<!DOCTYPE html> <html> <head> <title>Example 01.01 - Basic skeleton</title> <script type="text/javascript" src="../libs/three.js"> </script> <script type="text/javascript" src="../libs/jquery-1.9.0.js"> </script> <style> body{ /* set margin to 0 and overflow to hidden, to use the complete page */ margin: 0; overflow: hidden; } </style> </head> <body> <!--Div which will hold the Output --> <div id="WebGL-output"> </div> <!--Javascript code that runs our Three.js examples --> <script type="text/javascript"> // once everything is loaded, we run our Three.js stuff. $(function () { // here we'll put the Three.js stuff }); </script> </body> </html>
Three.js
and jquery-1.9.0.js
. Also in this block we prescribe a couple of lines for CSS design. In the previous snippet, you can see some JavaScript code. This small piece of code uses JQuery
when calling an unnamed JavaScript function when the page is fully loaded. We will put all the Three.js code inside this function.Three.js r60
project, which was released in August 2013. <script type="text/javascript"> $(function () { var scene = new THREE.Scene(); var camera = new THREE.PerspectiveCamera(45 , window.innerWidth / window.innerHeight , 0.1, 1000); var renderer = new THREE.WebGLRenderer(); renderer.setClearColorHex(0xEEEEEE); renderer.setSize(window.innerWidth, window.innerHeight); var axes = new THREE.AxisHelper( 20 ); scene.add(axes); var planeGeometry = new THREE.PlaneGeometry(60,20,1,1); var planeMaterial = new THREE.MeshBasicMaterial({color: 0xcccccc}); var plane = new THREE.Mesh(planeGeometry,planeMaterial); plane.rotation.x=-0.5*Math.PI; plane.position.x = 15; plane.position.y = 0; plane.position.z = 0; scene.add(plane); var cubeGeometry = new THREE.CubeGeometry(4,4,4); var cubeMaterial = new THREE.MeshBasicMaterial( {color: 0xff0000, wireframe: true}); var cube = new THREE.Mesh(cubeGeometry, cubeMaterial); cube.position.x = -4; cube.position.y = 3; cube.position.z = 0; scene.add(cube); var sphereGeometry = new THREE.SphereGeometry(4,20,20); var sphereMaterial = new THREE.MeshBasicMaterial( {color: 0x7777ff, wireframe: true}); var sphere = new THREE.Mesh(sphereGeometry,sphereMaterial); sphere.position.x = 20; sphere.position.y = 4; sphere.position.z = 2; scene.add(sphere); camera.position.x = -30; camera.position.y = 40; camera.position.z = 30; camera.lookAt(scene.position); $("#WebGL-output").append(renderer.domElement); renderer.render(scene, camera); });
var scene = new THREE.Scene(); var camera = new THREE.PerspectiveCamera(45 , window.innerWidth / window.innerHeight , 0.1, 1000); var renderer = new THREE.WebGLRenderer(); renderer.setClearColorHex(0xEEEEEE); renderer.setSize(window.innerWidth, window.innerHeight);
WebGLRenderer
object that will use the video card to render the scene.setClearColorHex()
function, we set the renderer's background color to almost white (0XEEEEEE), and also set the size of the rendered scene using the setSize()
function.render
and camera
. But, however, there is still nothing to visualize. The following code snippet adds auxiliary axes and a plane. var axes = new THREE.AxisHelper( 20 ); scene.add(axes); var planeGeometry = new THREE.PlaneGeometry(60,20); var planeMaterial = new THREE.MeshBasicMaterial( {color: 0xcccccc}); var plane = new THREE.Mesh(planeGeometry,planeMaterial); plane.rotation.x = -0.5*Math.PI; plane.position.x = 15; plane.position.y = 0; plane.position.z = 0; scene.add(plane);
scene.add()
function to add axes to our scene. Now we can create a plane. This is done in two steps. First we determine that the plane will be displayed using new THREE
. The code will be PlaneGeometry(60,20)
. In this case, our plane will have a width of 60 and a height of 20. We should also tell Three.js what our plane should look like (for example, its color or transparency). In Three.js, we do this by creating a material object. For the first example, we will create a base material (using the MeshBasicMaterial()
method) of color 0xcccccc. Next, we merge these two objects into one Mesh object called plane
. Before we add a plane
to the scene, we must put it in the correct position; we do this by first turning it 90 degrees around the x axis, and then we determine its position on the stage using the position
property. Finally, we have to add this object to the scene, just as we did with the axes
object.wireframe
property is true
, so let's move on to the final part of this example: camera.position.x = -30; camera.position.y = 40; camera.position.z = 30; camera.lookAt(scene.position); $("#WebGL-output").append(renderer.domElement); renderer.render(scene, camera);
position
attribute and it starts hovering over our scene. To make sure the camera is looking at our objects, we use the lookAt()
function to point to the center of our scene. var spotLight = new THREE.SpotLight( 0xffffff ); spotLight.position.set( -40, 60, -10 ); scene.add(spotLight );
SpotLight()
method illuminates our scene from the position of spotLight.position.set( -40, 60, -10 )
. If you render the scene again, you will notice that there is no difference with the previous example. The reason is that different materials react differently to light. The main material that we used in the previous example for objects (using the MeshBasicMaterial
() method) does not react to light sources on the stage. They simply visualized objects in a specific color. Thus, we need to change the materials for our plane, sphere and cube as shown below: var planeGeometry = new THREE.PlaneGeometry(60,20); var planeMaterial = new THREE.MeshLambertMaterial( {color: 0xffffff}); var plane = new THREE.Mesh(planeGeometry,planeMaterial); ... var cubeGeometry = new THREE.CubeGeometry(4,4,4); var cubeMaterial = new THREE.MeshLambertMaterial( {color: 0xff0000}); var cube = new THREE.Mesh(cubeGeometry, cubeMaterial); ... var sphereGeometry = new THREE.SphereGeometry(4,20,20); var sphereMaterial = new THREE.MeshLambertMaterial( {color: 0x7777ff}); var sphere = new THREE.Mesh(sphereGeometry,sphereMaterial);
