Microsoft Azure mobile services provide developers with a turnkey solution for implementing a cloud backend in an application. Azure Mobile Services now fully supports the creation of server logic using the ASP.NET Web API and is a great choice for developers designing mobile APIs using ASP.NET:
- Ready backend with a set of SDK for all mobile platforms . Using Azure mobile services, you can quickly add a full-featured backend for your iOS, Android, Windows, Windows Phone, or HTML applications, as well as for cross-platform solutions based on Xamarin, Sencha and PhoneGap. We provide client-side SDKs for each of these platforms, making it easy to integrate applications with the backend in the cloud.
')
- First-class mobile API hosting .
Mobile services are built using the Web API and you can publish any existing Web API controller to a mobile service. The main difference of mobile services from other computing environments is that we independently monitor the work of Web API controllers . And in case of problems with the Web API runtime or extensions, we fix this without your participation. We only contact you if a problem is found in the controller code.
- Useful mobile backend features . Your Web API code is available a large number of opportunities provided by mobile services. Among them: mobile push notifications , real-time messaging with SignalR , authorization through social networks , implementation of offline application operation .
- Connect to corporate systems . Corporate systems developers will be able to appreciate the possibility of authorization in the application using Active Directory and access to corporate resources, such as SharePoint and Office 365. In addition, we added a mechanism to help implement a seamless connection to local resources that are not accessible from the Internet as databases and internal web services.
- Integration with Visual Studio . Your favorite environment now contains a special project template and scaffolding for mobile services, and provides support for publishing and remote debugging code.
Ready backend with a set of SDK for all mobile platforms
Getting started implementing mobile services in Visual Studio is easy. You need to go to the
Microsoft Azure portal and create a new mobile service. On the first page, select .NET as the server side. After the service is created, go to the “Quickstart” tab and download the test project for the client platform on which you are developing.


In the event that you start working in
Visual Studio , you can create a mobile service after developing a local project to publish it to the service.

In any case, you will receive a Mobile Services .NET project template. Please note that this is a regular Web API project that uses additional NuGet packages.

