If you are developing HTML and JavaScript games, then this article is for you. We have already written a lot about the fact that under Windows 8.x you can develop applications on HTML / JS, and, as a rule, you can easily just take and use your current engine that works in modern browsers.
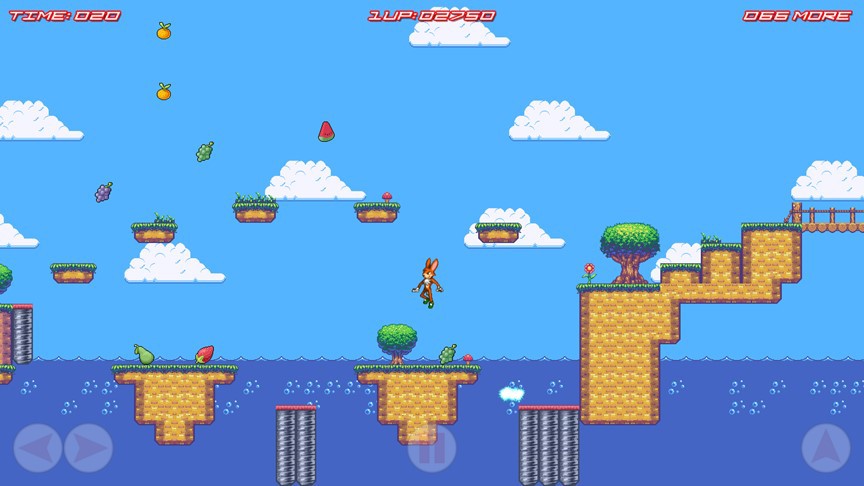
Just as an example: if you make a platformer, then you can use such an engine as Phaser (by the way, it supports TypeScript development!), Or our Platformer Game StarterKit for Windows 8 . By the way, if you want to make a toy in the genre of Tower Defense, then we have another Starter Kit . And if you want to create something three-dimensional using WebGL , then our all for you is Babylon.js .
')
Gamepad
But in this article I will not tell you how to create the game itself. We will ask another question: how to connect to the game for Windows 8.x or in the browser gamepad? For example, a game controller from Xbox 360 or Xbox One:
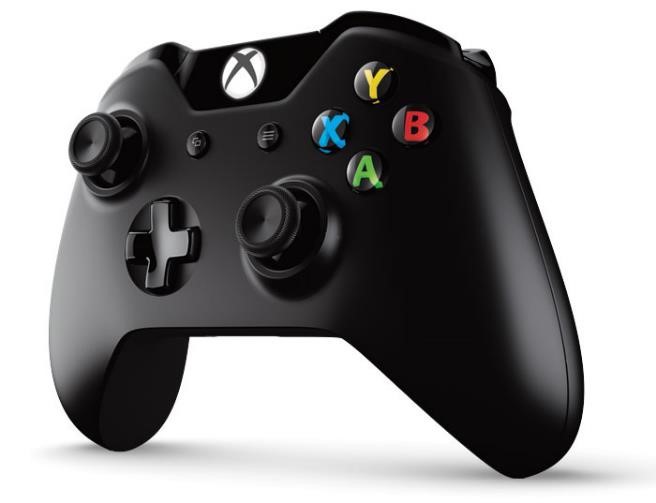
We assume that you have already connected the gamepad itself to your PC (
instructions for Xbox 360 ,
instructions for Xbox One ). Now let's see what you need to do to add his support in your game.
As an example, I will use
the RubbaRabbit platformer from the above start-whale. We will consider two options: a game for Windows 8.x and a game in the browser.
Game for Windows 8.x on HTML and JavaScript
To add gamepad support to the JavaScript game under Windows 8.x, you will need to learn how to work with
XInput interfaces. This may sound scary, because for this you need to dive into C ++ code, but we have already done almost everything that you need in order not to intersect with them directly.
To work, you need to download the sample
XInput and JavaScript controller sketch . Inside it, you can easily find a daddy with C ++ code in which there is a binding library project on XInput, with which you, in turn, can work in your game on JavaScript.