renderer.setClearColorHex(0xEEEEEE, 1.0); renderer.setSize(window.innerWidth, window.innerHeight); renderer.shadowMapEnabled = true;
render
that we want to include shadows. You can do this by setting the shadowMapEnabled
property to true
. If you look at the result of this action, we will not notice anything. This happens because we have to explicitly specify which objects will cast shadows and on which objects it will fall. In our example, we want the sphere and the cube to cast a shadow on our plane. You can do this by setting the corresponding properties to true: plane.receiveShadow = true; ... cube.castShadow = true; ... sphere.castShadow = true;
SpotLight()
method. Now we just have to set the correct property and finally visualize the shadows. spotLight.castShadow = true;
setInterval(function,interval)
. With this function, we can define a function that will be called every 100 milliseconds. The problem with this function is that it does not take into account what happens in the browser. If you are viewing another tab, then this function will still be called every few milliseconds. In addition, the setInterval()
method is not synchronized with screen redrawing. All this can lead to high CPU utilization and low efficiency.setInterval()
function: the requestAnimationFrame
() method. With this function you can specify a function that will be called at intervals defined by the browser. You just have to create a function that will handle rendering, as shown below: function renderScene() { requestAnimationFrame(renderScene); renderer.render(scene, camera); }
renderScene
() function, we call the requestAnimationFrame
() method again to keep the animation going. The only thing we need to do is change the location of the call to the renderer.render()
method after we have created the entire scene, we call the renderScene
() function renderScene
to start the animation: ... $("#WebGL-output").append(renderer.domElement); renderScene();
:
<script type="text/javascript" src="../libs/stats.js"></script>
,
<div id="Stats-output"></div>
, β , :
function initStats() { var stats = new Stats(); stats.setMode(0); stats.domElement.style.position = 'absolute'; stats.domElement.style.left = '0px'; stats.domElement.style.top = '0px'; $("#Stats-output").append(stats.domElement ); return stats; }
. setMode
(). 0, FPS, 1, . FPS, 0. jQuery :
$(function () { var stats = initStats(); β¦ }
render():
function render() { stats.update(); ... requestAnimationFrame(render); renderer.render(scene, camera); }
, , :
requestAnimationFrame
() . render
() , . :
function render() { ... cube.rotation.x += 0.02; cube.rotation.y += 0.02; cube.rotation.z += 0.02; ... requestAnimationFrame(render); renderer.render(scene, camera); }
, ? β rotation
0,02 render
(), . , , .
render
() :
var step=0; function render() { ... step+=0.04; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... requestAnimationFrame(render); renderer.render(scene, camera); }
rotation
; position
. , , . . Math.cos()
Math.sin()
step
. . , step+=0.04
. :
.
, . 3D-, , , . , , . , .
dat.GUI
Google dat.GUI( ), . , , :
, dat.GUI , :
<script type="text/javascript" src="../libs/dat.gui.js"></script>
β JavaScript , , , dat.GUI. JavaScript :
var controls = new function() { this.rotationSpeed = 0.02; this.bouncingSpeed = 0.03; }
: this.rotationSpeed
this.bouncingSpeed
. dat.GUI , :
var gui = new dat.GUI(); gui.add(controls, 'rotationSpeed',0,0.5); gui.add(controls, 'bouncingSpeed',0,0.5);
rotationSpeed
bouncingSpeed
0 0,5. , β , render
, , dat.GUI, . :
function render() { ... cube.rotation.x += controls.rotationSpeed; cube.rotation.y += controls.rotationSpeed; cube.rotation.z += controls.rotationSpeed; step+=controls.bouncingSpeed; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... }
, , , :
.
ASCII
3D- . Three.js , , . , : ASCII . , -ASCII, . :
$("#WebGL-output").append(renderer.domElement);
:
var effect = new THREE.AsciiEffect( renderer ); effect.setSize(window.innerWidth, window.innerHeight ); $("#WebGL-output").append(effect.domElement);
. renderer.render(scene, camera), effect.render(scene,camera). :
.
, , , Three.js .
. , Three.js .
, . , Three.js.
, : GitHub
:
<script type="text/javascript" src="../libs/stats.js"></script>
,
<div id="Stats-output"></div>
, β , :
function initStats() { var stats = new Stats(); stats.setMode(0); stats.domElement.style.position = 'absolute'; stats.domElement.style.left = '0px'; stats.domElement.style.top = '0px'; $("#Stats-output").append(stats.domElement ); return stats; }
. setMode
(). 0, FPS, 1, . FPS, 0. jQuery :
$(function () { var stats = initStats(); β¦ }
render():
function render() { stats.update(); ... requestAnimationFrame(render); renderer.render(scene, camera); }
, , :

requestAnimationFrame
() . render
() , . :
function render() { ... cube.rotation.x += 0.02; cube.rotation.y += 0.02; cube.rotation.z += 0.02; ... requestAnimationFrame(render); renderer.render(scene, camera); }
, ? β rotation
0,02 render
(), . , , .
render
() :
var step=0; function render() { ... step+=0.04; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... requestAnimationFrame(render); renderer.render(scene, camera); }
rotation
; position
. , , . . Math.cos()
Math.sin()
step
. . , step+=0.04
. :
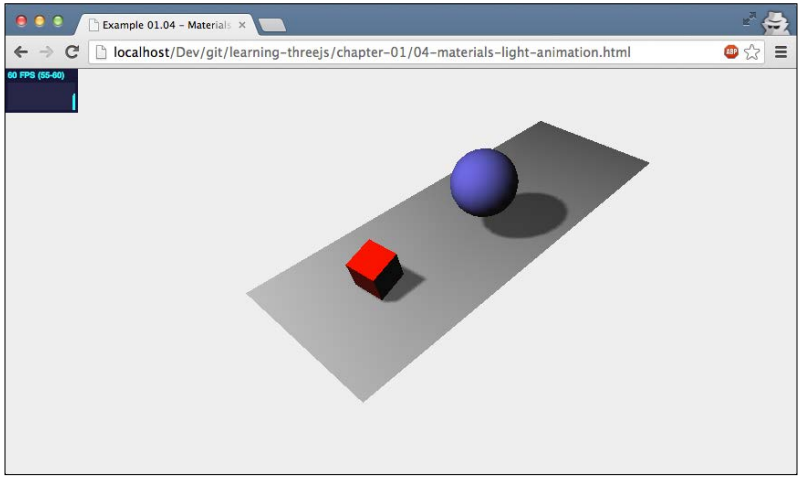
.
