Who, if not you? When, if not now?
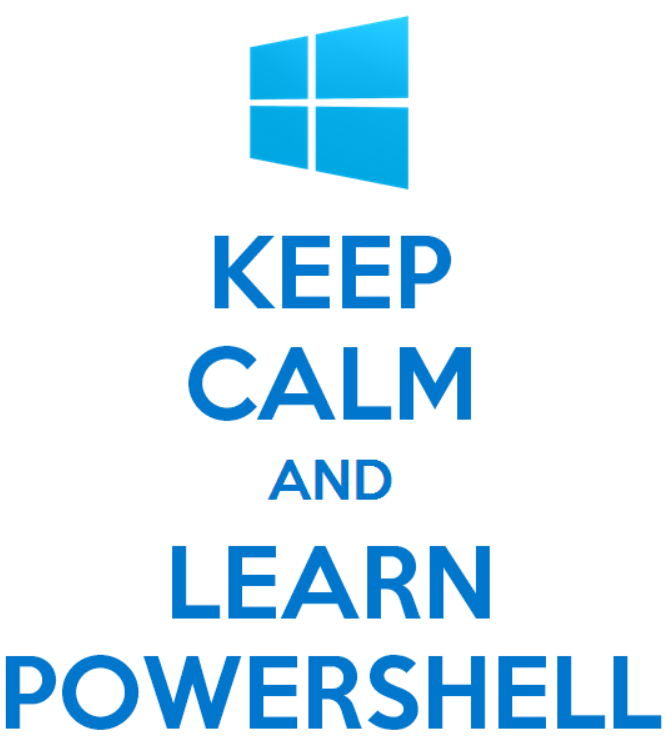
In the previous part, we learned how to run PowerShell, and we figured out the script execution policy. Understood what are cmdlets, we know how to pass them through the pipeline and how to get their properties. We learned that PowerShell has tremendous Help.
')
In this part, we will deal with variables, find out what types they are and how to refer to them, how to compare and display them in various ways. Be sure to understand the cycles and write a few functions.
â Go to Part IIntroduction
I want to start with the answers to the questions that have arisen in our first Jump Start:
What are the great features of PowerShell? Why do I need to study it?Everyone can use the phrase âtremendous opportunitiesâ for something different.
If I had to describe PowerShell in three words, I would say: this is a simplified administration environment that helps create tools for automation and control .
Itâs definitely worth exploring PowerShell if you administer Windows Server and Microsoftâs server software. Take, for example, one of the most popular products of Microsoft Exchange Server - and then you come across PowerShell Exchange Management Shell console.
Knowing PoSh, you can easily do any necessary data upload, set up control over any system processes, send reports, create users, solve their problems ...
I do not administer, what then?This can be compared with the way people of completely different professions are fond of creating websites. It seems they donât really need it, but they do it. For your own development, entertainment or pleasure.
Are you a student? Write yourself your first application that will solve the quadratic equation.
Or, for example, study parsing and make your application for downloading music or beautiful videos from youtube.
It all depends on you and your imagination. Look
here , PowerShell can even be taught to talk.
You can just watch the video on MVA!Yes, I totally agree with you - it can be more effective.
True, not everyone has time to view the 12-15 hour course and not everyone can perceive the information in this way.
Here I try to give basic knowledge with which you can successfully continue to develop my skills infinitely further.
Variables
- A variable in PowerShell starts with a $ sign
(in the European Union with a ⏠sign) and can contain any letters, numbers, and underscore in the name. - To assign a value to a variable, just assign it to it with an " = " sign. To display the value of a variable, you can simply write this variable. We will analyze the information in the text below.
$var = 619 $var

- Arithmetic operations can be performed on numbers, lines can be added. If you add a number to the line, the number is automatically converted to a string.
$a = 1 $b = 2 $c = $a + $b $c #c = 3 $str = $str = $str + $str #str = $str = $str + 2014 $str #str = 2014
- If we need to find out what type a variable has, you can use the GetType () method
$s = " ?" $s.GetType().FullName

- The type of PowerShell variable is determined automatically or can be manually assigned.
$var = "one" [string]$var = "one"
PowerShell uses the Microsoft .NET Framework data types. Consider the main ones:
Type / .NET class | Description |
---|
[string]
System.String | Line
$var = "one"
|
[char]
System.Char | Symbol
$var = [char]0x263b
|
[bool]
System.Boolean | Boolean May be $ true or $ false .
