
As you understand, we are directly related to the development of applications for Android, because this OS is installed on YotaPhone. And with this post we open a series of publications in which we will cover various aspects of developing applications for Android, share the experience of both our own and other specialists. By the way, some time ago we already wrote about the SDK and features of the YotaPhone architecture, which also partly echoes the topic of this publication: the SDK fully developed by us is based on principles similar to the standard Android SDK to give developers intuitive tools.
If you are developing applications for Android, then you most likely need to store data somewhere. You can choose one of the cloud services (in this case it is convenient to use the
SyncAdapter ) or use the built-in SQLite database. In the second case, you will have to choose between writing SQL queries using the Content Provider (if you plan to use the data by several applications) or
ORM .
')
In this post we will look at some of the Android ORM, which you can use in your applications.
Ormlite
Perhaps this is the first
ORM that comes to mind. However, this is not Android ORM, but Java ORM with support for SQL databases. It can be used wherever Java is used, for example, in
JDBC connections ,
Spring, and Android.
Here annotations are actively used, for example,
@DatabaseTable
for each class that defines the table, and
@DatabaseField
- for each field in the class.
A simple example of using OrmLite to define a table:
@DatabaseTable(tableName = "users") public class User { @DatabaseField(id = true) private String username; @DatabaseField private String password; public User() {
This is an open source project, you can find it on
GitHub . For more information, refer to the official
documentation .
Sugarorm
This ORM was created specifically for Android. Included is an API that is easy to learn and remember. He can create the necessary tables himself and contains simple methods of forming links of the type “one-with-one” and “one-with-many”. SugarORM also makes it easier to create, read, update and delete (CRUD) with just three functions:
save()
,
delete()
and
find()
(or
findById()
).
To use SugarORM in your application, you need to add four
meta-data
in
AndroidManifest.xml
:
<meta-data android:name="DATABASE" android:value="my_database.db" /> <meta-data android:name="VERSION" android:value="1" /> <meta-data android:name="QUERY_LOG" android:value="true" /> <meta-data android:name="DOMAIN_PACKAGE_NAME" android:value="com.my-domain" />
Now you can use this ORM in the classes that you want to turn into tables:
public class User extends SugarRecord<User> { String username; String password; int age; @Ignore String bio;
Add new user:
User johndoe = new User(getContext(),"john.doe","secret",19); johndoe.save();
Delete all users aged 19:
List<User> nineteens = User.find(User.class,"age = ?",new int[]{19}); foreach(user in nineteens) { user.delete(); }
You can learn more about the capabilities of SugarORM in its
documentation .
GreenDAO
If you need high performance, then be sure to look at
GreenDAO . As stated on their website, “most entities can be added, updated, or downloaded with a capacity of
several thousand operations per second .” And if the authors were cunning about the capabilities of their offspring, then it would hardly be used in these
well-known applications . Compared with the same OrmLite, GreenDAO is almost 4.5 times faster:
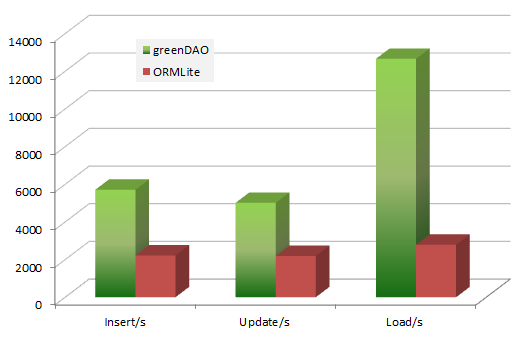
In size it is at least 100 Kb, which does not matter much.
See examples of using GreenDAO in a
tutorial using Android Studio. Also, anyone can get acquainted with the project code on
GitHub and study the
documentation .
ActiveAndroid
Like many other ORMs,
ActiveAndroid helps to store and retrieve records from SQLite without creating SQL queries.
To use ActiveAndroid, you need to add the jar file to the
/libs
folder. As stated in the
initial instructions , you need to copy the source code from
GitHub and compile it using
Maven . After connecting ActiveAndroid to your project, add
meta-data
tags to
AndroidManifest.xml
:
<meta-data android:name="AA_DB_NAME" android:value="my_database.db" /> <meta-data android:name="AA_DB_VERSION" android:value="1" />
After that, you can call
ActiveAndroid.initialize()
if necessary:
public class MyActivity extends Activity { @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); ActiveAndroid.initialize(this);
Now you can, using annotations, create models as Java classes:
@Table(name = "User") public class User extends Model { @Column(name = "username") public String username; @Column(name = "password") public String password; public User() { super(); } public User(String username,String password) { super(); this.username = username; this.password = password; } }
This is a simple example of using ActiveAndroid; for more complex uses, read the project
documentation .
Realm
The latest ORM in our review,
Realm is written in C ++ and runs directly on your device (without interpretation), which provides very high performance. The version code for iOS, if anyone is interested, can be found on
GitHub . Also at the Offsite, you can find examples of using Realm in Objective-C and Swift.
Conclusion
Of course, this is not the only ORM that exists in nature. Beyond this review, for example,
Androrm and
ORMDroid remained . Of course, every developer should be able to work with SQL, but creating queries is boring and lazy, so why not use one of the many ready-made ORMs to automate the process? They can significantly simplify your work.