With the release of the new version of InterSystems Caché, it became possible to use REST technology and build applications using various Javascript UI libraries, while the Caché server acts as a data server. This article will look at a very simple example of accessing Caché stored classes using REST technology.
If to simplify, the scheme of such an application is as follows:

As can be seen from the diagram, data exchange is performed using a REST broker, using the JSON format. Thus, Caché is used only for receiving data in json format, which is processed by any Javascript UI library. This allows you to completely separate the server business logic and user interface. If desired, the user interface can be placed in static html pages, which will improve application performance. Also, this approach has a great advantage in the design of interfaces, the interface developer does not need to know Caché, he has enough JSON data that comes from the server.
')
Let us turn to the simplest implementation of this approach. To do this, you need to create a simple REST broker. Create a new class and inherit it from
% CSP.REST :
Class User.DataGateway Extends% CSP.REST
{
XData UrlMap
{
< Routes >
< Route Url = "/ get" Method = "GET" Call = "GetData" />
</ Routes >
}
ClassMethod GetData () As% Status
{
write "hello"
quit $$$ OK
}
}In order for the broker to work, you need to configure the web application. In the management portal, go to “System Administration> Security> Applications> Web Applications”, and then modify the application (in this example, “/ csp / user”). In the class handler field, specify the class
User.DataGateway just created.
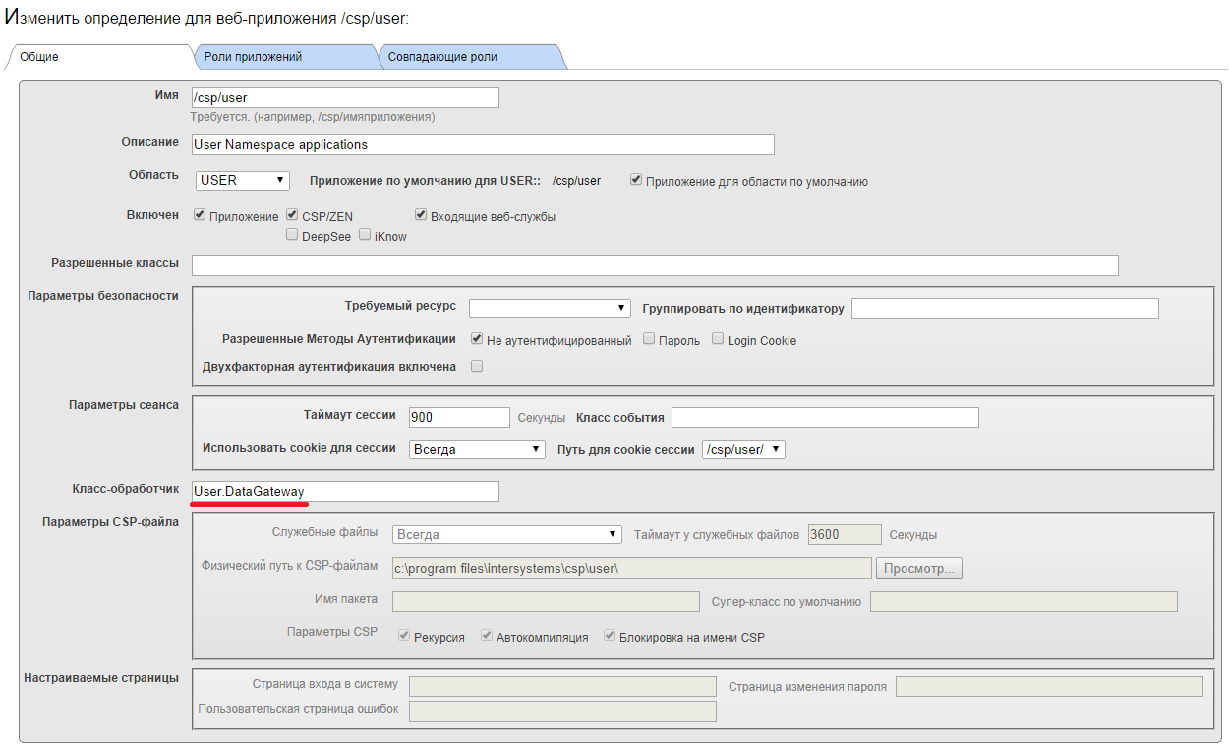
If everything is configured correctly, then go to
http: // localhost: 57772 / csp / user / get, we should see the text “hello”. Now we modify the method so that we can refer to any static class method. To do this, we use the
$ CLASSMETHOD function.
