Hello!
I think many of you have heard about the Raspberry Pi, moreover, I think quite a large number of you have seen it live. At the beginning of 2014, I decided that it was time for me to order myself a couple of RPi too and do something interesting for them. Since I am an iOS developer, I had the burning idea of necessarily attaching an application to this iOS project. Well, because RPi is pretty good at working with third-party hardware, I decided that I would make a small security system for personal use.
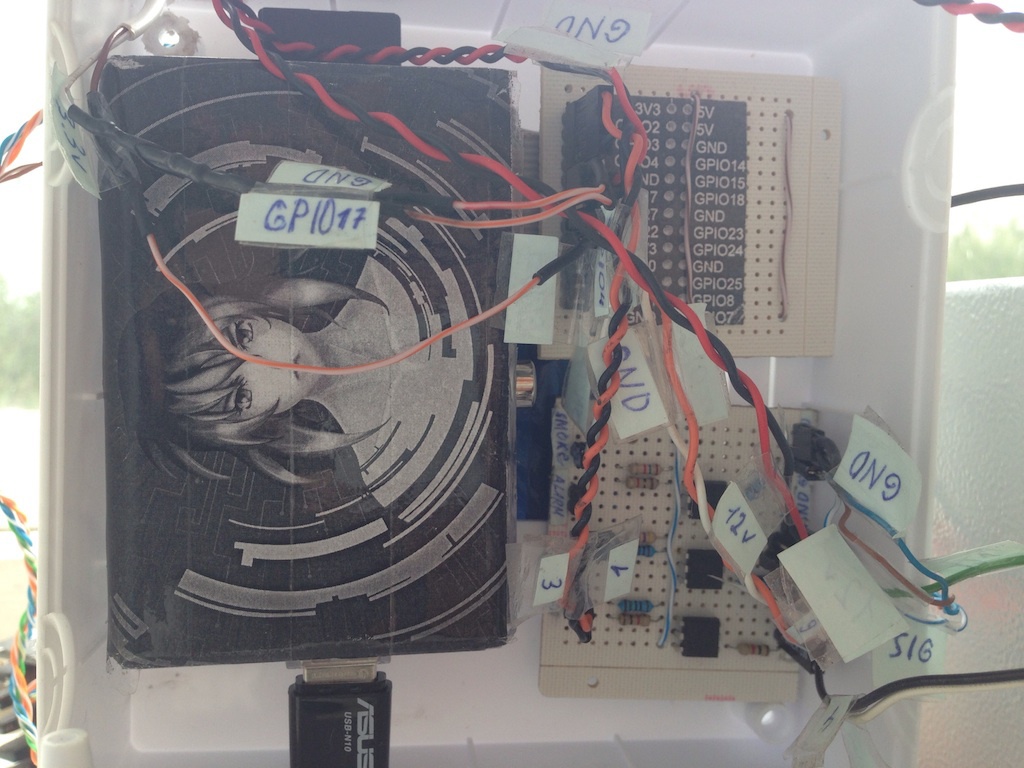
')
Introduction
The first thing to do in this case was to determine what I want from this system. I believe that for such a task there is nothing better than a thought map. In general, after 5 minutes of deep reflection, this is what happened:
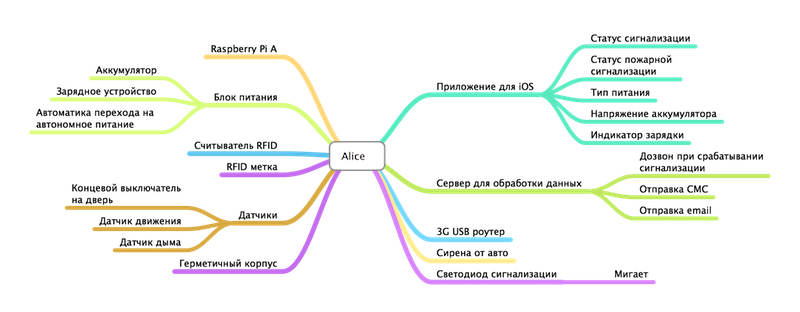
About a month later I received two RPi models A from the UK, i.e. with one USB, and no ethernet. I bought a 16GB flash drive and installed Raspbian on it. And then the first of the pitfalls began to emerge - drivers and the Internet. I already had a 3G router won at the competition a couple of years ago, and it was I who planned to connect it to RPi in order for the Internet to appear there. How wrong I was.
