include other tags, which in turn include tags, or text. Here is an example of a document from the previous chapter: <!doctype html> <html> <head> <title> </title> </head> <body> <h1> </h1> <p>, .</p> <p> ! <a href="http://eloquentjavascript.net"></a>.</p> </body> </html>
function talksAbout(node, string) { if (node.nodeType == document.ELEMENT_NODE) { for (var i = 0; i < node.childNodes.length; i++) { if (talksAbout(node.childNodes[i], string)) return true; } return false; } else if (node.nodeType == document.TEXT_NODE) { return node.nodeValue.indexOf(string) > -1; } } console.log(talksAbout(document.body, "")); // → true
var link = document.body.getElementsByTagName("a")[0]; console.log(link.href);
<p> :</p> <p><img id="gertrude" src="img/ostrich.png"></p> <script> var ostrich = document.getElementById("gertrude"); console.log(ostrich.src); </script>
<p></p> <p></p> <p></p> <script> var paragraphs = document.body.getElementsByTagName("p"); document.body.insertBefore(paragraphs[2], paragraphs[0]); </script>
<p> <img src="img/cat.png" alt=""> <img src="img/hat.png" alt="">.</p> <p><button onclick="replaceImages()"></button></p> <script> function replaceImages() { var images = document.body.getElementsByTagName("img"); for (var i = images.length - 1; i >= 0; i--) { var image = images[i]; if (image.alt) { var text = document.createTextNode(image.alt); image.parentNode.replaceChild(text, image); } } } </script>
var arrayish = {0: "", 1: "", length: 2}; var real = Array.prototype.slice.call(arrayish, 0); real.forEach(function(elt) { console.log(elt); }); // → //
<blockquote id="quote"> . , , . </blockquote> <script> function elt(type) { var node = document.createElement(type); for (var i = 1; i < arguments.length; i++) { var child = arguments[i]; if (typeof child == "string") child = document.createTextNode(child); node.appendChild(child); } return node; } document.getElementById("quote").appendChild( elt("footer", "—", elt("strong", " "), ", ", elt("em", " "), ", 1950")); </script>
<p data-classified="secret"> 00000000.</p> <p data-classified="unclassified"> .</p> <script> var paras = document.body.getElementsByTagName("p"); Array.prototype.forEach.call(paras, function(para) { if (para.getAttribute("data-classified") == "secret") para.parentNode.removeChild(para); }); </script>
function highlightCode(node, keywords) { var text = node.textContent; node.textContent = ""; // var match, pos = 0; while (match = keywords.exec(text)) { var before = text.slice(pos, match.index); node.appendChild(document.createTextNode(before)); var strong = document.createElement("strong"); strong.appendChild(document.createTextNode(match[0])); node.appendChild(strong); pos = keywords.lastIndex; } var after = text.slice(pos); node.appendChild(document.createTextNode(after)); }
var languages = { javascript: /\b(function|return|var)\b/g /* … etc */ }; function highlightAllCode() { var pres = document.body.getElementsByTagName("pre"); for (var i = 0; i < pres.length; i++) { var pre = pres[i]; var lang = pre.getAttribute("data-language"); if (languages.hasOwnProperty(lang)) highlightCode(pre, languages[lang]); } }
<p> , :</p> <pre data-language="javascript"> function id(x) { return x; } </pre> <script>highlightAllCode();</script>
<p style="border: 3px solid red"> </p> <script> var para = document.body.getElementsByTagName("p")[0]; console.log("clientHeight:", para.clientHeight); console.log("offsetHeight:", para.offsetHeight); </script>
<p><span id="one"></span></p> <p><span id="two"></span></p> <script> function time(name, action) { var start = Date.now(); // action(); console.log(name, "", Date.now() - start, "ms"); } time("", function() { var target = document.getElementById("one"); while (target.offsetWidth < 2000) target.appendChild(document.createTextNode("X")); }); // → 32 ms time("", function() { var target = document.getElementById("two"); target.appendChild(document.createTextNode("XXXXX")); var total = Math.ceil(2000 / (target.offsetWidth / 5)); for (var i = 5; i < total; i++) target.appendChild(document.createTextNode("X")); }); // → 1 ms </script>
.
, , . , , , . style ():
<p><a href="."> </a></p> <p><a href="." style="color: green"> </a></p>
style (color), . : “color: red; border: none”.
. , display , .
<strong></strong>, <strong style="display: block"> </strong>, <strong style="display: none"> </strong>.
, – display: none . . , .
JavaScript style. , . – , - .
<p id="para" style="color: purple"> </p> <script> var para = document.getElementById("para"); console.log(para.style.color); para.style.color = "magenta"; </script>
, font-family. JavaScript ( style["font-family"]), , : style.fontFamily
HTML CSS (Cascading Style Sheets, ). – . :
<style> strong { font-style: italic; color: gray; } </style> <p> <strong> strong</strong> .</p>
«» , . , , , font-style .
, . font-weight: normal, , , . , style, .
CSS . .abc , “abc”. #xyz id “xyz” ( id ).
.subtle { color: gray; font-size: 80%; } #header { background: blue; color: white; } /* p, a b, id main */ pab#main { margin-bottom: 20px; }
, . , , . , pa , p .a, .
p > a {…}
, .
pa {…}
, , .
. , 2-3 . (, , ) – - DOM.
querySelectorAll, document, -, , , .
<p> <span class="animal"></span></p> <p> </p> <p> <span class="character"> <span class="animal"> </span></span></p> <p> .</p> <script> function count(selector) { return document.querySelectorAll(selector).length; } console.log(count("p")); // <p> // → 4 console.log(count(".animal")); // animal // → 2 console.log(count("p .animal")); // animal <p> // → 2 console.log(count("p > .animal")); // <p> // → 1 </script>
getElementsByTagName, querySelectorAll . , .
querySelector ( All) . , . , null, .
position . static, , . relative, , top left . absolute, «» – , . , left top , position static. , .
. , .
<p style="text-align: center"> <img src="img/cat.png" style="position: relative"> </p> <script> var cat = document.querySelector("img"); var angle = 0, lastTime = null; function animate(time) { if (lastTime != null) angle += (time - lastTime) * 0.001; lastTime = time; cat.style.top = (Math.sin(angle) * 20) + "px"; cat.style.left = (Math.cos(angle) * 200) + "px"; requestAnimationFrame(animate); } requestAnimationFrame(animate); </script>
position: relative. top left , .
requestAnimationFrame animate , . animate requestAnimationFrame, . ( ) , 60 , .
DOM , . JavaScript, . requestAnimationFrame – , , , .
, ( lastTime), , . , , , .
Math.cos Math.sin. , , .
Math.cos Math.sin , (0, 0) . , 0 , , 2π ( 6.28) . Math.cos x , , Math.sin y. ( ) , 2π 0, – , a+2π , a.
angle , animation. image. top Math.sin 20 – . left Math.cos 200, .
. px , , ( , ems ). . – 0, .
JavaScript DOM. , JavaScript . DOM , . parentNode childNodes, .
, , - . , , color display. JavaScript style.
6. HTML . HTML :
<table> <tr> <th>name</th> <th>height</th> <th>country</th> </tr> <tr> <td>Kilimanjaro</td> <td>5895</td> <td>Tanzania</td> </tr> </table>
. : , .
, 6, MOUNTAINS.
buildTable, , , DOM, . , , , . Object.keys, , .
, , style.textAlign "right".
<style> /* */ table { border-collapse: collapse; } td, th { border: 1px solid black; padding: 3px 8px; } th { text-align: left; } </style> <script> function buildTable(data) { // } document.body.appendChild(buildTable(MOUNTAINS)); </script>
getElementsByTagName . , ( ) , .
, tagName. , . toLowerCase toUpperCase.
<h1> <span>span</span> .</h1> <p> <span></span>, <span></span> spans.</p> <script> function byTagName(node, tagName) { // } console.log(byTagName(document.body, "h1").length); // → 1 console.log(byTagName(document.body, "span").length); // → 3 var para = document.querySelector("p"); console.log(byTagName(para, "span").length); // → 2 </script>
, 
.
. - .
, . top left . , position.
<img src="img/cat.png" id="cat" style="position: absolute"> <img src="img/hat.png" id="hat" style="position: absolute"> <script> var cat = document.querySelector("#cat"); var hat = document.querySelector("#hat"); // Your code here. </script>
.
, , . , , , . style ():
<p><a href="."> </a></p> <p><a href="." style="color: green"> </a></p>
style (color), . : “color: red; border: none”.
. , display , .
<strong></strong>, <strong style="display: block"> </strong>, <strong style="display: none"> </strong>.
, – display: none . . , .
JavaScript style. , . – , - .
<p id="para" style="color: purple"> </p> <script> var para = document.getElementById("para"); console.log(para.style.color); para.style.color = "magenta"; </script>
, font-family. JavaScript ( style["font-family"]), , : style.fontFamily
HTML CSS (Cascading Style Sheets, ). – . :
<style> strong { font-style: italic; color: gray; } </style> <p> <strong> strong</strong> .</p>
«» , . , , , font-style .
, . font-weight: normal, , , . , style, .
CSS . .abc , “abc”. #xyz id “xyz” ( id ).
.subtle { color: gray; font-size: 80%; } #header { background: blue; color: white; } /* p, a b, id main */ pab#main { margin-bottom: 20px; }
, . , , . , pa , p .a, .
p > a {…}
, .
pa {…}
, , .
. , 2-3 . (, , ) – - DOM.
querySelectorAll, document, -, , , .
<p> <span class="animal"></span></p> <p> </p> <p> <span class="character"> <span class="animal"> </span></span></p> <p> .</p> <script> function count(selector) { return document.querySelectorAll(selector).length; } console.log(count("p")); // <p> // → 4 console.log(count(".animal")); // animal // → 2 console.log(count("p .animal")); // animal <p> // → 2 console.log(count("p > .animal")); // <p> // → 1 </script>
getElementsByTagName, querySelectorAll . , .
querySelector ( All) . , . , null, .
position . static, , . relative, , top left . absolute, «» – , . , left top , position static. , .
. , .
<p style="text-align: center"> <img src="img/cat.png" style="position: relative"> </p> <script> var cat = document.querySelector("img"); var angle = 0, lastTime = null; function animate(time) { if (lastTime != null) angle += (time - lastTime) * 0.001; lastTime = time; cat.style.top = (Math.sin(angle) * 20) + "px"; cat.style.left = (Math.cos(angle) * 200) + "px"; requestAnimationFrame(animate); } requestAnimationFrame(animate); </script>
position: relative. top left , .
requestAnimationFrame animate , . animate requestAnimationFrame, . ( ) , 60 , .
DOM , . JavaScript, . requestAnimationFrame – , , , .
, ( lastTime), , . , , , .
Math.cos Math.sin. , , .
Math.cos Math.sin , (0, 0) . , 0 , , 2π ( 6.28) . Math.cos x , , Math.sin y. ( ) , 2π 0, – , a+2π , a.
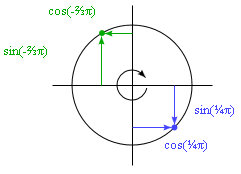
angle , animation. image. top Math.sin 20 – . left Math.cos 200, .
. px , , ( , ems ). . – 0, .
JavaScript DOM. , JavaScript . DOM , . parentNode childNodes, .
, , - . , , color display. JavaScript style.
6. HTML . HTML :
<table> <tr> <th>name</th> <th>height</th> <th>country</th> </tr> <tr> <td>Kilimanjaro</td> <td>5895</td> <td>Tanzania</td> </tr> </table>
. : , .
, 6, MOUNTAINS.
buildTable, , , DOM, . , , , . Object.keys, , .
, , style.textAlign "right".
<style> /* */ table { border-collapse: collapse; } td, th { border: 1px solid black; padding: 3px 8px; } th { text-align: left; } </style> <script> function buildTable(data) { // } document.body.appendChild(buildTable(MOUNTAINS)); </script>
getElementsByTagName . , ( ) , .
, tagName. , . toLowerCase toUpperCase.
<h1> <span>span</span> .</h1> <p> <span></span>, <span></span> spans.</p> <script> function byTagName(node, tagName) { // } console.log(byTagName(document.body, "h1").length); // → 1 console.log(byTagName(document.body, "span").length); // → 3 var para = document.querySelector("p"); console.log(byTagName(para, "span").length); // → 2 </script>
, 
.
. - .
, . top left . , position.
<img src="img/cat.png" id="cat" style="position: absolute"> <img src="img/hat.png" id="hat" style="position: absolute"> <script> var cat = document.querySelector("#cat"); var hat = document.querySelector("#hat"); // Your code here. </script>
.
, , . , , , . style ():
<p><a href="."> </a></p> <p><a href="." style="color: green"> </a></p>
style (color), . : “color: red; border: none”.
. , display , .
<strong></strong>, <strong style="display: block"> </strong>, <strong style="display: none"> </strong>.
, – display: none . . , .
JavaScript style. , . – , - .
<p id="para" style="color: purple"> </p> <script> var para = document.getElementById("para"); console.log(para.style.color); para.style.color = "magenta"; </script>
, font-family. JavaScript ( style["font-family"]), , : style.fontFamily
HTML CSS (Cascading Style Sheets, ). – . :
<style> strong { font-style: italic; color: gray; } </style> <p> <strong> strong</strong> .</p>
«» , . , , , font-style .
, . font-weight: normal, , , . , style, .
CSS . .abc , “abc”. #xyz id “xyz” ( id ).
.subtle { color: gray; font-size: 80%; } #header { background: blue; color: white; } /* p, a b, id main */ pab#main { margin-bottom: 20px; }
, . , , . , pa , p .a, .
p > a {…}
, .
pa {…}
, , .
. , 2-3 . (, , ) – - DOM.
querySelectorAll, document, -, , , .
<p> <span class="animal"></span></p> <p> </p> <p> <span class="character"> <span class="animal"> </span></span></p> <p> .</p> <script> function count(selector) { return document.querySelectorAll(selector).length; } console.log(count("p")); // <p> // → 4 console.log(count(".animal")); // animal // → 2 console.log(count("p .animal")); // animal <p> // → 2 console.log(count("p > .animal")); // <p> // → 1 </script>
getElementsByTagName, querySelectorAll . , .
querySelector ( All) . , . , null, .
position . static, , . relative, , top left . absolute, «» – , . , left top , position static. , .
. , .
<p style="text-align: center"> <img src="img/cat.png" style="position: relative"> </p> <script> var cat = document.querySelector("img"); var angle = 0, lastTime = null; function animate(time) { if (lastTime != null) angle += (time - lastTime) * 0.001; lastTime = time; cat.style.top = (Math.sin(angle) * 20) + "px"; cat.style.left = (Math.cos(angle) * 200) + "px"; requestAnimationFrame(animate); } requestAnimationFrame(animate); </script>
position: relative. top left , .
requestAnimationFrame animate , . animate requestAnimationFrame, . ( ) , 60 , .
DOM , . JavaScript, . requestAnimationFrame – , , , .
, ( lastTime), , . , , , .
Math.cos Math.sin. , , .
Math.cos Math.sin , (0, 0) . , 0 , , 2π ( 6.28) . Math.cos x , , Math.sin y. ( ) , 2π 0, – , a+2π , a.
angle , animation. image. top Math.sin 20 – . left Math.cos 200, .
. px , , ( , ems ). . – 0, .
JavaScript DOM. , JavaScript . DOM , . parentNode childNodes, .
, , - . , , color display. JavaScript style.
6. HTML . HTML :
<table> <tr> <th>name</th> <th>height</th> <th>country</th> </tr> <tr> <td>Kilimanjaro</td> <td>5895</td> <td>Tanzania</td> </tr> </table>
. : , .
, 6, MOUNTAINS.
buildTable, , , DOM, . , , , . Object.keys, , .
, , style.textAlign "right".
<style> /* */ table { border-collapse: collapse; } td, th { border: 1px solid black; padding: 3px 8px; } th { text-align: left; } </style> <script> function buildTable(data) { // } document.body.appendChild(buildTable(MOUNTAINS)); </script>
getElementsByTagName . , ( ) , .
, tagName. , . toLowerCase toUpperCase.
<h1> <span>span</span> .</h1> <p> <span></span>, <span></span> spans.</p> <script> function byTagName(node, tagName) { // } console.log(byTagName(document.body, "h1").length); // → 1 console.log(byTagName(document.body, "span").length); // → 3 var para = document.querySelector("p"); console.log(byTagName(para, "span").length); // → 2 </script>
, 
.
. - .
, . top left . , position.
<img src="img/cat.png" id="cat" style="position: absolute"> <img src="img/hat.png" id="hat" style="position: absolute"> <script> var cat = document.querySelector("#cat"); var hat = document.querySelector("#hat"); // Your code here. </script>
.
, , . , , , . style ():
<p><a href="."> </a></p> <p><a href="." style="color: green"> </a></p>
style (color), . : “color: red; border: none”.
. , display , .
<strong></strong>, <strong style="display: block"> </strong>, <strong style="display: none"> </strong>.
, – display: none . . , .
JavaScript style. , . – , - .
<p id="para" style="color: purple"> </p> <script> var para = document.getElementById("para"); console.log(para.style.color); para.style.color = "magenta"; </script>
, font-family. JavaScript ( style["font-family"]), , : style.fontFamily
HTML CSS (Cascading Style Sheets, ). – . :
<style> strong { font-style: italic; color: gray; } </style> <p> <strong> strong</strong> .</p>
«» , . , , , font-style .
, . font-weight: normal, , , . , style, .
CSS . .abc , “abc”. #xyz id “xyz” ( id ).
.subtle { color: gray; font-size: 80%; } #header { background: blue; color: white; } /* p, a b, id main */ pab#main { margin-bottom: 20px; }
, . , , . , pa , p .a, .
p > a {…}
, .
pa {…}
, , .
. , 2-3 . (, , ) – - DOM.
querySelectorAll, document, -, , , .
<p> <span class="animal"></span></p> <p> </p> <p> <span class="character"> <span class="animal"> </span></span></p> <p> .</p> <script> function count(selector) { return document.querySelectorAll(selector).length; } console.log(count("p")); // <p> // → 4 console.log(count(".animal")); // animal // → 2 console.log(count("p .animal")); // animal <p> // → 2 console.log(count("p > .animal")); // <p> // → 1 </script>
getElementsByTagName, querySelectorAll . , .
querySelector ( All) . , . , null, .
position . static, , . relative, , top left . absolute, «» – , . , left top , position static. , .
. , .
<p style="text-align: center"> <img src="img/cat.png" style="position: relative"> </p> <script> var cat = document.querySelector("img"); var angle = 0, lastTime = null; function animate(time) { if (lastTime != null) angle += (time - lastTime) * 0.001; lastTime = time; cat.style.top = (Math.sin(angle) * 20) + "px"; cat.style.left = (Math.cos(angle) * 200) + "px"; requestAnimationFrame(animate); } requestAnimationFrame(animate); </script>
position: relative. top left , .
requestAnimationFrame animate , . animate requestAnimationFrame, . ( ) , 60 , .
DOM , . JavaScript, . requestAnimationFrame – , , , .
, ( lastTime), , . , , , .
Math.cos Math.sin. , , .
Math.cos Math.sin , (0, 0) . , 0 , , 2π ( 6.28) . Math.cos x , , Math.sin y. ( ) , 2π 0, – , a+2π , a.
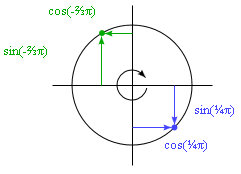
angle , animation. image. top Math.sin 20 – . left Math.cos 200, .
. px , , ( , ems ). . – 0, .
JavaScript DOM. , JavaScript . DOM , . parentNode childNodes, .
, , - . , , color display. JavaScript style.
6. HTML . HTML :
<table> <tr> <th>name</th> <th>height</th> <th>country</th> </tr> <tr> <td>Kilimanjaro</td> <td>5895</td> <td>Tanzania</td> </tr> </table>
. : , .
, 6, MOUNTAINS.
buildTable, , , DOM, . , , , . Object.keys, , .
, , style.textAlign "right".
<style> /* */ table { border-collapse: collapse; } td, th { border: 1px solid black; padding: 3px 8px; } th { text-align: left; } </style> <script> function buildTable(data) { // } document.body.appendChild(buildTable(MOUNTAINS)); </script>
getElementsByTagName . , ( ) , .
, tagName. , . toLowerCase toUpperCase.
<h1> <span>span</span> .</h1> <p> <span></span>, <span></span> spans.</p> <script> function byTagName(node, tagName) { // } console.log(byTagName(document.body, "h1").length); // → 1 console.log(byTagName(document.body, "span").length); // → 3 var para = document.querySelector("p"); console.log(byTagName(para, "span").length); // → 2 </script>
, 
.
. - .
, . top left . , position.
<img src="img/cat.png" id="cat" style="position: absolute"> <img src="img/hat.png" id="hat" style="position: absolute"> <script> var cat = document.querySelector("#cat"); var hat = document.querySelector("#hat"); // Your code here. </script>
.
, , . , , , . style ():
<p><a href="."> </a></p> <p><a href="." style="color: green"> </a></p>
style (color), . : “color: red; border: none”.
. , display , .
<strong></strong>, <strong style="display: block"> </strong>, <strong style="display: none"> </strong>.
, – display: none . . , .