ClassMethod GetData () As% Status
{
// Necessary for an example for cross-domain queries to work
do % response . SetHeader ( "Access-Control-Allow-Origin" , "*" )
// Get the class name and method name
set class = % request . Get ( "class" , "" )
if ( class ' = "" ) {
set method = % request . Get ( "method" , "" )
if ( method '= "" ) {
try {
// Execute the class method
do $ CLASSMETHOD ( class , method )
} catch err {
write err . AsSystemError ()
}
}
}
quit $$$ OK
}To test the work of a REST broker, create a simple class that describes the organization.
Class User.GridDemo Extends% Persistent
{
Property Code As% String ;
Property NameFull As% String ;
Property NameShort As% String ;
ClassMethod InitDemoData ()
{
set o = ## class ( User.GridDemo ). % New ()
set o . Code = "001"
set o . NameFull = "Organization One"
set o . NameShort = "Org 1"
do o . % Save ()
set o = ## class ( User.GridDemo ). % New ()
set o . Code = "002"
set o . NameFull = "Organization Two"
set o . NameShort = "Org 2"
do o . % Save ()
write "Done"
}
}
Following the link http: // localhost: 57772 / csp / user / get? Class = GridDemo & method = InitDemoData , we will see “Done”. Thus, we called the InitDemoData method of the GridDemo class. Now we add a method that will generate json for the grid (in this case, the format that uses the dhtmlx grid is considered).
ClassMethod GetGridData ()
{
// Select All Data
set sql = ## class ( % SQL.Statement ). % New ()
set st = sql . % Prepare ( "select * from GridDemo" )
if ( st '= 1) {
write "% Prepare failed" , $ System .Status . DisplayError ( st )
quit
}
set rset = sql . % Execute ()
if ( rset . % SQLCODE '= 0) {
write "query error:" , rset . % SQLCODE , "" , rset . % Message
quit
}
// Build json
set json = "{" "rows" ": ["
while rset . % Next () {
set json = json _ "{" "id" ":" _ rset . Id _ "," "data" ": ["
set json = json _ "" "" _ rset . Code _ "" "," "" _ rset . NameFull _ "" ""
set json = json _ "]},"
}
// Remove the comma at the end, if it is
set len = $ length ( json )
if ( $ extract ( json , len , len ) = "," ) set json = $ EXTRACT ( json , 1, len - 1)
set json = json _ "]}"
write json
}
After that, JSON data should be displayed at localhost : 57772 / csp / user / get? Class = GridDemo & method = GetGridData.
{ "rows": [ { "id": 1, "data": [ "001","Organization One" ] },{ "id": 2, "data": [ "002","Organization Two" ] } ] }
It is worth noting that this article does not address the issue of security, as well as the correct formation of JSON. These methods are given as an illustration of the approach to the design of the application and the construction of user interfaces. In short, it is necessary to restrict access to the class methods so that you can only call methods that are oriented to data processing, and also check user rights in each method separately.
Having received JSON with data, it remains only to write a page with a user interface. To do this, we use the dhtmlx library (or Sencha Ext JS, JQuery UI, etc., simply by changing the format of the outgoing JSON).
