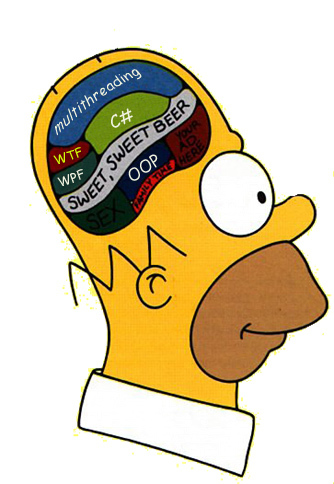
During the preparation for the exam, number 70-483 found many disparate sites with various links to manuals that helped me a little. But what helped me more is the fact that I have compiled for myself a memo on several pages, extracts from which I want to share.
The goal is not a detailed description of C #, the goal is to refresh and focus on some of the necessary topics. If any topics are unfamiliar to you, it means that you have spaces that need to be eliminated.
I cannot disclose the testing questions (and I don’t remember them already), but if many of the nuances, tricks and subtleties listed below help you, then you shouldn’t be offended (written with irony).
Let's start with simple things that are often forgotten, but which are often used in tests:
In order to declare a variable of type int, and assign the value 0 to it immediately, you can do this: var myInt = 0; or so int myInt = 0;
In order to declare a variable of type int, and set the default value to it immediately, you need to do this: int myInt = new int ();
Explicit and Implicit typingint i = 42;
Use implicit typing when:
Anonymous types - var anonymousType = new {Name = “Alex”};
Getting the result of a LINQ query - var queryExpression = from c in customers where c. Name == "Andrey" select c;
Using complex generic types - var searchList = new Dictionary ();
Implicit typing is possible only for local variables.
Boxing / unboxingBoxing is the conversion of a value type to a reference type.
Unboxing, on the contrary, converts a reference type into value. For Unboxing, Cast is required.
Boxing / Unboxing copies the value of a variable.
Boxing in terms of computation speed is an operation that takes up quite a lot of processor resources.
A simple example:
int i = 123;
An interesting example of an error:
double e = 2.718281828459045; object o = e;
Another example:
int count=1; object countObject=count;
Operator
?? called the
null-coalescing operator . It returns the left value if it is not null, otherwise the right value. Example:
int? x = null;
The
?: Operator is called the
conditional operator . It returns one of two values ​​based on a boolean expression. If the expression is true, then the first value is returned, otherwise the second one. For example 2 similar code:
if (input<0)
LINQAny object that implements an IEnumerable or IQueryable interface can be queried using LINQ.
You can use both orderby ... descending and OrderByDescending
orderby h.State, h.City - here orderby is written in one word, however group h by h.State is separate (there is an option to write in capital letters GroupBy)
Predicate is a condition that characterizes a subject. For example:
where i % 2 == 0
There are 2 options for writing LINQ (the syntax of the / method method and the syntax of the query / query):
var data=Enumerable.Range(1,100); var method=data.Where(x=>x%2==0).Select(x=>x.ToString()); var query=from d in data where d%2==0 select d.ToString();
Simple LINQ methods:
var values=new[]{“A”,”B”,”C”,”B”,”B”,”A”,”B”}; var distinct=values.Distinct();
It’s interesting to use where instead of && several times. For example:
var evenNumbers = from i in myArray where i % 2 == 0 where i > 5 select i;
You can use functions as a filter. For example:
var evenNumbers = from i in myArray where IsEvenAndGT5(i) select i; static bool IsEvenAndGT5(int i) { return (i % 2 == 0 && i > 5); }
If you need to select multiple values, you must use new {}
var names = from p in people select new { p.FirstName, p.LastName };
Moreover, you can even set pseudonyms:
var names = from p in people select new { First = p.FirstName, Last = p.LastName }; var names = people.Select(p => new { p.FirstName, p.LastName });
LINQ deferred execution - until the element is invoked, the request is not executed. Example:
int[] myArray = new int[10] { 1, 2, 3, 4, 5, 6, 7, 8, 9, 10 }; var evenNumbers = from i in myArray where i % 2 == 0 select i; foreach (int i in evenNumbers) { Console.WriteLine(i);
The build version consists of four parts:
Major, Minor, Build, and Revision')
To install the assembly in the
GAC (Global Assembly Cache) you need the Global Assembly Cache tool (Gacutil.exe). At the command prompt, enter the command: gacutil –i
But it is necessary that the assembly be signed with the strong name key using the SN tool.
