In one of the projects I was faced with the fact that the application needed to display lists of results for various queries (search by words, dates, tags, etc.). Since the lists were repeated in different Activities, the most obvious decision was to use fragments, and specifically create your own ListFragment class and reuse it in the project.

ListFragment is designed to display various lists and is notable for the fact that it has its own fields and methods for working with a list, as well as XML markup with a minimal set of views. Thanks to all this, we can not even create our own XML markup for the fragment using the standard one.
')
Before we start working with ListFragment, let's examine a little what is inside?
The default markup is from ~ \ sdk \ platforms \ android-XX \ data \ res \ layout \ list-content.xml
Some views of this markup have identifiers, thanks to which we can customize the parent container, the list and the text of the empty list.
If we run through the sources of the ListFragment class, we will see:
View mListContainer;
So, create our class:
import android.app.ListFragment; public class MyListFragment extends ListFragment{ }
And include it in the markup of our Activity (or you can install the fragment programmatically)
<fragment android:name="**.*******.********.MyListFragment" android:layout_width="match_parent" android:layout_height="match_parent"> </fragment>
Run the application, go to our Activity and immediately see the download animation:

Now, in order to hide the animation and display the list, we just need to install the adapter by calling the setAdapter method (ListAdapter adapter). In this project, I used the Loader in the Activity and OttoBus event, but there are plenty of options to transfer the list object to the fragment, so please do not pay attention to the method itself.
@Subscribe public void onMyEvent(MyEvent loaderEvent) throws InterruptedException { String[] listItems = {"item 1", "item 2", "item 3","item 4"};
Immediately after installing the adapter, the animation will disappear and we will see:
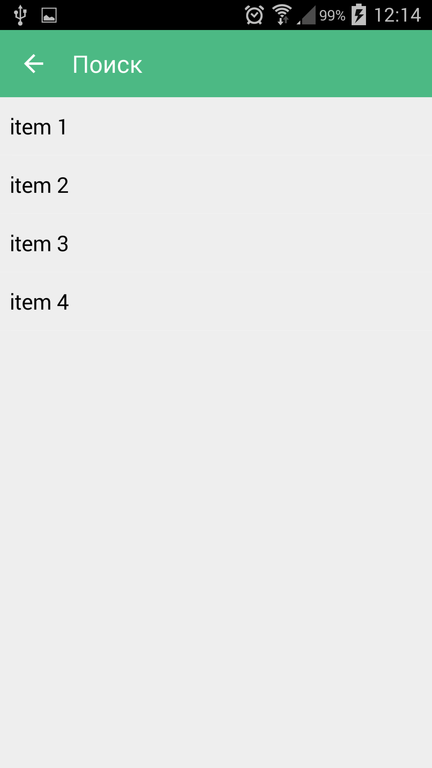
And if our list suddenly turns out to be empty, we will get another screen:

To set your empty text, use the method:
setEmptyText(getResources().getString(R.string.error));
The ListFragment class also has a list click processing method, which we can override
@Override public void onListItemClick(ListView l, View v, int position, long id) {
You can not use the standard markup, and set your own, but do not forget to specify the correct identifiers for your views.
Total ListFragment has its own ideas and logic of work.
I hope this article will allow you to accept to assess whether you have enough built-in implementation or whether you need to write your own.
PS Personally, I liked the simplicity and speed of implementation in the project, where I was able to successfully use one fragment class at once in 4 different Activities.
Pros:
* It is not necessary to create XML markup.
* No need to declare presentations in your class.
* No need to implement the logic of showing / hiding animation, list and empty-text.
* No need to install "listener"
* Less code.
Minuses:
* You need to learn the methods of the ListFragment class and how everything works.
* You can not change the built-in animation, even through its own markup.
* You can not override the logic of hiding / showing animation, list and empty-text.
PS Join the
chat Russian-speaking Android developers .