, . 3D-, , , . , , . , .
dat.GUI
Google dat.GUI( ), . , , :
, dat.GUI , :
<script type="text/javascript" src="../libs/dat.gui.js"></script>
β JavaScript , , , dat.GUI. JavaScript :
var controls = new function() { this.rotationSpeed = 0.02; this.bouncingSpeed = 0.03; }
: this.rotationSpeed
this.bouncingSpeed
. dat.GUI , :
var gui = new dat.GUI(); gui.add(controls, 'rotationSpeed',0,0.5); gui.add(controls, 'bouncingSpeed',0,0.5);
rotationSpeed
bouncingSpeed
0 0,5. , β , render
, , dat.GUI, . :
function render() { ... cube.rotation.x += controls.rotationSpeed; cube.rotation.y += controls.rotationSpeed; cube.rotation.z += controls.rotationSpeed; step+=controls.bouncingSpeed; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... }
, , , :

.
ASCII
3D- . Three.js , , . , : ASCII . , -ASCII, . :
$("#WebGL-output").append(renderer.domElement);
:
var effect = new THREE.AsciiEffect( renderer ); effect.setSize(window.innerWidth, window.innerHeight ); $("#WebGL-output").append(effect.domElement);
. renderer.render(scene, camera), effect.render(scene,camera). :
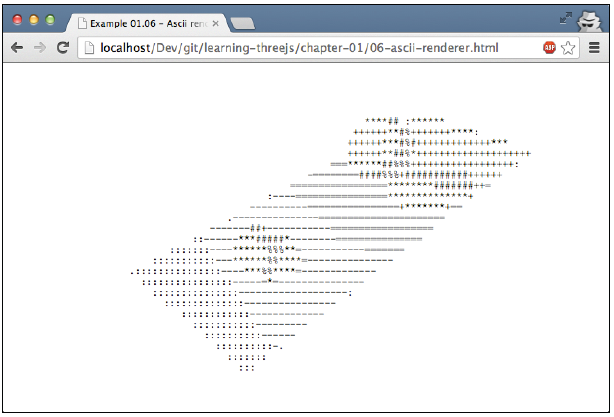
.
, , , Three.js .
. , Three.js .
, . , Three.js.
, : GitHub
:
<script type="text/javascript" src="../libs/stats.js"></script>
,
<div id="Stats-output"></div>
, β , :
function initStats() { var stats = new Stats(); stats.setMode(0); stats.domElement.style.position = 'absolute'; stats.domElement.style.left = '0px'; stats.domElement.style.top = '0px'; $("#Stats-output").append(stats.domElement ); return stats; }
. setMode
(). 0, FPS, 1, . FPS, 0. jQuery :
$(function () { var stats = initStats(); β¦ }
render():
function render() { stats.update(); ... requestAnimationFrame(render); renderer.render(scene, camera); }
, , :
requestAnimationFrame
() . render
() , . :
function render() { ... cube.rotation.x += 0.02; cube.rotation.y += 0.02; cube.rotation.z += 0.02; ... requestAnimationFrame(render); renderer.render(scene, camera); }
, ? β rotation
0,02 render
(), . , , .
render
() :
var step=0; function render() { ... step+=0.04; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... requestAnimationFrame(render); renderer.render(scene, camera); }
rotation
; position
. , , . . Math.cos()
Math.sin()
step
. . , step+=0.04
. :
.
, . 3D-, , , . , , . , .
dat.GUI
Google dat.GUI( ), . , , :
, dat.GUI , :
<script type="text/javascript" src="../libs/dat.gui.js"></script>
β JavaScript , , , dat.GUI. JavaScript :
var controls = new function() { this.rotationSpeed = 0.02; this.bouncingSpeed = 0.03; }
: this.rotationSpeed
this.bouncingSpeed
. dat.GUI , :
var gui = new dat.GUI(); gui.add(controls, 'rotationSpeed',0,0.5); gui.add(controls, 'bouncingSpeed',0,0.5);
rotationSpeed
bouncingSpeed
0 0,5. , β , render
, , dat.GUI, . :
function render() { ... cube.rotation.x += controls.rotationSpeed; cube.rotation.y += controls.rotationSpeed; cube.rotation.z += controls.rotationSpeed; step+=controls.bouncingSpeed; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... }
, , , :
.
ASCII
3D- . Three.js , , . , : ASCII . , -ASCII, . :
$("#WebGL-output").append(renderer.domElement);
:
var effect = new THREE.AsciiEffect( renderer ); effect.setSize(window.innerWidth, window.innerHeight ); $("#WebGL-output").append(effect.domElement);
. renderer.render(scene, camera), effect.render(scene,camera). :
.
, , , Three.js .
. , Three.js .
, . , Three.js.
, : GitHub
:
<script type="text/javascript" src="../libs/stats.js"></script>
,
<div id="Stats-output"></div>
, β , :
function initStats() { var stats = new Stats(); stats.setMode(0); stats.domElement.style.position = 'absolute'; stats.domElement.style.left = '0px'; stats.domElement.style.top = '0px'; $("#Stats-output").append(stats.domElement ); return stats; }
. setMode
(). 0, FPS, 1, . FPS, 0. jQuery :
$(function () { var stats = initStats(); β¦ }
render():
function render() { stats.update(); ... requestAnimationFrame(render); renderer.render(scene, camera); }
, , :

requestAnimationFrame
() . render
() , . :
function render() { ... cube.rotation.x += 0.02; cube.rotation.y += 0.02; cube.rotation.z += 0.02; ... requestAnimationFrame(render); renderer.render(scene, camera); }
, ? β rotation
0,02 render
(), . , , .
render
() :
var step=0; function render() { ... step+=0.04; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... requestAnimationFrame(render); renderer.render(scene, camera); }
rotation
; position
. , , . . Math.cos()
Math.sin()
step
. . , step+=0.04
. :
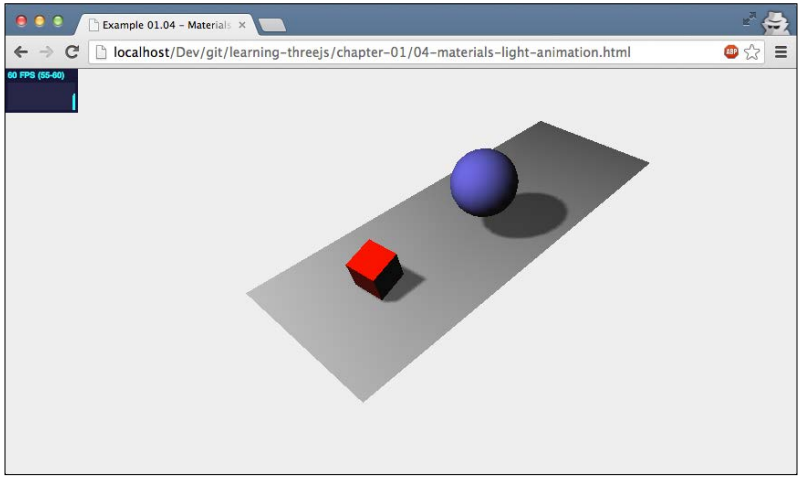
.