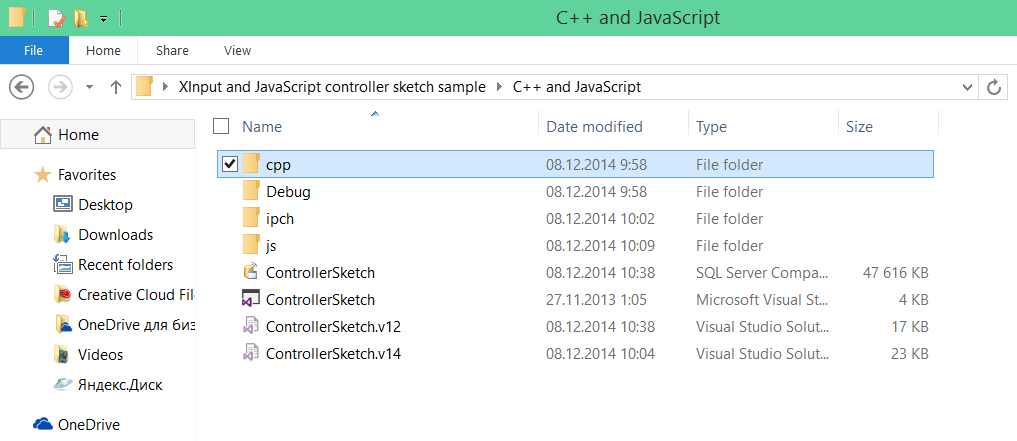
This project you need to add to your solution with the game and add a link to it inside the project for Windows 8.x:

If you wish, you can get inside the files in C ++ and find out that they actually put out a very simple interface for accessing the controller: the designer to get the link to the gamepad and the
getState function to get its current state with the projection on the Xbox gamepad buttons.
Now you need to add support for the gamepad in the game itself. For this, usually, you need to make quite small changes in the processing of input by the player.
Game cycle
As a rule, inside the game code you have a regular cycle, within which each next frame of the game is formed. In order to understand how the game situation has changed, you in particular check whether the user has pressed certain buttons.
In my case, the game loop is set inside the
update function:
this.update = function () { ... }
Somewhere inside this function, there is a button click processing that looks something like this:
if (touchleft || jaws.pressed("left") || jaws.pressed("a")) { player.vx = -move_speed; player.flipped = 1; } else if (touchright || jaws.pressed("right") || jaws.pressed("d")) { player.vx = +move_speed; player.flipped = 0; } if (!player.attacking && (touchjump || jaws.pressed("up") || jaws.pressed("w") || jaws.pressed("space"))) { if (!player.jumping && player.can_jump) { sfxjump(); player.vy = jump_strength; player.jumping = true; player.can_jump = false; } } else { player.can_jump = true; }
As you can see, the developers of the engine have already taken care of that the user can transmit commands not only from different buttons from the keyboard, but also, for example, from virtual buttons on a touch device (
touchleft ,
touchright , etc.).
In general, most likely, in your code there should be some variables that correspond to different “game actions” and accumulate various input methods for their activation. It is to work with such variables that the addition of support for the gamepad will be tied.
Gamepad support
To add support for the gamepad, there are quite a few left: you need to initialize the work with the controller when the toy starts and then regularly receive its status.
To initialize the controller, we create the
Controller object through the constructor, accessible to us from the previously connected C ++ library:
Next, we need to update the state of the controller with a certain periodicity. To do this, we will describe the
updateState function, which will request to call itself for each animation frame:
This is all (!) Code that you need to add to the toy so that it learns how to interact with the Xbox 360 gamepad. Do not forget to call the
initXboxpad function itself at the start.
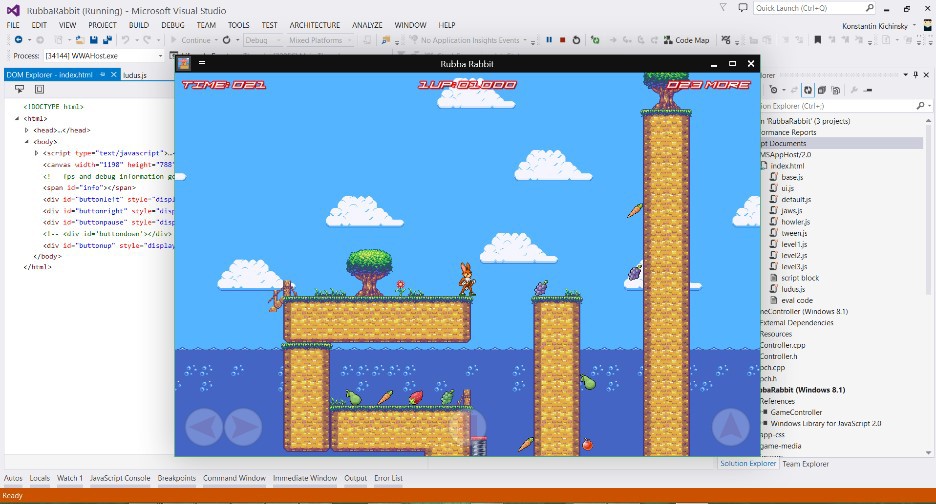
Please note that in this case we “exploit” the existing variables, accumulating commands from a possible touch interface, and update them depending on the current state of the controls on the gamepad. For example, if a player has pressed the “A” button, then the corresponding
state is. a will be
true and we project it on the “jump” in the game.
By the way, through the gamepad's
setState function, you can make it vibrate.
Browser game
Now let's see what we can do in the browser. For browsers, the W3C develops a special standard
Gamepad API that will allow you to work consistently with different types of gamepads.
The standard assumes that there is some “common model” to which various game controllers can be reduced:

If you want to bind the notation to a specific type of device (for example, an Xbox gamepad), you will need to do it yourself by writing the appropriate mapping in your code.