JavaScript style. , . – , - .
<p id="para" style="color: purple"> </p> <script> var para = document.getElementById("para"); console.log(para.style.color); para.style.color = "magenta"; </script>
, font-family. JavaScript ( style["font-family"]), , : style.fontFamily
HTML CSS (Cascading Style Sheets, ). – . :
<style> strong { font-style: italic; color: gray; } </style> <p> <strong> strong</strong> .</p>
«» , . , , , font-style .
, . font-weight: normal, , , . , style, .
CSS . .abc , “abc”. #xyz id “xyz” ( id ).
.subtle { color: gray; font-size: 80%; } #header { background: blue; color: white; } /* p, a b, id main */ pab#main { margin-bottom: 20px; }
, . , , . , pa , p .a, .
p > a {…}
, .
pa {…}
, , .
. , 2-3 . (, , ) – - DOM.
querySelectorAll, document, -, , , .
<p> <span class="animal"></span></p> <p> </p> <p> <span class="character"> <span class="animal"> </span></span></p> <p> .</p> <script> function count(selector) { return document.querySelectorAll(selector).length; } console.log(count("p")); // <p> // → 4 console.log(count(".animal")); // animal // → 2 console.log(count("p .animal")); // animal <p> // → 2 console.log(count("p > .animal")); // <p> // → 1 </script>
getElementsByTagName, querySelectorAll . , .
querySelector ( All) . , . , null, .
position . static, , . relative, , top left . absolute, «» – , . , left top , position static. , .
. , .
<p style="text-align: center"> <img src="img/cat.png" style="position: relative"> </p> <script> var cat = document.querySelector("img"); var angle = 0, lastTime = null; function animate(time) { if (lastTime != null) angle += (time - lastTime) * 0.001; lastTime = time; cat.style.top = (Math.sin(angle) * 20) + "px"; cat.style.left = (Math.cos(angle) * 200) + "px"; requestAnimationFrame(animate); } requestAnimationFrame(animate); </script>
position: relative. top left , .
requestAnimationFrame animate , . animate requestAnimationFrame, . ( ) , 60 , .
DOM , . JavaScript, . requestAnimationFrame – , , , .
, ( lastTime), , . , , , .
Math.cos Math.sin. , , .
Math.cos Math.sin , (0, 0) . , 0 , , 2π ( 6.28) . Math.cos x , , Math.sin y. ( ) , 2π 0, – , a+2π , a.
angle , animation. image. top Math.sin 20 – . left Math.cos 200, .
. px , , ( , ems ). . – 0, .
JavaScript DOM. , JavaScript . DOM , . parentNode childNodes, .
, , - . , , color display. JavaScript style.
6. HTML . HTML :
<table> <tr> <th>name</th> <th>height</th> <th>country</th> </tr> <tr> <td>Kilimanjaro</td> <td>5895</td> <td>Tanzania</td> </tr> </table>
. : , .
, 6, MOUNTAINS.
buildTable, , , DOM, . , , , . Object.keys, , .
, , style.textAlign "right".
<style> /* */ table { border-collapse: collapse; } td, th { border: 1px solid black; padding: 3px 8px; } th { text-align: left; } </style> <script> function buildTable(data) { // } document.body.appendChild(buildTable(MOUNTAINS)); </script>
getElementsByTagName . , ( ) , .
, tagName. , . toLowerCase toUpperCase.
<h1> <span>span</span> .</h1> <p> <span></span>, <span></span> spans.</p> <script> function byTagName(node, tagName) { // } console.log(byTagName(document.body, "h1").length); // → 1 console.log(byTagName(document.body, "span").length); // → 3 var para = document.querySelector("p"); console.log(byTagName(para, "span").length); // → 2 </script>
, 
.
. - .
, . top left . , position.
<img src="img/cat.png" id="cat" style="position: absolute"> <img src="img/hat.png" id="hat" style="position: absolute"> <script> var cat = document.querySelector("#cat"); var hat = document.querySelector("#hat"); // Your code here. </script>
.
, , . , , , . style ():
<p><a href="."> </a></p> <p><a href="." style="color: green"> </a></p>
style (color), . : “color: red; border: none”.
. , display , .
<strong></strong>, <strong style="display: block"> </strong>, <strong style="display: none"> </strong>.
, – display: none . . , .
JavaScript style. , . – , - .
<p id="para" style="color: purple"> </p> <script> var para = document.getElementById("para"); console.log(para.style.color); para.style.color = "magenta"; </script>
, font-family. JavaScript ( style["font-family"]), , : style.fontFamily
HTML CSS (Cascading Style Sheets, ). – . :
<style> strong { font-style: italic; color: gray; } </style> <p> <strong> strong</strong> .</p>
«» , . , , , font-style .
, . font-weight: normal, , , . , style, .
CSS . .abc , “abc”. #xyz id “xyz” ( id ).
.subtle { color: gray; font-size: 80%; } #header { background: blue; color: white; } /* p, a b, id main */ pab#main { margin-bottom: 20px; }
, . , , . , pa , p .a, .
p > a {…}
, .
pa {…}
, , .
. , 2-3 . (, , ) – - DOM.
querySelectorAll, document, -, , , .
<p> <span class="animal"></span></p> <p> </p> <p> <span class="character"> <span class="animal"> </span></span></p> <p> .</p> <script> function count(selector) { return document.querySelectorAll(selector).length; } console.log(count("p")); // <p> // → 4 console.log(count(".animal")); // animal // → 2 console.log(count("p .animal")); // animal <p> // → 2 console.log(count("p > .animal")); // <p> // → 1 </script>
getElementsByTagName, querySelectorAll . , .
querySelector ( All) . , . , null, .
position . static, , . relative, , top left . absolute, «» – , . , left top , position static. , .
. , .
<p style="text-align: center"> <img src="img/cat.png" style="position: relative"> </p> <script> var cat = document.querySelector("img"); var angle = 0, lastTime = null; function animate(time) { if (lastTime != null) angle += (time - lastTime) * 0.001; lastTime = time; cat.style.top = (Math.sin(angle) * 20) + "px"; cat.style.left = (Math.cos(angle) * 200) + "px"; requestAnimationFrame(animate); } requestAnimationFrame(animate); </script>
position: relative. top left , .
requestAnimationFrame animate , . animate requestAnimationFrame, . ( ) , 60 , .
DOM , . JavaScript, . requestAnimationFrame – , , , .
, ( lastTime), , . , , , .
Math.cos Math.sin. , , .
Math.cos Math.sin , (0, 0) . , 0 , , 2π ( 6.28) . Math.cos x , , Math.sin y. ( ) , 2π 0, – , a+2π , a.
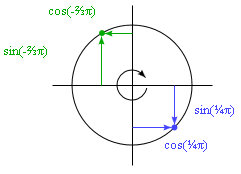
angle , animation. image. top Math.sin 20 – . left Math.cos 200, .
. px , , ( , ems ). . – 0, .
JavaScript DOM. , JavaScript . DOM , . parentNode childNodes, .
, , - . , , color display. JavaScript style.
6. HTML . HTML :
<table> <tr> <th>name</th> <th>height</th> <th>country</th> </tr> <tr> <td>Kilimanjaro</td> <td>5895</td> <td>Tanzania</td> </tr> </table>
. : , .
, 6, MOUNTAINS.
buildTable, , , DOM, . , , , . Object.keys, , .
, , style.textAlign "right".
<style> /* */ table { border-collapse: collapse; } td, th { border: 1px solid black; padding: 3px 8px; } th { text-align: left; } </style> <script> function buildTable(data) { // } document.body.appendChild(buildTable(MOUNTAINS)); </script>
getElementsByTagName . , ( ) , .
, tagName. , . toLowerCase toUpperCase.
<h1> <span>span</span> .</h1> <p> <span></span>, <span></span> spans.</p> <script> function byTagName(node, tagName) { // } console.log(byTagName(document.body, "h1").length); // → 1 console.log(byTagName(document.body, "span").length); // → 3 var para = document.querySelector("p"); console.log(byTagName(para, "span").length); // → 2 </script>
, 
.
. - .
, . top left . , position.
<img src="img/cat.png" id="cat" style="position: absolute"> <img src="img/hat.png" id="hat" style="position: absolute"> <script> var cat = document.querySelector("#cat"); var hat = document.querySelector("#hat"); // Your code here. </script>
.
, , . , , , . style ():
<p><a href="."> </a></p> <p><a href="." style="color: green"> </a></p>
style (color), . : “color: red; border: none”.
. , display , .
<strong></strong>, <strong style="display: block"> </strong>, <strong style="display: none"> </strong>.
, – display: none . . , .
JavaScript style. , . – , - .
<p id="para" style="color: purple"> </p> <script> var para = document.getElementById("para"); console.log(para.style.color); para.style.color = "magenta"; </script>
, font-family. JavaScript ( style["font-family"]), , : style.fontFamily
HTML CSS (Cascading Style Sheets, ). – . :
<style> strong { font-style: italic; color: gray; } </style> <p> <strong> strong</strong> .</p>
«» , . , , , font-style .
, . font-weight: normal, , , . , style, .
CSS . .abc , “abc”. #xyz id “xyz” ( id ).
.subtle { color: gray; font-size: 80%; } #header { background: blue; color: white; } /* p, a b, id main */ pab#main { margin-bottom: 20px; }
, . , , . , pa , p .a, .
p > a {…}
, .
pa {…}
, , .
. , 2-3 . (, , ) – - DOM.
querySelectorAll, document, -, , , .
<p> <span class="animal"></span></p> <p> </p> <p> <span class="character"> <span class="animal"> </span></span></p> <p> .</p> <script> function count(selector) { return document.querySelectorAll(selector).length; } console.log(count("p")); // <p> // → 4 console.log(count(".animal")); // animal // → 2 console.log(count("p .animal")); // animal <p> // → 2 console.log(count("p > .animal")); // <p> // → 1 </script>
getElementsByTagName, querySelectorAll . , .
querySelector ( All) . , . , null, .
position . static, , . relative, , top left . absolute, «» – , . , left top , position static. , .
. , .
<p style="text-align: center"> <img src="img/cat.png" style="position: relative"> </p> <script> var cat = document.querySelector("img"); var angle = 0, lastTime = null; function animate(time) { if (lastTime != null) angle += (time - lastTime) * 0.001; lastTime = time; cat.style.top = (Math.sin(angle) * 20) + "px"; cat.style.left = (Math.cos(angle) * 200) + "px"; requestAnimationFrame(animate); } requestAnimationFrame(animate); </script>
position: relative. top left , .
requestAnimationFrame animate , . animate requestAnimationFrame, . ( ) , 60 , .
DOM , . JavaScript, . requestAnimationFrame – , , , .
, ( lastTime), , . , , , .
Math.cos Math.sin. , , .
Math.cos Math.sin , (0, 0) . , 0 , , 2π ( 6.28) . Math.cos x , , Math.sin y. ( ) , 2π 0, – , a+2π , a.
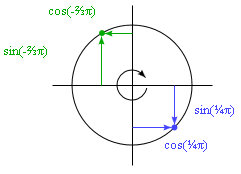
angle , animation. image. top Math.sin 20 – . left Math.cos 200, .
. px , , ( , ems ). . – 0, .
JavaScript DOM. , JavaScript . DOM , . parentNode childNodes, .
, , - . , , color display. JavaScript style.
6. HTML . HTML :
<table> <tr> <th>name</th> <th>height</th> <th>country</th> </tr> <tr> <td>Kilimanjaro</td> <td>5895</td> <td>Tanzania</td> </tr> </table>
. : , .
, 6, MOUNTAINS.
buildTable, , , DOM, . , , , . Object.keys, , .
, , style.textAlign "right".
<style> /* */ table { border-collapse: collapse; } td, th { border: 1px solid black; padding: 3px 8px; } th { text-align: left; } </style> <script> function buildTable(data) { // } document.body.appendChild(buildTable(MOUNTAINS)); </script>
getElementsByTagName . , ( ) , .
, tagName. , . toLowerCase toUpperCase.
<h1> <span>span</span> .</h1> <p> <span></span>, <span></span> spans.</p> <script> function byTagName(node, tagName) { // } console.log(byTagName(document.body, "h1").length); // → 1 console.log(byTagName(document.body, "span").length); // → 3 var para = document.querySelector("p"); console.log(byTagName(para, "span").length); // → 2 </script>
, 
.
. - .
, . top left . , position.
<img src="img/cat.png" id="cat" style="position: absolute"> <img src="img/hat.png" id="hat" style="position: absolute"> <script> var cat = document.querySelector("#cat"); var hat = document.querySelector("#hat"); // Your code here. </script>
.
, , . , , , . style ():
<p><a href="."> </a></p> <p><a href="." style="color: green"> </a></p>
style (color), . : “color: red; border: none”.
. , display , .
<strong></strong>, <strong style="display: block"> </strong>, <strong style="display: none"> </strong>.
, – display: none . . , .
JavaScript style. , . – , - .
<p id="para" style="color: purple"> </p> <script> var para = document.getElementById("para"); console.log(para.style.color); para.style.color = "magenta"; </script>
, font-family. JavaScript ( style["font-family"]), , : style.fontFamily
HTML CSS (Cascading Style Sheets, ). – . :
<style> strong { font-style: italic; color: gray; } </style> <p> <strong> strong</strong> .</p>
«» , . , , , font-style .
, . font-weight: normal, , , . , style, .
CSS . .abc , “abc”. #xyz id “xyz” ( id ).
.subtle { color: gray; font-size: 80%; } #header { background: blue; color: white; } /* p, a b, id main */ pab#main { margin-bottom: 20px; }
, . , , . , pa , p .a, .
p > a {…}
, .
pa {…}
, , .
. , 2-3 . (, , ) – - DOM.
querySelectorAll, document, -, , , .
<p> <span class="animal"></span></p> <p> </p> <p> <span class="character"> <span class="animal"> </span></span></p> <p> .</p> <script> function count(selector) { return document.querySelectorAll(selector).length; } console.log(count("p")); // <p> // → 4 console.log(count(".animal")); // animal // → 2 console.log(count("p .animal")); // animal <p> // → 2 console.log(count("p > .animal")); // <p> // → 1 </script>
getElementsByTagName, querySelectorAll . , .
querySelector ( All) . , . , null, .
position . static, , . relative, , top left . absolute, «» – , . , left top , position static. , .
. , .
<p style="text-align: center"> <img src="img/cat.png" style="position: relative"> </p> <script> var cat = document.querySelector("img"); var angle = 0, lastTime = null; function animate(time) { if (lastTime != null) angle += (time - lastTime) * 0.001; lastTime = time; cat.style.top = (Math.sin(angle) * 20) + "px"; cat.style.left = (Math.cos(angle) * 200) + "px"; requestAnimationFrame(animate); } requestAnimationFrame(animate); </script>
position: relative. top left , .
requestAnimationFrame animate , . animate requestAnimationFrame, . ( ) , 60 , .
DOM , . JavaScript, . requestAnimationFrame – , , , .
, ( lastTime), , . , , , .
Math.cos Math.sin. , , .
Math.cos Math.sin , (0, 0) . , 0 , , 2π ( 6.28) . Math.cos x , , Math.sin y. ( ) , 2π 0, – , a+2π , a.
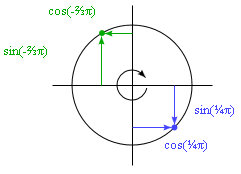
angle , animation. image. top Math.sin 20 – . left Math.cos 200, .
. px , , ( , ems ). . – 0, .
JavaScript DOM. , JavaScript . DOM , . parentNode childNodes, .
, , - . , , color display. JavaScript style.
6. HTML . HTML :
<table> <tr> <th>name</th> <th>height</th> <th>country</th> </tr> <tr> <td>Kilimanjaro</td> <td>5895</td> <td>Tanzania</td> </tr> </table>
. : , .
, 6, MOUNTAINS.
buildTable, , , DOM, . , , , . Object.keys, , .
, , style.textAlign "right".
<style> /* */ table { border-collapse: collapse; } td, th { border: 1px solid black; padding: 3px 8px; } th { text-align: left; } </style> <script> function buildTable(data) { // } document.body.appendChild(buildTable(MOUNTAINS)); </script>
getElementsByTagName . , ( ) , .
, tagName. , . toLowerCase toUpperCase.
<h1> <span>span</span> .</h1> <p> <span></span>, <span></span> spans.</p> <script> function byTagName(node, tagName) { // } console.log(byTagName(document.body, "h1").length); // → 1 console.log(byTagName(document.body, "span").length); // → 3 var para = document.querySelector("p"); console.log(byTagName(para, "span").length); // → 2 </script>
, 
.
. - .
, . top left . , position.
<img src="img/cat.png" id="cat" style="position: absolute"> <img src="img/hat.png" id="hat" style="position: absolute"> <script> var cat = document.querySelector("#cat"); var hat = document.querySelector("#hat"); // Your code here. </script>
.
, , . , , , . style ():
<p><a href="."> </a></p> <p><a href="." style="color: green"> </a></p>
style (color), . : “color: red; border: none”.
. , display , .
<strong></strong>, <strong style="display: block"> </strong>, <strong style="display: none"> </strong>.
, – display: none . . , .
JavaScript style. , . – , - .
<p id="para" style="color: purple"> </p> <script> var para = document.getElementById("para"); console.log(para.style.color); para.style.color = "magenta"; </script>
, font-family. JavaScript ( style["font-family"]), , : style.fontFamily
HTML CSS (Cascading Style Sheets, ). – . :
<style> strong { font-style: italic; color: gray; } </style> <p> <strong> strong</strong> .</p>
«» , . , , , font-style .
, . font-weight: normal, , , . , style, .
CSS . .abc , “abc”. #xyz id “xyz” ( id ).
.subtle { color: gray; font-size: 80%; } #header { background: blue; color: white; } /* p, a b, id main */ pab#main { margin-bottom: 20px; }
, . , , . , pa , p .a, .
p > a {…}
, .
pa {…}
, , .
. , 2-3 . (, , ) – - DOM.
querySelectorAll, document, -, , , .
<p> <span class="animal"></span></p> <p> </p> <p> <span class="character"> <span class="animal"> </span></span></p> <p> .</p> <script> function count(selector) { return document.querySelectorAll(selector).length; } console.log(count("p")); // <p> // → 4 console.log(count(".animal")); // animal // → 2 console.log(count("p .animal")); // animal <p> // → 2 console.log(count("p > .animal")); // <p> // → 1 </script>
getElementsByTagName, querySelectorAll . , .
querySelector ( All) . , . , null, .
position . static, , . relative, , top left . absolute, «» – , . , left top , position static. , .
. , .
<p style="text-align: center"> <img src="img/cat.png" style="position: relative"> </p> <script> var cat = document.querySelector("img"); var angle = 0, lastTime = null; function animate(time) { if (lastTime != null) angle += (time - lastTime) * 0.001; lastTime = time; cat.style.top = (Math.sin(angle) * 20) + "px"; cat.style.left = (Math.cos(angle) * 200) + "px"; requestAnimationFrame(animate); } requestAnimationFrame(animate); </script>
position: relative. top left , .
requestAnimationFrame animate , . animate requestAnimationFrame, . ( ) , 60 , .
DOM , . JavaScript, . requestAnimationFrame – , , , .
, ( lastTime), , . , , , .
Math.cos Math.sin. , , .
Math.cos Math.sin , (0, 0) . , 0 , , 2π ( 6.28) . Math.cos x , , Math.sin y. ( ) , 2π 0, – , a+2π , a.
angle , animation. image. top Math.sin 20 – . left Math.cos 200, .
. px , , ( , ems ). . – 0, .
JavaScript DOM. , JavaScript . DOM , . parentNode childNodes, .
, , - . , , color display. JavaScript style.
6. HTML . HTML :
<table> <tr> <th>name</th> <th>height</th> <th>country</th> </tr> <tr> <td>Kilimanjaro</td> <td>5895</td> <td>Tanzania</td> </tr> </table>
. : , .
, 6, MOUNTAINS.
buildTable, , , DOM, . , , , . Object.keys, , .
, , style.textAlign "right".
<style> /* */ table { border-collapse: collapse; } td, th { border: 1px solid black; padding: 3px 8px; } th { text-align: left; } </style> <script> function buildTable(data) { // } document.body.appendChild(buildTable(MOUNTAINS)); </script>
getElementsByTagName . , ( ) , .
, tagName. , . toLowerCase toUpperCase.
<h1> <span>span</span> .</h1> <p> <span></span>, <span></span> spans.</p> <script> function byTagName(node, tagName) { // } console.log(byTagName(document.body, "h1").length); // → 1 console.log(byTagName(document.body, "span").length); // → 3 var para = document.querySelector("p"); console.log(byTagName(para, "span").length); // → 2 </script>
, 
.
. - .
, . top left . , position.
<img src="img/cat.png" id="cat" style="position: absolute"> <img src="img/hat.png" id="hat" style="position: absolute"> <script> var cat = document.querySelector("#cat"); var hat = document.querySelector("#hat"); // Your code here. </script>
.
, , . , , , . style ():
<p><a href="."> </a></p> <p><a href="." style="color: green"> </a></p>
style (color), . : “color: red; border: none”.
. , display , .
<strong></strong>, <strong style="display: block"> </strong>, <strong style="display: none"> </strong>.
, – display: none . . , .
JavaScript style. , . – , - .