Example of creating a key: “C: \ GACKey \ GACkey.snk” sn –k
Building with a strong name (strong name) consists of 5 parts:
Friendly Name, Version, Culture, Public Key Token, and Processor ArchitectureWhen signing your builds, keep the key in a safe place, and give the developers an open part of the key, which allows them to use the builds without a full signature.
Select the open part of the key by the following command:
Sn.exe –p "key.snk" "public key.snk"
Since the build version is important at the moment when the runtime is trying to find the build, you can publish different versions of the same build to the GAC and avoid problems that happen with regular dll.
This is called side-by-side hosting, in which many versions of the same assembly are hosted simultaneously on the same computer (starting with .Net 4).
Shallow copy - a trap when copying arraysWhen copying objects of a reference type, a pointer to the value can be copied, rather than the value itself. Example:
Person[] orginal = new Person[1]; orginal[0] = new Person() { Name = "John" }; Person[] clone = (Person[])orginal.Clone(); clone[0].Name = "Mary";
Closures var funcs = new List<Func>(); for (int i = 0; i < 3; i++) { funcs.Add(() => i); } foreach (var f in funcs) Console.WriteLine(f());
This result is due to two reasons:
1. during closures, variables are captured, not variable values ​​and
2. in the above code snippet, there is one instance of the variable i, which changes on each iteration of the loop, and does not create a new instance on each iteration.
To fix this situation is quite simple:
var funcs = new List<Func>(); for (int i = 0; i < 3; ++i) { int tmp = i;
Do you use compiler directives? Here are a couple of example guidelines:
#region and #endregion - limit some part of the code and allow folding / unrolling
#define and #undef - define set true or false according to some values.
#error - generates an error
An example of a condition that if the assembly in the DEBUG mode executes one piece of code, and otherwise the other:
#if DEBUG
#elif
#endif
What is PCL? Portable Component Library is essentially a dll, but with cross platform capabilities. That is, you can create PCL and assign the platforms in which it will be used (for example: WP8, WinRT, Xamarin ...). In essence, adding each platform narrows the build
PDB is an acronym for Program database file.
The PDB contains debugging and project status information to enable sequential layout of the debug configuration of the program.
Anyone with access to the dll / exe file can easily perform reverse engineering in order to generate source code with or without a PDB, using tools such as, for example, reflector. So, providing or not providing PDB is in no way affected by the security code.
Implicit and Explicit interface implementationsSuppose we have 2 interfaces with the same method:
interface InterfaceOne { void InterfaceMethod(); } interface InterfaceTwo { void InterfaceMethod(); }
We create a class inherited from two interfaces and declare the interfaceMethod method in it:
public class MyClass : InterfaceOne, InterfaceTwo { public void InterfaceMethod() { Console.WriteLine(" ?"); } }
Now we used the Implicit interface implementation.
And if we specify the interface name, it will be an Explicit implementation:
public class MyClass : InterfaceOne, InterfaceTwo { void InterfaceOne.InterfaceMethod() { Console.WriteLine(" , ?"); } void InterfaceTwo.InterfaceMethod() { Console.WriteLine(" , ?"); } }
In computer science, reflection or
reflection (synonym introspection,
reflection ) means a process during which a program can monitor and modify its own structure and behavior at run time.
Example:
void Method() { var horse=new Animal(); var type=horse.GetType(); var method=type.GetMethod(“Speak”); var value=(string)method.Invoke(horse,null);
With reflection, the assembly can be loaded in various ways:
Assembly.LoadFrom () can redirect to another similar assembly
Assembly.LoadFile () loads the assembly specifically from the file.
Assembly.Load () loads the assembly by specifying its full name.
The difference between the
Convert and
Parse methods is that Parse accepts only a string as an input value, while Convert can accept other data types.