$bvar = $true
|
[int]
System.Int32 | 32-bit integer
$i = 123456789
|
[long]
System.Int64 | 64-bit integer
$long_var = 12345678910
|
[decimal]
System.Decimal | 128 bit decimal number. The letter d at the end of the number is required
$dec_var = 12345.6789d
|
[double]
System.Double | 8-byte floating point decimal
[double]$double_var = 12345.6789
|
[single]
System.Single | 32 bit floating point number
[single]$var = 123456789.101112
|
[DateTime]
System.DateTime | Variable date and time.
$dt_var = Get-Date
|
[array]
System.Object [] | Array The index of the elements of the array begins with 0 â to refer to the first element of the $ mas array, write $ mas [0] .
$mas = "one", "two", "three";
To add an element to an array, you can write
$mas = $mas + "four"
Each array element can have its own type.
$mas[4] = 1
|
[hashtable]
System.Collections.Hashtable | Hash tables. The difference between hash tables and arrays is that indexes are used in arrays, and named keys are used in a hash table. Hash tables are built on the principle: @ {key = "value"}
$ht = @{odin="one"; dva="two"; tri="three"}
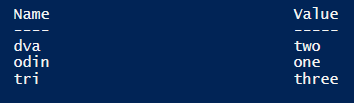
To add an element to the hash table, you can either assign it a key that does not yet exist, or use the Add () method. If assignment is done to an existing key, the key value will change to the assigned one. To remove an item from the hash table, there is a Remove () method.
$ht.Add("chetyre", "four") $ht.pyat = "five" $ht.Remove("dva")

|
You probably already understood that not only a certain value can be written to a variable, but you also noticed the System.Object [] class in the table. You can also write the output of any cmdlet to a variable.
Let's sort out the combat task : you need to know the
IP-address and
MAC-address of several computers on the network. Names of computers domain-comp1, domain-comp2. Solution option under the spoiler:
Show code $MAC = Get-WmiObject -ComputerName domain-comp1, domain-comp2 -Class Win32_NetworkAdapterConfiguration -Filter IPEnabled=True | Select-Object -Property * | SELECT PSComputerName, @{Name="IPAddress";Expression={$_.IPAddress.get(0)}}, MACAddress, Description $MAC
What's happening:
We write to the
$ MAC variable the result of the execution of the
Get-WmiObject cmdlet, with which we send the WMI request to the computers specified in the -computername parameter and return the necessary information from the
Win32_NetworkAdapterConfiguration class.
Please note that if you need to get the IP address and MAC address of the current computer, then instead of the computer names, we will pass the period to the -computername parameter. And, let's, in the following example, save the result in the file D: \ comp_mac_ip.csv:
$MAC = Get-WmiObject -ComputerName . -Class Win32_NetworkAdapterConfiguration -Filter IPEnabled=True | Select-Object -Property * | SELECT PSComputerName, @{Name="IPAddress";Expression={$_.IPAddress.get(0)}}, MACAddress, Description $MAC | Export-Csv D:comp_ip_mac.csv -Encoding UTF8
Variable scope
The scope of a variable in PowerShell can be either
local or
global . By default, the variable has a local scope and is limited to the scope, for example, it is available only in a function or only in the current script. The global variable is valid throughout the current PowerShell session. In order to designate a global variable, it suffices to write the following construction:
$ Global: variable = value $Global:var = 12
And another trick on the topic of variables ... Somehow, discussing with a colleague 1C and his Russian-language programming environment, they asked:
- It is interesting, but if you set a function or variable in Russian in PowerShell, will it work?- In theory, it should work, these are also symbols.
And yes, indeed:
function () { ", $env:USERNAME. ?" Start-Sleep 3 " ?" } function () { & "C:\Program Files\Internet Explorer\iexplore.exe" http:

Comparison operators and logical operators
Comparison operators and logical operators verify equality or consistency between two values.
- When the condition is met, the comparison construct always returns the logical value $ true or $ false if the condition is false.
