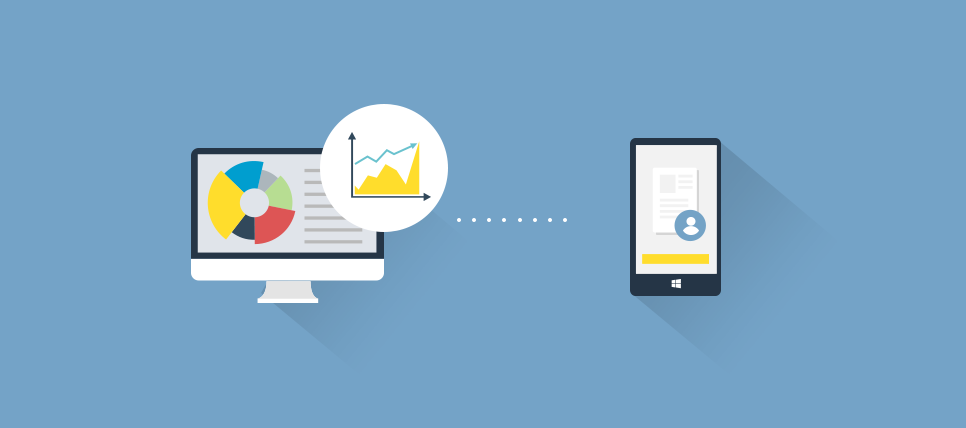
Development and promotion of relatively serious mobile applications is almost impossible without analyzing what the user does in your application, from what source he installed and analyzing various statistical parameters in the app store. Today, there are three main areas in mobile application analytics:
- Tracking installation sources (tools such as Mobile App Tracking , AppsFlyer and ADXtracking , are used, as a rule, to promote applications);
- Analytics within the application (the most well-known tools are Flurry , Google Analytics , Yandex.Metrica and Mixpanel , which provide an opportunity to monitor the behavior of users within the mobile application, which allows you to decide on the further development of your product);
- Collecting statistics from app stores (the most popular services are App Annie and Distimo , which allow you to track the position in the stack of both your app and competitors).
Since this article is intended for developers, it will be about how to embed analytics in a Windows Phone application. Flurry Analytics will be considered as an analytics system.
Service Description
Flurry is the most popular tool, primarily due to the fact that it is completely free, available for all major mobile platforms and has a fairly powerful functionality. Consider the main indicators that provides this tool:
- The number of new and active users;
- The number of sessions and their length;
- Frequency of use of the application;
- Failure statistics;
- Application audience (gender, age, language, geography of use);
- Information about product versions and devices;
- Events inside the application;
- Navigation on screens, etc.
All questions about what to do with this data, let's leave to analysts, we are also interested in what the developer needs to do in order for this data to become available.
Integrating the Flurry SDK into a Windows Phone application
After creating an account on the site
https://dev.flurry.com , you will have the opportunity to create a new application for any of the main platforms.
')

To do this, you need to specify the name of the application and the category to which it belongs.

A unique key will be generated for the application and the Flurry SDK download will become available. You can start developing!
The first step is to add a link to the FlurryWP8SDK.dll assembly to the project and make some changes to the application manifest. Namely, set ID_CAP_NETWORKING to transfer analytics data to the Flurry server and ID_CAP_IDENTITY_DEVICE to access information about the device.
To start collecting analytics, you will need to start a new session when the application starts:
private void Application_Launching(object sender, LaunchingEventArgs e) { FlurryWP8SDK.Api.StartSession("Your API Key"); }
As a parameter, the function accepts a unique key of your application. You should also start a new session after re-activating the application:
private void Application_Activated(object sender, ActivatedEventArgs e) { FlurryWP8SDK.Api.StartSession("Your API Key"); }
In this case, all analytics will be tracked in the old session. However, if the application is activated after more than 10 seconds, then a new session is created and the analytics will be tracked within the framework of the new session. To change the period during which the old session can be resumed, you can use the SetSessionContinueSeconds function, passing it as a parameter the required number of seconds:
private void Application_Deactivated(object sender, DeactivatedEventArgs e) { FlurryWP8SDK.Api.SetSessionContinueSeconds(60); }

There is also a function to force the session to terminate, but it is not necessary to call it, since by default it is already associated with closing the application. But if in some case you need to end the current session, you can do this just as easily:
private void Application_Closing(object sender, ClosingEventArgs e) { FlurryWP8SDK.Api.EndSession(); }

Registration of application crashes
When handling exceptions, as a rule, we expect a certain type of errors, but sometimes unforeseen situations arise and the application simply “crashes”. In this case, getting information about the unhandled exception is priceless! Flurry gives us this opportunity:
public App() { UnhandledException += Application_UnhandledException; } private void Application_UnhandledException(object sender, ApplicationUnhandledExceptionEventArgs e) { FlurryWP8SDK.Api.LogError("Some error", e.ExceptionObject); }
As parameters, the LogError function takes the error message and the exception itself.