<p id="para" style="color: purple"> </p> <script> var para = document.getElementById("para"); console.log(para.style.color); para.style.color = "magenta"; </script>
, font-family. JavaScript ( style["font-family"]), , : style.fontFamily
HTML CSS (Cascading Style Sheets, ). – . :
<style> strong { font-style: italic; color: gray; } </style> <p> <strong> strong</strong> .</p>
«» , . , , , font-style .
, . font-weight: normal, , , . , style, .
CSS . .abc , “abc”. #xyz id “xyz” ( id ).
.subtle { color: gray; font-size: 80%; } #header { background: blue; color: white; } /* p, a b, id main */ pab#main { margin-bottom: 20px; }
, . , , . , pa , p .a, .
p > a {…}
, .
pa {…}
, , .
. , 2-3 . (, , ) – - DOM.
querySelectorAll, document, -, , , .
<p> <span class="animal"></span></p> <p> </p> <p> <span class="character"> <span class="animal"> </span></span></p> <p> .</p> <script> function count(selector) { return document.querySelectorAll(selector).length; } console.log(count("p")); // <p> // → 4 console.log(count(".animal")); // animal // → 2 console.log(count("p .animal")); // animal <p> // → 2 console.log(count("p > .animal")); // <p> // → 1 </script>
getElementsByTagName, querySelectorAll . , .
querySelector ( All) . , . , null, .
position . static, , . relative, , top left . absolute, «» – , . , left top , position static. , .
. , .
<p style="text-align: center"> <img src="img/cat.png" style="position: relative"> </p> <script> var cat = document.querySelector("img"); var angle = 0, lastTime = null; function animate(time) { if (lastTime != null) angle += (time - lastTime) * 0.001; lastTime = time; cat.style.top = (Math.sin(angle) * 20) + "px"; cat.style.left = (Math.cos(angle) * 200) + "px"; requestAnimationFrame(animate); } requestAnimationFrame(animate); </script>
position: relative. top left , .
requestAnimationFrame animate , . animate requestAnimationFrame, . ( ) , 60 , .
DOM , . JavaScript, . requestAnimationFrame – , , , .
, ( lastTime), , . , , , .
Math.cos Math.sin. , , .
Math.cos Math.sin , (0, 0) . , 0 , , 2π ( 6.28) . Math.cos x , , Math.sin y. ( ) , 2π 0, – , a+2π , a.
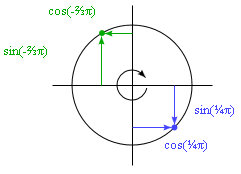
angle , animation. image. top Math.sin 20 – . left Math.cos 200, .
. px , , ( , ems ). . – 0, .
JavaScript DOM. , JavaScript . DOM , . parentNode childNodes, .
, , - . , , color display. JavaScript style.
6. HTML . HTML :
<table> <tr> <th>name</th> <th>height</th> <th>country</th> </tr> <tr> <td>Kilimanjaro</td> <td>5895</td> <td>Tanzania</td> </tr> </table>
. : , .
, 6, MOUNTAINS.
buildTable, , , DOM, . , , , . Object.keys, , .
, , style.textAlign "right".
<style> /* */ table { border-collapse: collapse; } td, th { border: 1px solid black; padding: 3px 8px; } th { text-align: left; } </style> <script> function buildTable(data) { // } document.body.appendChild(buildTable(MOUNTAINS)); </script>
getElementsByTagName . , ( ) , .
, tagName. , . toLowerCase toUpperCase.
<h1> <span>span</span> .</h1> <p> <span></span>, <span></span> spans.</p> <script> function byTagName(node, tagName) { // } console.log(byTagName(document.body, "h1").length); // → 1 console.log(byTagName(document.body, "span").length); // → 3 var para = document.querySelector("p"); console.log(byTagName(para, "span").length); // → 2 </script>
, 
.
. - .
, . top left . , position.
<img src="img/cat.png" id="cat" style="position: absolute"> <img src="img/hat.png" id="hat" style="position: absolute"> <script> var cat = document.querySelector("#cat"); var hat = document.querySelector("#hat"); // Your code here. </script>
.
, , . , , , . style ():
<p><a href="."> </a></p> <p><a href="." style="color: green"> </a></p>
style (color), . : “color: red; border: none”.
. , display , .
<strong></strong>, <strong style="display: block"> </strong>, <strong style="display: none"> </strong>.
, – display: none . . , .
JavaScript style. , . – , - .
<p id="para" style="color: purple"> </p> <script> var para = document.getElementById("para"); console.log(para.style.color); para.style.color = "magenta"; </script>
, font-family. JavaScript ( style["font-family"]), , : style.fontFamily
HTML CSS (Cascading Style Sheets, ). – . :
<style> strong { font-style: italic; color: gray; } </style> <p> <strong> strong</strong> .</p>
«» , . , , , font-style .
, . font-weight: normal, , , . , style, .
CSS . .abc , “abc”. #xyz id “xyz” ( id ).
.subtle { color: gray; font-size: 80%; } #header { background: blue; color: white; } /* p, a b, id main */ pab#main { margin-bottom: 20px; }
, . , , . , pa , p .a, .
p > a {…}
, .
pa {…}
, , .
. , 2-3 . (, , ) – - DOM.
querySelectorAll, document, -, , , .
<p> <span class="animal"></span></p> <p> </p> <p> <span class="character"> <span class="animal"> </span></span></p> <p> .</p> <script> function count(selector) { return document.querySelectorAll(selector).length; } console.log(count("p")); // <p> // → 4 console.log(count(".animal")); // animal // → 2 console.log(count("p .animal")); // animal <p> // → 2 console.log(count("p > .animal")); // <p> // → 1 </script>
getElementsByTagName, querySelectorAll . , .
querySelector ( All) . , . , null, .
position . static, , . relative, , top left . absolute, «» – , . , left top , position static. , .
. , .
<p style="text-align: center"> <img src="img/cat.png" style="position: relative"> </p> <script> var cat = document.querySelector("img"); var angle = 0, lastTime = null; function animate(time) { if (lastTime != null) angle += (time - lastTime) * 0.001; lastTime = time; cat.style.top = (Math.sin(angle) * 20) + "px"; cat.style.left = (Math.cos(angle) * 200) + "px"; requestAnimationFrame(animate); } requestAnimationFrame(animate); </script>
position: relative. top left , .
requestAnimationFrame animate , . animate requestAnimationFrame, . ( ) , 60 , .
DOM , . JavaScript, . requestAnimationFrame – , , , .
, ( lastTime), , . , , , .
Math.cos Math.sin. , , .
Math.cos Math.sin , (0, 0) . , 0 , , 2π ( 6.28) . Math.cos x , , Math.sin y. ( ) , 2π 0, – , a+2π , a.
angle , animation. image. top Math.sin 20 – . left Math.cos 200, .
. px , , ( , ems ). . – 0, .
JavaScript DOM. , JavaScript . DOM , . parentNode childNodes, .
, , - . , , color display. JavaScript style.
6. HTML . HTML :
<table> <tr> <th>name</th> <th>height</th> <th>country</th> </tr> <tr> <td>Kilimanjaro</td> <td>5895</td> <td>Tanzania</td> </tr> </table>
. : , .
, 6, MOUNTAINS.
buildTable, , , DOM, . , , , . Object.keys, , .
, , style.textAlign "right".
<style> /* */ table { border-collapse: collapse; } td, th { border: 1px solid black; padding: 3px 8px; } th { text-align: left; } </style> <script> function buildTable(data) { // } document.body.appendChild(buildTable(MOUNTAINS)); </script>
getElementsByTagName . , ( ) , .
, tagName. , . toLowerCase toUpperCase.
<h1> <span>span</span> .</h1> <p> <span></span>, <span></span> spans.</p> <script> function byTagName(node, tagName) { // } console.log(byTagName(document.body, "h1").length); // → 1 console.log(byTagName(document.body, "span").length); // → 3 var para = document.querySelector("p"); console.log(byTagName(para, "span").length); // → 2 </script>
, 
.
. - .
, . top left . , position.
<img src="img/cat.png" id="cat" style="position: absolute"> <img src="img/hat.png" id="hat" style="position: absolute"> <script> var cat = document.querySelector("#cat"); var hat = document.querySelector("#hat"); // Your code here. </script>
.
, , . , , , . style ():
<p><a href="."> </a></p> <p><a href="." style="color: green"> </a></p>
style (color), . : “color: red; border: none”.
. , display , .
<strong></strong>, <strong style="display: block"> </strong>, <strong style="display: none"> </strong>.
, – display: none . . , .
JavaScript style. , . – , - .
<p id="para" style="color: purple"> </p> <script> var para = document.getElementById("para"); console.log(para.style.color); para.style.color = "magenta"; </script>
, font-family. JavaScript ( style["font-family"]), , : style.fontFamily
HTML CSS (Cascading Style Sheets, ). – . :
<style> strong { font-style: italic; color: gray; } </style> <p> <strong> strong</strong> .</p>
«» , . , , , font-style .
, . font-weight: normal, , , . , style, .
CSS . .abc , “abc”. #xyz id “xyz” ( id ).
.subtle { color: gray; font-size: 80%; } #header { background: blue; color: white; } /* p, a b, id main */ pab#main { margin-bottom: 20px; }
, . , , . , pa , p .a, .
p > a {…}
, .
pa {…}
, , .
. , 2-3 . (, , ) – - DOM.
querySelectorAll, document, -, , , .
<p> <span class="animal"></span></p> <p> </p> <p> <span class="character"> <span class="animal"> </span></span></p> <p> .</p> <script> function count(selector) { return document.querySelectorAll(selector).length; } console.log(count("p")); // <p> // → 4 console.log(count(".animal")); // animal // → 2 console.log(count("p .animal")); // animal <p> // → 2 console.log(count("p > .animal")); // <p> // → 1 </script>
getElementsByTagName, querySelectorAll . , .
querySelector ( All) . , . , null, .
position . static, , . relative, , top left . absolute, «» – , . , left top , position static. , .
. , .
<p style="text-align: center"> <img src="img/cat.png" style="position: relative"> </p> <script> var cat = document.querySelector("img"); var angle = 0, lastTime = null; function animate(time) { if (lastTime != null) angle += (time - lastTime) * 0.001; lastTime = time; cat.style.top = (Math.sin(angle) * 20) + "px"; cat.style.left = (Math.cos(angle) * 200) + "px"; requestAnimationFrame(animate); } requestAnimationFrame(animate); </script>
position: relative. top left , .
requestAnimationFrame animate , . animate requestAnimationFrame, . ( ) , 60 , .
DOM , . JavaScript, . requestAnimationFrame – , , , .
, ( lastTime), , . , , , .
Math.cos Math.sin. , , .
Math.cos Math.sin , (0, 0) . , 0 , , 2π ( 6.28) . Math.cos x , , Math.sin y. ( ) , 2π 0, – , a+2π , a.
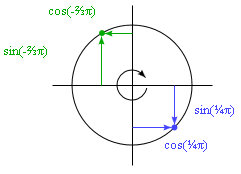
angle , animation. image. top Math.sin 20 – . left Math.cos 200, .
. px , , ( , ems ). . – 0, .
JavaScript DOM. , JavaScript . DOM , . parentNode childNodes, .
, , - . , , color display. JavaScript style.
6. HTML . HTML :
<table> <tr> <th>name</th> <th>height</th> <th>country</th> </tr> <tr> <td>Kilimanjaro</td> <td>5895</td> <td>Tanzania</td> </tr> </table>
. : , .
, 6, MOUNTAINS.
buildTable, , , DOM, . , , , . Object.keys, , .
, , style.textAlign "right".
<style> /* */ table { border-collapse: collapse; } td, th { border: 1px solid black; padding: 3px 8px; } th { text-align: left; } </style> <script> function buildTable(data) { // } document.body.appendChild(buildTable(MOUNTAINS)); </script>
getElementsByTagName . , ( ) , .
, tagName. , . toLowerCase toUpperCase.
<h1> <span>span</span> .</h1> <p> <span></span>, <span></span> spans.</p> <script> function byTagName(node, tagName) { // } console.log(byTagName(document.body, "h1").length); // → 1 console.log(byTagName(document.body, "span").length); // → 3 var para = document.querySelector("p"); console.log(byTagName(para, "span").length); // → 2 </script>
, 
.
. - .
, . top left . , position.
<img src="img/cat.png" id="cat" style="position: absolute"> <img src="img/hat.png" id="hat" style="position: absolute"> <script> var cat = document.querySelector("#cat"); var hat = document.querySelector("#hat"); // Your code here. </script>
.
, , . , , , . style ():
<p><a href="."> </a></p> <p><a href="." style="color: green"> </a></p>
style (color), . : “color: red; border: none”.
. , display , .
<strong></strong>, <strong style="display: block"> </strong>, <strong style="display: none"> </strong>.
, – display: none . . , .
JavaScript style. , . – , - .
<p id="para" style="color: purple"> </p> <script> var para = document.getElementById("para"); console.log(para.style.color); para.style.color = "magenta"; </script>
, font-family. JavaScript ( style["font-family"]), , : style.fontFamily
HTML CSS (Cascading Style Sheets, ). – . :
<style> strong { font-style: italic; color: gray; } </style> <p> <strong> strong</strong> .</p>
«» , . , , , font-style .
, . font-weight: normal, , , . , style, .
CSS . .abc , “abc”. #xyz id “xyz” ( id ).
.subtle { color: gray; font-size: 80%; } #header { background: blue; color: white; } /* p, a b, id main */ pab#main { margin-bottom: 20px; }
, . , , . , pa , p .a, .
p > a {…}
, .
pa {…}
, , .
. , 2-3 . (, , ) – - DOM.
querySelectorAll, document, -, , , .
<p> <span class="animal"></span></p> <p> </p> <p> <span class="character"> <span class="animal"> </span></span></p> <p> .</p> <script> function count(selector) { return document.querySelectorAll(selector).length; } console.log(count("p")); // <p> // → 4 console.log(count(".animal")); // animal // → 2 console.log(count("p .animal")); // animal <p> // → 2 console.log(count("p > .animal")); // <p> // → 1 </script>
getElementsByTagName, querySelectorAll . , .
querySelector ( All) . , . , null, .
position . static, , . relative, , top left . absolute, «» – , . , left top , position static. , .
. , .
<p style="text-align: center"> <img src="img/cat.png" style="position: relative"> </p> <script> var cat = document.querySelector("img"); var angle = 0, lastTime = null; function animate(time) { if (lastTime != null) angle += (time - lastTime) * 0.001; lastTime = time; cat.style.top = (Math.sin(angle) * 20) + "px"; cat.style.left = (Math.cos(angle) * 200) + "px"; requestAnimationFrame(animate); } requestAnimationFrame(animate); </script>
position: relative. top left , .
requestAnimationFrame animate , . animate requestAnimationFrame, . ( ) , 60 , .
DOM , . JavaScript, . requestAnimationFrame – , , , .
, ( lastTime), , . , , , .
Math.cos Math.sin. , , .
Math.cos Math.sin , (0, 0) . , 0 , , 2π ( 6.28) . Math.cos x , , Math.sin y. ( ) , 2π 0, – , a+2π , a.
angle , animation. image. top Math.sin 20 – . left Math.cos 200, .
. px , , ( , ems ). . – 0, .
JavaScript DOM. , JavaScript . DOM , . parentNode childNodes, .
, , - . , , color display. JavaScript style.
6. HTML . HTML :
<table> <tr> <th>name</th> <th>height</th> <th>country</th> </tr> <tr> <td>Kilimanjaro</td> <td>5895</td> <td>Tanzania</td> </tr> </table>
. : , .
, 6, MOUNTAINS.
buildTable, , , DOM, . , , , . Object.keys, , .
, , style.textAlign "right".
<style> /* */ table { border-collapse: collapse; } td, th { border: 1px solid black; padding: 3px 8px; } th { text-align: left; } </style> <script> function buildTable(data) { // } document.body.appendChild(buildTable(MOUNTAINS)); </script>
getElementsByTagName . , ( ) , .
, tagName. , . toLowerCase toUpperCase.
<h1> <span>span</span> .</h1> <p> <span></span>, <span></span> spans.</p> <script> function byTagName(node, tagName) { // } console.log(byTagName(document.body, "h1").length); // → 1 console.log(byTagName(document.body, "span").length); // → 3 var para = document.querySelector("p"); console.log(byTagName(para, "span").length); // → 2 </script>
, 
.
. - .
, . top left . , position.
<img src="img/cat.png" id="cat" style="position: absolute"> <img src="img/hat.png" id="hat" style="position: absolute"> <script> var cat = document.querySelector("#cat"); var hat = document.querySelector("#hat"); // Your code here. </script>
.
, , . , , , . style ():
<p><a href="."> </a></p> <p><a href="." style="color: green"> </a></p>
style (color), . : “color: red; border: none”.
. , display , .
<strong></strong>, <strong style="display: block"> </strong>, <strong style="display: none"> </strong>.
, – display: none . . , .
JavaScript style. , . – , - .
<p id="para" style="color: purple"> </p> <script> var para = document.getElementById("para"); console.log(para.style.color); para.style.color = "magenta"; </script>
, font-family. JavaScript ( style["font-family"]), , : style.fontFamily
HTML CSS (Cascading Style Sheets, ). – . :
<style> strong { font-style: italic; color: gray; } </style> <p> <strong> strong</strong> .</p>
«» , . , , , font-style .
, . font-weight: normal, , , . , style, .
CSS . .abc , “abc”. #xyz id “xyz” ( id ).
.subtle { color: gray; font-size: 80%; } #header { background: blue; color: white; } /* p, a b, id main */ pab#main { margin-bottom: 20px; }
, . , , . , pa , p .a, .
p > a {…}
, .
pa {…}
, , .
. , 2-3 . (, , ) – - DOM.
querySelectorAll, document, -, , , .
<p> <span class="animal"></span></p> <p> </p> <p> <span class="character"> <span class="animal"> </span></span></p> <p> .</p> <script> function count(selector) { return document.querySelectorAll(selector).length; } console.log(count("p")); // <p> // → 4 console.log(count(".animal")); // animal // → 2 console.log(count("p .animal")); // animal <p> // → 2 console.log(count("p > .animal")); // <p> // → 1 </script>
getElementsByTagName, querySelectorAll . , .
querySelector ( All) . , . , null, .
position . static, , . relative, , top left . absolute, «» – , . , left top , position static. , .
. , .
<p style="text-align: center"> <img src="img/cat.png" style="position: relative"> </p> <script> var cat = document.querySelector("img"); var angle = 0, lastTime = null; function animate(time) { if (lastTime != null) angle += (time - lastTime) * 0.001; lastTime = time; cat.style.top = (Math.sin(angle) * 20) + "px"; cat.style.left = (Math.cos(angle) * 200) + "px"; requestAnimationFrame(animate); } requestAnimationFrame(animate); </script>
position: relative. top left , .
requestAnimationFrame animate , . animate requestAnimationFrame, . ( ) , 60 , .
DOM , . JavaScript, . requestAnimationFrame – , , , .
, ( lastTime), , . , , , .
Math.cos Math.sin. , , .
Math.cos Math.sin , (0, 0) . , 0 , , 2π ( 6.28) . Math.cos x , , Math.sin y. ( ) , 2π 0, – , a+2π , a.
angle , animation. image. top Math.sin 20 – . left Math.cos 200, .
. px , , ( , ems ). . – 0, .
JavaScript DOM. , JavaScript . DOM , . parentNode childNodes, .
, , - . , , color display. JavaScript style.
6. HTML . HTML :
<table> <tr> <th>name</th> <th>height</th> <th>country</th> </tr> <tr> <td>Kilimanjaro</td> <td>5895</td> <td>Tanzania</td> </tr> </table>
. : , .
, 6, MOUNTAINS.
buildTable, , , DOM, . , , , . Object.keys, , .
, , style.textAlign "right".
<style> /* */ table { border-collapse: collapse; } td, th { border: 1px solid black; padding: 3px 8px; } th { text-align: left; } </style> <script> function buildTable(data) { // } document.body.appendChild(buildTable(MOUNTAINS)); </script>
getElementsByTagName . , ( ) , .
, tagName. , . toLowerCase toUpperCase.
<h1> <span>span</span> .</h1> <p> <span></span>, <span></span> spans.</p> <script> function byTagName(node, tagName) { // } console.log(byTagName(document.body, "h1").length); // → 1 console.log(byTagName(document.body, "span").length); // → 3 var para = document.querySelector("p"); console.log(byTagName(para, "span").length); // → 2 </script>
, 
.
. - .
, . top left . , position.
<img src="img/cat.png" id="cat" style="position: absolute"> <img src="img/hat.png" id="hat" style="position: absolute"> <script> var cat = document.querySelector("#cat"); var hat = document.querySelector("#hat"); // Your code here. </script>
.
, , . , , , . style ():
<p><a href="."> </a></p> <p><a href="." style="color: green"> </a></p>
style (color), . : “color: red; border: none”.
. , display , .
<strong></strong>, <strong style="display: block"> </strong>, <strong style="display: none"> </strong>.
, – display: none . . , .
JavaScript style. , . – , - .
<p id="para" style="color: purple"> </p> <script> var para = document.getElementById("para"); console.log(para.style.color); para.style.color = "magenta"; </script>
, font-family. JavaScript ( style["font-family"]), , : style.fontFamily
HTML CSS (Cascading Style Sheets, ). – . :
<style> strong { font-style: italic; color: gray; } </style> <p> <strong> strong</strong> .</p>
«» , . , , , font-style .