The table below shows the comparison operators:
Operator | Description | Example |
---|
-eq | Equal / Equals (=) | $var = "619" $var -eq 123 #$false
|
-ne | Not equal / (<>) | $var = "619" $var -ne 123 |
-gt | Greater than / More (>) | $var = "619" $var -gt 123 |
-ge | Greater than or equal / Greater than or equal (> =) | $var = "619" $var -ge 123 #$true |
-lt | Less than / <(<) | $var = "619" $var -lt 123 |
-le | Less than or equal / Less than or equal (<=) | $var = "619" $var -le 123 #$false |
-like | Comparison based on wildcard characters | "Habra" -like "habr*" #true |
-notlike | Comparison for inconsistent wildcard characters | "Habra" -notlike "habr*" #false |
-contains | Does the value on the left contain the value on the right | 1, 2, 3, 4, 5 -contains 3 #$true |
-notcontains | If the value on the left does not contain the value on the right, we get true | 1, 2, 3, 4, 5 -notcontains 3 #$false |
-match | Using regular expressions to match pattern | $str = "http://habrahabr.ru" $str -match "^http://(\S+)+(.ru)$" #$true |
-notmatch | Using regular expressions to look for pattern inconsistencies | $str = "http://habrahabr.ru" $str -notmatch "^http://(\S+)+(.com)$" #true |
-replace | Replaces part or all of the value to the left of the operator | "Microhabr" -replace "Micro","Habra" #Habrahabr |
Let us examine an example. In the example, we form the path to the possible user profile on the remote computer, depending on the operating system. Solution under spoiler:
Show code # Function UProfile ($UCompName, $ULogin) { [string]$CompOS = Get-WmiObject -ComputerName $UCompName -Class Win32_OperatingSystem | SELECT Caption # if ($CompOS -match "Windows 7") { # "Windows 7" [string]$LinkUserProfile = "\\$UCompName\d$\users\$ULogin" # $LinkUserProfile } elseif ($CompOS -match "Windows XP") { # "Windows XP" [string]$LinkUserProfile = "\\$UCompName\d$\Documents and Settings\$ULogin" # $LinkUserProfile } } UProfile domain-comp1 Administrator # - -
Yes, here we do not check if the profile exists. But you can check. To do this, add another condition in which we will test the existence of a path with the
Test-Path cmdlet:
# Function UProfile ($UCompName, $ULogin) { [string]$CompOS = Get-WmiObject -ComputerName $UCompName -Class Win32_OperatingSystem | SELECT Caption # if ($CompOS -match "Windows 7") { # "Windows 7" [string]$LinkUserProfile = "\\$UCompName\d$\users\$ULogin" # } elseif ($CompOS -match "Windows XP") { # "Windows XP" [string]$LinkUserProfile = "\\$UCompName\d$\Documents and Settings\$ULogin" # } if (Test-Path $LinkUserProfile) { $LinkUserProfile } else { " $ULogin $UCompName" } # } UProfile domain-comp1 Administrator # - -
By the way, the
regular expression in PowerShell is very well described by Xaegr in his blog . It is worth it to spend a couple of hours reading and to grasp the ability to write regular expressions.Operator | Description | Example |
---|
-and | Logical and | ("" -eq "") -and (619 -eq 619) #$true |
-or | Logical or | ("" -eq "") -or (619 -eq 123) #$true |
-not | Logical not | -not (123 -gt 324) or
!(123 -gt 324) |
A couple of words about if ... elseif and else
if ( 1- ) { - } # 1 , , - elseif ( 2- ) { - } # : , 2 , else { - } # : ,
Everything is quite simple. If the condition is true, we execute the code following the condition in curly braces. If the condition is not true, then we skip the execution and see if the condition continues further.
If we find elseif, we check the new condition and also, if successful, execute the code, otherwise, we look again whether there is a continuation as elseif or else.
If the condition is met, the code is executed and the elseif or else branch is skipped.