, . 3D-, , , . , , . , .
dat.GUI
Google dat.GUI( ), . , , :
, dat.GUI , :
<script type="text/javascript" src="../libs/dat.gui.js"></script>
β JavaScript , , , dat.GUI. JavaScript :
var controls = new function() { this.rotationSpeed = 0.02; this.bouncingSpeed = 0.03; }
: this.rotationSpeed
this.bouncingSpeed
. dat.GUI , :
var gui = new dat.GUI(); gui.add(controls, 'rotationSpeed',0,0.5); gui.add(controls, 'bouncingSpeed',0,0.5);
rotationSpeed
bouncingSpeed
0 0,5. , β , render
, , dat.GUI, . :
function render() { ... cube.rotation.x += controls.rotationSpeed; cube.rotation.y += controls.rotationSpeed; cube.rotation.z += controls.rotationSpeed; step+=controls.bouncingSpeed; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... }
, , , :

.
ASCII
3D- . Three.js , , . , : ASCII . , -ASCII, . :
$("#WebGL-output").append(renderer.domElement);
:
var effect = new THREE.AsciiEffect( renderer ); effect.setSize(window.innerWidth, window.innerHeight ); $("#WebGL-output").append(effect.domElement);
. renderer.render(scene, camera), effect.render(scene,camera). :
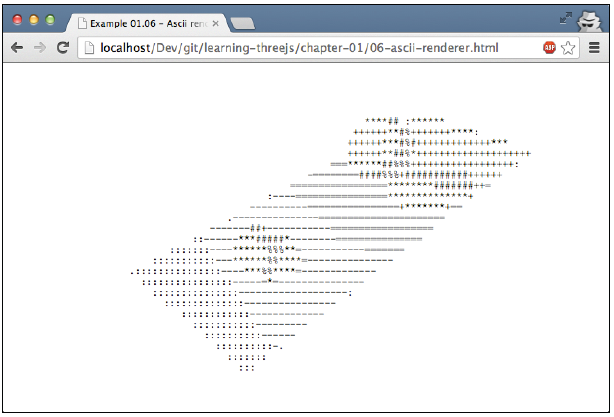
.
, , , Three.js .
. , Three.js .
, . , Three.js.
, : GitHub
:
<script type="text/javascript" src="../libs/stats.js"></script>
,
<div id="Stats-output"></div>
, β , :
function initStats() { var stats = new Stats(); stats.setMode(0); stats.domElement.style.position = 'absolute'; stats.domElement.style.left = '0px'; stats.domElement.style.top = '0px'; $("#Stats-output").append(stats.domElement ); return stats; }
. setMode
(). 0, FPS, 1, . FPS, 0. jQuery :
$(function () { var stats = initStats(); β¦ }
render():
function render() { stats.update(); ... requestAnimationFrame(render); renderer.render(scene, camera); }
, , :
requestAnimationFrame
() . render
() , . :
function render() { ... cube.rotation.x += 0.02; cube.rotation.y += 0.02; cube.rotation.z += 0.02; ... requestAnimationFrame(render); renderer.render(scene, camera); }
, ? β rotation
0,02 render
(), . , , .
render
() :
var step=0; function render() { ... step+=0.04; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... requestAnimationFrame(render); renderer.render(scene, camera); }
rotation
; position
. , , . . Math.cos()
Math.sin()
step
. . , step+=0.04
. :
.
, . 3D-, , , . , , . , .
dat.GUI
Google dat.GUI( ), . , , :
, dat.GUI , :
<script type="text/javascript" src="../libs/dat.gui.js"></script>
β JavaScript , , , dat.GUI. JavaScript :
var controls = new function() { this.rotationSpeed = 0.02; this.bouncingSpeed = 0.03; }
: this.rotationSpeed
this.bouncingSpeed
. dat.GUI , :
var gui = new dat.GUI(); gui.add(controls, 'rotationSpeed',0,0.5); gui.add(controls, 'bouncingSpeed',0,0.5);
rotationSpeed
bouncingSpeed
0 0,5. , β , render
, , dat.GUI, . :
function render() { ... cube.rotation.x += controls.rotationSpeed; cube.rotation.y += controls.rotationSpeed; cube.rotation.z += controls.rotationSpeed; step+=controls.bouncingSpeed; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... }
, , , :
.
ASCII
3D- . Three.js , , . , : ASCII . , -ASCII, . :
$("#WebGL-output").append(renderer.domElement);
:
var effect = new THREE.AsciiEffect( renderer ); effect.setSize(window.innerWidth, window.innerHeight ); $("#WebGL-output").append(effect.domElement);
. renderer.render(scene, camera), effect.render(scene,camera). :
.
, , , Three.js .
. , Three.js .
, . , Three.js.
, : GitHub
:
<script type="text/javascript" src="../libs/stats.js"></script>
,
<div id="Stats-output"></div>
, β , :
function initStats() { var stats = new Stats(); stats.setMode(0); stats.domElement.style.position = 'absolute'; stats.domElement.style.left = '0px'; stats.domElement.style.top = '0px'; $("#Stats-output").append(stats.domElement ); return stats; }
. setMode
(). 0, FPS, 1, . FPS, 0. jQuery :
$(function () { var stats = initStats(); β¦ }
render():
function render() { stats.update(); ... requestAnimationFrame(render); renderer.render(scene, camera); }
, , :

requestAnimationFrame
() . render
() , . :
function render() { ... cube.rotation.x += 0.02; cube.rotation.y += 0.02; cube.rotation.z += 0.02; ... requestAnimationFrame(render); renderer.render(scene, camera); }
, ? β rotation
0,02 render
(), . , , .
