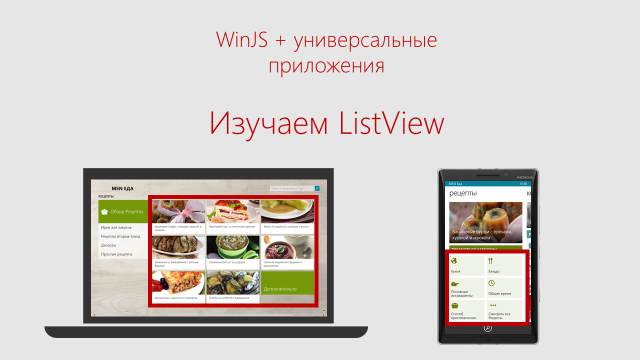
In the
previous article, I talked about how to create an image gallery using the FlipView control. Today we are going to look at the ListView control, which allows you not only to display various data in a list, but also to work with them - group, drag and reorder.
First, let's look at what data display options are available in the ListView control. If you are not familiar with the WinJS library, you can read about the controls included in the library
here .
')
Display and link data in a ListView control
To add a ListView, add a div block with the data-win-control attribute to the page, and assign it the value
WinJS.UI.ListView .
<div id="myListView" data-win-control="WinJS.UI.ListView"></div>
To work with ListView you need:
- Add data sources
- Set a template for displaying data
- Define layouts for displaying data
Add data source
To prepare the data to add to the ListView control, create an array:
(function () { "use strict"; var myData = [ { title: "Basic banana", text: "Low-fat frozen yogurt", picture: "images/60banana.png" }, { title: "Banana blast", text: "Ice cream", picture: "images/60banana.png" }, { title: "Brilliant banana", text: "Frozen custard", picture: "images/60banana.png" }, ... ]; })();
Each of the objects has three properties: title, text, and image. In this example, images are stored locally. Let's compile the Binding.List list from our array of objects:
var dataList = new WinJS.Binding.List(myData);
To make the list globally accessible (this is required for a declarative description), do not forget to create a namespace (in this case, DataExample) that provides an open itemList that returns a list. Using the
WinJS.Namespace.define function:
var publicMembers = { itemList: dataList }; WinJS.Namespace.define("DataExample", publicMembers);
The WinJS.Namespace.define function takes two parameters: the name of the namespace to be created and an object containing one or more property-value pairs. Each property is the public name of the element, and each value is the base variable, property, or function in your private code that you want to share.
In order to add the created list to the ListView control, you need to set the “itemDataSource” attribute to DataExample.itemList.dataSource.
Remember to create a template to display the data. You can read about how to create a template
here .
Note : To apply an item template, use the select syntax to set the
itemTemplate property
to your
ListView item template.
In addition to the template for each object separately, you need to define one of the available template layouts for displaying the data of the ListView control.
Standard Layouts for Displaying ListView Data
The
ListView element has three standard layouts for displaying data — a list, a grid, and a cell. To use one of the layouts, add the value
WinJS.UI.ListLayout ,
WinJS.UI.GridLayout or
WinJS.UI.CellSpanningLayout for the
layout property .
WinJS.UI.ListLayout WinJS.UI.GridLayout layouts are used to create a list and a grid of elements, respectively. As for the layout of
WinJS.UI.CellSpanningLayout - it is designed to create a grid with several different sizes.
<div id="myListView" data-win-control="WinJS.UI.ListView" data-win-options="{ itemDataSource : DataExample.itemList.dataSource, itemTemplate: select('#mediumListIconTextTemplate'), layout: {type: WinJS.UI.GridLayout}}"> </div>
Please note: The layout as a cell is available only for Windows.

Similarly for Windows:
Layout List (ListLayout)

Layout Grid (GridLayout)

Layout Cell (Layout CellSpanningLayout)

Styling the ListView control
The design of the ListView control is very easy to change. To stylize the ListView control, you can:
- Use WinJS.Binding.Template to describe a single item template
- Describe CSS styles for ListView elements, for example:
- win-listview sets the style of the entire ListView object
- win-viewport sets the style of the viewport. Here, if necessary, displays a scroll bar.
- win-surface sets the style of the scrollable area of ​​the ListView . If the viewport is smaller than the scrollable area, scroll bars appear in it.

A complete list of classes can be found on the
ListView reference page .
For example, you can add a background image and frame for the ListView element:
#myListView .win-listview { background-image: url('../images/icecream.png'); border: 2px solid red; }

Fine! We got acquainted with the ListView control, looked at creating and adding data, and also looked at the available layouts for display. Now let's learn how to work with items inside the ListView.
Changing the order of items in the ListView
Imagine that you have a list of data that you want to somehow reorder, swap the lines of the list. Let's solve this problem.
In the Listview control, there is a itemsReorderable property that allows you to swap items. The itemsReorderable property takes two values: true and false. In case we want to allow the permutation of elements, set the itemsReorderable property to true.
Note : The itemsReorderable property is not available for Windows Phone.
Declarative task of reordering elements
<div id="listView" class="win-selectionstylefilled" data-win-control="WinJS.UI.ListView" data-win-options="{ itemDataSource: myData.dataSource, itemTemplate: smallListIconTextTemplate, itemsReorderable: true, layout: { type: WinJS.UI.GridLayout } }"> </div>
Programming the possibility of reordering elements
(function () {

We decided to work with the list - now we can manually reorder the elements. And what to do if we want to somehow group them? For example, distribute them into groups, depending on the first letter of the element name.
Grouping Items in ListView
Consider an example of grouping data by the first letter of the name of each element. To implement grouping, create a version of your data source that will contain information about grouping elements. The grouping process will be as follows: we will first sort the group headers by comparing the unicode initial letters in the string, and then we will bring the headings of all the groups into one type and create the groups themselves.