The difference between Parse / TryParse and Convert is that Convert can take null values ​​and does not throw an ArgumentNullException.
There are two special constants for representing positive and negative infinity in C #:
System.Double.NegativeInfinity and
System.Double.PositiveInfinityNegativeInfinity - The value of this constant is the result of dividing a negative number by zero; this constant is also returned if the result of the operation is less than the minimum value (MinValue).
PositiveInfinity is the result of dividing a positive number by 0. The constant is returned if the value is greater than MaxValue.
Infinity includes both NegativeInfinity and PositiveInfinity
The
unchecked keyword is used to suppress overflow checking when performing arithmetic operations and conversions with integer data. If the unchecked environment is removed, a compilation error occurs. 2 examples:
unchecked { int1 = 2147483647 + 10; } int1 = unchecked(2147483647 + 10);
By default, C # does not throw an exception if an error with int and float types occurs during a narrowing / narrowing conversion.
In order for the exception to occur, you can use the keyword
checked . Example:
checked { int big = 1000000; short small = (short)big; }
You can also check in the properties of the project about the occurrence of an exception during overflow / underflow
Formatting Console.WriteLine(somevariable.ToString("c"))
Rethrow exceptionThe error stack is cleared every time an error is thrown.
static string ReadAFile(string fileName) { string result = string.Empty; try { result = File.ReadAllLines(fileName); } catch(Exception ex) { throw ex;
It is recommended to use just throw, since in this case information about the original error will be saved.
As an option, you can create your own error -
custom exception [Serializable()] public class YourCustomException : System.Exception {
And then you can throw an error:
try { throw new YourCustomException(" ");
Do not inherit from System.ApplicationException. The idea was that all C # runtime errors should inherit from System.Exception, and all custom exceptions from System.ApplicationException. But in reality, the System.ApplicationException class is not used at all.
The
try / catch / finally - finally
block always runs. Only in the case of a call in the Environment.FailFast (String) or FailFast (String, Exception) code, which interrupt the current process, in case of any critical damage to the application, the program will be terminated and finally will not be executed.
CodeDOM is a set of classes that allow you to generate code.
The System.CodeDom.CodeCompileUnit class is a top-level class, a container for all objects in the generated class.
The System.CodeDom.CodeDOMProvider class generates a file class in C #, VB, or JScript.
Structures like classes can have methods, properties, constructors, etc.
Structures, unlike classes, cannot contain destructors (in fact, destructors are Finalize methods ~ Name of the Class that allow you to destroy an object)
You cannot declare an empty constructor for a structure.
Also, structures cannot have inheritance hierarchies (to save memory). In C #, the structures are sealed - implicitly sealed. Which means that you cannot inherit from a structure.
Structures is the value data type = stored on the stack.
Classes are the reference data type = stored on the heap.
In C #, the Value data type includes structures and enumerations (structs and enumerations).
Structures, in turn, are divided into subcategories: numeric type (integer - integral types, floating-point types, 128-bit decimals), boolean type (bool) and custom structures.
An enumeration is a type set declared using the enum keyword.
The data type value has limitations. You cannot inherit from the value type, and also the value type cannot contain the value null.
enum Months {Jan, Feb, Mar, Apr, May, Jun, Jul, Aug, Sept, Oct, Nov, Dec};
Class
Interlocked - Provides atomic operations for variables shared by multiple threads (operations Decrement (Int), Increment (Int), Add (Int, Int)) The value of the variable will be changed simultaneously for all threads.
The CompareExchange method compares two values ​​for equality and, if they are equal, replaces one of the values.