, . font-weight: normal, , , . , style, .
CSS . .abc , “abc”. #xyz id “xyz” ( id ).
.subtle { color: gray; font-size: 80%; } #header { background: blue; color: white; } /* p, a b, id main */ pab#main { margin-bottom: 20px; }
, . , , . , pa , p .a, .
p > a {…}
, .
pa {…}
, , .
. , 2-3 . (, , ) – - DOM.
querySelectorAll, document, -, , , .
<p> <span class="animal"></span></p> <p> </p> <p> <span class="character"> <span class="animal"> </span></span></p> <p> .</p> <script> function count(selector) { return document.querySelectorAll(selector).length; } console.log(count("p")); // <p> // → 4 console.log(count(".animal")); // animal // → 2 console.log(count("p .animal")); // animal <p> // → 2 console.log(count("p > .animal")); // <p> // → 1 </script>
getElementsByTagName, querySelectorAll . , .
querySelector ( All) . , . , null, .
position . static, , . relative, , top left . absolute, «» – , . , left top , position static. , .
. , .
<p style="text-align: center"> <img src="img/cat.png" style="position: relative"> </p> <script> var cat = document.querySelector("img"); var angle = 0, lastTime = null; function animate(time) { if (lastTime != null) angle += (time - lastTime) * 0.001; lastTime = time; cat.style.top = (Math.sin(angle) * 20) + "px"; cat.style.left = (Math.cos(angle) * 200) + "px"; requestAnimationFrame(animate); } requestAnimationFrame(animate); </script>
position: relative. top left , .
requestAnimationFrame animate , . animate requestAnimationFrame, . ( ) , 60 , .
DOM , . JavaScript, . requestAnimationFrame – , , , .
, ( lastTime), , . , , , .
Math.cos Math.sin. , , .
Math.cos Math.sin , (0, 0) . , 0 , , 2π ( 6.28) . Math.cos x , , Math.sin y. ( ) , 2π 0, – , a+2π , a.
angle , animation. image. top Math.sin 20 – . left Math.cos 200, .
. px , , ( , ems ). . – 0, .
JavaScript DOM. , JavaScript . DOM , . parentNode childNodes, .
, , - . , , color display. JavaScript style.
6. HTML . HTML :
<table> <tr> <th>name</th> <th>height</th> <th>country</th> </tr> <tr> <td>Kilimanjaro</td> <td>5895</td> <td>Tanzania</td> </tr> </table>
. : , .
, 6, MOUNTAINS.
buildTable, , , DOM, . , , , . Object.keys, , .
, , style.textAlign "right".
<style> /* */ table { border-collapse: collapse; } td, th { border: 1px solid black; padding: 3px 8px; } th { text-align: left; } </style> <script> function buildTable(data) { // } document.body.appendChild(buildTable(MOUNTAINS)); </script>
getElementsByTagName . , ( ) , .
, tagName. , . toLowerCase toUpperCase.
<h1> <span>span</span> .</h1> <p> <span></span>, <span></span> spans.</p> <script> function byTagName(node, tagName) { // } console.log(byTagName(document.body, "h1").length); // → 1 console.log(byTagName(document.body, "span").length); // → 3 var para = document.querySelector("p"); console.log(byTagName(para, "span").length); // → 2 </script>
, 
.
. - .
, . top left . , position.
<img src="img/cat.png" id="cat" style="position: absolute"> <img src="img/hat.png" id="hat" style="position: absolute"> <script> var cat = document.querySelector("#cat"); var hat = document.querySelector("#hat"); // Your code here. </script>
.
, , . , , , . style ():
<p><a href="."> </a></p> <p><a href="." style="color: green"> </a></p>
style (color), . : “color: red; border: none”.
. , display , .
<strong></strong>, <strong style="display: block"> </strong>, <strong style="display: none"> </strong>.
, – display: none . . , .
JavaScript style. , . – , - .
<p id="para" style="color: purple"> </p> <script> var para = document.getElementById("para"); console.log(para.style.color); para.style.color = "magenta"; </script>
, font-family. JavaScript ( style["font-family"]), , : style.fontFamily
HTML CSS (Cascading Style Sheets, ). – . :
<style> strong { font-style: italic; color: gray; } </style> <p> <strong> strong</strong> .</p>
«» , . , , , font-style .
, . font-weight: normal, , , . , style, .
CSS . .abc , “abc”. #xyz id “xyz” ( id ).
.subtle { color: gray; font-size: 80%; } #header { background: blue; color: white; } /* p, a b, id main */ pab#main { margin-bottom: 20px; }
, . , , . , pa , p .a, .
p > a {…}
, .
pa {…}
, , .
. , 2-3 . (, , ) – - DOM.
querySelectorAll, document, -, , , .
<p> <span class="animal"></span></p> <p> </p> <p> <span class="character"> <span class="animal"> </span></span></p> <p> .</p> <script> function count(selector) { return document.querySelectorAll(selector).length; } console.log(count("p")); // <p> // → 4 console.log(count(".animal")); // animal // → 2 console.log(count("p .animal")); // animal <p> // → 2 console.log(count("p > .animal")); // <p> // → 1 </script>
getElementsByTagName, querySelectorAll . , .
querySelector ( All) . , . , null, .
position . static, , . relative, , top left . absolute, «» – , . , left top , position static. , .
. , .
<p style="text-align: center"> <img src="img/cat.png" style="position: relative"> </p> <script> var cat = document.querySelector("img"); var angle = 0, lastTime = null; function animate(time) { if (lastTime != null) angle += (time - lastTime) * 0.001; lastTime = time; cat.style.top = (Math.sin(angle) * 20) + "px"; cat.style.left = (Math.cos(angle) * 200) + "px"; requestAnimationFrame(animate); } requestAnimationFrame(animate); </script>
position: relative. top left , .
requestAnimationFrame animate , . animate requestAnimationFrame, . ( ) , 60 , .
DOM , . JavaScript, . requestAnimationFrame – , , , .
, ( lastTime), , . , , , .
Math.cos Math.sin. , , .
Math.cos Math.sin , (0, 0) . , 0 , , 2π ( 6.28) . Math.cos x , , Math.sin y. ( ) , 2π 0, – , a+2π , a.
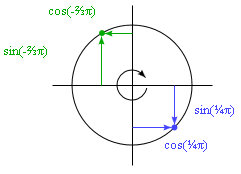
angle , animation. image. top Math.sin 20 – . left Math.cos 200, .
. px , , ( , ems ). . – 0, .
JavaScript DOM. , JavaScript . DOM , . parentNode childNodes, .
, , - . , , color display. JavaScript style.
6. HTML . HTML :
<table> <tr> <th>name</th> <th>height</th> <th>country</th> </tr> <tr> <td>Kilimanjaro</td> <td>5895</td> <td>Tanzania</td> </tr> </table>
. : , .
, 6, MOUNTAINS.
buildTable, , , DOM, . , , , . Object.keys, , .
, , style.textAlign "right".
<style> /* */ table { border-collapse: collapse; } td, th { border: 1px solid black; padding: 3px 8px; } th { text-align: left; } </style> <script> function buildTable(data) { // } document.body.appendChild(buildTable(MOUNTAINS)); </script>
getElementsByTagName . , ( ) , .
, tagName. , . toLowerCase toUpperCase.
<h1> <span>span</span> .</h1> <p> <span></span>, <span></span> spans.</p> <script> function byTagName(node, tagName) { // } console.log(byTagName(document.body, "h1").length); // → 1 console.log(byTagName(document.body, "span").length); // → 3 var para = document.querySelector("p"); console.log(byTagName(para, "span").length); // → 2 </script>
, 
.
. - .
, . top left . , position.
<img src="img/cat.png" id="cat" style="position: absolute"> <img src="img/hat.png" id="hat" style="position: absolute"> <script> var cat = document.querySelector("#cat"); var hat = document.querySelector("#hat"); // Your code here. </script>
.
, , . , , , . style ():
<p><a href="."> </a></p> <p><a href="." style="color: green"> </a></p>
style (color), . : “color: red; border: none”.
. , display , .
<strong></strong>, <strong style="display: block"> </strong>, <strong style="display: none"> </strong>.
, – display: none . . , .
JavaScript style. , . – , - .
<p id="para" style="color: purple"> </p> <script> var para = document.getElementById("para"); console.log(para.style.color); para.style.color = "magenta"; </script>
, font-family. JavaScript ( style["font-family"]), , : style.fontFamily
HTML CSS (Cascading Style Sheets, ). – . :
<style> strong { font-style: italic; color: gray; } </style> <p> <strong> strong</strong> .</p>
«» , . , , , font-style .
, . font-weight: normal, , , . , style, .
CSS . .abc , “abc”. #xyz id “xyz” ( id ).
.subtle { color: gray; font-size: 80%; } #header { background: blue; color: white; } /* p, a b, id main */ pab#main { margin-bottom: 20px; }
, . , , . , pa , p .a, .
p > a {…}
, .
pa {…}
, , .
. , 2-3 . (, , ) – - DOM.
querySelectorAll, document, -, , , .
<p> <span class="animal"></span></p> <p> </p> <p> <span class="character"> <span class="animal"> </span></span></p> <p> .</p> <script> function count(selector) { return document.querySelectorAll(selector).length; } console.log(count("p")); // <p> // → 4 console.log(count(".animal")); // animal // → 2 console.log(count("p .animal")); // animal <p> // → 2 console.log(count("p > .animal")); // <p> // → 1 </script>
getElementsByTagName, querySelectorAll . , .
querySelector ( All) . , . , null, .
position . static, , . relative, , top left . absolute, «» – , . , left top , position static. , .
. , .
<p style="text-align: center"> <img src="img/cat.png" style="position: relative"> </p> <script> var cat = document.querySelector("img"); var angle = 0, lastTime = null; function animate(time) { if (lastTime != null) angle += (time - lastTime) * 0.001; lastTime = time; cat.style.top = (Math.sin(angle) * 20) + "px"; cat.style.left = (Math.cos(angle) * 200) + "px"; requestAnimationFrame(animate); } requestAnimationFrame(animate); </script>
position: relative. top left , .
requestAnimationFrame animate , . animate requestAnimationFrame, . ( ) , 60 , .
DOM , . JavaScript, . requestAnimationFrame – , , , .
, ( lastTime), , . , , , .
Math.cos Math.sin. , , .
Math.cos Math.sin , (0, 0) . , 0 , , 2π ( 6.28) . Math.cos x , , Math.sin y. ( ) , 2π 0, – , a+2π , a.
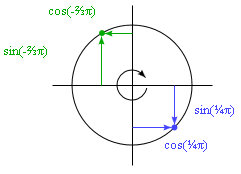
angle , animation. image. top Math.sin 20 – . left Math.cos 200, .
. px , , ( , ems ). . – 0, .
JavaScript DOM. , JavaScript . DOM , . parentNode childNodes, .
, , - . , , color display. JavaScript style.
6. HTML . HTML :
<table> <tr> <th>name</th> <th>height</th> <th>country</th> </tr> <tr> <td>Kilimanjaro</td> <td>5895</td> <td>Tanzania</td> </tr> </table>
. : , .
, 6, MOUNTAINS.
buildTable, , , DOM, . , , , . Object.keys, , .
, , style.textAlign "right".
<style> /* */ table { border-collapse: collapse; } td, th { border: 1px solid black; padding: 3px 8px; } th { text-align: left; } </style> <script> function buildTable(data) { // } document.body.appendChild(buildTable(MOUNTAINS)); </script>
getElementsByTagName . , ( ) , .
, tagName. , . toLowerCase toUpperCase.
<h1> <span>span</span> .</h1> <p> <span></span>, <span></span> spans.</p> <script> function byTagName(node, tagName) { // } console.log(byTagName(document.body, "h1").length); // → 1 console.log(byTagName(document.body, "span").length); // → 3 var para = document.querySelector("p"); console.log(byTagName(para, "span").length); // → 2 </script>
, 
.
. - .
, . top left . , position.
<img src="img/cat.png" id="cat" style="position: absolute"> <img src="img/hat.png" id="hat" style="position: absolute"> <script> var cat = document.querySelector("#cat"); var hat = document.querySelector("#hat"); // Your code here. </script>
.
, , . , , , . style ():
<p><a href="."> </a></p> <p><a href="." style="color: green"> </a></p>
style (color), . : “color: red; border: none”.
. , display , .
<strong></strong>, <strong style="display: block"> </strong>, <strong style="display: none"> </strong>.
, – display: none . . , .
JavaScript style. , . – , - .
<p id="para" style="color: purple"> </p> <script> var para = document.getElementById("para"); console.log(para.style.color); para.style.color = "magenta"; </script>
, font-family. JavaScript ( style["font-family"]), , : style.fontFamily
HTML CSS (Cascading Style Sheets, ). – . :
<style> strong { font-style: italic; color: gray; } </style> <p> <strong> strong</strong> .</p>
«» , . , , , font-style .
, . font-weight: normal, , , . , style, .
CSS . .abc , “abc”. #xyz id “xyz” ( id ).
.subtle { color: gray; font-size: 80%; } #header { background: blue; color: white; } /* p, a b, id main */ pab#main { margin-bottom: 20px; }
, . , , . , pa , p .a, .
p > a {…}
, .
pa {…}
, , .
. , 2-3 . (, , ) – - DOM.
querySelectorAll, document, -, , , .
<p> <span class="animal"></span></p> <p> </p> <p> <span class="character"> <span class="animal"> </span></span></p> <p> .</p> <script> function count(selector) { return document.querySelectorAll(selector).length; } console.log(count("p")); // <p> // → 4 console.log(count(".animal")); // animal // → 2 console.log(count("p .animal")); // animal <p> // → 2 console.log(count("p > .animal")); // <p> // → 1 </script>
getElementsByTagName, querySelectorAll . , .
querySelector ( All) . , . , null, .
position . static, , . relative, , top left . absolute, «» – , . , left top , position static. , .
. , .
<p style="text-align: center"> <img src="img/cat.png" style="position: relative"> </p> <script> var cat = document.querySelector("img"); var angle = 0, lastTime = null; function animate(time) { if (lastTime != null) angle += (time - lastTime) * 0.001; lastTime = time; cat.style.top = (Math.sin(angle) * 20) + "px"; cat.style.left = (Math.cos(angle) * 200) + "px"; requestAnimationFrame(animate); } requestAnimationFrame(animate); </script>
position: relative. top left , .
requestAnimationFrame animate , . animate requestAnimationFrame, . ( ) , 60 , .
DOM , . JavaScript, . requestAnimationFrame – , , , .
, ( lastTime), , . , , , .
Math.cos Math.sin. , , .
Math.cos Math.sin , (0, 0) . , 0 , , 2π ( 6.28) . Math.cos x , , Math.sin y. ( ) , 2π 0, – , a+2π , a.
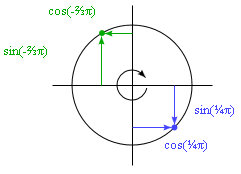
angle , animation. image. top Math.sin 20 – . left Math.cos 200, .
. px , , ( , ems ). . – 0, .
JavaScript DOM. , JavaScript . DOM , . parentNode childNodes, .
, , - . , , color display. JavaScript style.
6. HTML . HTML :
<table> <tr> <th>name</th> <th>height</th> <th>country</th> </tr> <tr> <td>Kilimanjaro</td> <td>5895</td> <td>Tanzania</td> </tr> </table>
. : , .
, 6, MOUNTAINS.
buildTable, , , DOM, . , , , . Object.keys, , .
, , style.textAlign "right".
<style> /* */ table { border-collapse: collapse; } td, th { border: 1px solid black; padding: 3px 8px; } th { text-align: left; } </style> <script> function buildTable(data) { // } document.body.appendChild(buildTable(MOUNTAINS)); </script>
getElementsByTagName . , ( ) , .
, tagName. , . toLowerCase toUpperCase.
<h1> <span>span</span> .</h1> <p> <span></span>, <span></span> spans.</p> <script> function byTagName(node, tagName) { // } console.log(byTagName(document.body, "h1").length); // → 1 console.log(byTagName(document.body, "span").length); // → 3 var para = document.querySelector("p"); console.log(byTagName(para, "span").length); // → 2 </script>
, 
.
. - .
, . top left . , position.
<img src="img/cat.png" id="cat" style="position: absolute"> <img src="img/hat.png" id="hat" style="position: absolute"> <script> var cat = document.querySelector("#cat"); var hat = document.querySelector("#hat"); // Your code here. </script>
.
, , . , , , . style ():
<p><a href="."> </a></p> <p><a href="." style="color: green"> </a></p>
style (color), . : “color: red; border: none”.
. , display , .
<strong></strong>, <strong style="display: block"> </strong>, <strong style="display: none"> </strong>.
, – display: none . . , .
JavaScript style. , . – , - .
<p id="para" style="color: purple"> </p> <script> var para = document.getElementById("para"); console.log(para.style.color); para.style.color = "magenta"; </script>
, font-family. JavaScript ( style["font-family"]), , : style.fontFamily
HTML CSS (Cascading Style Sheets, ). – . :
<style> strong { font-style: italic; color: gray; } </style> <p> <strong> strong</strong> .</p>
«» , . , , , font-style .
, . font-weight: normal, , , . , style, .
CSS . .abc , “abc”. #xyz id “xyz” ( id ).
.subtle { color: gray; font-size: 80%; } #header { background: blue; color: white; } /* p, a b, id main */ pab#main { margin-bottom: 20px; }
, . , , . , pa , p .a, .
p > a {…}
, .
pa {…}
, , .
. , 2-3 . (, , ) – - DOM.
querySelectorAll, document, -, , , .
<p> <span class="animal"></span></p> <p> </p> <p> <span class="character"> <span class="animal"> </span></span></p> <p> .</p> <script> function count(selector) { return document.querySelectorAll(selector).length; } console.log(count("p")); // <p> // → 4 console.log(count(".animal")); // animal // → 2 console.log(count("p .animal")); // animal <p> // → 2 console.log(count("p > .animal")); // <p> // → 1 </script>
getElementsByTagName, querySelectorAll . , .
querySelector ( All) . , . , null, .
position . static, , . relative, , top left . absolute, «» – , . , left top , position static. , .
. , .
<p style="text-align: center"> <img src="img/cat.png" style="position: relative"> </p> <script> var cat = document.querySelector("img"); var angle = 0, lastTime = null; function animate(time) { if (lastTime != null) angle += (time - lastTime) * 0.001; lastTime = time; cat.style.top = (Math.sin(angle) * 20) + "px"; cat.style.left = (Math.cos(angle) * 200) + "px"; requestAnimationFrame(animate); } requestAnimationFrame(animate); </script>
position: relative. top left , .
requestAnimationFrame animate , . animate requestAnimationFrame, . ( ) , 60 , .
DOM , . JavaScript, . requestAnimationFrame – , , , .
, ( lastTime), , . , , , .
Math.cos Math.sin. , , .
Math.cos Math.sin , (0, 0) . , 0 , , 2π ( 6.28) . Math.cos x , , Math.sin y. ( ) , 2π 0, – , a+2π , a.
angle , animation. image. top Math.sin 20 – . left Math.cos 200, .
. px , , ( , ems ). . – 0, .
JavaScript DOM. , JavaScript . DOM , . parentNode childNodes, .
, , - . , , color display. JavaScript style.
6. HTML . HTML :
<table> <tr> <th>name</th> <th>height</th> <th>country</th> </tr> <tr> <td>Kilimanjaro</td> <td>5895</td> <td>Tanzania</td> </tr> </table>
. : , .
, 6, MOUNTAINS.
buildTable, , , DOM, . , , , . Object.keys, , .
, , style.textAlign "right".
<style> /* */ table { border-collapse: collapse; } td, th { border: 1px solid black; padding: 3px 8px; } th { text-align: left; } </style> <script> function buildTable(data) { // } document.body.appendChild(buildTable(MOUNTAINS)); </script>
getElementsByTagName . , ( ) , .
, tagName. , . toLowerCase toUpperCase.
<h1> <span>span</span> .</h1> <p> <span></span>, <span></span> spans.</p> <script> function byTagName(node, tagName) { // } console.log(byTagName(document.body, "h1").length); // → 1 console.log(byTagName(document.body, "span").length); // → 3 var para = document.querySelector("p"); console.log(byTagName(para, "span").length); // → 2 </script>
, 
.
. - .
, . top left . , position.
<img src="img/cat.png" id="cat" style="position: absolute"> <img src="img/hat.png" id="hat" style="position: absolute"> <script> var cat = document.querySelector("#cat"); var hat = document.querySelector("#hat"); // Your code here. </script>
.
, , . , , , . style ():
<p><a href="."> </a></p> <p><a href="." style="color: green"> </a></p>
style (color), . : “color: red; border: none”.
. , display , .
<strong></strong>, <strong style="display: block"> </strong>, <strong style="display: none"> </strong>.
, – display: none . . , .
JavaScript style. , . – , - .
<p id="para" style="color: purple"> </p> <script> var para = document.getElementById("para"); console.log(para.style.color); para.style.color = "magenta"; </script>
, font-family. JavaScript ( style["font-family"]), , : style.fontFamily
HTML CSS (Cascading Style Sheets, ). – . :
<style> strong { font-style: italic; color: gray; } </style> <p> <strong> strong</strong> .</p>
«» , . , , , font-style .