Event registration
To track user events that occur during a session, use the LogEvent function. It has a number of overloads, this is because Flurry supports several types of events:
- Ordinary events (events);
- Events with specified parameters (event parameters);
- Events with a measured duration (timed event).
protected override void OnNavigatedFrom(NavigationEventArgs e) {
As you can see from the code when registering a regular event, you just need to pass the unique identifier of this event as a string. In the case of a parameterized event, a list of parameters is added that needs to be associated with the event. If you want to create a timed event (for example, determine how long the user has been on a particular page, as was done in the example), you need to pass true and when necessary, end the event by calling the EndTimedEvent function, passing it the same event identifier . It is also possible to associate a list of parameters with timed event.

Everything is quite simple, however, there are a number of limitations:
- Each project supports no more than 300 events;
- Each event can have no more than 10 parameters;
- Unique event identifier, parameter name and parameter value cannot exceed 255 characters.

Flurry configuration
In addition, Flurry SDK has several configuration methods. They should be called (although not necessarily) after starting a new session (calling the StartSession method).
Advanced Script
If you are not satisfied with calling LogEvent every time you register an event, you can go for a little trick - create a helper class for Flurry:
public class FlurryBehavior : Behavior<UIElement> { public string EventMessage { get { return (string)GetValue(EventMessageProperty); } set { SetValue(EventMessageProperty, value); } } public static readonly DependencyProperty EventMessageProperty = DependencyProperty.Register("EventMessage", typeof (string), typeof (FlurryBehavior), new PropertyMetadata(string.Empty)); protected override void OnAttached() { base.OnAttached(); AssociatedObject.Tap += OnTap; } protected override void OnDetaching() { base.OnDetaching(); AssociatedObject.Tap -= OnTap; } private void OnTap(object sender, GestureEventArgs gestureEventArgs) { if (string.IsNullOrEmpty(EventMessage)) return; FlurryWP8SDK.Api.LogEvent(EventMessage); } }
Here we simply subscribe to the Tap event of the control and when this event occurs, we register the event on the Flurry server. An example of using this class can be viewed on the standard Panorama App template:
xmlns:i="clr-namespace:System.Windows.Interactivity;assembly=System.Windows.Interactivity" xmlns:behaviors="clr-namespace:FlurryApp" ... <phone:PanoramaItem Header="first item"> <phone:LongListSelector Margin="0,0,-22,0" ItemsSource="{Binding Items}"> <phone:LongListSelector.ItemTemplate> <DataTemplate> <StackPanel Margin="0,-6,0,12"> <TextBlock Text="{Binding LineOne}" TextWrapping="Wrap" Style="{StaticResource PhoneTextExtraLargeStyle}" FontSize="{StaticResource PhoneFontSizeExtraLarge}"/> <i:Interaction.Behaviors> <behaviors:FlurryBehavior EventMessage="{Binding LineOne}"/> </i:Interaction.Behaviors> </StackPanel> </DataTemplate> </phone:LongListSelector.ItemTemplate> </phone:LongListSelector> </phone:PanoramaItem>
Now, with tapes on the list item, we will register an event without extra code.

This example is elementary, but it can be improved:
- add parameter transfer;
- Unique identifiers of events to bring in resources;
- track application navigation events, etc.
And do not forget that for this example to work, you will need to connect the System.Windows.Interactivity assembly to the project.
findings
So, Flurry Analytics is able to measure the most popular analytical indicators, most of which can be viewed without additional configuration, which is called out of the box. The service is provided free of charge, and integration into the application is very simple. As a drawback, it is possible to note the incomplete specification of reports on failures in the application and the fact that updating the analytical data on the portal takes some time (although this is also relevant for other systems).
Using this tool in a real
Tinkoff Mobile Wallet project, based on the user transfer statistics inside the application, we decided to abandon the “Cash Deposit” section due to its low demand (making sure that users understand where this section is, but simply do not see it as ). At the same time, the “Transfer to a new bank card” section is now quite deep, and is one of the most popular. In the new version it will be closer to the main page and change the thematic section.