Half an hour later I was already in the store looking for a USB-Ethernet adapter, the seller hardly found me a copy of D-Link. Just before the release, I decided to play it safe and buy another ASUS wifi adapter, since the time was later, and I really wanted to start work today, so there was simply no room for a mistake. On both devices support Linux naturally flaunted.


When I got home, I killed a good hour trying to install from a disk, and then find the latest D-Link drivers on the Internet. It turned out that the guys from this company just stopped supporting this device 2 years ago! I realized that they sold me junk (
which I successfully changed the next day for two more flash drives ). There was a plan B, which worked surprisingly without any drivers, I just inserted the adapter from ASUS into USB and it was immediately defined in the system as a network card. I’ll say right away that it’s just impossible to work on the RPi model A in the graphical shell mode, everything is very slow, after the MacBook with the SSD, this is about hell. So I decided not to torture him, just set up ssh for myself.
Next came the question of the language in which I would write a system for working with hardware. Of all, I chose Python, since there is work with iron out of the box, there is no need to additionally install any libraries and the like, well, plus I have long wanted to learn this language.
In about a week, I learned everything I needed from Python, it was a very interesting week. Remember in episode 9 of the first season of The Big Bang Theory, the main characters attached switches to some devices in their apartment that could be controlled via the Internet, and anyone could turn on / off their light or audio system? I did the same with the LED connected to the RPi. This is certainly not an audio system, but when the LED lights up from a mouse click on the opposite hemisphere of the planet, the sensations were indescribable :)

Work with iron
The next question that I had to solve was how to connect and control the iron, because the motion and smoke sensor is not a LED, everything is somewhat more complicated. With this question, I turned to my good friend, who just knows a lot about circuit design and is friends with a soldering iron better than me.