render
() :
var step=0; function render() { ... step+=0.04; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... requestAnimationFrame(render); renderer.render(scene, camera); }
rotation
; position
. , , . . Math.cos()
Math.sin()
step
. . , step+=0.04
. :
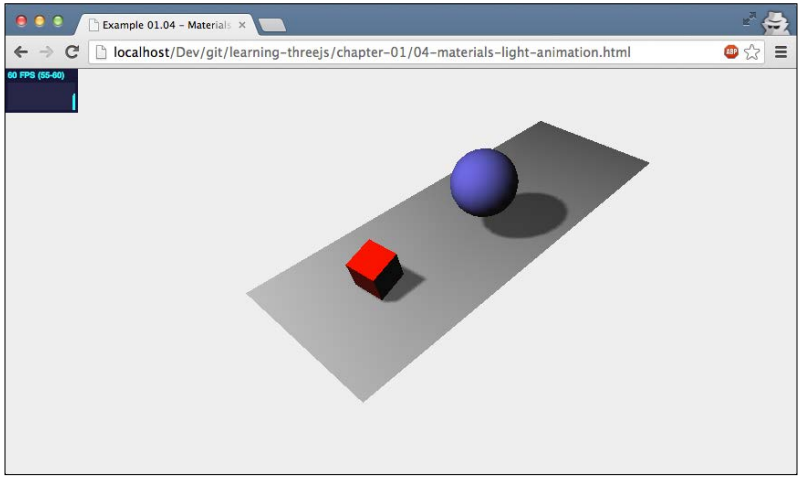
.
, . 3D-, , , . , , . , .
dat.GUI
Google dat.GUI( ), . , , :
, dat.GUI , :
<script type="text/javascript" src="../libs/dat.gui.js"></script>
β JavaScript , , , dat.GUI. JavaScript :
var controls = new function() { this.rotationSpeed = 0.02; this.bouncingSpeed = 0.03; }
: this.rotationSpeed
this.bouncingSpeed
. dat.GUI , :
var gui = new dat.GUI(); gui.add(controls, 'rotationSpeed',0,0.5); gui.add(controls, 'bouncingSpeed',0,0.5);
rotationSpeed
bouncingSpeed
0 0,5. , β , render
, , dat.GUI, . :
function render() { ... cube.rotation.x += controls.rotationSpeed; cube.rotation.y += controls.rotationSpeed; cube.rotation.z += controls.rotationSpeed; step+=controls.bouncingSpeed; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... }
, , , :

.
ASCII
3D- . Three.js , , . , : ASCII . , -ASCII, . :
$("#WebGL-output").append(renderer.domElement);
:
var effect = new THREE.AsciiEffect( renderer ); effect.setSize(window.innerWidth, window.innerHeight ); $("#WebGL-output").append(effect.domElement);
. renderer.render(scene, camera), effect.render(scene,camera). :
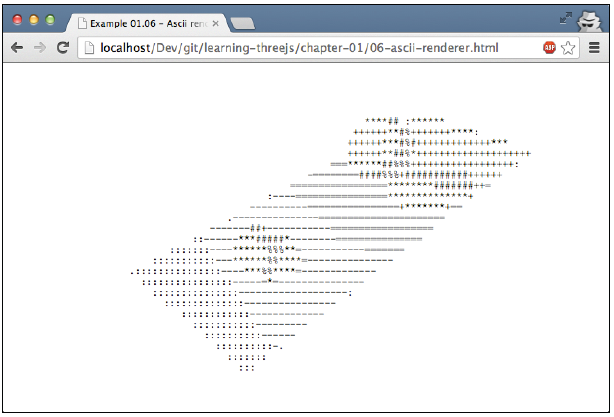
.
, , , Three.js .
. , Three.js .
, . , Three.js.
, : GitHub
:
<script type="text/javascript" src="../libs/stats.js"></script>
,
<div id="Stats-output"></div>
, β , :
function initStats() { var stats = new Stats(); stats.setMode(0); stats.domElement.style.position = 'absolute'; stats.domElement.style.left = '0px'; stats.domElement.style.top = '0px'; $("#Stats-output").append(stats.domElement ); return stats; }
. setMode
(). 0, FPS, 1, . FPS, 0. jQuery :
$(function () { var stats = initStats(); β¦ }
render():
function render() { stats.update(); ... requestAnimationFrame(render); renderer.render(scene, camera); }
, , :
requestAnimationFrame
() . render
() , . :
function render() { ... cube.rotation.x += 0.02; cube.rotation.y += 0.02; cube.rotation.z += 0.02; ... requestAnimationFrame(render); renderer.render(scene, camera); }
, ? β rotation
0,02 render
(), . , , .
render
() :
var step=0; function render() { ... step+=0.04; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... requestAnimationFrame(render); renderer.render(scene, camera); }
rotation
; position
. , , . . Math.cos()
Math.sin()
step
. . , step+=0.04
. :
.
, . 3D-, , , . , , . , .
dat.GUI
Google dat.GUI( ), . , , :
, dat.GUI , :
<script type="text/javascript" src="../libs/dat.gui.js"></script>
β JavaScript , , , dat.GUI. JavaScript :
var controls = new function() { this.rotationSpeed = 0.02; this.bouncingSpeed = 0.03; }
: this.rotationSpeed
this.bouncingSpeed
. dat.GUI , :
var gui = new dat.GUI(); gui.add(controls, 'rotationSpeed',0,0.5); gui.add(controls, 'bouncingSpeed',0,0.5);
rotationSpeed
bouncingSpeed
0 0,5. , β , render
, , dat.GUI, . :
function render() { ... cube.rotation.x += controls.rotationSpeed; cube.rotation.y += controls.rotationSpeed; cube.rotation.z += controls.rotationSpeed; step+=controls.bouncingSpeed; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... }
, , , :
.
ASCII
3D- . Three.js , , . , : ASCII . , -ASCII, . :
$("#WebGL-output").append(renderer.domElement);
:
var effect = new THREE.AsciiEffect( renderer ); effect.setSize(window.innerWidth, window.innerHeight ); $("#WebGL-output").append(effect.domElement);
. renderer.render(scene, camera), effect.render(scene,camera). :
.
, , , Three.js .
. , Three.js .
, . , Three.js.
