One of the innovations of iOS 8 are interactive notifications. Up to four actions can be assigned to such notifications, each of which will be represented by a separate button at the bottom of the banner with the notification text. Thus, the user has additional opportunities to respond to messages without activating (usually) the application. Consider how to add support for this functionality.
Action definition
First, it is necessary to determine the actions that can be performed on the notification. This is done using instances of the
UIMutableUserNotificationAction class:
UIMutableUserNotificationAction *action_like = [[UIMutableUserNotificationAction alloc] init]; action_like.identifier = @"Like"; action_like.title = NSLocalizedString(@"Like",nil); action_like.activationMode = UIUserNotificationActivationModeBackground; action_like.destructive = NO; action_like.authenticationRequired = NO;
Instances of this class contain properties:
')
identifier - the action
identifier string. This property will be used further to distinguish actions from each other.
title - the title that will be shown on the button.
activationMode - determines whether the action can be processed in the background or the application must be activated.
destructive - determines if the action is destructive. If so, the button for such an action will be highlighted (usually in red).
authenticationRequired - determines whether user
authentication is required to perform an action.
An example of the destructive action definition for which the application will be activated:
UIMutableUserNotificationAction *action_del = [[UIMutableUserNotificationAction alloc] init]; action_del.identifier = @"Delete"; action_del.title = NSLocalizedString(@"Delete",nil); action_del.activationMode = UIUserNotificationActivationModeForeground; action_del.destructive = YES; action_del.authenticationRequired = NO;
Creating a category and registering notification processing parameters
Secondly, we need to create a category for our notifications using an instance of the
UIMutableUserNotificationCategory class:
UIMutableUserNotificationCategory *category= [[UIMutableUserNotificationCategory alloc] init]; category.identifier = @"my_category"; [category setActions:@[action_like, action_del] forContext:UIUserNotificationActionContextDefault]; NSSet *categories = [NSSet setWithObjects:category, nil];
The category identifier should be sent as the
category key in the
pyaload 'push notifications or the
category notification properties of the local notification.
The forContext argument specifies the number of valid actions to be notified.
Check in:
UIUserNotificationSettings *notificationSettings = [UIUserNotificationSettings settingsForTypes:UIUserNotificationTypeAlert|UIUserNotificationTypeBadge|UIUserNotificationTypeSound categories:categories]; [[UIApplication sharedApplication] registerUserNotificationSettings:notificationSettings];
Submission Notification
Sending a notification of the following form:
{'aps': {'alert': 'A sample of interactive notification text', 'category': 'my_category', 'some_id': 'my_id' } }
(taking into account the restrictions on the size of the payload, it makes sense to set the notification category with a not very long identifier; but since this is a training example,
my_category is used), we will receive the following notifications.
On lock-screen:

and after the shift:
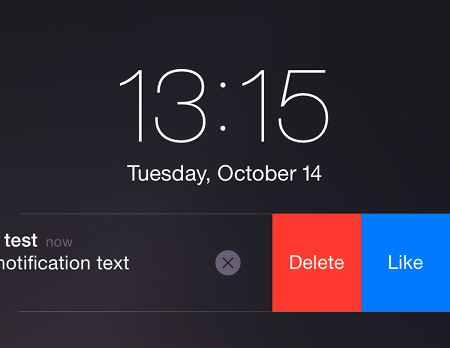
In the notification area:
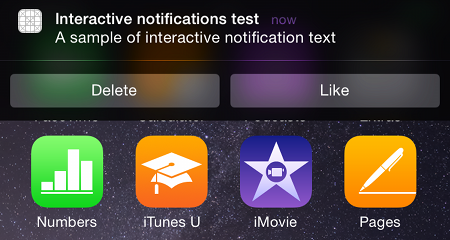
And the Notification Center:
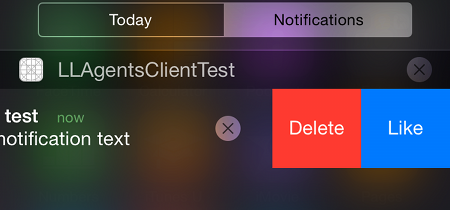
Button press processing
To handle button presses, define a method.
- application:handleActionWithIdentifier:forLocalNotification:completionHandler:
for handling local notifications.
AND
- application:handleActionWithIdentifier:forRemoteNotification:completionHandler:
for handling push notifications.
The second argument of these methods is the action identifier, which we previously indicated as:
action_del.identifier = @"Delete";
The last argument is a block that must
be called after the action is processed:
- (void) application:(UIApplication *)application handleActionWithIdentifier:(NSString *)identifier forRemoteNotification:(NSDictionary *)userInfo completionHandler:(void (^)())completionHandler { NSLog(@"NOTIFICATION IDENT: %@ USER INFO: %@", identifier, userInfo); completionHandler(); }
Please note that clicking on the '
Like ' button does not activate the application. Unlike clicking on '
Delete '.
Conclusion
Thus, by “flick of the wrist” (c) you can add additional functionality to the application, increasing its attractiveness to the user (in any case, try to increase the attractiveness).