Hello.
The seemingly trivial task to write the post was a trivial task - monitoring the temperature in the server room. There are quite a few different solutions on this topic (for example, hang a video camera and a thermometer in front of it), but most of the steep monitoring systems, automatic control of air conditioners, etc. worth a lot of money. The difference is the proposed option - the budget. About 250 Russian rubles and a little brain (priceless).
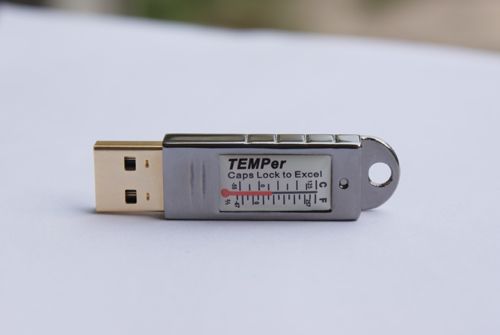
The solution of the problem began, naturally, with the search for a temperature sensor. Cheap, because there are a lot of dear ones, and for a long time to explain to the management. Also, in order to save, the USB sensor option was chosen (LAN is more expensive). Where do we have the cheapest sensors? Right on ebay. We go there and at the request of “usb thermometer” we quickly find a toy like the one in the picture. We order, arrange, wait for delivery.
At this financial spending ends and begins the work of the brain.
Included with the sensor is a CD with the program
TEMPer 24.4.4 . Despite the impressive version number, it is impossible to look at this miracle of programmer thought without tears. Yes, it has a lot of functions: temperature graph, sending messages by email, msn and skype, a log in a text file, setting critical temperatures for alarms. And it even works. There is no only the most important - start in service mode or command line. So, there is no implied automation capability (parsing of logs is not counted).
')
So, the program proposed by the developers is not suitable for all parameters. What is the alternative? Own software for temperature. In the base case, I needed a program that showed the temperature from the sensor. All further processing such as entering into the database, sending letters of alarm, analyzing the situation, etc. Easily hung on the scheduler, polling the sensor every minute.
Searching the Internet easily leads to a module for
python , called
temper-python . Fine! This is already a normal software with a cross-platform interpreter. FIG there. This module only works under linux. And not in vain in the title made another OSes. My application server runs on Windows. But this is python! So, the script can certainly be remade to work in Windows. And this will do.
First, insert the sensor itself into the usb port of the computer (server). This is enough for an experiment, but in practice it is much better to supplement the sensor with a remote extension stand. It can be ordered together with a sensor from China, bought in any store or found in any server room - as a trophy from various transmitters or flash drives. Windows will automatically install standard drivers on the sensor and identify it as a “Composite USB device.”
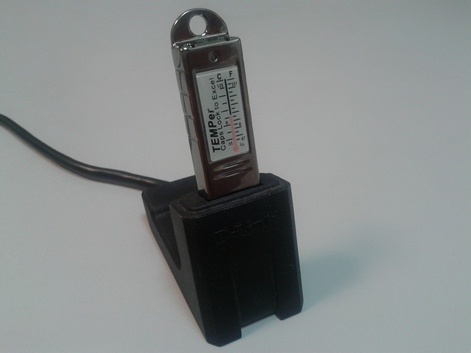
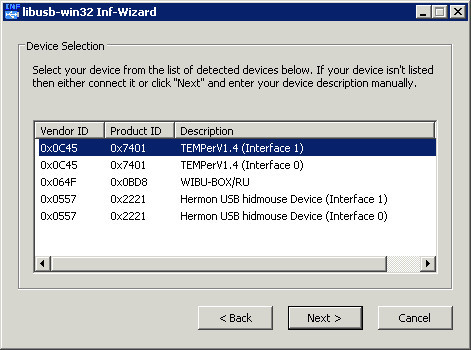
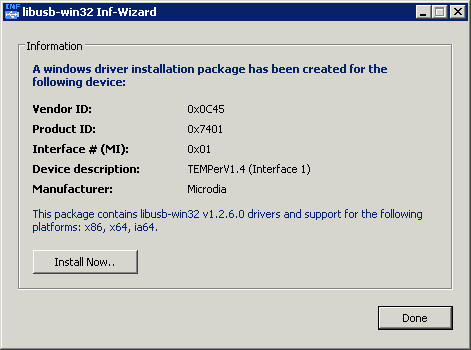
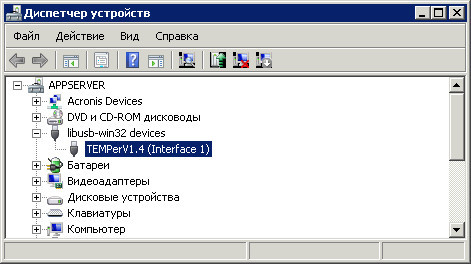
I didn’t care which python to work with, so I took the latest available version,
3.4.1 . Actually, if it is important for someone, it’s not a problem to remake the script for the younger version - here and there you can remove brackets and quotes. Download, set,
enjoy :
www.python.org .