, : GitHub
:
<script type="text/javascript" src="../libs/stats.js"></script>
,
<div id="Stats-output"></div>
, β , :
function initStats() { var stats = new Stats(); stats.setMode(0); stats.domElement.style.position = 'absolute'; stats.domElement.style.left = '0px'; stats.domElement.style.top = '0px'; $("#Stats-output").append(stats.domElement ); return stats; }
. setMode
(). 0, FPS, 1, . FPS, 0. jQuery :
$(function () { var stats = initStats(); β¦ }
render():
function render() { stats.update(); ... requestAnimationFrame(render); renderer.render(scene, camera); }
, , :

requestAnimationFrame
() . render
() , . :
function render() { ... cube.rotation.x += 0.02; cube.rotation.y += 0.02; cube.rotation.z += 0.02; ... requestAnimationFrame(render); renderer.render(scene, camera); }
, ? β rotation
0,02 render
(), . , , .
render
() :
var step=0; function render() { ... step+=0.04; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... requestAnimationFrame(render); renderer.render(scene, camera); }
rotation
; position
. , , . . Math.cos()
Math.sin()
step
. . , step+=0.04
. :
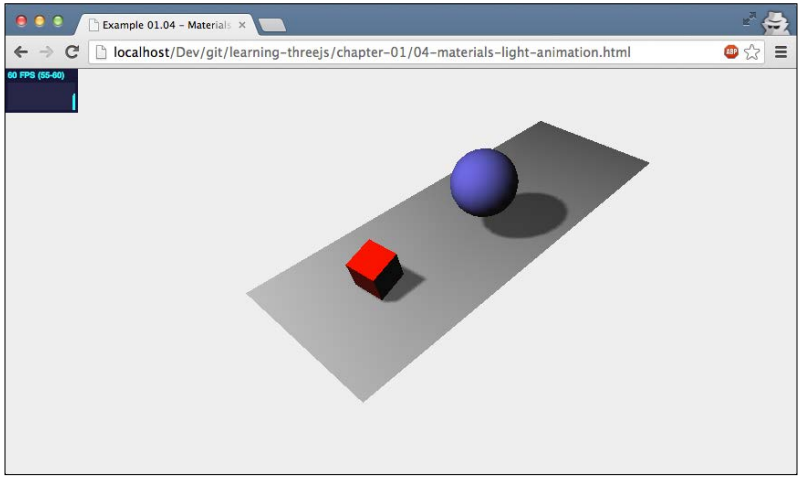
.
, . 3D-, , , . , , . , .
dat.GUI
Google dat.GUI( ), . , , :
, dat.GUI , :
<script type="text/javascript" src="../libs/dat.gui.js"></script>
β JavaScript , , , dat.GUI. JavaScript :
var controls = new function() { this.rotationSpeed = 0.02; this.bouncingSpeed = 0.03; }
: this.rotationSpeed
this.bouncingSpeed
. dat.GUI , :
var gui = new dat.GUI(); gui.add(controls, 'rotationSpeed',0,0.5); gui.add(controls, 'bouncingSpeed',0,0.5);
rotationSpeed
bouncingSpeed
0 0,5. , β , render
, , dat.GUI, . :
function render() { ... cube.rotation.x += controls.rotationSpeed; cube.rotation.y += controls.rotationSpeed; cube.rotation.z += controls.rotationSpeed; step+=controls.bouncingSpeed; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... }
, , , :

.
ASCII
3D- . Three.js , , . , : ASCII . , -ASCII, . :
$("#WebGL-output").append(renderer.domElement);
:
var effect = new THREE.AsciiEffect( renderer ); effect.setSize(window.innerWidth, window.innerHeight ); $("#WebGL-output").append(effect.domElement);
. renderer.render(scene, camera), effect.render(scene,camera). :
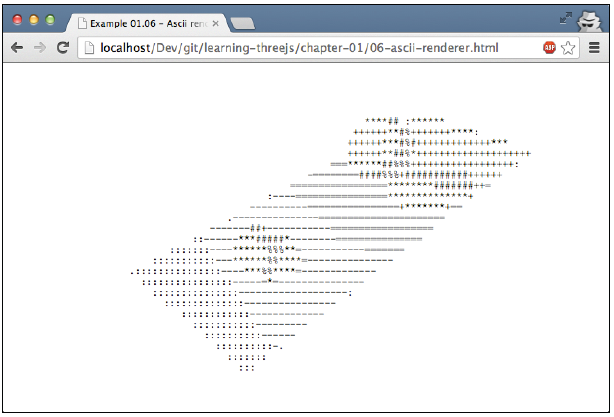
.
, , , Three.js .
. , Three.js .
, . , Three.js.
, : GitHub
:
<script type="text/javascript" src="../libs/stats.js"></script>
,
<div id="Stats-output"></div>
, β , :
function initStats() { var stats = new Stats(); stats.setMode(0); stats.domElement.style.position = 'absolute'; stats.domElement.style.left = '0px'; stats.domElement.style.top = '0px'; $("#Stats-output").append(stats.domElement ); return stats; }
. setMode
(). 0, FPS, 1, . FPS, 0. jQuery :
$(function () { var stats = initStats(); β¦ }
render():
function render() { stats.update(); ... requestAnimationFrame(render); renderer.render(scene, camera); }
, , :
requestAnimationFrame
() . render
() , . :
function render() { ... cube.rotation.x += 0.02; cube.rotation.y += 0.02; cube.rotation.z += 0.02; ... requestAnimationFrame(render); renderer.render(scene, camera); }
, ? β rotation
0,02 render
(), . , , .
render
() :
var step=0; function render() { ... step+=0.04; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... requestAnimationFrame(render); renderer.render(scene, camera); }
rotation
; position
. , , . . Math.cos()
Math.sin()
step
. . , step+=0.04
. :
.
, . 3D-, , , . , , . , .
dat.GUI
Google dat.GUI( ), . , , :
, dat.GUI , :
<script type="text/javascript" src="../libs/dat.gui.js"></script>
β JavaScript , , , dat.GUI. JavaScript :
var controls = new function() { this.rotationSpeed = 0.02; this.bouncingSpeed = 0.03; }
: this.rotationSpeed
this.bouncingSpeed
. dat.GUI , :
var gui = new dat.GUI(); gui.add(controls, 'rotationSpeed',0,0.5); gui.add(controls, 'bouncingSpeed',0,0.5);
rotationSpeed
bouncingSpeed
0 0,5. , β , render
, , dat.GUI, . :
function render() { ... cube.rotation.x += controls.rotationSpeed; cube.rotation.y += controls.rotationSpeed; cube.rotation.z += controls.rotationSpeed; step+=controls.bouncingSpeed; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... }
, , , :
.
ASCII
3D- . Three.js , , . , : ASCII . , -ASCII, . :
$("#WebGL-output").append(renderer.domElement);
:
var effect = new THREE.AsciiEffect( renderer ); effect.setSize(window.innerWidth, window.innerHeight ); $("#WebGL-output").append(effect.domElement);
. renderer.render(scene, camera), effect.render(scene,camera). :
.