, . font-weight: normal, , , . , style, .
CSS . .abc , “abc”. #xyz id “xyz” ( id ).
.subtle { color: gray; font-size: 80%; } #header { background: blue; color: white; } /* p, a b, id main */ pab#main { margin-bottom: 20px; }
, . , , . , pa , p .a, .
p > a {…}
, .
pa {…}
, , .
. , 2-3 . (, , ) – - DOM.
querySelectorAll, document, -, , , .
<p> <span class="animal"></span></p> <p> </p> <p> <span class="character"> <span class="animal"> </span></span></p> <p> .</p> <script> function count(selector) { return document.querySelectorAll(selector).length; } console.log(count("p")); // <p> // → 4 console.log(count(".animal")); // animal // → 2 console.log(count("p .animal")); // animal <p> // → 2 console.log(count("p > .animal")); // <p> // → 1 </script>
getElementsByTagName, querySelectorAll . , .
querySelector ( All) . , . , null, .
position . static, , . relative, , top left . absolute, «» – , . , left top , position static. , .
. , .
<p style="text-align: center"> <img src="img/cat.png" style="position: relative"> </p> <script> var cat = document.querySelector("img"); var angle = 0, lastTime = null; function animate(time) { if (lastTime != null) angle += (time - lastTime) * 0.001; lastTime = time; cat.style.top = (Math.sin(angle) * 20) + "px"; cat.style.left = (Math.cos(angle) * 200) + "px"; requestAnimationFrame(animate); } requestAnimationFrame(animate); </script>
position: relative. top left , .
requestAnimationFrame animate , . animate requestAnimationFrame, . ( ) , 60 , .
DOM , . JavaScript, . requestAnimationFrame – , , , .
, ( lastTime), , . , , , .
Math.cos Math.sin. , , .
Math.cos Math.sin , (0, 0) . , 0 , , 2π ( 6.28) . Math.cos x , , Math.sin y. ( ) , 2π 0, – , a+2π , a.
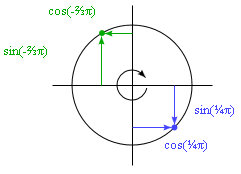
angle , animation. image. top Math.sin 20 – . left Math.cos 200, .
. px , , ( , ems ). . – 0, .
JavaScript DOM. , JavaScript . DOM , . parentNode childNodes, .
, , - . , , color display. JavaScript style.
6. HTML . HTML :
<table> <tr> <th>name</th> <th>height</th> <th>country</th> </tr> <tr> <td>Kilimanjaro</td> <td>5895</td> <td>Tanzania</td> </tr> </table>
. : , .
, 6, MOUNTAINS.
buildTable, , , DOM, . , , , . Object.keys, , .
, , style.textAlign "right".
<style> /* */ table { border-collapse: collapse; } td, th { border: 1px solid black; padding: 3px 8px; } th { text-align: left; } </style> <script> function buildTable(data) { // } document.body.appendChild(buildTable(MOUNTAINS)); </script>
getElementsByTagName . , ( ) , .
, tagName. , . toLowerCase toUpperCase.
<h1> <span>span</span> .</h1> <p> <span></span>, <span></span> spans.</p> <script> function byTagName(node, tagName) { // } console.log(byTagName(document.body, "h1").length); // → 1 console.log(byTagName(document.body, "span").length); // → 3 var para = document.querySelector("p"); console.log(byTagName(para, "span").length); // → 2 </script>
, 
.
. - .
, . top left . , position.
<img src="img/cat.png" id="cat" style="position: absolute"> <img src="img/hat.png" id="hat" style="position: absolute"> <script> var cat = document.querySelector("#cat"); var hat = document.querySelector("#hat"); // Your code here. </script>
.
, , . , , , . style ():
<p><a href="."> </a></p> <p><a href="." style="color: green"> </a></p>
style (color), . : “color: red; border: none”.
. , display , .
<strong></strong>, <strong style="display: block"> </strong>, <strong style="display: none"> </strong>.
, – display: none . . , .
JavaScript style. , . – , - .
<p id="para" style="color: purple"> </p> <script> var para = document.getElementById("para"); console.log(para.style.color); para.style.color = "magenta"; </script>
, font-family. JavaScript ( style["font-family"]), , : style.fontFamily
HTML CSS (Cascading Style Sheets, ). – . :
<style> strong { font-style: italic; color: gray; } </style> <p> <strong> strong</strong> .</p>
«» , . , , , font-style .
, . font-weight: normal, , , . , style, .
CSS . .abc , “abc”. #xyz id “xyz” ( id ).
.subtle { color: gray; font-size: 80%; } #header { background: blue; color: white; } /* p, a b, id main */ pab#main { margin-bottom: 20px; }
, . , , . , pa , p .a, .
p > a {…}
, .
pa {…}
, , .
. , 2-3 . (, , ) – - DOM.
querySelectorAll, document, -, , , .
<p> <span class="animal"></span></p> <p> </p> <p> <span class="character"> <span class="animal"> </span></span></p> <p> .</p> <script> function count(selector) { return document.querySelectorAll(selector).length; } console.log(count("p")); // <p> // → 4 console.log(count(".animal")); // animal // → 2 console.log(count("p .animal")); // animal <p> // → 2 console.log(count("p > .animal")); // <p> // → 1 </script>
getElementsByTagName, querySelectorAll . , .
querySelector ( All) . , . , null, .
position . static, , . relative, , top left . absolute, «» – , . , left top , position static. , .
. , .
<p style="text-align: center"> <img src="img/cat.png" style="position: relative"> </p> <script> var cat = document.querySelector("img"); var angle = 0, lastTime = null; function animate(time) { if (lastTime != null) angle += (time - lastTime) * 0.001; lastTime = time; cat.style.top = (Math.sin(angle) * 20) + "px"; cat.style.left = (Math.cos(angle) * 200) + "px"; requestAnimationFrame(animate); } requestAnimationFrame(animate); </script>
position: relative. top left , .
requestAnimationFrame animate , . animate requestAnimationFrame, . ( ) , 60 , .
DOM , . JavaScript, . requestAnimationFrame – , , , .
, ( lastTime), , . , , , .
Math.cos Math.sin. , , .
Math.cos Math.sin , (0, 0) . , 0 , , 2π ( 6.28) . Math.cos x , , Math.sin y. ( ) , 2π 0, – , a+2π , a.
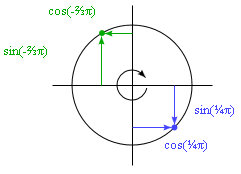
angle , animation. image. top Math.sin 20 – . left Math.cos 200, .
. px , , ( , ems ). . – 0, .
JavaScript DOM. , JavaScript . DOM , . parentNode childNodes, .
, , - . , , color display. JavaScript style.
6. HTML . HTML :
<table> <tr> <th>name</th> <th>height</th> <th>country</th> </tr> <tr> <td>Kilimanjaro</td> <td>5895</td> <td>Tanzania</td> </tr> </table>
. : , .
, 6, MOUNTAINS.
buildTable, , , DOM, . , , , . Object.keys, , .
, , style.textAlign "right".
<style> /* */ table { border-collapse: collapse; } td, th { border: 1px solid black; padding: 3px 8px; } th { text-align: left; } </style> <script> function buildTable(data) { // } document.body.appendChild(buildTable(MOUNTAINS)); </script>
getElementsByTagName . , ( ) , .
, tagName. , . toLowerCase toUpperCase.
<h1> <span>span</span> .</h1> <p> <span></span>, <span></span> spans.</p> <script> function byTagName(node, tagName) { // } console.log(byTagName(document.body, "h1").length); // → 1 console.log(byTagName(document.body, "span").length); // → 3 var para = document.querySelector("p"); console.log(byTagName(para, "span").length); // → 2 </script>
, 
.
. - .
, . top left . , position.
<img src="img/cat.png" id="cat" style="position: absolute"> <img src="img/hat.png" id="hat" style="position: absolute"> <script> var cat = document.querySelector("#cat"); var hat = document.querySelector("#hat"); // Your code here. </script>
.
, , . , , , . style ():
<p><a href="."> </a></p> <p><a href="." style="color: green"> </a></p>
style (color), . : “color: red; border: none”.
. , display , .
<strong></strong>, <strong style="display: block"> </strong>, <strong style="display: none"> </strong>.
, – display: none . . , .
JavaScript style. , . – , - .
<p id="para" style="color: purple"> </p> <script> var para = document.getElementById("para"); console.log(para.style.color); para.style.color = "magenta"; </script>
, font-family. JavaScript ( style["font-family"]), , : style.fontFamily
HTML CSS (Cascading Style Sheets, ). – . :
<style> strong { font-style: italic; color: gray; } </style> <p> <strong> strong</strong> .</p>
«» , . , , , font-style .
, . font-weight: normal, , , . , style, .
CSS . .abc , “abc”. #xyz id “xyz” ( id ).
.subtle { color: gray; font-size: 80%; } #header { background: blue; color: white; } /* p, a b, id main */ pab#main { margin-bottom: 20px; }
, . , , . , pa , p .a, .
p > a {…}
, .
pa {…}
, , .
. , 2-3 . (, , ) – - DOM.
querySelectorAll, document, -, , , .
<p> <span class="animal"></span></p> <p> </p> <p> <span class="character"> <span class="animal"> </span></span></p> <p> .</p> <script> function count(selector) { return document.querySelectorAll(selector).length; } console.log(count("p")); // <p> // → 4 console.log(count(".animal")); // animal // → 2 console.log(count("p .animal")); // animal <p> // → 2 console.log(count("p > .animal")); // <p> // → 1 </script>
getElementsByTagName, querySelectorAll . , .
querySelector ( All) . , . , null, .
position . static, , . relative, , top left . absolute, «» – , . , left top , position static. , .
. , .
<p style="text-align: center"> <img src="img/cat.png" style="position: relative"> </p> <script> var cat = document.querySelector("img"); var angle = 0, lastTime = null; function animate(time) { if (lastTime != null) angle += (time - lastTime) * 0.001; lastTime = time; cat.style.top = (Math.sin(angle) * 20) + "px"; cat.style.left = (Math.cos(angle) * 200) + "px"; requestAnimationFrame(animate); } requestAnimationFrame(animate); </script>
position: relative. top left , .
requestAnimationFrame animate , . animate requestAnimationFrame, . ( ) , 60 , .
DOM , . JavaScript, . requestAnimationFrame – , , , .
, ( lastTime), , . , , , .
Math.cos Math.sin. , , .
Math.cos Math.sin , (0, 0) . , 0 , , 2π ( 6.28) . Math.cos x , , Math.sin y. ( ) , 2π 0, – , a+2π , a.
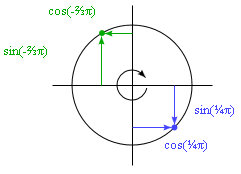
angle , animation. image. top Math.sin 20 – . left Math.cos 200, .
. px , , ( , ems ). . – 0, .
JavaScript DOM. , JavaScript . DOM , . parentNode childNodes, .
, , - . , , color display. JavaScript style.
6. HTML . HTML :
<table> <tr> <th>name</th> <th>height</th> <th>country</th> </tr> <tr> <td>Kilimanjaro</td> <td>5895</td> <td>Tanzania</td> </tr> </table>
. : , .
, 6, MOUNTAINS.
buildTable, , , DOM, . , , , . Object.keys, , .
, , style.textAlign "right".
<style> /* */ table { border-collapse: collapse; } td, th { border: 1px solid black; padding: 3px 8px; } th { text-align: left; } </style> <script> function buildTable(data) { // } document.body.appendChild(buildTable(MOUNTAINS)); </script>
getElementsByTagName . , ( ) , .
, tagName. , . toLowerCase toUpperCase.
<h1> <span>span</span> .</h1> <p> <span></span>, <span></span> spans.</p> <script> function byTagName(node, tagName) { // } console.log(byTagName(document.body, "h1").length); // → 1 console.log(byTagName(document.body, "span").length); // → 3 var para = document.querySelector("p"); console.log(byTagName(para, "span").length); // → 2 </script>
, 
.
. - .
, . top left . , position.
<img src="img/cat.png" id="cat" style="position: absolute"> <img src="img/hat.png" id="hat" style="position: absolute"> <script> var cat = document.querySelector("#cat"); var hat = document.querySelector("#hat"); // Your code here. </script>
.
, , . , , , . style ():
<p><a href="."> </a></p> <p><a href="." style="color: green"> </a></p>
style (color), . : “color: red; border: none”.
. , display , .
<strong></strong>, <strong style="display: block"> </strong>, <strong style="display: none"> </strong>.
, – display: none . . , .
JavaScript style. , . – , - .
<p id="para" style="color: purple"> </p> <script> var para = document.getElementById("para"); console.log(para.style.color); para.style.color = "magenta"; </script>
, font-family. JavaScript ( style["font-family"]), , : style.fontFamily
HTML CSS (Cascading Style Sheets, ). – . :
<style> strong { font-style: italic; color: gray; } </style> <p> <strong> strong</strong> .</p>
«» , . , , , font-style .
, . font-weight: normal, , , . , style, .
CSS . .abc , “abc”. #xyz id “xyz” ( id ).
.subtle { color: gray; font-size: 80%; } #header { background: blue; color: white; } /* p, a b, id main */ pab#main { margin-bottom: 20px; }
, . , , . , pa , p .a, .
p > a {…}
, .
pa {…}
, , .
. , 2-3 . (, , ) – - DOM.
querySelectorAll, document, -, , , .
<p> <span class="animal"></span></p> <p> </p> <p> <span class="character"> <span class="animal"> </span></span></p> <p> .</p> <script> function count(selector) { return document.querySelectorAll(selector).length; } console.log(count("p")); // <p> // → 4 console.log(count(".animal")); // animal // → 2 console.log(count("p .animal")); // animal <p> // → 2 console.log(count("p > .animal")); // <p> // → 1 </script>
getElementsByTagName, querySelectorAll . , .
querySelector ( All) . , . , null, .
position . static, , . relative, , top left . absolute, «» – , . , left top , position static. , .
. , .
<p style="text-align: center"> <img src="img/cat.png" style="position: relative"> </p> <script> var cat = document.querySelector("img"); var angle = 0, lastTime = null; function animate(time) { if (lastTime != null) angle += (time - lastTime) * 0.001; lastTime = time; cat.style.top = (Math.sin(angle) * 20) + "px"; cat.style.left = (Math.cos(angle) * 200) + "px"; requestAnimationFrame(animate); } requestAnimationFrame(animate); </script>
position: relative. top left , .
requestAnimationFrame animate , . animate requestAnimationFrame, . ( ) , 60 , .
DOM , . JavaScript, . requestAnimationFrame – , , , .
, ( lastTime), , . , , , .
Math.cos Math.sin. , , .
Math.cos Math.sin , (0, 0) . , 0 , , 2π ( 6.28) . Math.cos x , , Math.sin y. ( ) , 2π 0, – , a+2π , a.
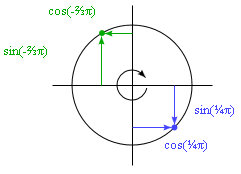
angle , animation. image. top Math.sin 20 – . left Math.cos 200, .
. px , , ( , ems ). . – 0, .
JavaScript DOM. , JavaScript . DOM , . parentNode childNodes, .
, , - . , , color display. JavaScript style.
6. HTML . HTML :
<table> <tr> <th>name</th> <th>height</th> <th>country</th> </tr> <tr> <td>Kilimanjaro</td> <td>5895</td> <td>Tanzania</td> </tr> </table>
. : , .
, 6, MOUNTAINS.
buildTable, , , DOM, . , , , . Object.keys, , .
, , style.textAlign "right".
<style> /* */ table { border-collapse: collapse; } td, th { border: 1px solid black; padding: 3px 8px; } th { text-align: left; } </style> <script> function buildTable(data) { // } document.body.appendChild(buildTable(MOUNTAINS)); </script>
getElementsByTagName . , ( ) , .
, tagName. , . toLowerCase toUpperCase.
<h1> <span>span</span> .</h1> <p> <span></span>, <span></span> spans.</p> <script> function byTagName(node, tagName) { // } console.log(byTagName(document.body, "h1").length); // → 1 console.log(byTagName(document.body, "span").length); // → 3 var para = document.querySelector("p"); console.log(byTagName(para, "span").length); // → 2 </script>
, 
.
. - .
, . top left . , position.
<img src="img/cat.png" id="cat" style="position: absolute"> <img src="img/hat.png" id="hat" style="position: absolute"> <script> var cat = document.querySelector("#cat"); var hat = document.querySelector("#hat"); // Your code here. </script>
.
, , . , , , . style ():
<p><a href="."> </a></p> <p><a href="." style="color: green"> </a></p>
style (color), . : “color: red; border: none”.
. , display , .
<strong></strong>, <strong style="display: block"> </strong>, <strong style="display: none"> </strong>.
, – display: none . . , .
JavaScript style. , . – , - .
<p id="para" style="color: purple"> </p> <script> var para = document.getElementById("para"); console.log(para.style.color); para.style.color = "magenta"; </script>
, font-family. JavaScript ( style["font-family"]), , : style.fontFamily
HTML CSS (Cascading Style Sheets, ). – . :
<style> strong { font-style: italic; color: gray; } </style> <p> <strong> strong</strong> .</p>
«» , . , , , font-style .
, . font-weight: normal, , , . , style, .
CSS . .abc , “abc”. #xyz id “xyz” ( id ).
.subtle { color: gray; font-size: 80%; } #header { background: blue; color: white; } /* p, a b, id main */ pab#main { margin-bottom: 20px; }
, . , , . , pa , p .a, .
p > a {…}
, .
pa {…}
, , .
. , 2-3 . (, , ) – - DOM.
querySelectorAll, document, -, , , .
<p> <span class="animal"></span></p> <p> </p> <p> <span class="character"> <span class="animal"> </span></span></p> <p> .</p> <script> function count(selector) { return document.querySelectorAll(selector).length; } console.log(count("p")); // <p> // → 4 console.log(count(".animal")); // animal // → 2 console.log(count("p .animal")); // animal <p> // → 2 console.log(count("p > .animal")); // <p> // → 1 </script>
getElementsByTagName, querySelectorAll . , .
querySelector ( All) . , . , null, .
position . static, , . relative, , top left . absolute, «» – , . , left top , position static. , .
. , .
<p style="text-align: center"> <img src="img/cat.png" style="position: relative"> </p> <script> var cat = document.querySelector("img"); var angle = 0, lastTime = null; function animate(time) { if (lastTime != null) angle += (time - lastTime) * 0.001; lastTime = time; cat.style.top = (Math.sin(angle) * 20) + "px"; cat.style.left = (Math.cos(angle) * 200) + "px"; requestAnimationFrame(animate); } requestAnimationFrame(animate); </script>
position: relative. top left , .
requestAnimationFrame animate , . animate requestAnimationFrame, . ( ) , 60 , .
DOM , . JavaScript, . requestAnimationFrame – , , , .
, ( lastTime), , . , , , .
Math.cos Math.sin. , , .
Math.cos Math.sin , (0, 0) . , 0 , , 2π ( 6.28) . Math.cos x , , Math.sin y. ( ) , 2π 0, – , a+2π , a.
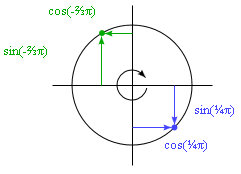
angle , animation. image. top Math.sin 20 – . left Math.cos 200, .
. px , , ( , ems ). . – 0, .
JavaScript DOM. , JavaScript . DOM , . parentNode childNodes, .
, , - . , , color display. JavaScript style.
6. HTML . HTML :
<table> <tr> <th>name</th> <th>height</th> <th>country</th> </tr> <tr> <td>Kilimanjaro</td> <td>5895</td> <td>Tanzania</td> </tr> </table>
. : , .
, 6, MOUNTAINS.
buildTable, , , DOM, . , , , . Object.keys, , .
, , style.textAlign "right".
<style> /* */ table { border-collapse: collapse; } td, th { border: 1px solid black; padding: 3px 8px; } th { text-align: left; } </style> <script> function buildTable(data) { // } document.body.appendChild(buildTable(MOUNTAINS)); </script>
getElementsByTagName . , ( ) , .
, tagName. , . toLowerCase toUpperCase.
<h1> <span>span</span> .</h1> <p> <span></span>, <span></span> spans.</p> <script> function byTagName(node, tagName) { // } console.log(byTagName(document.body, "h1").length); // → 1 console.log(byTagName(document.body, "span").length); // → 3 var para = document.querySelector("p"); console.log(byTagName(para, "span").length); // → 2 </script>
, 
.
. - .
, . top left . , position.
<img src="img/cat.png" id="cat" style="position: absolute"> <img src="img/hat.png" id="hat" style="position: absolute"> <script> var cat = document.querySelector("#cat"); var hat = document.querySelector("#hat"); // Your code here. </script>
.
, , . , , , . style ():
<p><a href="."> </a></p> <p><a href="." style="color: green"> </a></p>
style (color), . : “color: red; border: none”.
. , display , .
<strong></strong>, <strong style="display: block"> </strong>, <strong style="display: none"> </strong>.
