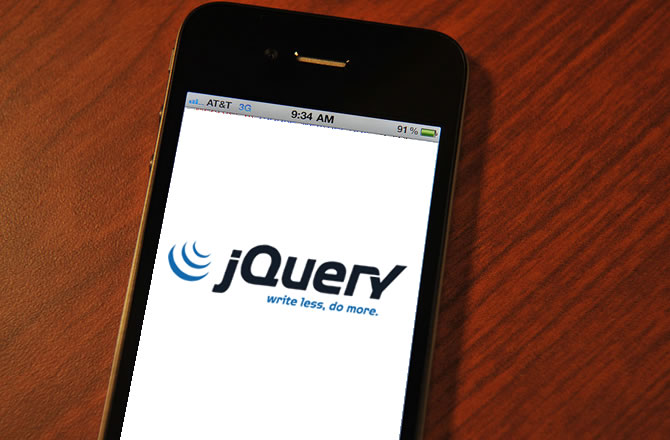
This is a rather loose translation of an
article that caught me on the Internet. Its author is TJ VanToll. He has been involved in web development for many years and, in particular, in optimizing websites for better performance on mobile phones. Under the cat reviewed several ways to optimize, as well as the results of testing on various devices.
Should I use jQuery for mobile devices?
I am often asked this question, but for all this time not a single study has been conducted that could explain it. The jQuery library is often said to be “big” and “bloated”, but these concepts are relative, they prove nothing without experimental confirmation. It would be correct to ask this question like this: “Do jQuery dimensions affect so much that we can refuse to use this library at the expense of functionality?”. To answer this question, we must determine the speed of loading the library on a mobile device. This article is devoted just to this issue, but from the beginning we need to understand what is happening inside the <script> tag.
<Script> tag
<script src="jQuery.js"></script>
We all have seen this code a large number of times, but what happens in the browser when it sees this line? In fact, many events occur, but we are only interested in the two most important processes.
- Getting the file (there are two options: from the cache or from the network);
- Parsing and executing the script.
Let's break down each of these items separately.
')
JQuery loading
For the best result, we will make an assumption that the user gets to your site for the first time and, accordingly, he does not have any data in the cache. When the browser does not have data in the cache, it is forced to download all the files from the server. Let's try to measure the time of such a load, it is influenced by two factors: throughput and delay.
Bandwidth.
Bandwidth is the speed with which the browser can download a file from the server, most often measured in Mbps.
In 2012, the average speed of mobile networks around the world ranged from ~ 2 Mbit in some Asian countries to ~ 1 Mbit in Russia and India. Of course, this data is a bit outdated, new networks promise greater speed, for example:
- Virgin Mobile claims that its 4G network has a download speed of 3-6 Mbit;
- Verizon Wireless claims its 4G network has a download speed of 5-12 Mbit;
- LTE networks offer speeds of ~ 6.5 Mbit in the USA and ~ 24.5 Mbit in Australia.
Since this article is about jQuery, let's use these numbers to determine how long jQuery loads. We are based on the compressed version of the library 2.1.0, which weighs 28.65 KB.
A network with a download speed of 1 Mb can download 125 KB per second, which means that in one second you can download jQuery 4.36 times. Or more specifically:
- 229ms to load at the worst speed (1 Mbps);
- 46ms to download at medium speed (5 Mbps)
- 19ms to download at high speed (12 Mbps).
Later we will return to this calculation, but, you see, is this more or less specific information? But in fact, network bandwidth is not the main problem of mobile web performance. For example, Ilya Grigorik (Google) noted that when switching from a network at a speed of 5 Mbit to a network at a speed of 10 Mbit, the download time is reduced by only 5%.
So, we found out that network bandwidth is not a bottleneck, then what is the reason?
Delay
In the context of web applications, latency is the time it takes to connect to the server. Delay is usually measured in RTT (Round Trip Time) - this is the time during which the signal reaches the server and is returned to the client.
Historically, we didn’t worry too much about the delay time on personal computers, since RTT is short, about 50ms. But with mobile phones, we were less fortunate. In 2012, the average time for RTT mobile networks in the United States was about 344ms. And this time delay is not only HTTP requests, which now amounts to 93ms, but also each DNS and TCP request.
Now the average RTT has improved slightly. Virgin Mobile announces that the average delay on the 4G network is now ~ 150ms. So far, the average latency is improving, but there is a problem with the final limit of the transmission rate, which does not allow us to minimize the delay.
But in all this there is a bright side. Remember, we talked about the fact that there is an easy way to avoid big delays when using jQuery: instead of loading the library with a separate file, attach the contents to your script file and load them together! As shown below:
<!-- Before --> <script src="jQuery.js"></script> <script src="app.js"></script> <!-- After --> <script src="app.js"></script>
This simple change negates the delay that occurs when you separately load jQuery into your application. The same can be done when loading a library from an external CDN. Because of the fragmentation of CDN providers, the chances of using the CDN cache are low — and downloading from the server can be done in several steps (DNS lookup, TCP connection, and sending an HTTP GET request).
Previously, we calculated the time required to download the jQuery file from the web. It turns out that we can download the file in 50ms, using medium quality networks, if we avoid delays by loading the combined JavaScript file. But, downloading the file, the browser processes it. Let's look at this point in more detail.
Parsing and Script Execution
After the browser has downloaded the file, it must turn the JavaScript code into bytecode and execute it. This process is quite complex and if we try to examine it in detail, we will go far beyond the scope of this article; we are interested in another.