$str = "" if ( 1 -gt 2 ) { $str = "" } elseif ( $str -eq "" ) { $str } else { $str = ""; $str }
Information output
Consider a few basic options for displaying information on the screen:
- Write to file using out-file
$str = "Hello Habr!" $str | Out-File D:\out_test1.txt
or, as we said in part I, use the " > " sign
$str = "Hello Habr!" $str > D:\out_test2.txt
- Export data to a .csv file using Export-Csv
$p = Get-Process $p | SELECT Name, Path | Export-Csv -Path D:\out_test3.csv
- Writing to an HTML file using ConvertTo-Html
$h = Get-Process $h | ConvertTo-Html -Property Name, Path > D:\out_test4.html & D:\out_test4.html
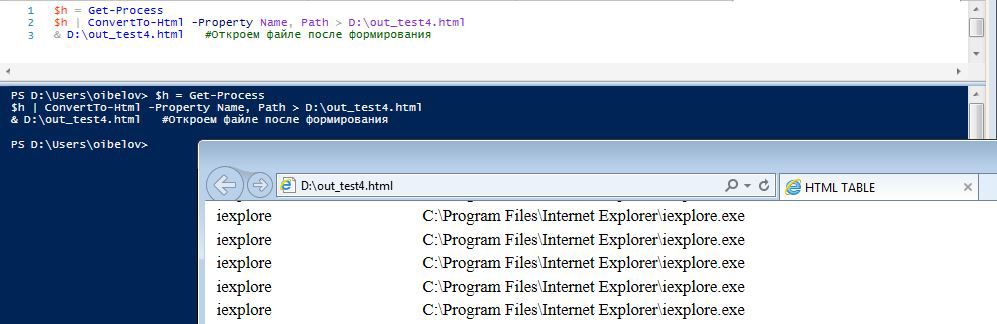
Working with HTML output is actually very nice. Having the skills of web-programming, you can add styles and get very nice reports on output.
Get-Content cmdlet familiar to us will help us to read the file.
Get-Content D:\out_test1.txt

Cycles
Loops in PowerShell are not much different from loops in other languages. Almost everything as elsewhere:
Do while
Do as long as the condition is true
$x = 10 do { $x $x = $x - 1 } while ($x -gt 0)
Do until
A loop that repeats a set of commands while the condition is met.
$x = 0 do { $x $x = $x + 1 } until ($x -gt 10)
For
A cycle that repeats the same steps a certain number of times.
for ($i = 0; $i -lt 10; $i++) { $i }
ForEach
Cycle through the collection of objects
$collection = "-1", "-2", "-3" foreach ($item in $collection) { $item }
We write functions
For example, the function for getting the square of a number on PowerShell would look like this:
function sqr ($a) { return $a * $a } sqr 2
And lastly, an example of a function that will help us perform transliteration. In this example, everything that we considered today is:
Show code # function Translit ([string]$inString) { $Translit = @{ # - [char]'' = "a" [char]'' = "A" [char]'' = "b" [char]'' = "B" [char]'' = "v" [char]'' = "V" [char]'' = "g" [char]'' = "G" [char]'' = "d" [char]'' = "D" [char]'' = "e" [char]'' = "E" [char]'' = "yo" [char]'' = "Yo" [char]'' = "zh" [char]'' = "Zh" [char]'' = "z" [char]'' = "Z" [char]'' = "i" [char]'' = "I" [char]'' = "j" [char]'' = "J" [char]'' = "k" [char]'' = "K" [char]'' = "l" [char]'' = "L" [char]'' = "m" [char]'' = "M" [char]'' = "n" [char]'' = "N" [char]'' = "o" [char]'' = "O" [char]'' = "p" [char]'' = "P" [char]'' = "r" [char]'' = "R" [char]'' = "s" [char]'' = "S" [char]'' = "t" [char]'' = "T" [char]'' = "u" [char]'' = "U" [char]'' = "f" [char]'' = "F" [char]'' = "h" [char]'' = "H" [char]'' = "c" [char]'' = "C" [char]'' = "ch" [char]'' = "Ch" [char]'' = "sh" [char]'' = "Sh" [char]'' = "sch" [char]'' = "Sch" [char]'' = "" [char]'' = "" [char]'' = "y" [char]'' = "Y" [char]'' = "" [char]'' = "" [char]'' = "e" [char]'' = "E" [char]'' = "yu" [char]'' = "Yu" [char]'' = "ya" [char]'' = "Ya" } $TranslitText = "" foreach ($CHR in $inCHR = $inString.ToCharArray()) { if ($Translit[$CHR] -cne $Null) { $TranslitText += $Translit[$CHR] } # $TranslitText = $TranslitText + $Translit[$CHR] else { $TranslitText += $CHR } } return $TranslitText } Translit #: Habrahabr
Afterword
Now we know enough information to start diving into PowerShell even deeper. In this part, we met with variable, data types, sorted out the information output, comparison operators, cycles ... Look, itâs not so difficult to start, it turns out!
Keep calm and learn PowerShell.To be continued...
Additional Information