From that moment on, two people began to work on the project, and I became a frequent visitor to the store for radio amateurs.
In more detail about the operation of the scheme, I will tell in another article, but for now I’ll briefly tell you how everything works.
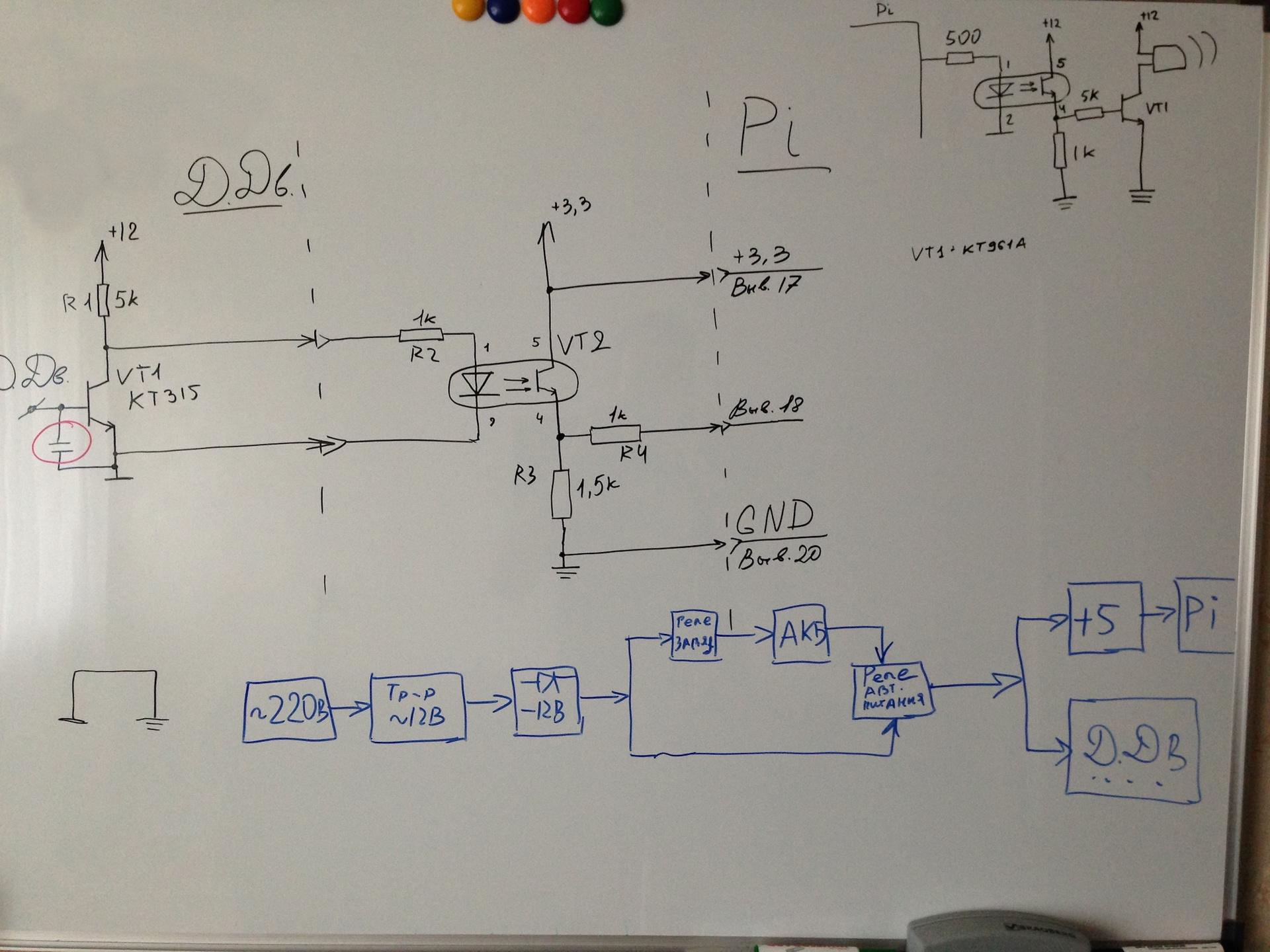
In this diagram, there is a motion sensor on the left, a car alarm on the right, and a power supply connection below.
Looking ahead, I want to say that the automatic switching to battery power was excluded from the circuit, because A battery was purchased, which is connected just parallel with the mains supply and automatically starts feeding the circuit when 220V is lost. It was decided to replace the limit switch for the door with reed switches.
To connect and power the entire circuit, two separate boards were made, on one, work with iron through optocouplers was implemented, and on the other, mains voltage conversion of 220V to constant 12V and 5V. From 12V sensors and a siren are powered, and from 5V, respectively, RPi.
All this is good, but the next question was even more interesting, how do we get the data from the sensors. We decided to do all the work with iron through GPIO, to be honest, I think this is the only way, the remaining inputs / outputs are designed for specific equipment, and we just need to get a 1 or 0 status from the sensors.
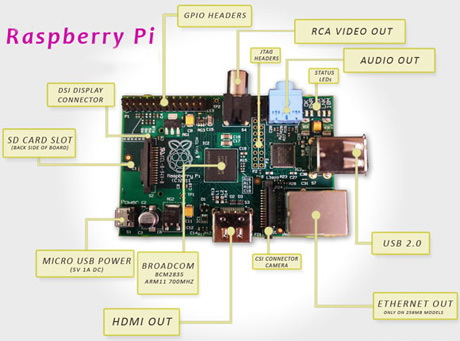
The GPIO has 26 pins, from them: 2 to 5V, 2 to 3B, 5 ground, the rest can be both inputs and outputs, depending on the configuration that you specify.

Programming system work with iron
I decided to make a three-tier architecture:
RPi - Web Server - iOS . First, to increase resiliency, because if we exclude the server from the chain, then the whole system becomes very vulnerable due to the fact that RPi can fail and we will not know how to do it. Secondly, I decided to refuse sending SMS and dialing in favor of push-notifications, which of course the server will send.