, , , Three.js .
. , Three.js .
, . , Three.js.
, : GitHub
:
<script type="text/javascript" src="../libs/stats.js"></script>
,
<div id="Stats-output"></div>
, β , :
function initStats() { var stats = new Stats(); stats.setMode(0); stats.domElement.style.position = 'absolute'; stats.domElement.style.left = '0px'; stats.domElement.style.top = '0px'; $("#Stats-output").append(stats.domElement ); return stats; }
. setMode
(). 0, FPS, 1, . FPS, 0. jQuery :
$(function () { var stats = initStats(); β¦ }
render():
function render() { stats.update(); ... requestAnimationFrame(render); renderer.render(scene, camera); }
, , :

requestAnimationFrame
() . render
() , . :
function render() { ... cube.rotation.x += 0.02; cube.rotation.y += 0.02; cube.rotation.z += 0.02; ... requestAnimationFrame(render); renderer.render(scene, camera); }
, ? β rotation
0,02 render
(), . , , .
render
() :
var step=0; function render() { ... step+=0.04; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... requestAnimationFrame(render); renderer.render(scene, camera); }
rotation
; position
. , , . . Math.cos()
Math.sin()
step
. . , step+=0.04
. :
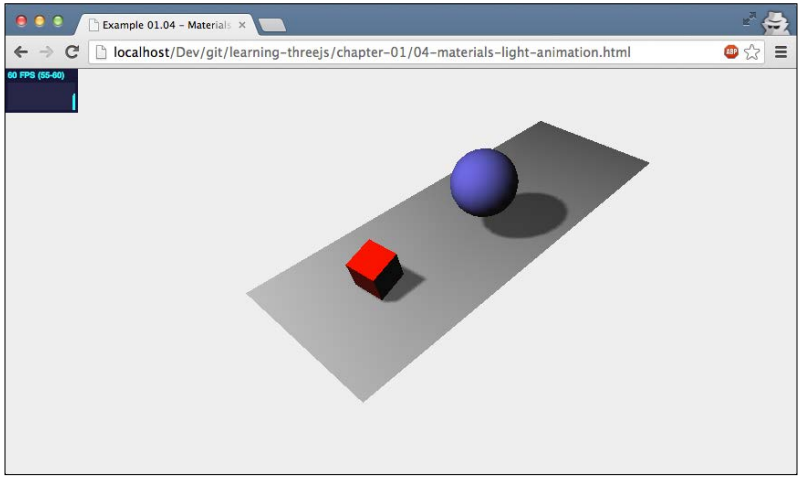
.
, . 3D-, , , . , , . , .
dat.GUI
Google dat.GUI( ), . , , :
, dat.GUI , :
<script type="text/javascript" src="../libs/dat.gui.js"></script>
β JavaScript , , , dat.GUI. JavaScript :
var controls = new function() { this.rotationSpeed = 0.02; this.bouncingSpeed = 0.03; }
: this.rotationSpeed
this.bouncingSpeed
. dat.GUI , :
var gui = new dat.GUI(); gui.add(controls, 'rotationSpeed',0,0.5); gui.add(controls, 'bouncingSpeed',0,0.5);
rotationSpeed
bouncingSpeed
0 0,5. , β , render
, , dat.GUI, . :
function render() { ... cube.rotation.x += controls.rotationSpeed; cube.rotation.y += controls.rotationSpeed; cube.rotation.z += controls.rotationSpeed; step+=controls.bouncingSpeed; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... }
, , , :

.
ASCII
3D- . Three.js , , . , : ASCII . , -ASCII, . :
$("#WebGL-output").append(renderer.domElement);
:
var effect = new THREE.AsciiEffect( renderer ); effect.setSize(window.innerWidth, window.innerHeight ); $("#WebGL-output").append(effect.domElement);
. renderer.render(scene, camera), effect.render(scene,camera). :
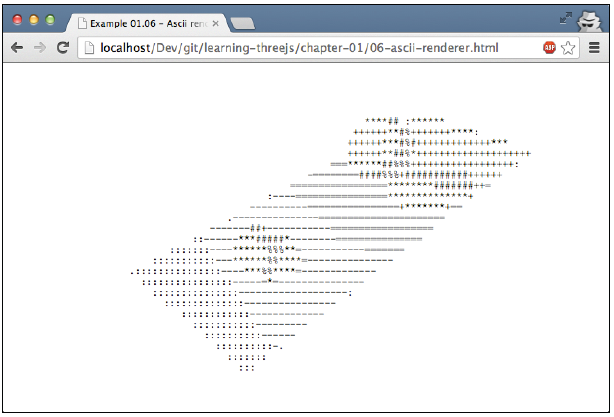
.
, , , Three.js .
. , Three.js .
, . , Three.js.
, : GitHub
:
<script type="text/javascript" src="../libs/stats.js"></script>
,
<div id="Stats-output"></div>
, β , :
function initStats() { var stats = new Stats(); stats.setMode(0); stats.domElement.style.position = 'absolute'; stats.domElement.style.left = '0px'; stats.domElement.style.top = '0px'; $("#Stats-output").append(stats.domElement ); return stats; }
. setMode
(). 0, FPS, 1, . FPS, 0. jQuery :
$(function () { var stats = initStats(); β¦ }
render():
function render() { stats.update(); ... requestAnimationFrame(render); renderer.render(scene, camera); }
, , :

requestAnimationFrame
() . render
() , . :
function render() { ... cube.rotation.x += 0.02; cube.rotation.y += 0.02; cube.rotation.z += 0.02; ... requestAnimationFrame(render); renderer.render(scene, camera); }
, ? β rotation
0,02 render
(), . , , .
render
() :
var step=0; function render() { ... step+=0.04; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... requestAnimationFrame(render); renderer.render(scene, camera); }
rotation
; position
. , , . . Math.cos()
Math.sin()
step
. . , step+=0.04
. :
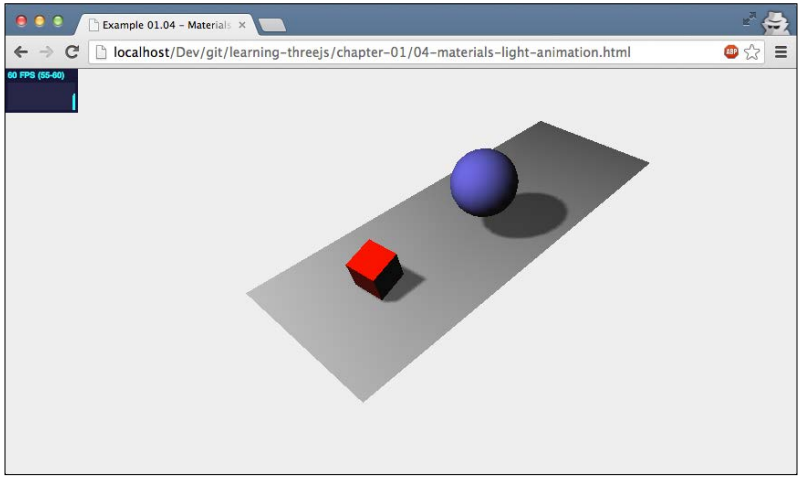
.