, – display: none . . , .
JavaScript style. , . – , - .
<p id="para" style="color: purple"> </p> <script> var para = document.getElementById("para"); console.log(para.style.color); para.style.color = "magenta"; </script>
, font-family. JavaScript ( style["font-family"]), , : style.fontFamily
HTML CSS (Cascading Style Sheets, ). – . :
<style> strong { font-style: italic; color: gray; } </style> <p> <strong> strong</strong> .</p>
«» , . , , , font-style .
, . font-weight: normal, , , . , style, .
CSS . .abc , “abc”. #xyz id “xyz” ( id ).
.subtle { color: gray; font-size: 80%; } #header { background: blue; color: white; } /* p, a b, id main */ pab#main { margin-bottom: 20px; }
, . , , . , pa , p .a, .
p > a {…}
, .
pa {…}
, , .
. , 2-3 . (, , ) – - DOM.
querySelectorAll, document, -, , , .
<p> <span class="animal"></span></p> <p> </p> <p> <span class="character"> <span class="animal"> </span></span></p> <p> .</p> <script> function count(selector) { return document.querySelectorAll(selector).length; } console.log(count("p")); // <p> // → 4 console.log(count(".animal")); // animal // → 2 console.log(count("p .animal")); // animal <p> // → 2 console.log(count("p > .animal")); // <p> // → 1 </script>
getElementsByTagName, querySelectorAll . , .
querySelector ( All) . , . , null, .
position . static, , . relative, , top left . absolute, «» – , . , left top , position static. , .
. , .
<p style="text-align: center"> <img src="img/cat.png" style="position: relative"> </p> <script> var cat = document.querySelector("img"); var angle = 0, lastTime = null; function animate(time) { if (lastTime != null) angle += (time - lastTime) * 0.001; lastTime = time; cat.style.top = (Math.sin(angle) * 20) + "px"; cat.style.left = (Math.cos(angle) * 200) + "px"; requestAnimationFrame(animate); } requestAnimationFrame(animate); </script>
position: relative. top left , .
requestAnimationFrame animate , . animate requestAnimationFrame, . ( ) , 60 , .
DOM , . JavaScript, . requestAnimationFrame – , , , .
, ( lastTime), , . , , , .
Math.cos Math.sin. , , .
Math.cos Math.sin , (0, 0) . , 0 , , 2π ( 6.28) . Math.cos x , , Math.sin y. ( ) , 2π 0, – , a+2π , a.
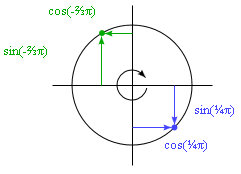
angle , animation. image. top Math.sin 20 – . left Math.cos 200, .
. px , , ( , ems ). . – 0, .
JavaScript DOM. , JavaScript . DOM , . parentNode childNodes, .
, , - . , , color display. JavaScript style.
6. HTML . HTML :
<table> <tr> <th>name</th> <th>height</th> <th>country</th> </tr> <tr> <td>Kilimanjaro</td> <td>5895</td> <td>Tanzania</td> </tr> </table>
. : , .
, 6, MOUNTAINS.
buildTable, , , DOM, . , , , . Object.keys, , .
, , style.textAlign "right".
<style> /* */ table { border-collapse: collapse; } td, th { border: 1px solid black; padding: 3px 8px; } th { text-align: left; } </style> <script> function buildTable(data) { // } document.body.appendChild(buildTable(MOUNTAINS)); </script>
getElementsByTagName . , ( ) , .
, tagName. , . toLowerCase toUpperCase.
<h1> <span>span</span> .</h1> <p> <span></span>, <span></span> spans.</p> <script> function byTagName(node, tagName) { // } console.log(byTagName(document.body, "h1").length); // → 1 console.log(byTagName(document.body, "span").length); // → 3 var para = document.querySelector("p"); console.log(byTagName(para, "span").length); // → 2 </script>
, 
.
. - .
, . top left . , position.
<img src="img/cat.png" id="cat" style="position: absolute"> <img src="img/hat.png" id="hat" style="position: absolute"> <script> var cat = document.querySelector("#cat"); var hat = document.querySelector("#hat"); // Your code here. </script>
.
, , . , , , . style ():
<p><a href="."> </a></p> <p><a href="." style="color: green"> </a></p>
style (color), . : “color: red; border: none”.
. , display , .
<strong></strong>, <strong style="display: block"> </strong>, <strong style="display: none"> </strong>.
, – display: none . . , .
JavaScript style. , . – , - .
<p id="para" style="color: purple"> </p> <script> var para = document.getElementById("para"); console.log(para.style.color); para.style.color = "magenta"; </script>
, font-family. JavaScript ( style["font-family"]), , : style.fontFamily
HTML CSS (Cascading Style Sheets, ). – . :
<style> strong { font-style: italic; color: gray; } </style> <p> <strong> strong</strong> .</p>
«» , . , , , font-style .
, . font-weight: normal, , , . , style, .
CSS . .abc , “abc”. #xyz id “xyz” ( id ).
.subtle { color: gray; font-size: 80%; } #header { background: blue; color: white; } /* p, a b, id main */ pab#main { margin-bottom: 20px; }
, . , , . , pa , p .a, .
p > a {…}
, .
pa {…}
, , .
. , 2-3 . (, , ) – - DOM.
querySelectorAll, document, -, , , .
<p> <span class="animal"></span></p> <p> </p> <p> <span class="character"> <span class="animal"> </span></span></p> <p> .</p> <script> function count(selector) { return document.querySelectorAll(selector).length; } console.log(count("p")); // <p> // → 4 console.log(count(".animal")); // animal // → 2 console.log(count("p .animal")); // animal <p> // → 2 console.log(count("p > .animal")); // <p> // → 1 </script>
getElementsByTagName, querySelectorAll . , .
querySelector ( All) . , . , null, .
position . static, , . relative, , top left . absolute, «» – , . , left top , position static. , .
. , .
<p style="text-align: center"> <img src="img/cat.png" style="position: relative"> </p> <script> var cat = document.querySelector("img"); var angle = 0, lastTime = null; function animate(time) { if (lastTime != null) angle += (time - lastTime) * 0.001; lastTime = time; cat.style.top = (Math.sin(angle) * 20) + "px"; cat.style.left = (Math.cos(angle) * 200) + "px"; requestAnimationFrame(animate); } requestAnimationFrame(animate); </script>
position: relative. top left , .
requestAnimationFrame animate , . animate requestAnimationFrame, . ( ) , 60 , .
DOM , . JavaScript, . requestAnimationFrame – , , , .
, ( lastTime), , . , , , .
Math.cos Math.sin. , , .
Math.cos Math.sin , (0, 0) . , 0 , , 2π ( 6.28) . Math.cos x , , Math.sin y. ( ) , 2π 0, – , a+2π , a.
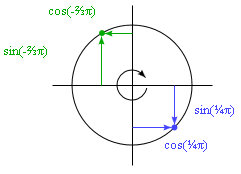
angle , animation. image. top Math.sin 20 – . left Math.cos 200, .
. px , , ( , ems ). . – 0, .
JavaScript DOM. , JavaScript . DOM , . parentNode childNodes, .
, , - . , , color display. JavaScript style.
6. HTML . HTML :
<table> <tr> <th>name</th> <th>height</th> <th>country</th> </tr> <tr> <td>Kilimanjaro</td> <td>5895</td> <td>Tanzania</td> </tr> </table>
. : , .
, 6, MOUNTAINS.
buildTable, , , DOM, . , , , . Object.keys, , .
, , style.textAlign "right".
<style> /* */ table { border-collapse: collapse; } td, th { border: 1px solid black; padding: 3px 8px; } th { text-align: left; } </style> <script> function buildTable(data) { // } document.body.appendChild(buildTable(MOUNTAINS)); </script>
getElementsByTagName . , ( ) , .
, tagName. , . toLowerCase toUpperCase.
<h1> <span>span</span> .</h1> <p> <span></span>, <span></span> spans.</p> <script> function byTagName(node, tagName) { // } console.log(byTagName(document.body, "h1").length); // → 1 console.log(byTagName(document.body, "span").length); // → 3 var para = document.querySelector("p"); console.log(byTagName(para, "span").length); // → 2 </script>
, 
.
. - .
, . top left . , position.
<img src="img/cat.png" id="cat" style="position: absolute"> <img src="img/hat.png" id="hat" style="position: absolute"> <script> var cat = document.querySelector("#cat"); var hat = document.querySelector("#hat"); // Your code here. </script>
.
, , . , , , . style ():
<p><a href="."> </a></p> <p><a href="." style="color: green"> </a></p>
style (color), . : “color: red; border: none”.
. , display , .
<strong></strong>, <strong style="display: block"> </strong>, <strong style="display: none"> </strong>.
, – display: none . . , .
JavaScript style. , . – , - .
<p id="para" style="color: purple"> </p> <script> var para = document.getElementById("para"); console.log(para.style.color); para.style.color = "magenta"; </script>
, font-family. JavaScript ( style["font-family"]), , : style.fontFamily
HTML CSS (Cascading Style Sheets, ). – . :
<style> strong { font-style: italic; color: gray; } </style> <p> <strong> strong</strong> .</p>
«» , . , , , font-style .
, . font-weight: normal, , , . , style, .
CSS . .abc , “abc”. #xyz id “xyz” ( id ).
.subtle { color: gray; font-size: 80%; } #header { background: blue; color: white; } /* p, a b, id main */ pab#main { margin-bottom: 20px; }
, . , , . , pa , p .a, .
p > a {…}
, .
pa {…}
, , .
. , 2-3 . (, , ) – - DOM.
querySelectorAll, document, -, , , .
<p> <span class="animal"></span></p> <p> </p> <p> <span class="character"> <span class="animal"> </span></span></p> <p> .</p> <script> function count(selector) { return document.querySelectorAll(selector).length; } console.log(count("p")); // <p> // → 4 console.log(count(".animal")); // animal // → 2 console.log(count("p .animal")); // animal <p> // → 2 console.log(count("p > .animal")); // <p> // → 1 </script>
getElementsByTagName, querySelectorAll . , .
querySelector ( All) . , . , null, .
position . static, , . relative, , top left . absolute, «» – , . , left top , position static. , .
. , .
<p style="text-align: center"> <img src="img/cat.png" style="position: relative"> </p> <script> var cat = document.querySelector("img"); var angle = 0, lastTime = null; function animate(time) { if (lastTime != null) angle += (time - lastTime) * 0.001; lastTime = time; cat.style.top = (Math.sin(angle) * 20) + "px"; cat.style.left = (Math.cos(angle) * 200) + "px"; requestAnimationFrame(animate); } requestAnimationFrame(animate); </script>
position: relative. top left , .
requestAnimationFrame animate , . animate requestAnimationFrame, . ( ) , 60 , .
DOM , . JavaScript, . requestAnimationFrame – , , , .
, ( lastTime), , . , , , .
Math.cos Math.sin. , , .
Math.cos Math.sin , (0, 0) . , 0 , , 2π ( 6.28) . Math.cos x , , Math.sin y. ( ) , 2π 0, – , a+2π , a.
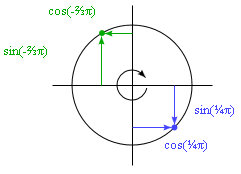
angle , animation. image. top Math.sin 20 – . left Math.cos 200, .
. px , , ( , ems ). . – 0, .
JavaScript DOM. , JavaScript . DOM , . parentNode childNodes, .
, , - . , , color display. JavaScript style.
6. HTML . HTML :
<table> <tr> <th>name</th> <th>height</th> <th>country</th> </tr> <tr> <td>Kilimanjaro</td> <td>5895</td> <td>Tanzania</td> </tr> </table>
. : , .
, 6, MOUNTAINS.
buildTable, , , DOM, . , , , . Object.keys, , .
, , style.textAlign "right".
<style> /* */ table { border-collapse: collapse; } td, th { border: 1px solid black; padding: 3px 8px; } th { text-align: left; } </style> <script> function buildTable(data) { // } document.body.appendChild(buildTable(MOUNTAINS)); </script>
getElementsByTagName . , ( ) , .
, tagName. , . toLowerCase toUpperCase.
<h1> <span>span</span> .</h1> <p> <span></span>, <span></span> spans.</p> <script> function byTagName(node, tagName) { // } console.log(byTagName(document.body, "h1").length); // → 1 console.log(byTagName(document.body, "span").length); // → 3 var para = document.querySelector("p"); console.log(byTagName(para, "span").length); // → 2 </script>
, 
.
. - .
, . top left . , position.
<img src="img/cat.png" id="cat" style="position: absolute"> <img src="img/hat.png" id="hat" style="position: absolute"> <script> var cat = document.querySelector("#cat"); var hat = document.querySelector("#hat"); // Your code here. </script>
.
, , . , , , . style ():
<p><a href="."> </a></p> <p><a href="." style="color: green"> </a></p>
style (color), . : “color: red; border: none”.
. , display , .
<strong></strong>, <strong style="display: block"> </strong>, <strong style="display: none"> </strong>.
, – display: none . . , .
JavaScript style. , . – , - .
<p id="para" style="color: purple"> </p> <script> var para = document.getElementById("para"); console.log(para.style.color); para.style.color = "magenta"; </script>
, font-family. JavaScript ( style["font-family"]), , : style.fontFamily
HTML CSS (Cascading Style Sheets, ). – . :
<style> strong { font-style: italic; color: gray; } </style> <p> <strong> strong</strong> .</p>
«» , . , , , font-style .
, . font-weight: normal, , , . , style, .
CSS . .abc , “abc”. #xyz id “xyz” ( id ).
.subtle { color: gray; font-size: 80%; } #header { background: blue; color: white; } /* p, a b, id main */ pab#main { margin-bottom: 20px; }
, . , , . , pa , p .a, .
p > a {…}
, .
pa {…}
, , .
. , 2-3 . (, , ) – - DOM.
querySelectorAll, document, -, , , .
<p> <span class="animal"></span></p> <p> </p> <p> <span class="character"> <span class="animal"> </span></span></p> <p> .</p> <script> function count(selector) { return document.querySelectorAll(selector).length; } console.log(count("p")); // <p> // → 4 console.log(count(".animal")); // animal // → 2 console.log(count("p .animal")); // animal <p> // → 2 console.log(count("p > .animal")); // <p> // → 1 </script>
getElementsByTagName, querySelectorAll . , .
querySelector ( All) . , . , null, .
position . static, , . relative, , top left . absolute, «» – , . , left top , position static. , .
. , .
<p style="text-align: center"> <img src="img/cat.png" style="position: relative"> </p> <script> var cat = document.querySelector("img"); var angle = 0, lastTime = null; function animate(time) { if (lastTime != null) angle += (time - lastTime) * 0.001; lastTime = time; cat.style.top = (Math.sin(angle) * 20) + "px"; cat.style.left = (Math.cos(angle) * 200) + "px"; requestAnimationFrame(animate); } requestAnimationFrame(animate); </script>
position: relative. top left , .
requestAnimationFrame animate , . animate requestAnimationFrame, . ( ) , 60 , .
DOM , . JavaScript, . requestAnimationFrame – , , , .
, ( lastTime), , . , , , .
Math.cos Math.sin. , , .
Math.cos Math.sin , (0, 0) . , 0 , , 2π ( 6.28) . Math.cos x , , Math.sin y. ( ) , 2π 0, – , a+2π , a.
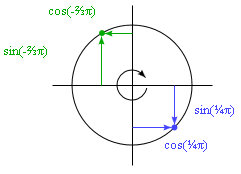
angle , animation. image. top Math.sin 20 – . left Math.cos 200, .
. px , , ( , ems ). . – 0, .
JavaScript DOM. , JavaScript . DOM , . parentNode childNodes, .
, , - . , , color display. JavaScript style.
6. HTML . HTML :
<table> <tr> <th>name</th> <th>height</th> <th>country</th> </tr> <tr> <td>Kilimanjaro</td> <td>5895</td> <td>Tanzania</td> </tr> </table>
. : , .
, 6, MOUNTAINS.
buildTable, , , DOM, . , , , . Object.keys, , .
, , style.textAlign "right".
<style> /* */ table { border-collapse: collapse; } td, th { border: 1px solid black; padding: 3px 8px; } th { text-align: left; } </style> <script> function buildTable(data) { // } document.body.appendChild(buildTable(MOUNTAINS)); </script>
getElementsByTagName . , ( ) , .
, tagName. , . toLowerCase toUpperCase.
<h1> <span>span</span> .</h1> <p> <span></span>, <span></span> spans.</p> <script> function byTagName(node, tagName) { // } console.log(byTagName(document.body, "h1").length); // → 1 console.log(byTagName(document.body, "span").length); // → 3 var para = document.querySelector("p"); console.log(byTagName(para, "span").length); // → 2 </script>
, 
.
. - .
, . top left . , position.
<img src="img/cat.png" id="cat" style="position: absolute"> <img src="img/hat.png" id="hat" style="position: absolute"> <script> var cat = document.querySelector("#cat"); var hat = document.querySelector("#hat"); // Your code here. </script>
.
, , . , , , . style ():
<p><a href="."> </a></p> <p><a href="." style="color: green"> </a></p>
style (color), . : “color: red; border: none”.
. , display , .
<strong></strong>, <strong style="display: block"> </strong>, <strong style="display: none"> </strong>.
, – display: none . . , .
JavaScript style. , . – , - .
<p id="para" style="color: purple"> </p> <script> var para = document.getElementById("para"); console.log(para.style.color); para.style.color = "magenta"; </script>
, font-family. JavaScript ( style["font-family"]), , : style.fontFamily
HTML CSS (Cascading Style Sheets, ). – . :
<style> strong { font-style: italic; color: gray; } </style> <p> <strong> strong</strong> .</p>
«» , . , , , font-style .
, . font-weight: normal, , , . , style, .
CSS . .abc , “abc”. #xyz id “xyz” ( id ).
.subtle { color: gray; font-size: 80%; } #header { background: blue; color: white; } /* p, a b, id main */ pab#main { margin-bottom: 20px; }
, . , , . , pa , p .a, .
p > a {…}
, .
pa {…}
, , .
. , 2-3 . (, , ) – - DOM.
querySelectorAll, document, -, , , .
<p> <span class="animal"></span></p> <p> </p> <p> <span class="character"> <span class="animal"> </span></span></p> <p> .</p> <script> function count(selector) { return document.querySelectorAll(selector).length; } console.log(count("p")); // <p> // → 4 console.log(count(".animal")); // animal // → 2 console.log(count("p .animal")); // animal <p> // → 2 console.log(count("p > .animal")); // <p> // → 1 </script>
getElementsByTagName, querySelectorAll . , .
querySelector ( All) . , . , null, .
position . static, , . relative, , top left . absolute, «» – , . , left top , position static. , .
. , .
<p style="text-align: center"> <img src="img/cat.png" style="position: relative"> </p> <script> var cat = document.querySelector("img"); var angle = 0, lastTime = null; function animate(time) { if (lastTime != null) angle += (time - lastTime) * 0.001; lastTime = time; cat.style.top = (Math.sin(angle) * 20) + "px"; cat.style.left = (Math.cos(angle) * 200) + "px"; requestAnimationFrame(animate); } requestAnimationFrame(animate); </script>
position: relative. top left , .
requestAnimationFrame animate , . animate requestAnimationFrame, . ( ) , 60 , .
DOM , . JavaScript, . requestAnimationFrame – , , , .
, ( lastTime), , . , , , .
Math.cos Math.sin. , , .
Math.cos Math.sin , (0, 0) . , 0 , , 2π ( 6.28) . Math.cos x , , Math.sin y. ( ) , 2π 0, – , a+2π , a.
angle , animation. image. top Math.sin 20 – . left Math.cos 200, .
. px , , ( , ems ). . – 0, .
JavaScript DOM. , JavaScript . DOM , . parentNode childNodes, .
, , - . , , color display. JavaScript style.
6. HTML . HTML :
<table> <tr> <th>name</th> <th>height</th> <th>country</th> </tr> <tr> <td>Kilimanjaro</td> <td>5895</td> <td>Tanzania</td> </tr> </table>
. : , .
, 6, MOUNTAINS.
buildTable, , , DOM, . , , , . Object.keys, , .
, , style.textAlign "right".
<style> /* */ table { border-collapse: collapse; } td, th { border: 1px solid black; padding: 3px 8px; } th { text-align: left; } </style> <script> function buildTable(data) { // } document.body.appendChild(buildTable(MOUNTAINS)); </script>
getElementsByTagName . , ( ) , .
, tagName. , . toLowerCase toUpperCase.
<h1> <span>span</span> .</h1> <p> <span></span>, <span></span> spans.</p> <script> function byTagName(node, tagName) { // } console.log(byTagName(document.body, "h1").length); // → 1 console.log(byTagName(document.body, "span").length); // → 3 var para = document.querySelector("p"); console.log(byTagName(para, "span").length); // → 2 </script>
, 
.
. - .
, . top left . , position.
<img src="img/cat.png" id="cat" style="position: absolute"> <img src="img/hat.png" id="hat" style="position: absolute"> <script> var cat = document.querySelector("#cat"); var hat = document.querySelector("#hat"); // Your code here. </script>
.
, , . , , , . style ():
<p><a href="."> </a></p> <p><a href="." style="color: green"> </a></p>
style (color), . : “color: red; border: none”.