So, for programming the system to work with iron, I chose Python 2.5. The first thing to do is install
WebIOPi . This is an excellent open-source project that allows you to conveniently debug work with equipment through a browser. It shows the status of GPIO ports, allows them to be configured for input / output and set manually at output 0 or 1. Everything is installed very simply, on the Internet even there is a detailed instruction in Russian.
As I wrote above, in Python "out of the box" there is work with GPIO, for this you just need to connect a special library.
import RPi.GPIO as GPIO
The GPIO has two contact numbering modes, by account on the board and by internal contact number. I recommend using account numbering, it's just easier.
GPIO.setmode(GPIO.BOARD)
Before starting the system, we need to initialize all the necessary ports, namely, indicate how they will work, at the input or at the output, as well as specify the initial value of the port.
GPIO.setup(12, GPIO.OUT, initial=GPIO.LOW) GPIO.setup(11, GPIO.IN, pull_up_down=GPIO.PUD_DOWN)
To read the port you need to use the following code:
if (GPIO.input(11) == GPIO.HIGH)
To set port 1 or 0 output:
GPIO.output(12, GPIO.HIGH) GPIO.output(12, GPIO.LOW)
Since the motion sensor issues a signal for a very short period of time, we need to read the ports all the time, and here we must say that this is very convenient in RPi.GPIO. You can make it so that when an input is received at 1 or 0, a certain function is called. Also for each port is set the frequency of reading.
GPIO.add_event_detect(11, GPIO.FALLING, callback=move_sensor_callback, bouncetime=500)
Several important pitfalls. In the program loop, it is necessary to sleep (1), otherwise the processor will always be 100% loaded, also when exiting the program it is recommended to perform GPIO.cleanup ().
try: while True: sleep(1) finally: GPIO.cleanup()
Then I will tell you about an even more strange problem, the reasons for which I did not find, but I found a completely adequate solution. After about 6 hours of continuous operation, the script stops responding to the sensors. Most likely, the most convenient system that causes a callback function when a certain event occurs falls off. This is solved very simply, the script must sometimes be reloaded, for example with the help of cron. For greater reliability, on my system, cron reloads the script every hour, plus every minute it checks if the script is running and starts it if it is not.
I did the work of the program as a daemon, I think I don’t need to explain anything here, this is a pretty standard thing. If you wish, you can find examples on the Internet.
Let's talk a little about the system architecture. I carried out work with each piece of iron into a separate module. Each module can initialize ports, turn on / off its hardware, carry out self-diagnostics, send messages to the server, for example, when a motion sensor is triggered, and of course, write your status to the log.
Self-diagnostics is done quite simply, during initialization all the iron should have a unit at the output, so if we have zero there, then the sensor does not work or is not in the closed position (reed switch). Accordingly, the whole system is built on the fact that the sensors have 1 in a quiet state, and accordingly 0, when they are triggered or something breaks.
In general, the system consists of 4 sensors: a 360 ° motion sensor, a smoke sensor, 2 reed switches. And of course the siren, ringing, car, in a closed room just breaks your ears.
This is where the first part comes to an end, the next time I will tell you how the server part and the iOS application were made. Thank you all for your attention, and if possible I will answer all questions on hardware and software in the comments.
Well, at the end of a small spoiler for the second part :)
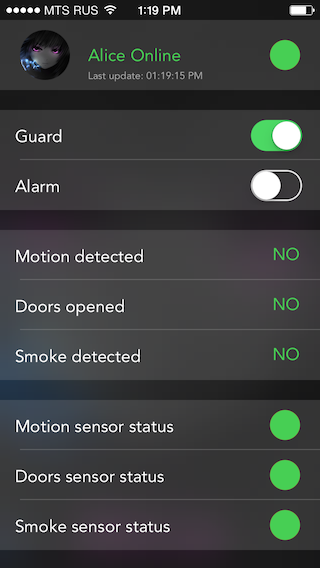