So how long does this process take?
I wrote a simple test to find out how long it takes to parse and execute the script.
<script>var start = new Date();</script> <script> </script> <script>alert( new Date() - start );</script>
I experimented with console.time () and console.timeEnd (), but didn’t use them because they are not supported in older mobile browsers. In browsers that supported these methods, I got an almost identical result proposed earlier.
In order to allocate the time of parsing and execution, I wrote jQuery as an inline script (i.e. directly inserted code in the html markup). The execution results in various browsers are shown below.
Browser | OS | JQuery parsing / execution time 2.1.0 (in ms) |
---|
IE 11 | Windows 8.1 | 18, 21, 14, 16, 15 |
Chrome 33 | OS X 10.9.2 | 15, 8, 5, 7, 6 |
Safari 7 | OS X 10.9.2 | 9, 5, 3, 3, 2 |
Firefox 27 | OS X 10.9.2 | 12, 12, 11, 12, 12 |
Safari | iOS 7 | 178, 125, 44, 87, 96 |
Default Android | Android 2.2 | 1184, 268, 299, 216, 422 |
Default Android | Android 4.0 | 603, 465, 106, 145, 243 |
Chrome 33 | Android 4.4 | 219, 86, 38, 86, 123 |
Firefox 27 | Android 4.4 | 269, 367, 400, 381, 264 |
Tests of the desktop browser were carried out on MacBook Pro, I conducted tests of mobile browsers on improvised devices. I did not clear the cache browser between downloads, I just clicked the Refresh button several times. This led to interesting results: WebKit browsers (Chrome, Safari) when loading for the first time save data to the cache and speed on subsequent downloads is significantly higher, while Trident (IE) and Gecko (FireFox) browsers do not do this. If any developers of these engines read this article, I would like to know the details of the parsing in more detail. Also, if you have other suggestions how to calculate the time, please share them in the comments.
All desktop browsers - IE, Chrome, Safari and Firefox - easily coped with this task. Even IE showed a pretty good average result ~ 17ms.
But mobile browsers showed a completely different result. While Mobile Safari on iOS7 and Chrome on Android handled this task with dignity. The standard browser on Android showed very poor results. My first run of this test on my Android 2.2 took a full second. And although I did not have a wide variety of devices for testing, I suspect that on many other devices the results will be even worse.
In my opinion, such slow parsing and script execution on mobile browsers, especially on standard Android browsers, is a big plus for those who prefer to use small JavaScript libraries instead of jQuery.
However, in our test can be traced and good points. Here there is Moore's law, the difference between Android 2.2 and Android 4.0 is clearly noticeable, and most likely this trend will continue.
To summarize, is the size of jQuery so terrible as they say about it?
So, we reviewed all the necessary indicators that affect the download speed, it's time to answer this question. Like any answer to a software development question, our answer depends on the specific situation. Let's start with a summary of all the data.
JQuery 2.1.0 load time of 28.56KB in size takes:
- 229ms when downloading in bad networks at a speed of 1Mbps;
- 46ms when downloading in normal networks at a speed of 5Mbps;
- 19ms when downloading in good networks at 12Mbps.
The delay time when loading jQuery 2.1.0 is:
- 0ms if you put the contents of the library into your script file;
- 150-1000ms if you download the library as a separate file.
Parsing / Execution jQuery 2.1.0 takes:
- 15-20ms for desktop browsers;
- ~ 270ms average load time on all mobile browsers studied.
These numbers make it clear that the old versions of browsers on Android 2.2 can add us an extra second to download. This must be taken into account when developing, relying on the target audience, since This may adversely affect performance.
I recommend that you study statistics and know your audience. If the percentage of visits from older mobile browsers is high, you should probably stop using jQuery. But keep in mind that the point here is no longer in the library itself, but in the processing time of the JavaScript code. It all depends on the specific application, it may turn out that jQuery will load faster than the code you write, especially this library saves you time. In the end, the motto jQuery - write less, do more.
But let's go back to the numbers. From the above data, it follows that on average, loading jQuery from the network will take ~ 50ms, plus parsing time ~ 250ms. Let's prove that time is money. Consider for example Amazon. They claim that because of every 100ms of delay when loading a page on amazon.com, they lose 1% of sales. It is easy to calculate that the connected jQuery library costs them 3% of sales. This sounds awful, but you should consider the positive aspects, because using jQuery improves the performance and functionality of the site, sufficient to cover the 3 percent loss of sales.
It is also necessary to understand that the library consists of modules. Therefore, if you are not satisfied with the download speed, you can easily remove unnecessary modules, leaving only the necessary ones. But before doing this, let's consider other problems.
You probably have many more ways to increase the efficiency of your applications.
According to the
HTTP archive , the average webpage is:
- Weighs more than 1.7MB;
- Creates more than 90 HTTP requests;
- It has more than 275KB JavaScript scripts;
- Creates 17 HTTP requests for one JavaScript only;
- Includes 1MB images;
- Only 46% of the page is cached.
Therefore, first of all it is necessary to pay attention to the following things.
- Need to minimize CSS / JS;
- Merge all CSS / JS files into one;
- Use special tools for compressing HTML / CSS / JS documents;
- Compress images;
- Allow the browser to save files to the cache;
- Remove unnecessary HTTP requests.
Often, by deleting several images, you can achieve much better results than by deleting jQuery. If you’ve done some optimization, but still have performance issues on mobile devices, and are thinking about removing jQuery — just check to see if you’ve done everything you can or are there other ways?