. , display , .
<strong></strong>, <strong style="display: block"> </strong>, <strong style="display: none"> </strong>.
, – display: none . . , .
JavaScript style. , . – , - .
<p id="para" style="color: purple"> </p> <script> var para = document.getElementById("para"); console.log(para.style.color); para.style.color = "magenta"; </script>
, font-family. JavaScript ( style["font-family"]), , : style.fontFamily
HTML CSS (Cascading Style Sheets, ). – . :
<style> strong { font-style: italic; color: gray; } </style> <p> <strong> strong</strong> .</p>
«» , . , , , font-style .
, . font-weight: normal, , , . , style, .
CSS . .abc , “abc”. #xyz id “xyz” ( id ).
.subtle { color: gray; font-size: 80%; } #header { background: blue; color: white; } /* p, a b, id main */ pab#main { margin-bottom: 20px; }
, . , , . , pa , p .a, .
p > a {…}
, .
pa {…}
, , .
. , 2-3 . (, , ) – - DOM.
querySelectorAll, document, -, , , .
<p> <span class="animal"></span></p> <p> </p> <p> <span class="character"> <span class="animal"> </span></span></p> <p> .</p> <script> function count(selector) { return document.querySelectorAll(selector).length; } console.log(count("p")); // <p> // → 4 console.log(count(".animal")); // animal // → 2 console.log(count("p .animal")); // animal <p> // → 2 console.log(count("p > .animal")); // <p> // → 1 </script>
getElementsByTagName, querySelectorAll . , .
querySelector ( All) . , . , null, .
position . static, , . relative, , top left . absolute, «» – , . , left top , position static. , .
. , .
<p style="text-align: center"> <img src="img/cat.png" style="position: relative"> </p> <script> var cat = document.querySelector("img"); var angle = 0, lastTime = null; function animate(time) { if (lastTime != null) angle += (time - lastTime) * 0.001; lastTime = time; cat.style.top = (Math.sin(angle) * 20) + "px"; cat.style.left = (Math.cos(angle) * 200) + "px"; requestAnimationFrame(animate); } requestAnimationFrame(animate); </script>
position: relative. top left , .
requestAnimationFrame animate , . animate requestAnimationFrame, . ( ) , 60 , .
DOM , . JavaScript, . requestAnimationFrame – , , , .
, ( lastTime), , . , , , .
Math.cos Math.sin. , , .
Math.cos Math.sin , (0, 0) . , 0 , , 2π ( 6.28) . Math.cos x , , Math.sin y. ( ) , 2π 0, – , a+2π , a.
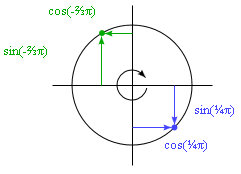
angle , animation. image. top Math.sin 20 – . left Math.cos 200, .
. px , , ( , ems ). . – 0, .
JavaScript DOM. , JavaScript . DOM , . parentNode childNodes, .
, , - . , , color display. JavaScript style.
6. HTML . HTML :
<table> <tr> <th>name</th> <th>height</th> <th>country</th> </tr> <tr> <td>Kilimanjaro</td> <td>5895</td> <td>Tanzania</td> </tr> </table>
. : , .
, 6, MOUNTAINS.
buildTable, , , DOM, . , , , . Object.keys, , .
, , style.textAlign "right".
<style> /* */ table { border-collapse: collapse; } td, th { border: 1px solid black; padding: 3px 8px; } th { text-align: left; } </style> <script> function buildTable(data) { // } document.body.appendChild(buildTable(MOUNTAINS)); </script>
getElementsByTagName . , ( ) , .
, tagName. , . toLowerCase toUpperCase.
<h1> <span>span</span> .</h1> <p> <span></span>, <span></span> spans.</p> <script> function byTagName(node, tagName) { // } console.log(byTagName(document.body, "h1").length); // → 1 console.log(byTagName(document.body, "span").length); // → 3 var para = document.querySelector("p"); console.log(byTagName(para, "span").length); // → 2 </script>
, 
.
. - .
, . top left . , position.
<img src="img/cat.png" id="cat" style="position: absolute"> <img src="img/hat.png" id="hat" style="position: absolute"> <script> var cat = document.querySelector("#cat"); var hat = document.querySelector("#hat"); // Your code here. </script>
.
, , . , , , . style ():
<p><a href="."> </a></p> <p><a href="." style="color: green"> </a></p>
style (color), . : “color: red; border: none”.
. , display , .
<strong></strong>, <strong style="display: block"> </strong>, <strong style="display: none"> </strong>.
, – display: none . . , .
JavaScript style. , . – , - .
<p id="para" style="color: purple"> </p> <script> var para = document.getElementById("para"); console.log(para.style.color); para.style.color = "magenta"; </script>
, font-family. JavaScript ( style["font-family"]), , : style.fontFamily
HTML CSS (Cascading Style Sheets, ). – . :
<style> strong { font-style: italic; color: gray; } </style> <p> <strong> strong</strong> .</p>
«» , . , , , font-style .
, . font-weight: normal, , , . , style, .
CSS . .abc , “abc”. #xyz id “xyz” ( id ).
.subtle { color: gray; font-size: 80%; } #header { background: blue; color: white; } /* p, a b, id main */ pab#main { margin-bottom: 20px; }
, . , , . , pa , p .a, .
p > a {…}
, .
pa {…}
, , .
. , 2-3 . (, , ) – - DOM.
querySelectorAll, document, -, , , .
<p> <span class="animal"></span></p> <p> </p> <p> <span class="character"> <span class="animal"> </span></span></p> <p> .</p> <script> function count(selector) { return document.querySelectorAll(selector).length; } console.log(count("p")); // <p> // → 4 console.log(count(".animal")); // animal // → 2 console.log(count("p .animal")); // animal <p> // → 2 console.log(count("p > .animal")); // <p> // → 1 </script>
getElementsByTagName, querySelectorAll . , .
querySelector ( All) . , . , null, .
position . static, , . relative, , top left . absolute, «» – , . , left top , position static. , .
. , .
<p style="text-align: center"> <img src="img/cat.png" style="position: relative"> </p> <script> var cat = document.querySelector("img"); var angle = 0, lastTime = null; function animate(time) { if (lastTime != null) angle += (time - lastTime) * 0.001; lastTime = time; cat.style.top = (Math.sin(angle) * 20) + "px"; cat.style.left = (Math.cos(angle) * 200) + "px"; requestAnimationFrame(animate); } requestAnimationFrame(animate); </script>
position: relative. top left , .
requestAnimationFrame animate , . animate requestAnimationFrame, . ( ) , 60 , .
DOM , . JavaScript, . requestAnimationFrame – , , , .
, ( lastTime), , . , , , .
Math.cos Math.sin. , , .
Math.cos Math.sin , (0, 0) . , 0 , , 2π ( 6.28) . Math.cos x , , Math.sin y. ( ) , 2π 0, – , a+2π , a.
angle , animation. image. top Math.sin 20 – . left Math.cos 200, .
. px , , ( , ems ). . – 0, .
JavaScript DOM. , JavaScript . DOM , . parentNode childNodes, .
, , - . , , color display. JavaScript style.
6. HTML . HTML :
<table> <tr> <th>name</th> <th>height</th> <th>country</th> </tr> <tr> <td>Kilimanjaro</td> <td>5895</td> <td>Tanzania</td> </tr> </table>
. : , .
, 6, MOUNTAINS.
buildTable, , , DOM, . , , , . Object.keys, , .
, , style.textAlign "right".
<style> /* */ table { border-collapse: collapse; } td, th { border: 1px solid black; padding: 3px 8px; } th { text-align: left; } </style> <script> function buildTable(data) { // } document.body.appendChild(buildTable(MOUNTAINS)); </script>
getElementsByTagName . , ( ) , .
, tagName. , . toLowerCase toUpperCase.
<h1> <span>span</span> .</h1> <p> <span></span>, <span></span> spans.</p> <script> function byTagName(node, tagName) { // } console.log(byTagName(document.body, "h1").length); // → 1 console.log(byTagName(document.body, "span").length); // → 3 var para = document.querySelector("p"); console.log(byTagName(para, "span").length); // → 2 </script>
, 
.
. - .
, . top left . , position.
<img src="img/cat.png" id="cat" style="position: absolute"> <img src="img/hat.png" id="hat" style="position: absolute"> <script> var cat = document.querySelector("#cat"); var hat = document.querySelector("#hat"); // Your code here. </script>
.
, , . , , , . style ():
<p><a href="."> </a></p> <p><a href="." style="color: green"> </a></p>
style (color), . : “color: red; border: none”.
. , display , .
<strong></strong>, <strong style="display: block"> </strong>, <strong style="display: none"> </strong>.
, – display: none . . , .
JavaScript style. , . – , - .
<p id="para" style="color: purple"> </p> <script> var para = document.getElementById("para"); console.log(para.style.color); para.style.color = "magenta"; </script>
, font-family. JavaScript ( style["font-family"]), , : style.fontFamily
HTML CSS (Cascading Style Sheets, ). – . :
<style> strong { font-style: italic; color: gray; } </style> <p> <strong> strong</strong> .</p>
«» , . , , , font-style .
, . font-weight: normal, , , . , style, .
CSS . .abc , “abc”. #xyz id “xyz” ( id ).
.subtle { color: gray; font-size: 80%; } #header { background: blue; color: white; } /* p, a b, id main */ pab#main { margin-bottom: 20px; }
, . , , . , pa , p .a, .
p > a {…}
, .
pa {…}
, , .
. , 2-3 . (, , ) – - DOM.
querySelectorAll, document, -, , , .
<p> <span class="animal"></span></p> <p> </p> <p> <span class="character"> <span class="animal"> </span></span></p> <p> .</p> <script> function count(selector) { return document.querySelectorAll(selector).length; } console.log(count("p")); // <p> // → 4 console.log(count(".animal")); // animal // → 2 console.log(count("p .animal")); // animal <p> // → 2 console.log(count("p > .animal")); // <p> // → 1 </script>
getElementsByTagName, querySelectorAll . , .
querySelector ( All) . , . , null, .
position . static, , . relative, , top left . absolute, «» – , . , left top , position static. , .
. , .
<p style="text-align: center"> <img src="img/cat.png" style="position: relative"> </p> <script> var cat = document.querySelector("img"); var angle = 0, lastTime = null; function animate(time) { if (lastTime != null) angle += (time - lastTime) * 0.001; lastTime = time; cat.style.top = (Math.sin(angle) * 20) + "px"; cat.style.left = (Math.cos(angle) * 200) + "px"; requestAnimationFrame(animate); } requestAnimationFrame(animate); </script>
position: relative. top left , .
requestAnimationFrame animate , . animate requestAnimationFrame, . ( ) , 60 , .
DOM , . JavaScript, . requestAnimationFrame – , , , .
, ( lastTime), , . , , , .
Math.cos Math.sin. , , .
Math.cos Math.sin , (0, 0) . , 0 , , 2π ( 6.28) . Math.cos x , , Math.sin y. ( ) , 2π 0, – , a+2π , a.
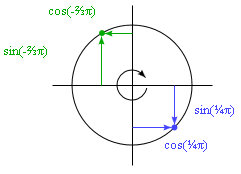
angle , animation. image. top Math.sin 20 – . left Math.cos 200, .
. px , , ( , ems ). . – 0, .
JavaScript DOM. , JavaScript . DOM , . parentNode childNodes, .
, , - . , , color display. JavaScript style.
6. HTML . HTML :
<table> <tr> <th>name</th> <th>height</th> <th>country</th> </tr> <tr> <td>Kilimanjaro</td> <td>5895</td> <td>Tanzania</td> </tr> </table>
. : , .
, 6, MOUNTAINS.
buildTable, , , DOM, . , , , . Object.keys, , .
, , style.textAlign "right".
<style> /* */ table { border-collapse: collapse; } td, th { border: 1px solid black; padding: 3px 8px; } th { text-align: left; } </style> <script> function buildTable(data) { // } document.body.appendChild(buildTable(MOUNTAINS)); </script>
getElementsByTagName . , ( ) , .
, tagName. , . toLowerCase toUpperCase.
<h1> <span>span</span> .</h1> <p> <span></span>, <span></span> spans.</p> <script> function byTagName(node, tagName) { // } console.log(byTagName(document.body, "h1").length); // → 1 console.log(byTagName(document.body, "span").length); // → 3 var para = document.querySelector("p"); console.log(byTagName(para, "span").length); // → 2 </script>
, 
.
. - .
, . top left . , position.
<img src="img/cat.png" id="cat" style="position: absolute"> <img src="img/hat.png" id="hat" style="position: absolute"> <script> var cat = document.querySelector("#cat"); var hat = document.querySelector("#hat"); // Your code here. </script>
.
, , . , , , . style ():
<p><a href="."> </a></p> <p><a href="." style="color: green"> </a></p>
style (color), . : “color: red; border: none”.
. , display , .
<strong></strong>, <strong style="display: block"> </strong>, <strong style="display: none"> </strong>.
, – display: none . . , .
JavaScript style. , . – , - .
<p id="para" style="color: purple"> </p> <script> var para = document.getElementById("para"); console.log(para.style.color); para.style.color = "magenta"; </script>
, font-family. JavaScript ( style["font-family"]), , : style.fontFamily
HTML CSS (Cascading Style Sheets, ). – . :
<style> strong { font-style: italic; color: gray; } </style> <p> <strong> strong</strong> .</p>
«» , . , , , font-style .
, . font-weight: normal, , , . , style, .
CSS . .abc , “abc”. #xyz id “xyz” ( id ).
.subtle { color: gray; font-size: 80%; } #header { background: blue; color: white; } /* p, a b, id main */ pab#main { margin-bottom: 20px; }
, . , , . , pa , p .a, .
p > a {…}
, .
pa {…}
, , .
. , 2-3 . (, , ) – - DOM.
querySelectorAll, document, -, , , .
<p> <span class="animal"></span></p> <p> </p> <p> <span class="character"> <span class="animal"> </span></span></p> <p> .</p> <script> function count(selector) { return document.querySelectorAll(selector).length; } console.log(count("p")); // <p> // → 4 console.log(count(".animal")); // animal // → 2 console.log(count("p .animal")); // animal <p> // → 2 console.log(count("p > .animal")); // <p> // → 1 </script>
getElementsByTagName, querySelectorAll . , .
querySelector ( All) . , . , null, .
position . static, , . relative, , top left . absolute, «» – , . , left top , position static. , .
. , .
<p style="text-align: center"> <img src="img/cat.png" style="position: relative"> </p> <script> var cat = document.querySelector("img"); var angle = 0, lastTime = null; function animate(time) { if (lastTime != null) angle += (time - lastTime) * 0.001; lastTime = time; cat.style.top = (Math.sin(angle) * 20) + "px"; cat.style.left = (Math.cos(angle) * 200) + "px"; requestAnimationFrame(animate); } requestAnimationFrame(animate); </script>
position: relative. top left , .
requestAnimationFrame animate , . animate requestAnimationFrame, . ( ) , 60 , .
DOM , . JavaScript, . requestAnimationFrame – , , , .
, ( lastTime), , . , , , .
Math.cos Math.sin. , , .
Math.cos Math.sin , (0, 0) . , 0 , , 2π ( 6.28) . Math.cos x , , Math.sin y. ( ) , 2π 0, – , a+2π , a.
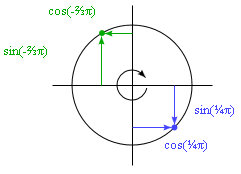
angle , animation. image. top Math.sin 20 – . left Math.cos 200, .
. px , , ( , ems ). . – 0, .
JavaScript DOM. , JavaScript . DOM , . parentNode childNodes, .
, , - . , , color display. JavaScript style.
6. HTML . HTML :
<table> <tr> <th>name</th> <th>height</th> <th>country</th> </tr> <tr> <td>Kilimanjaro</td> <td>5895</td> <td>Tanzania</td> </tr> </table>
. : , .
, 6, MOUNTAINS.
buildTable, , , DOM, . , , , . Object.keys, , .
, , style.textAlign "right".
<style> /* */ table { border-collapse: collapse; } td, th { border: 1px solid black; padding: 3px 8px; } th { text-align: left; } </style> <script> function buildTable(data) { // } document.body.appendChild(buildTable(MOUNTAINS)); </script>
getElementsByTagName . , ( ) , .
, tagName. , . toLowerCase toUpperCase.
<h1> <span>span</span> .</h1> <p> <span></span>, <span></span> spans.</p> <script> function byTagName(node, tagName) { // } console.log(byTagName(document.body, "h1").length); // → 1 console.log(byTagName(document.body, "span").length); // → 3 var para = document.querySelector("p"); console.log(byTagName(para, "span").length); // → 2 </script>
, 
.
. - .
, . top left . , position.
<img src="img/cat.png" id="cat" style="position: absolute"> <img src="img/hat.png" id="hat" style="position: absolute"> <script> var cat = document.querySelector("#cat"); var hat = document.querySelector("#hat"); // Your code here. </script>
.
, , . , , , . style ():
<p><a href="."> </a></p> <p><a href="." style="color: green"> </a></p>
style (color), . : “color: red; border: none”.
. , display , .
<strong></strong>, <strong style="display: block"> </strong>, <strong style="display: none"> </strong>.
, – display: none . . , .
JavaScript style. , . – , - .
<p id="para" style="color: purple"> </p> <script> var para = document.getElementById("para"); console.log(para.style.color); para.style.color = "magenta"; </script>
, font-family. JavaScript ( style["font-family"]), , : style.fontFamily
HTML CSS (Cascading Style Sheets, ). – . :
<style> strong { font-style: italic; color: gray; } </style> <p> <strong> strong</strong> .</p>
«» , . , , , font-style .
, . font-weight: normal, , , . , style, .
CSS . .abc , “abc”. #xyz id “xyz” ( id ).
.subtle { color: gray; font-size: 80%; } #header { background: blue; color: white; } /* p, a b, id main */ pab#main { margin-bottom: 20px; }
, . , , . , pa , p .a, .
p > a {…}
, .
pa {…}
, , .
. , 2-3 . (, , ) – - DOM.
querySelectorAll, document, -, , , .
<p> <span class="animal"></span></p> <p> </p> <p> <span class="character"> <span class="animal"> </span></span></p> <p> .</p> <script> function count(selector) { return document.querySelectorAll(selector).length; } console.log(count("p")); // <p> // → 4 console.log(count(".animal")); // animal // → 2 console.log(count("p .animal")); // animal <p> // → 2 console.log(count("p > .animal")); // <p> // → 1 </script>
getElementsByTagName, querySelectorAll . , .
querySelector ( All) . , . , null, .
position . static, , . relative, , top left . absolute, «» – , . , left top , position static. , .
. , .
<p style="text-align: center"> <img src="img/cat.png" style="position: relative"> </p> <script> var cat = document.querySelector("img"); var angle = 0, lastTime = null; function animate(time) { if (lastTime != null) angle += (time - lastTime) * 0.001; lastTime = time; cat.style.top = (Math.sin(angle) * 20) + "px"; cat.style.left = (Math.cos(angle) * 200) + "px"; requestAnimationFrame(animate); } requestAnimationFrame(animate); </script>
position: relative. top left , .
requestAnimationFrame animate , . animate requestAnimationFrame, . ( ) , 60 , .
DOM , . JavaScript, . requestAnimationFrame – , , , .
, ( lastTime), , . , , , .
Math.cos Math.sin. , , .
Math.cos Math.sin , (0, 0) . , 0 , , 2π ( 6.28) . Math.cos x , , Math.sin y. ( ) , 2π 0, – , a+2π , a.
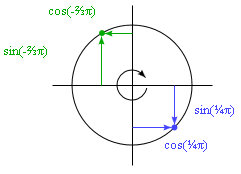
angle , animation. image. top Math.sin 20 – . left Math.cos 200, .
. px , , ( , ems ). . – 0, .
JavaScript DOM. , JavaScript . DOM , . parentNode childNodes, .
, , - . , , color display. JavaScript style.
6. HTML . HTML :
<table> <tr> <th>name</th> <th>height</th> <th>country</th> </tr> <tr> <td>Kilimanjaro</td> <td>5895</td> <td>Tanzania</td> </tr> </table>
. : , .
, 6, MOUNTAINS.
buildTable, , , DOM, . , , , . Object.keys, , .
, , style.textAlign "right".
<style> /* */ table { border-collapse: collapse; } td, th { border: 1px solid black; padding: 3px 8px; } th { text-align: left; } </style> <script> function buildTable(data) { // } document.body.appendChild(buildTable(MOUNTAINS)); </script>
getElementsByTagName . , ( ) , .
, tagName. , . toLowerCase toUpperCase.
<h1> <span>span</span> .</h1> <p> <span></span>, <span></span> spans.</p> <script> function byTagName(node, tagName) { // } console.log(byTagName(document.body, "h1").length); // → 1 console.log(byTagName(document.body, "span").length); // → 3 var para = document.querySelector("p"); console.log(byTagName(para, "span").length); // → 2 </script>
, 
.
. - .
, . top left . , position.
<img src="img/cat.png" id="cat" style="position: absolute"> <img src="img/hat.png" id="hat" style="position: absolute"> <script> var cat = document.querySelector("#cat"); var hat = document.querySelector("#hat"); // Your code here. </script>
.