I will not dive into the standard itself in this article, since there are already a sufficient number of review articles on the Internet. For example, here is the
documentation from Mozilla . The only thing I want to mention here is that it is outdated regarding the assumption that the Gamepad API does not support Internet Explorer.
In fact , in the
fresh builds of Internet Explorer 11, the
Gamepad API is already
supported .
In the context of this article about the standard, you need to know only one thing: it provides access to raw data, broken down along axes and numbered buttons. To understand the real match, you will need to do certain mental operations in your code.
Game cycle and game code
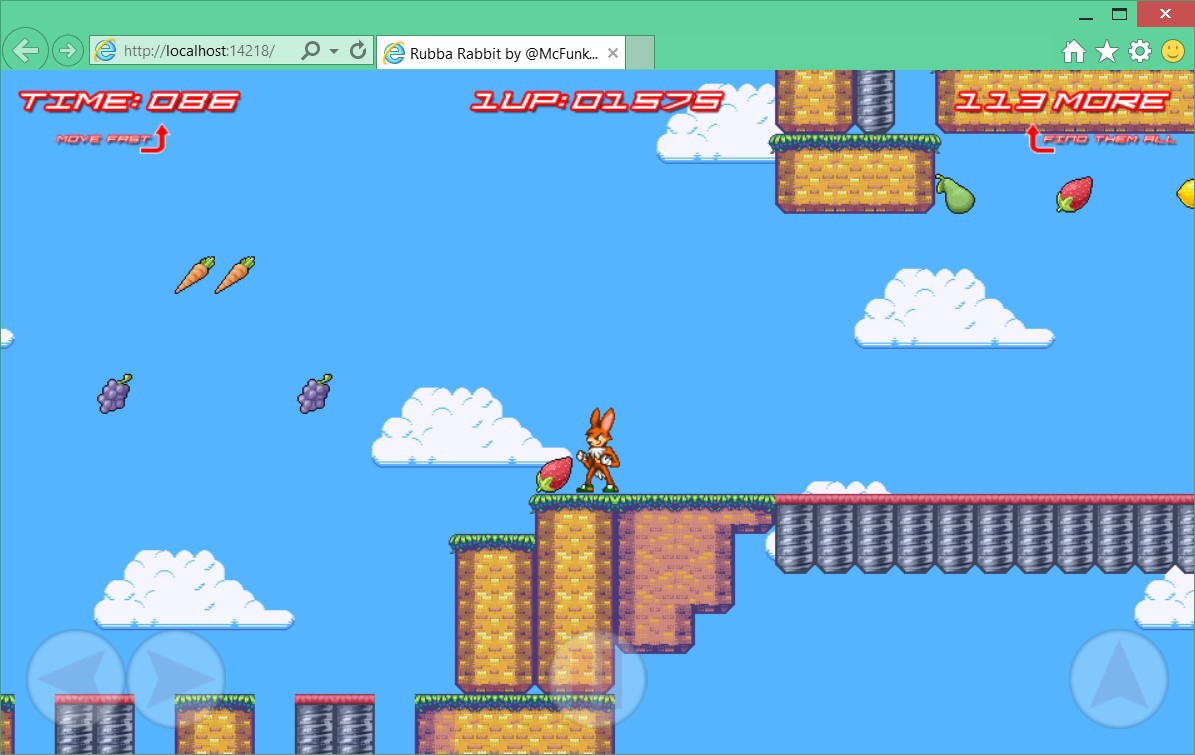
For the demonstration, I continue to use the same example from the starter whale, only this time I create an empty web project and copy the original source files into it. Since they do not really have any strings on the Windows platform, the game simply works in the browser:

Everything else remains unchanged, and the same assumptions about the game cycle that we made for the project under Windows 8 are true.
Gamepad support
To simplify my work with the gamepad, I will use the ready-made code from the Babylon.js library -
babylon.gamepads.ts (GitHub). You can simply copy the library yourself or fork it.
The library does several important things:
- is able to simulate an event model over the Gamepad API (if you suddenly need it);
- simplifies access to individual elements of the controller (for example, it combines the axes of the joystick into one object);
- distinguishes between the Xbox controller, making it necessary for me to display generalized buttons on specific ones.
Please note that the library is written in TypeScript. Nearby you can also find a compiled version for javascript. In my case, I just add the library inside the project, Visual Studio includes TypeScript support and automatically generates js-files when saving.
Do not forget to connect the library to the page with the game:
<script src="js/babylon.gamepads.js"></script>
Further, the connection scheme of the gamepad is very similar to what we did in the case of Windows 8.x:
Inside the BABYLON.Gamepads function, an event handler for connecting a gamepad to the computer is passed. As you can see, adding a gamepad support to the project is about 20 lines of code!
Option code with event model:
As a result, we can easily control the actions of the hero in the game directly from the connected gamepad:

useful links