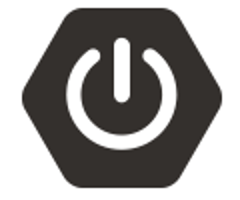
Most of my professional Java projects over the past decade have been based on
Spring or
JEE . Both platforms are developing quite confidently, but still suffer from various problems.
Spring and JEE are still the gold standards for large-scale Java projects and large development teams working on complex enterprise solutions. However, the inevitable consequence of the maturity of the Java community was the partial loss of the former enthusiasm and innovation, which significantly affected the authority of both.
')
JEE has changed quite dramatically over the years, but is still condemned by developers for approaches and solutions that have been designated by the creators of the platform as deprecated since the EJB 2.x version. Many people still call JEE as “J2EE”, although the name change took place 8 years ago!
Spring also improved significantly, but not all users perceive it. Despite the possibility of creating with the help of Spring 3.x and higher modern applications with a transparent, unworn from the code configuration, most of the projects continue to dazzle with XML files and outdated architectural solutions. The problem is that many developers for one reason or another do not change the approach worked out on previous versions.
For these reasons, the road has opened to other languages ​​and frameworks. Java was probably the most popular language for personal projects over a decade ago, but young developers today seem to be paying more attention to Python, Scala, etc. The most influential web frameworks of the previous decade,
Rails , and based on Ruby
Sinatra, have spawned a number of micro-frameworks over the past five years.
Groovy and
Grails , in the Java world, were the first serious answer from Ruby and Rails. They can now be found in the toolkit of even the most conservative enterprise development teams. However, new JVM-based frameworks go much further. Instead of simply wrapping the JEE and Spring APIs into the easy-to-use
Play wrapper, the framework began, so to speak, from scratch, discarding even the seemingly fundamental model of the Java servlet.
Spring is no longer considered a modern innovative tool. Developers still use it, especially for older applications, but mostly because they have to, and not because they themselves want it. I can't remember the last time I spoke with a developer who used Spring in a personal project, while Play or a completely different platform is found everywhere.
It's a shame, because Spring is an incredibly powerful tool, if you manage to configure it correctly.
Spring Data JPA provides simple management of relational databases without writing DAO classes, also Spring Data JPA allows you to get the same functionality with respect to NoSQL data stores, if you manage to configure it correctly. In addition, with the help of Spring you have the opportunity to carry out
enterprise integration , access to the
API of the most popular social services for your web or
android applications, take care of the security of your application through
Spring Security , but, again, if you get everything right configure.
Building an application based on Spring can be a very painful process. This is partly due to the large number of existing alternatives when choosing technologies. For example, is it worth exploring and using Spring Data JPA if you have already spent time learning JdbcTemplate and Hibernate / JPA? What is the difference between
Spring Data REST and
Spring HATEOAS ?
Another complicating factor is that Spring rarely recognizes something deprecated, and does not provide sufficient information to make an informed decision on the choice of technology, to eliminate various conflicts and to solve other frequently occurring problems. If you search the Internet for an example of how to fix a problem, links to outdated approaches and solutions will be in the top of search results. For the most part, it is because of this that the XML configuration remains so prevalent, despite the simplicity of implementation and configuration support based on annotations. Instead of using a pure template system such as
Thymeleaf or
Velocity , most applications still use JSP with JSTL.
Thirst for the elimination of these problems has never faded away from the creators of Spring. Inspired by the
Rails command-line tool , they presented
Spring Roo , a rapid development system that also allows you to create elements such as web controllers or JPA entities via the command line. However, for non-trivial use, it is almost as difficult to master Spring Roo as building applications manually. Many developers were repelled by the abundance of annotations and AspectJ files, which Roo is everywhere adding to the project to ensure its “magic”. Although it is stated that Roo can be easily and painlessly removed from the project, if necessary, the reality turns out to be more severe than the theory. And even if you did it all, converting AspectJ to Java completely prevents you from using the magic command line tool.