<!DOCTYPE html> <html> <head> <title>Grid Demo</title> <link href="js/dhtmlxw/dhtmlx.css" rel="stylesheet"> <script src="js/dhtmlxw/dhtmlx.js"></script> <script src="js/jquery-1.10.2.min.js"></script> <script language='javascript'> var g = null; var l = null; $(document).ready(function (){ l = new dhtmlXLayoutObject(document.body, '1C'); g = l.cells("a").attachGrid(); l.cells("a").setText("Grid Demo"); g.setImagePath("/js/dhtmlxw/imgs/"); g.setHeader("Code,Full Name,Short name"); g.setColumnIds("Code,NameFull,NameShort"); g.attachHeader("#text_filter,#text_filter,#text_filter,#text_filter"); g.setInitWidths("120,*,200"); g.setColAlign("center,left,left,left"); g.setColTypes("ro,ro,ro,ro"); g.setColSorting('str,str,str,str'); g.init(); $.getJSON( "http://localhost:57772/csp/user/get?class=GridDemo&method=GetGridData&table=GridDemo", onDataLoaded); }); function onDataLoaded(data) { g.clearAll(); g.parse(data,'json'); } </script> </head> <body style="height:600px"> </body> </html>
For requests to the server in this example, the jQuery method getJSON is used, after which in the onDataLoaded callback, data is transferred to the grid. If done correctly, a grid should appear with the data.
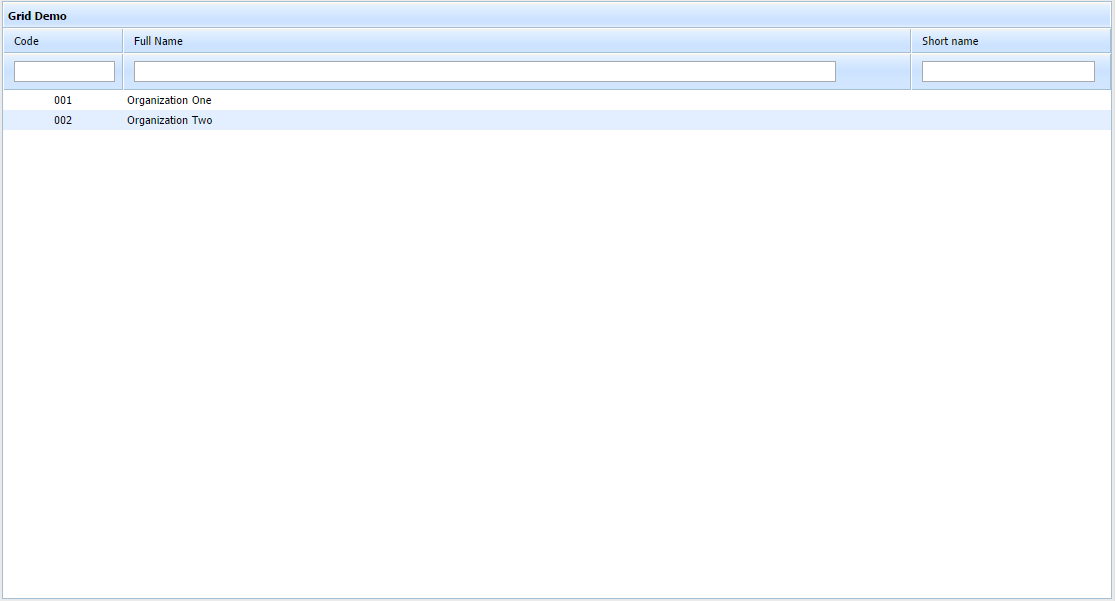
Conclusion
The article reviewed one of the methods of interacting with the Caché server through REST technology. A universal mechanism for access to any static methods has been created. Using it, JSON is formed with data, which is processed on the client using the UI library.
This article has not been reviewed:
- Security when accessing classes using REST (user rights, naming web methods).
- Formation of a correct JSON (zconvert,% ZEN.Auxiliary.jsonSQLProvider, etc.).
- Formation of an extended user interface (layout, tabs, treeview, etc.).
The example used in the article can be downloaded
here.