, , . , , , . style ():
<p><a href="."> </a></p> <p><a href="." style="color: green"> </a></p>
style (color), . : “color: red; border: none”.
. , display , .
<strong></strong>, <strong style="display: block"> </strong>, <strong style="display: none"> </strong>.
, – display: none . . , .
JavaScript style. , . – , - .
<p id="para" style="color: purple"> </p> <script> var para = document.getElementById("para"); console.log(para.style.color); para.style.color = "magenta"; </script>
, font-family. JavaScript ( style["font-family"]), , : style.fontFamily
HTML CSS (Cascading Style Sheets, ). – . :
<style> strong { font-style: italic; color: gray; } </style> <p> <strong> strong</strong> .</p>
«» , . , , , font-style .
, . font-weight: normal, , , . , style, .
CSS . .abc , “abc”. #xyz id “xyz” ( id ).
.subtle { color: gray; font-size: 80%; } #header { background: blue; color: white; } /* p, a b, id main */ pab#main { margin-bottom: 20px; }
, . , , . , pa , p .a, .
p > a {…}
, .
pa {…}
, , .
. , 2-3 . (, , ) – - DOM.
querySelectorAll, document, -, , , .
<p> <span class="animal"></span></p> <p> </p> <p> <span class="character"> <span class="animal"> </span></span></p> <p> .</p> <script> function count(selector) { return document.querySelectorAll(selector).length; } console.log(count("p")); // <p> // → 4 console.log(count(".animal")); // animal // → 2 console.log(count("p .animal")); // animal <p> // → 2 console.log(count("p > .animal")); // <p> // → 1 </script>
getElementsByTagName, querySelectorAll . , .
querySelector ( All) . , . , null, .
position . static, , . relative, , top left . absolute, «» – , . , left top , position static. , .
. , .
<p style="text-align: center"> <img src="img/cat.png" style="position: relative"> </p> <script> var cat = document.querySelector("img"); var angle = 0, lastTime = null; function animate(time) { if (lastTime != null) angle += (time - lastTime) * 0.001; lastTime = time; cat.style.top = (Math.sin(angle) * 20) + "px"; cat.style.left = (Math.cos(angle) * 200) + "px"; requestAnimationFrame(animate); } requestAnimationFrame(animate); </script>
position: relative. top left , .
requestAnimationFrame animate , . animate requestAnimationFrame, . ( ) , 60 , .
DOM , . JavaScript, . requestAnimationFrame – , , , .
, ( lastTime), , . , , , .
Math.cos Math.sin. , , .
Math.cos Math.sin , (0, 0) . , 0 , , 2π ( 6.28) . Math.cos x , , Math.sin y. ( ) , 2π 0, – , a+2π , a.
angle , animation. image. top Math.sin 20 – . left Math.cos 200, .
. px , , ( , ems ). . – 0, .
JavaScript DOM. , JavaScript . DOM , . parentNode childNodes, .
, , - . , , color display. JavaScript style.
6. HTML . HTML :
<table> <tr> <th>name</th> <th>height</th> <th>country</th> </tr> <tr> <td>Kilimanjaro</td> <td>5895</td> <td>Tanzania</td> </tr> </table>
. : , .
, 6, MOUNTAINS.
buildTable, , , DOM, . , , , . Object.keys, , .
, , style.textAlign "right".
<style> /* */ table { border-collapse: collapse; } td, th { border: 1px solid black; padding: 3px 8px; } th { text-align: left; } </style> <script> function buildTable(data) { // } document.body.appendChild(buildTable(MOUNTAINS)); </script>
getElementsByTagName . , ( ) , .
, tagName. , . toLowerCase toUpperCase.
<h1> <span>span</span> .</h1> <p> <span></span>, <span></span> spans.</p> <script> function byTagName(node, tagName) { // } console.log(byTagName(document.body, "h1").length); // → 1 console.log(byTagName(document.body, "span").length); // → 3 var para = document.querySelector("p"); console.log(byTagName(para, "span").length); // → 2 </script>
, 
.
. - .
, . top left . , position.
<img src="img/cat.png" id="cat" style="position: absolute"> <img src="img/hat.png" id="hat" style="position: absolute"> <script> var cat = document.querySelector("#cat"); var hat = document.querySelector("#hat"); // Your code here. </script>
.
, , . , , , . style ():
<p><a href="."> </a></p> <p><a href="." style="color: green"> </a></p>
style (color), . : “color: red; border: none”.
. , display , .
<strong></strong>, <strong style="display: block"> </strong>, <strong style="display: none"> </strong>.
, – display: none . . , .
JavaScript style. , . – , - .
<p id="para" style="color: purple"> </p> <script> var para = document.getElementById("para"); console.log(para.style.color); para.style.color = "magenta"; </script>
, font-family. JavaScript ( style["font-family"]), , : style.fontFamily
HTML CSS (Cascading Style Sheets, ). – . :
<style> strong { font-style: italic; color: gray; } </style> <p> <strong> strong</strong> .</p>
«» , . , , , font-style .
, . font-weight: normal, , , . , style, .
CSS . .abc , “abc”. #xyz id “xyz” ( id ).
.subtle { color: gray; font-size: 80%; } #header { background: blue; color: white; } /* p, a b, id main */ pab#main { margin-bottom: 20px; }
, . , , . , pa , p .a, .
p > a {…}
, .
pa {…}
, , .
. , 2-3 . (, , ) – - DOM.
querySelectorAll, document, -, , , .
<p> <span class="animal"></span></p> <p> </p> <p> <span class="character"> <span class="animal"> </span></span></p> <p> .</p> <script> function count(selector) { return document.querySelectorAll(selector).length; } console.log(count("p")); // <p> // → 4 console.log(count(".animal")); // animal // → 2 console.log(count("p .animal")); // animal <p> // → 2 console.log(count("p > .animal")); // <p> // → 1 </script>
getElementsByTagName, querySelectorAll . , .
querySelector ( All) . , . , null, .
position . static, , . relative, , top left . absolute, «» – , . , left top , position static. , .
. , .
<p style="text-align: center"> <img src="img/cat.png" style="position: relative"> </p> <script> var cat = document.querySelector("img"); var angle = 0, lastTime = null; function animate(time) { if (lastTime != null) angle += (time - lastTime) * 0.001; lastTime = time; cat.style.top = (Math.sin(angle) * 20) + "px"; cat.style.left = (Math.cos(angle) * 200) + "px"; requestAnimationFrame(animate); } requestAnimationFrame(animate); </script>
position: relative. top left , .
requestAnimationFrame animate , . animate requestAnimationFrame, . ( ) , 60 , .
DOM , . JavaScript, . requestAnimationFrame – , , , .
, ( lastTime), , . , , , .
Math.cos Math.sin. , , .
Math.cos Math.sin , (0, 0) . , 0 , , 2π ( 6.28) . Math.cos x , , Math.sin y. ( ) , 2π 0, – , a+2π , a.
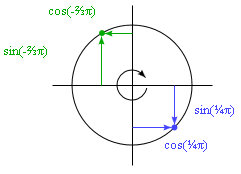
angle , animation. image. top Math.sin 20 – . left Math.cos 200, .
. px , , ( , ems ). . – 0, .
JavaScript DOM. , JavaScript . DOM , . parentNode childNodes, .
, , - . , , color display. JavaScript style.
6. HTML . HTML :
<table> <tr> <th>name</th> <th>height</th> <th>country</th> </tr> <tr> <td>Kilimanjaro</td> <td>5895</td> <td>Tanzania</td> </tr> </table>
. : , .
, 6, MOUNTAINS.
buildTable, , , DOM, . , , , . Object.keys, , .
, , style.textAlign "right".
<style> /* */ table { border-collapse: collapse; } td, th { border: 1px solid black; padding: 3px 8px; } th { text-align: left; } </style> <script> function buildTable(data) { // } document.body.appendChild(buildTable(MOUNTAINS)); </script>
getElementsByTagName . , ( ) , .
, tagName. , . toLowerCase toUpperCase.
<h1> <span>span</span> .</h1> <p> <span></span>, <span></span> spans.</p> <script> function byTagName(node, tagName) { // } console.log(byTagName(document.body, "h1").length); // → 1 console.log(byTagName(document.body, "span").length); // → 3 var para = document.querySelector("p"); console.log(byTagName(para, "span").length); // → 2 </script>
, 
.
. - .
, . top left . , position.
<img src="img/cat.png" id="cat" style="position: absolute"> <img src="img/hat.png" id="hat" style="position: absolute"> <script> var cat = document.querySelector("#cat"); var hat = document.querySelector("#hat"); // Your code here. </script>
.
, , . , , , . style ():
<p><a href="."> </a></p> <p><a href="." style="color: green"> </a></p>
style (color), . : “color: red; border: none”.
. , display , .
<strong></strong>, <strong style="display: block"> </strong>, <strong style="display: none"> </strong>.
, – display: none . . , .
JavaScript style. , . – , - .
<p id="para" style="color: purple"> </p> <script> var para = document.getElementById("para"); console.log(para.style.color); para.style.color = "magenta"; </script>
, font-family. JavaScript ( style["font-family"]), , : style.fontFamily
HTML CSS (Cascading Style Sheets, ). – . :
<style> strong { font-style: italic; color: gray; } </style> <p> <strong> strong</strong> .</p>
«» , . , , , font-style .
, . font-weight: normal, , , . , style, .
CSS . .abc , “abc”. #xyz id “xyz” ( id ).
.subtle { color: gray; font-size: 80%; } #header { background: blue; color: white; } /* p, a b, id main */ pab#main { margin-bottom: 20px; }
, . , , . , pa , p .a, .
p > a {…}
, .
pa {…}
, , .
. , 2-3 . (, , ) – - DOM.
querySelectorAll, document, -, , , .
<p> <span class="animal"></span></p> <p> </p> <p> <span class="character"> <span class="animal"> </span></span></p> <p> .</p> <script> function count(selector) { return document.querySelectorAll(selector).length; } console.log(count("p")); // <p> // → 4 console.log(count(".animal")); // animal // → 2 console.log(count("p .animal")); // animal <p> // → 2 console.log(count("p > .animal")); // <p> // → 1 </script>
getElementsByTagName, querySelectorAll . , .
querySelector ( All) . , . , null, .
position . static, , . relative, , top left . absolute, «» – , . , left top , position static. , .
. , .
<p style="text-align: center"> <img src="img/cat.png" style="position: relative"> </p> <script> var cat = document.querySelector("img"); var angle = 0, lastTime = null; function animate(time) { if (lastTime != null) angle += (time - lastTime) * 0.001; lastTime = time; cat.style.top = (Math.sin(angle) * 20) + "px"; cat.style.left = (Math.cos(angle) * 200) + "px"; requestAnimationFrame(animate); } requestAnimationFrame(animate); </script>
position: relative. top left , .
requestAnimationFrame animate , . animate requestAnimationFrame, . ( ) , 60 , .
DOM , . JavaScript, . requestAnimationFrame – , , , .
, ( lastTime), , . , , , .
Math.cos Math.sin. , , .
Math.cos Math.sin , (0, 0) . , 0 , , 2π ( 6.28) . Math.cos x , , Math.sin y. ( ) , 2π 0, – , a+2π , a.
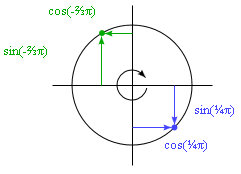
angle , animation. image. top Math.sin 20 – . left Math.cos 200, .
. px , , ( , ems ). . – 0, .
JavaScript DOM. , JavaScript . DOM , . parentNode childNodes, .
, , - . , , color display. JavaScript style.
6. HTML . HTML :
<table> <tr> <th>name</th> <th>height</th> <th>country</th> </tr> <tr> <td>Kilimanjaro</td> <td>5895</td> <td>Tanzania</td> </tr> </table>
. : , .
, 6, MOUNTAINS.
buildTable, , , DOM, . , , , . Object.keys, , .
, , style.textAlign "right".
<style> /* */ table { border-collapse: collapse; } td, th { border: 1px solid black; padding: 3px 8px; } th { text-align: left; } </style> <script> function buildTable(data) { // } document.body.appendChild(buildTable(MOUNTAINS)); </script>
getElementsByTagName . , ( ) , .
, tagName. , . toLowerCase toUpperCase.
<h1> <span>span</span> .</h1> <p> <span></span>, <span></span> spans.</p> <script> function byTagName(node, tagName) { // } console.log(byTagName(document.body, "h1").length); // → 1 console.log(byTagName(document.body, "span").length); // → 3 var para = document.querySelector("p"); console.log(byTagName(para, "span").length); // → 2 </script>
, 
.
. - .
, . top left . , position.
<img src="img/cat.png" id="cat" style="position: absolute"> <img src="img/hat.png" id="hat" style="position: absolute"> <script> var cat = document.querySelector("#cat"); var hat = document.querySelector("#hat"); // Your code here. </script>
.
, , . , , , . style ():
<p><a href="."> </a></p> <p><a href="." style="color: green"> </a></p>
style (color), . : “color: red; border: none”.
. , display , .
<strong></strong>, <strong style="display: block"> </strong>, <strong style="display: none"> </strong>.
, – display: none . . , .
JavaScript style. , . – , - .
<p id="para" style="color: purple"> </p> <script> var para = document.getElementById("para"); console.log(para.style.color); para.style.color = "magenta"; </script>
, font-family. JavaScript ( style["font-family"]), , : style.fontFamily
HTML CSS (Cascading Style Sheets, ). – . :
<style> strong { font-style: italic; color: gray; } </style> <p> <strong> strong</strong> .</p>
«» , . , , , font-style .
, . font-weight: normal, , , . , style, .
CSS . .abc , “abc”. #xyz id “xyz” ( id ).
.subtle { color: gray; font-size: 80%; } #header { background: blue; color: white; } /* p, a b, id main */ pab#main { margin-bottom: 20px; }
, . , , . , pa , p .a, .
p > a {…}
, .
pa {…}
, , .
. , 2-3 . (, , ) – - DOM.
querySelectorAll, document, -, , , .
<p> <span class="animal"></span></p> <p> </p> <p> <span class="character"> <span class="animal"> </span></span></p> <p> .</p> <script> function count(selector) { return document.querySelectorAll(selector).length; } console.log(count("p")); // <p> // → 4 console.log(count(".animal")); // animal // → 2 console.log(count("p .animal")); // animal <p> // → 2 console.log(count("p > .animal")); // <p> // → 1 </script>
getElementsByTagName, querySelectorAll . , .
querySelector ( All) . , . , null, .
position . static, , . relative, , top left . absolute, «» – , . , left top , position static. , .
. , .
<p style="text-align: center"> <img src="img/cat.png" style="position: relative"> </p> <script> var cat = document.querySelector("img"); var angle = 0, lastTime = null; function animate(time) { if (lastTime != null) angle += (time - lastTime) * 0.001; lastTime = time; cat.style.top = (Math.sin(angle) * 20) + "px"; cat.style.left = (Math.cos(angle) * 200) + "px"; requestAnimationFrame(animate); } requestAnimationFrame(animate); </script>
position: relative. top left , .
requestAnimationFrame animate , . animate requestAnimationFrame, . ( ) , 60 , .
DOM , . JavaScript, . requestAnimationFrame – , , , .
, ( lastTime), , . , , , .
Math.cos Math.sin. , , .
Math.cos Math.sin , (0, 0) . , 0 , , 2π ( 6.28) . Math.cos x , , Math.sin y. ( ) , 2π 0, – , a+2π , a.
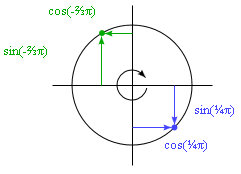
angle , animation. image. top Math.sin 20 – . left Math.cos 200, .
. px , , ( , ems ). . – 0, .
JavaScript DOM. , JavaScript . DOM , . parentNode childNodes, .
, , - . , , color display. JavaScript style.
6. HTML . HTML :
<table> <tr> <th>name</th> <th>height</th> <th>country</th> </tr> <tr> <td>Kilimanjaro</td> <td>5895</td> <td>Tanzania</td> </tr> </table>
. : , .
, 6, MOUNTAINS.
buildTable, , , DOM, . , , , . Object.keys, , .
, , style.textAlign "right".
<style> /* */ table { border-collapse: collapse; } td, th { border: 1px solid black; padding: 3px 8px; } th { text-align: left; } </style> <script> function buildTable(data) { // } document.body.appendChild(buildTable(MOUNTAINS)); </script>
getElementsByTagName . , ( ) , .
, tagName. , . toLowerCase toUpperCase.
<h1> <span>span</span> .</h1> <p> <span></span>, <span></span> spans.</p> <script> function byTagName(node, tagName) { // } console.log(byTagName(document.body, "h1").length); // → 1 console.log(byTagName(document.body, "span").length); // → 3 var para = document.querySelector("p"); console.log(byTagName(para, "span").length); // → 2 </script>
, 
.
. - .
, . top left . , position.
<img src="img/cat.png" id="cat" style="position: absolute"> <img src="img/hat.png" id="hat" style="position: absolute"> <script> var cat = document.querySelector("#cat"); var hat = document.querySelector("#hat"); // Your code here. </script>
.
, , . , , , . style ():
<p><a href="."> </a></p> <p><a href="." style="color: green"> </a></p>
style (color), . : “color: red; border: none”.
. , display , .
<strong></strong>, <strong style="display: block"> </strong>, <strong style="display: none"> </strong>.
, – display: none . . , .
JavaScript style. , . – , - .
<p id="para" style="color: purple"> </p> <script> var para = document.getElementById("para"); console.log(para.style.color); para.style.color = "magenta"; </script>
, font-family. JavaScript ( style["font-family"]), , : style.fontFamily
HTML CSS (Cascading Style Sheets, ). – . :
<style> strong { font-style: italic; color: gray; } </style> <p> <strong> strong</strong> .</p>
«» , . , , , font-style .
, . font-weight: normal, , , . , style, .
CSS . .abc , “abc”. #xyz id “xyz” ( id ).
.subtle { color: gray; font-size: 80%; } #header { background: blue; color: white; } /* p, a b, id main */ pab#main { margin-bottom: 20px; }
, . , , . , pa , p .a, .
p > a {…}
, .
pa {…}
, , .
. , 2-3 . (, , ) – - DOM.
querySelectorAll, document, -, , , .
<p> <span class="animal"></span></p> <p> </p> <p> <span class="character"> <span class="animal"> </span></span></p> <p> .</p> <script> function count(selector) { return document.querySelectorAll(selector).length; } console.log(count("p")); // <p> // → 4 console.log(count(".animal")); // animal // → 2 console.log(count("p .animal")); // animal <p> // → 2 console.log(count("p > .animal")); // <p> // → 1 </script>
getElementsByTagName, querySelectorAll . , .
querySelector ( All) . , . , null, .
position . static, , . relative, , top left . absolute, «» – , . , left top , position static. , .
. , .
<p style="text-align: center"> <img src="img/cat.png" style="position: relative"> </p> <script> var cat = document.querySelector("img"); var angle = 0, lastTime = null; function animate(time) { if (lastTime != null) angle += (time - lastTime) * 0.001; lastTime = time; cat.style.top = (Math.sin(angle) * 20) + "px"; cat.style.left = (Math.cos(angle) * 200) + "px"; requestAnimationFrame(animate); } requestAnimationFrame(animate); </script>
position: relative. top left , .
requestAnimationFrame animate , . animate requestAnimationFrame, . ( ) , 60 , .
DOM , . JavaScript, . requestAnimationFrame – , , , .
, ( lastTime), , . , , , .
Math.cos Math.sin. , , .
Math.cos Math.sin , (0, 0) . , 0 , , 2π ( 6.28) . Math.cos x , , Math.sin y. ( ) , 2π 0, – , a+2π , a.
angle , animation. image. top Math.sin 20 – . left Math.cos 200, .
. px , , ( , ems ). . – 0, .
JavaScript DOM. , JavaScript . DOM , . parentNode childNodes, .
, , - . , , color display. JavaScript style.
6. HTML . HTML :
<table> <tr> <th>name</th> <th>height</th> <th>country</th> </tr> <tr> <td>Kilimanjaro</td> <td>5895</td> <td>Tanzania</td> </tr> </table>
. : , .
, 6, MOUNTAINS.
buildTable, , , DOM, . , , , . Object.keys, , .
, , style.textAlign "right".
<style> /* */ table { border-collapse: collapse; } td, th { border: 1px solid black; padding: 3px 8px; } th { text-align: left; } </style> <script> function buildTable(data) { // } document.body.appendChild(buildTable(MOUNTAINS)); </script>
getElementsByTagName . , ( ) , .
, tagName. , . toLowerCase toUpperCase.
<h1> <span>span</span> .</h1> <p> <span></span>, <span></span> spans.</p> <script> function byTagName(node, tagName) { // } console.log(byTagName(document.body, "h1").length); // → 1 console.log(byTagName(document.body, "span").length); // → 3 var para = document.querySelector("p"); console.log(byTagName(para, "span").length); // → 2 </script>
, 
.
. - .
, . top left . , position.
<img src="img/cat.png" id="cat" style="position: absolute"> <img src="img/hat.png" id="hat" style="position: absolute"> <script> var cat = document.querySelector("#cat"); var hat = document.querySelector("#hat"); // Your code here. </script>
Source: https://habr.com/ru/post/243815/
All Articles