Spring Boot is the next generation of tools to simplify the process of configuring Spring applications. It is not a means of automatically generating code, but is a plugin for an automation system for building projects (supports Maven and Gradle).
The plugin provides features for testing and deploying Spring applications. The mvn spring-boot: run command runs your application on port 8080. This is somewhat similar to the rather popular Maven
Jetty plugin. In addition, Spring Boot allows you to pack an application into a separate jar file, with a full-fledged Tomcat container embedded. This approach is borrowed from the Play Framework application deployment model (however, you can also create traditional war files).
The main benefit of Spring Boot is resource configuration based on the classpath content. For example, if the pom.xml file of your Maven project contains JPA dependencies and a PostgreSQL driver, the Spring Boot will configure the persistence module for PostgreSQL. If you add a web dependency, you will get the Spring MVC configured by default. If you need persistence and nothing else, Spring Boot configures Hibernate as a JPA provider with the HSQLDB database. If you are creating a web application, but do not specify anything further, the Spring Boot will configure the view resolver for the Thymeleaf template system.
Speaking of the default configuration, Spring Boot is quite intuitive in this regard. You may not always agree with his choice of settings, but at least he will provide you with a working module. This is a very useful approach, especially for novice developers who can start working with the default settings, and then, as they explore existing alternatives, make changes to the configuration. Agree, it is much better than to get a bunch of complex issues, without solving which it is simply impossible to start. In addition, the
official project page has a number of full-fledged tutorials that allow you to quickly understand and practically implement all major types of projects at the “Hello world” level.
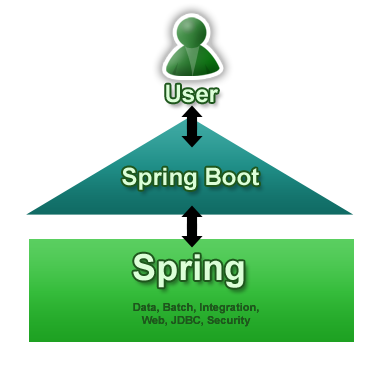
Building the infrastructure of the application actually consists of adding the necessary modules to pom.xml:
... <parent> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-parent</artifactId> <version>1.0.0.RC3</version> </parent> ... <properties> <start-class>com.mypackage.Application</start-class> <java.version>1.7</java.version> </properties> ... <dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-data-jpa</artifactId> </dependency> <dependency> <groupId>com.h2database</groupId> <artifactId>h2</artifactId> <version>1.3.174</version> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-test</artifactId> <scope>test</scope> </dependency> </dependencies> ... <build> <plugins> <plugin> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-maven-plugin</artifactId> </plugin> </plugins> </build> ...
and also writing the main Java class as follows:
@Configuration @EnableAutoConfiguration @ComponentScan @PropertySource("classpath:application.properties") public class Application { @Bean MyCustomService myCustomService() { return new MyCustomService(""); } public static void main(String[] args) { ApplicationContext ctx = SpringApplication.run(Application.class, args); System.out.println("Let's inspect the beans provided by Spring Boot:"); String[] beanNames = ctx.getBeanDefinitionNames(); Arrays.sort(beanNames); for (String beanName : beanNames) { System.out.println(beanName); } } }
For most cases, changing the default settings, just change the POM file. So, in the example above, adding dependencies for the H2 database is demonstrated. Spring Boot, seeing this change, configures the JPA persistence module for the H2 database instead of the default HSQLDB. If you want to use Jetty as a built-in container, instead of the default Tomcat, just add the appropriate dependency.
At the moment, Spring Boot is in its infancy, and, of course, before reaching a stable level, it has to go through a lot of metamorphosis. It may be too early to use it for building serious systems, but it is quite suitable for performing various personal, training and test projects, the implementation of which makes it very important to get rid of the undesirable amount of unproductive, routine work that is in no way associated with the creation of useful functionality.
In the context of the potential prospects for Spring Boot to grow into a serious tool for Spring development in the future, the presence of acceptable technical documentation is especially encouraging (although in English only and still rather scarce compared to the
800 page encyclopedia of the Spring Framework ).