We learned to distribute the elements into groups. And what to do if there are too many elements and groups and moving along the list becomes difficult? For this, the WinJS library provides a Semantic Zoom control.
Implement navigation in a ListView using the SemanticZoom control
The SemanticZoom control allows you to switch between two different views of the elements. One of these views is the content view itself. The second allows you to implement quick navigation between content, for example, display group headers for quick access to the contents of each group.
In order to add a SemanticZoom control, you need to create a div block and assign the WinJS.UI.SemanticZoom attribute to the data-win-control attribute.
<div data-win-control="WinJS.UI.SemanticZoom"></div>
Define three templates for ListView objects: one for a detailed view of the elements, another for group headers in a detailed view of elements, and the third for group headers in a general view. You also need to add 2 ListView controls. The first will define the detailed view, and the second will define the overall view.
We proceed directly to the addition of the SemanticZoom control. To do this, simply add a ListView control to the SemanticZoom control block for detailed and general presentation.
Pay attention to:
- Data Sources (itemDataSource)
- Templates for displaying data
<div id="semanticZoomDiv" data-win-control="WinJS.UI.SemanticZoom"> <div id="zoomedInListView" data-win-control="WinJS.UI.ListView" data-win-options="{ itemDataSource: myData.groupedItemsList.dataSource, itemTemplate: select('#mediumListIconTextTemplate'), groupHeaderTemplate: select('#headerTemplate'), groupDataSource: myData.groupedItemsList.groups.dataSource, selectionMode: 'none', tapBehavior: 'none', swipeBehavior: 'none' }" ></div> <div id="zoomedOutListView" data-win-control="WinJS.UI.ListView" data-win-options="{ itemDataSource: myData.groupedItemsList.<B>groups</B>.dataSource, itemTemplate: select('#semanticZoomTemplate'), selectionMode: 'none', tapBehavior: 'invoke', swipeBehavior: 'none' }" ></div> </div>
itemDataSource: myData.groupedItemsList.dataSource, itemTemplate: select ( '# mediumListIconTextTemplate'), groupHeaderTemplate: select ( '# headerTemplate'), groupDataSource: myData.groupedItemsList.groups.dataSource, selectionMode: 'none <div id="semanticZoomDiv" data-win-control="WinJS.UI.SemanticZoom"> <div id="zoomedInListView" data-win-control="WinJS.UI.ListView" data-win-options="{ itemDataSource: myData.groupedItemsList.dataSource, itemTemplate: select('#mediumListIconTextTemplate'), groupHeaderTemplate: select('#headerTemplate'), groupDataSource: myData.groupedItemsList.groups.dataSource, selectionMode: 'none', tapBehavior: 'none', swipeBehavior: 'none' }" ></div> <div id="zoomedOutListView" data-win-control="WinJS.UI.ListView" data-win-options="{ itemDataSource: myData.groupedItemsList.<B>groups</B>.dataSource, itemTemplate: select('#semanticZoomTemplate'), selectionMode: 'none', tapBehavior: 'invoke', swipeBehavior: 'none' }" ></div> </div>

Important! Remember to define your own styles for the overall presentation.

We got acquainted with what possibilities can be realized within one list in the ListView control. The next stage is working with several lists, namely, moving items from one list to another.
Drag and Drop support in the ListView control
Imagine a situation where you have two lists, and you want to move items from one list to another. To solve this problem, you can use the drag and drop operations available for the ListView control. These operations are compatible with the drag and drop function in HTML5. You can drag and drop between two ListView controls, between
ItemContainer and ListView, as well as between any HTML and ListView controls. You can allow the user to drag items to a specific place in the ListView, and you can also control where the draggable items are inserted. Read more about handling drag and drop events in HTML5
here .
Note : Drag and Drop items are not available for Windows Phone.
In order to allow dragging items from a ListView, we process it using special drag and drop events that have been added to HTML5. Conventionally, the entire processing of dragging an item can be divided into two steps:
Saving the data of the dragged item at the start of a drag operation using the
setData method.
Retrieving previously saved data after a dragged item is moved to its receiving item using the
getData method
.Consider the case in which the dragged item is added to any place in the list. Handle events to drag the item.
(function () { "use strict"; var page = WinJS.UI.Pages.define("/html/drag.html", { ready: function (element, options) { var dragging = false;
Thus, we have implemented the function of dragging an item from one list to any place in another list. In the screenshot, the portable item is highlighted in red.

Results
So, we got acquainted with the ListView control. It gives you the ability to work with list items. We reviewed various data display layouts, got acquainted with the method of implementing the reordering of the elements in the list, as well as with the grouping of elements by the first letter of the name of each element. Using the example of working with two lists, we implemented the ability to drag items from one list to another. As an example of how ListView works with other controls, the possibility of implementing navigation using the Semantic Zoom control was considered.
Additional links:
Quick Start: Adding a ListView Control (HTML)Quick Start Guide: Adding a Semantic Zoom Control (HTML)Controls (HTML with JavaScript)Creating a unique ListView layout using cell-spanning in HTMLMVA mobile development course for web developersDownload Microsoft Visual Studio here.