Interlocked.CompareExchange(ref value, newvalue, comparevalue)
Exchange method - sets a variable with the specified value - as an atomic operation and returns the initial value. Example:
if (Interlocked.Exchange(ref isInUse, 1) ==0){
Parameters passed to functions static int CalculateBMI(int weight, int height) { return (weight * 703) / (height * height); }
By default, the function is called like this:
CalculateBMI (123, 64);If you do not remember the order, you can call the function by specifying the name:
CalculateBMI(weight: 123, height: 64); CalculateBMI(height: 64, weight: 123);
You can first specify the parameter in order, and then by name
CalculateBMI(123, height: 64);
But you can not specify the first parameter by name, and then in order (will cause an error)
You can specify values ​​for the default parameters and call the function after the function without specifying these parameters:
public void ExampleMethod(int required, string optionalstr = "default string", int optionalint = 10)
Using StringWriter and StringReader to Write and Read Strings StringWriter strwtr = new StringWriter(); for(int i=0; i < 10; i++) strwtr.WriteLine("This is i: " + i); StringReader strrdr = new StringReader(strwtr.ToString());
Generics versus ArrayList var objects=new ArrayList(); objects.Add(1); objects.Add(2); objects.Add(“three”);
Regex (regular expressions)IsMatch example:
if (Regex.IsMatch(phone, @"^\d{3}-\d{4}$"))
Matches - Looks for all occurrences of a regular expression in the input string and returns all matches:
string pattern = @"\b\w+es\b"; Regex rgx = new Regex(pattern); string sentence = "Who writes these notes?"; foreach (Match match in rgx.Matches(sentence)) Console.WriteLine("Found '{0}' at position {1}", match.Value, match.Index);
Match - works exactly the same as Matches, but only searches for the first match.
Split - Splits the input string into an array of substrings at the positions specified by the regular expression match.
string input = "plum-pear";
string pattern = "(-)";
string[] substrings = Regex.Split(input, pattern);
Replace - In the specified input string replaces all the lines that match the regular expression pattern specified by the replacement string.
^ - beginning of line $ - end of line. - any character
* - repeating the previous 0 or several times
+ - repeat the previous 1 or several times
? - repeat the previous 0 or 1 time
[abc] - some of the characters a, b or c [^ abc] - any of the characters except these [az] - the characters from and to [^ az] - the characters except from and to
{n} - match n repetitions {n,} - match from n to infinity {n, m} - match from n and up to m times
\ d - number \ D - not number \ s - space, tab, etc. \ S - no space
Some "bad" advice
Despite the ban on the dissemination of information about the exam, it is quite possible to find dumps (information plums on questions) in particular for this exam when searching the network. Is it worth using them and how much can they help in passing the exam? Even judging by the blog entries, many use them.
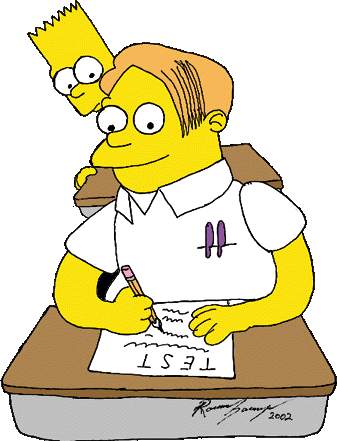
In my opinion, do not dwell on the dumps. For example, because they contain a lot of mistakes. Also, it should be noted that the questions are replaced from time to time. Is it possible to cheat when passing? Perhaps a little luck with questions may be, but after all, for us, not a piece of paper, but a command of the language is important, right?
A fairly legal way to find examples of questions is to familiarize yourself with questions from the books: “MCSD Certification Tool Exam 70-483 Programming in C #” and “Wouter de Kort Programming in C # Exam Ref 70-483”. Books in English (as well as the exam itself) can help you to improve your knowledge of technical English. I would advise, studying various questions, to pay attention not to the answer options, but to the very topic of the question. Thus, it is possible to find out some nuances of the language, as well as to work out unknown areas.
From video courses I can advise you a course on MVA (the portal is completely free)
www.microsoftvirtualacademy.com/training-courses/developer-training-with-programming-in-cQuite an interesting, educational, not only C #, but also, if desired, Java online game is located at
www.codehunt.com. It is not suitable for testing, but may be interesting as a warm-up.
The best and most resolved way to raise your score with your mind, and not at the expense of cramming or cheating, is the good old way: after completing the testing, review and think over all the answers to all the questions.

At this "harmful" tips end. This is only part of what you need to know and remember.
I wish everyone a high score, as well as, of course, knowledge and understanding of the C # language
