM:
npm install jquery.facedetection
After installing the plugin, you need to create an HTML page and connect jQuery and FaceDetection plugin to it:
<script src="http://code.jquery.com/jquery-1.11.1.min.js"></script> <script src="path/to/dist/jquery.facedetection.min.js"></script>
Let's try to test the plugin. To begin, move to the folder with the HTML page any image with a face (in my case it will be photo.png) and connect it to the page with the img tag:
<img id="photo" src="photo.png" alt="photo" />
Now add to the page a little (4 lines) of JavaScript code using FaceDetection for face recognition:
$('#photo').faceDetection({ complete: function (faces) { console.log(faces); }});
And look at the browser console by opening this page in it. In case of successful recognition, we will see something like this there:

Now let's take each of these points apart.
width - face width
height - face height
x - coordinates of the person along the X axis
y - coordinates of the face along the y axis
positionX - position X relative to the document
positionY - position Y relative to the document
offsetX - the position of X relative to the parent element
offsetY - the position of Y relative to the parent element
scaleX - the ratio between the real and the displayed width of the image
scaleY - the ratio between the real and the displayed height of the image
Now, based on this knowledge, we can already write code to visualize face recognition. Simply put, we will circle the faces with a colored frame.
First, add some CSS code to our HTML page to create a frame:
.face { position: absolute; border: 4px solid white; }
If necessary, you can add the border-radius parameter to the CSS, which will make a round frame out of a square frame, since faces are more often round than square. I’ll do without it at this stage.
Now let's improve our JavaScript code, make it so that it displays the frame exactly where the face is in the photo.
$('#photo').faceDetection({ complete: function (faces) { console.log(faces); for (var i = 0; i < faces.length; i++) { $('<div>', { 'class': 'face', 'css': { 'position': 'absolute', 'left': faces[i].x * faces[i].scaleX + 'px', 'top': faces[i].y * faces[i].scaleY + 'px', 'width': faces[i].width * faces[i].scaleX + 'px', 'height': faces[i].height * faces[i].scaleY + 'px' } }) .insertAfter(this); } }, error:function (code, message) { alert('Error: ' + message); });
Save the HTML page and run in the browser. As a result, you should get a photo in which the face is surrounded by a white frame.
That's what happened with me:
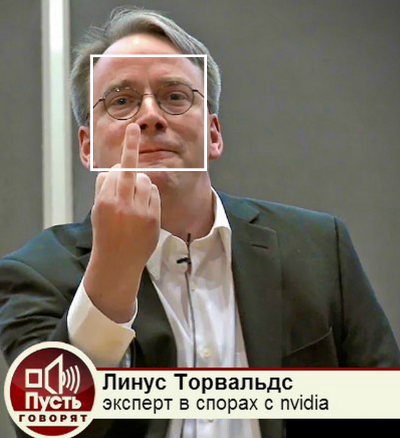
That's all, the simplest face recognition application is ready.
Tip: reduce and shrink images before recognition starts, this will speed up the recognition process and reduce the risk of the user's browser hanging.
Face recognition on video is done in much the same way, only instead of the image you add video to the page.
I must also say that sometimes recognition does not work correctly. In any case, you can try to correct errors in the plugin or algorithm, the source code is available on GitHub.
I hope this article was able to help you in real projects or was just useful. Thanks for reading,% username%!