Open the controller file TodoItemController.cs and examine its contents. Set a breakpoint inside the GetAllTodoitems method. This controller will show you how to work with data using Mobile Services .NET support:
public class TodoItemController : TableController<TodoItem> { protected override void Initialize(HttpControllerContext controllerContext) { base.Initialize(controllerContext); csharp_testContext context = new csharp_testContext(); DomainManager = new EntityDomainManager<TodoItem>(context, Request, Services); }
Please note that all key TodoItem CRUD methods are already defined. By default, the EntityDomainManager controller wraps the Entity Framework model, which provides an easy transition to using alternative data storage:
With the support of .NET code in mobile services, running and debugging the logic of the mobile backend is available locally. We start the service with the F5 key, and on the service start page in the browser, click "Try it out".
.NET support in mobile services provides automatic generation of reference information for your Web API as a separate page. You can get a description of the method implementation using
GET tables / TodoItem . Click on “Try this out” and then “Send” to call the GetAllTodoItems () method, and you get to the breakpoint that you set earlier.
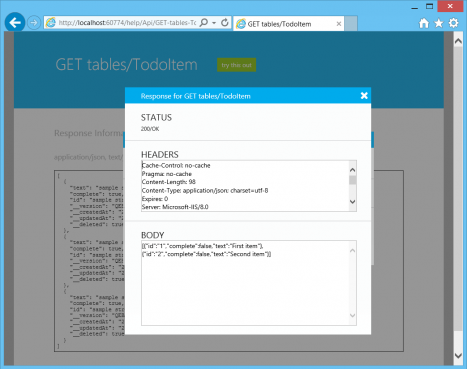
After completing the development of the server-side API, you can publish your Web API to a mobile service. Publishing support is built into Visual Studio, just right-click on the project and select “Publish”. You can choose an existing mobile service or create a new one directly from Visual Studio without having to visit the Azure portal.
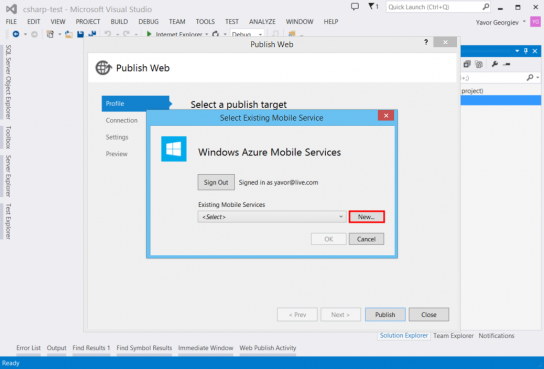
You can publish any existing WebAPI solution to a mobile service and immediately transfer its management and monitoring to Azure.
More information about the support of the .NET backend in mobile services can be found in the documentation:
First-class mobile API hosting
Let's talk a little about the benefits that the developer gets when publishing his API to the mobile service, because on the other hand there are many other ways to host the Web API in Azure.
We list only some of the advantages provided by mobile services:
- Monitoring and diagnostics. Mobile services provide a 99.9% SLA level based on the monitoring and monitoring capabilities created in Azure to take care of your API. Paid services include monitoring of HTTP traffic and SQL connection, plus we will notify you with a sharp increase in the number of errors. And these are just additions to a range of features that you can use yourself, including endpoint monitoring and alerts .
- Automatic environment update . We update the platform code weekly, without the need to redeploy your application. Changes in the mobile services runtime or new features — all of these updates will be added to your mobile services without interrupting their work.
- Automatic initialization of cloud resources . When creating any mobile service, we automatically provide and configure the SQL database and notification hub, and check the configuration of all relevant connections. Also, with a couple of mouse clicks, it is possible to quickly create scheduled tasks and hybrid connections in the cloud. Mobile services provide fast connection with all the necessary cloud resources necessary for comfortable work of your service.
Useful mobile backend features
Mobile services offer a large amount of functionality for your application right out of the box.
For example:
- Ready authentication. For each mobile service, a whole set of cloud resources and the implementation of the most common authentication scenarios are provided. Quick connect authorization with a Microsoft account, Facebook, Twitter, or Google, or with an organization account using AAD. You do not need to be an OAuth expert, because all the necessary mechanisms are ready and assembled, it is enough to clarify some configuration parameters, and the Azure services will take care of the rest.
Detailed information is available at the following link . - Scalable push notifications. The mobile service is always equipped with a push notification hub, which ensures delivery of a large number of notifications to devices on all major platforms: Windows, iOS, Android and Kindle. In order to send a notification to millions of connected devices, you need just one API call. Tagging support is also implemented to divide the audience and customize notification templates for each group.
Detailed step by step instructions are provided in the document . - Offline mode of the application . To date, one of the features expected by users of the application has been support for working with data in the absence of an Internet connection. Azure Mobile Services provides native support for offline application and data synchronization for all major platforms, based on SQLite.
Detailed information about this feature can be found here . - Real-time messaging . Push notifications work great to attract users to your application, but providing low-latency scalable high bandwidth for your application requires a different set of tools. Mobile services provide built-in support for real-time data exchange based on WebSocket and SignalR, providing two-way messaging in your application. The service is provided with a built-in messaging bus for seamless, scalable deployment as your application grows. It is integrated with the same authentication channel as the rest of your services, facilitating the authentication and authorization of users.
Additional information is available here .
Connect to corporate systems
The choice of the .NET platform is one of the frequent corporate development scenarios, since the .NET family of languages ​​provides a large number of relevant scenarios for creating
business applications :
- Registration and authorization with an Active Directory account . For corporate applications, it is very important to be able to authenticate with an AD account. The implementation of this mechanism simplifies account management, and also provides users with access to cloud resources protected by Active Directory, such as SharePoint Online and Office 365.
For more information on setting up Active Directory authorization in the application, we recommend that you study the following material . Video - instructions for setting up integrated access is here . - Connect to data inside the corporate network . In the case when corporate resources such as databases and web services are forced to be stored locally on the company's equipment and cannot be transferred to the cloud, Microsoft Azure provides the ability to access them via mobile services. Using the “Hybrid connections” function, which allows you to transfer local traffic to the cloud, you can ensure access to data within your corporate network in your application.
Links to a detailed guide and video instructions . - Xamarin SDK . Xamarin provides a ready-made set of tools for creating cross-platform applications (Windows, Windows Phone, Android and IOS) in C #, implementing one of the most productive application creation mechanisms, based on the reuse of skills for developers familiar with the Microsoft stack on other platforms . Microsoft Azure mobile services provide this SDK, as well as a huge amount of other resources needed to create Xamarin applications.
- Accelerators Sometimes the easiest way to implement a script is to use a ready-made solution. The Microsoft Azure team has created a set of "accelerators", which are ready-to-use applications that cover the main vertical scenarios of some of our clients and demonstrate the use of mobile services. These applications are published in the Windows store and are available as source codes for download and modification.
Detailed information is available at the following link .
Integration with Visual Studio
In addition to the already listed functionality,
Visual Studio contains many other useful tools for developing and debugging your mobile service. For example, built-in scaffolding, which allows you to create table controllers (for storing relational data), user controllers (for building arbitrary APIs, HTTP), and scheduled tasks.

Visual Studio also provides remote debugging. Simply right-click on the mobile service in the "Solution Explorer" and select "Attach Debugger." In order to use this feature, make sure that you publish the code in “Debug” mode.

Another interesting feature in Solution Explorer is the “View Logs” command, which allows you to view logs filled in by your mobile service in the cloud, including error messages and stack traces.

We hope that you will appreciate the range of new features and capabilities of
Azure Mobile Services, and encourage you to try them on your next project.
For recommendations or feedback, use Twitter:
@theYavor .
useful links