Immediately to the python you need to put the library to work with USB. No problem either. It is called
pyusb . We take it from the project page on
sourceforge.net . The archive has a file
setup.py . Run it from the command line:
cd C:\Downloads\pyusb-1.0.0a2
C:\Python34\python.exe setup.py install
Pyusb works with usb system access libraries -
libusb 0.1 ,
libusb 1.0 ,
libusbx ,
libusb-win32 and
OpenUSB . For Windows users, the
libusb-win32 option is available, which also needs to be installed and configured. Download the archive from the project page on
sourceforge.net . And do not be confused by
win32 in the name - the driver works fine under
x64 .
Unpack, go to the
bin folder and run the
inf-wizard.exe file . This program will automatically generate a folder of drivers for a specific device and install them.
In the list of devices to install the driver, you must select
TEMPerV1.4 (Interface 1) . Click
Next , agree with everything you can agree with and get to the window for saving drivers. Here it is better to create a separate folder for
temper , where the utility will add all the necessary drivers. After that, you will be prompted to install the created driver. Do this with the
Install Now button
...If everything went well, the "
Composite USB device " in the Device Manager will disappear and
TEMPerV1.4 (Interface 1) will appear.
In the
bin / amd64 folder of the
libusb-win32 distribution kit there is a utility
testlibusb-win.exe . Run it to make sure that the device we need is visible to the system. If everything is good and the device is visible, the most interesting thing remains - the python script.
As already written above, I took the
temper-python module as a
basis . This module is heaped up, which needs to be installed, in which there is a config for amendments, the choice of Celsius or Fahrenheit, and even support for SNMP. This is all cool, but I do not need. Therefore, simplify every one script that displays the temperature in the console. In addition, in
temper-python there is also a bunch of lines that are needed only in linux, and in windows they simply do not work.
Let's start by setting global variables:
import sys, usb VENDOR_ID = 0x0c45 PRODUCT_ID = 0x7401 TIMEOUT = 5000 OFFSET = 0 INTERFACE = 1 REQ_INT_LEN = 8 ENDPOINT = 0x82 COMMANDS = { 'temp': b'\x01\x80\x33\x01\x00\x00\x00\x00', 'ini1': b'\x01\x82\x77\x01\x00\x00\x00\x00', 'ini2': b'\x01\x86\xff\x01\x00\x00\x00\x00', }
By
VENDOR_ID and
PRODUCT_ID, the script will find among all Temper devices and will use it.
TIMEOUT and
OFFSET, in general, are understandable without explanation - the access timeout to the sensor and temperature correction. The amendment is necessary, since a cheap Chinese sensor can make mistakes within a few degrees, but it is enough to determine it once from a room thermometer and forget it.
The remaining variables refer to the api sensor and do not touch them.
Now the most important thing is the class for accessing the sensor.
class TemperDevice(object): def __init__(self, device): self._device = device def get_temperature(self): if self._device is None: return "Device not ready" else: try: self._device.set_configuration() ret = self._device.ctrl_transfer(bmRequestType=0x21, bRequest=0x09, wValue=0x0201, wIndex=0x00, data_or_wLength=b'\x01\x01', timeout=TIMEOUT) self._control_transfer(COMMANDS['temp']) self._interrupt_read() self._control_transfer(COMMANDS['ini1']) self._interrupt_read() self._control_transfer(COMMANDS['ini2']) self._interrupt_read() self._interrupt_read() self._control_transfer(COMMANDS['temp']) data = self._interrupt_read() self._device.reset() temp = (data[3] & 0xFF) + (data[2] << 8) temp_c = temp * (125.0 / 32000.0) temp_c = temp_c + OFFSET return temp_c except usb.USBError as err: if "not permitted" in str(err): return "Permission problem accessing USB." else: return err except: return "Unexpected error:", sys.exc_info()[0] raise def _control_transfer(self, data): ret = self._device.ctrl_transfer(bmRequestType=0x21, bRequest=0x09, wValue=0x0200, wIndex=0x01, data_or_wLength=data, timeout=TIMEOUT) def _interrupt_read(self): data = self._device.read(ENDPOINT, REQ_INT_LEN, interface=INTERFACE, timeout=TIMEOUT) return data
_control_transfer is responsible for sending commands to the sensor,
_interrupt_read is
responsible for reading the response. In
get_temperature, we actually send all these commands, read the answer, and convert the set of numbers to human-readable temperature.
To make it all work at startup, a few lines to initialize:
dev = usb.core.find(idVendor=VENDOR_ID, idProduct=PRODUCT_ID) TDev = TemperDevice(dev) output = TDev.get_temperature() print(output)
The first line is looking for our
TEMPer among the devices, the second and third - request the temperature.
That's all. You can check the script operation using the standard command in the console:
C:\Python34\python.exe temper.py
The temperature will be displayed from the sensor. What to do with it - let everyone decide for himself.