, . 3D-, , , . , , . , .
dat.GUI
Google dat.GUI( ), . , , :
, dat.GUI , :
<script type="text/javascript" src="../libs/dat.gui.js"></script>
β JavaScript , , , dat.GUI. JavaScript :
var controls = new function() { this.rotationSpeed = 0.02; this.bouncingSpeed = 0.03; }
: this.rotationSpeed
this.bouncingSpeed
. dat.GUI , :
var gui = new dat.GUI(); gui.add(controls, 'rotationSpeed',0,0.5); gui.add(controls, 'bouncingSpeed',0,0.5);
rotationSpeed
bouncingSpeed
0 0,5. , β , render
, , dat.GUI, . :
function render() { ... cube.rotation.x += controls.rotationSpeed; cube.rotation.y += controls.rotationSpeed; cube.rotation.z += controls.rotationSpeed; step+=controls.bouncingSpeed; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... }
, , , :

.
ASCII
3D- . Three.js , , . , : ASCII . , -ASCII, . :
$("#WebGL-output").append(renderer.domElement);
:
var effect = new THREE.AsciiEffect( renderer ); effect.setSize(window.innerWidth, window.innerHeight ); $("#WebGL-output").append(effect.domElement);
. renderer.render(scene, camera), effect.render(scene,camera). :
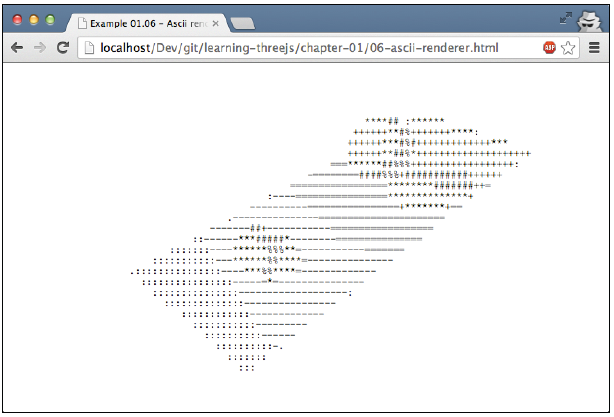
.
, , , Three.js .
. , Three.js .
, . , Three.js.
, : GitHub
:
<script type="text/javascript" src="../libs/stats.js"></script>
,
<div id="Stats-output"></div>
, β , :
function initStats() { var stats = new Stats(); stats.setMode(0); stats.domElement.style.position = 'absolute'; stats.domElement.style.left = '0px'; stats.domElement.style.top = '0px'; $("#Stats-output").append(stats.domElement ); return stats; }
. setMode
(). 0, FPS, 1, . FPS, 0. jQuery :
$(function () { var stats = initStats(); β¦ }
render():
function render() { stats.update(); ... requestAnimationFrame(render); renderer.render(scene, camera); }
, , :

requestAnimationFrame
() . render
() , . :
function render() { ... cube.rotation.x += 0.02; cube.rotation.y += 0.02; cube.rotation.z += 0.02; ... requestAnimationFrame(render); renderer.render(scene, camera); }
, ? β rotation
0,02 render
(), . , , .
render
() :
var step=0; function render() { ... step+=0.04; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... requestAnimationFrame(render); renderer.render(scene, camera); }
rotation
; position
. , , . . Math.cos()
Math.sin()
step
. . , step+=0.04
. :
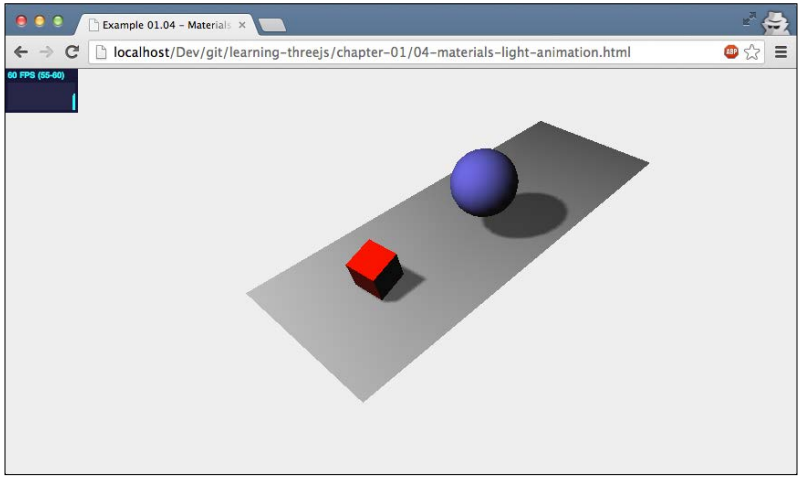
.
, . 3D-, , , . , , . , .
dat.GUI
Google dat.GUI( ), . , , :
, dat.GUI , :
<script type="text/javascript" src="../libs/dat.gui.js"></script>
β JavaScript , , , dat.GUI. JavaScript :
var controls = new function() { this.rotationSpeed = 0.02; this.bouncingSpeed = 0.03; }
: this.rotationSpeed
this.bouncingSpeed
. dat.GUI , :
var gui = new dat.GUI(); gui.add(controls, 'rotationSpeed',0,0.5); gui.add(controls, 'bouncingSpeed',0,0.5);
rotationSpeed
bouncingSpeed
0 0,5. , β , render
, , dat.GUI, . :
function render() { ... cube.rotation.x += controls.rotationSpeed; cube.rotation.y += controls.rotationSpeed; cube.rotation.z += controls.rotationSpeed; step+=controls.bouncingSpeed; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... }
, , , :

.
ASCII
3D- . Three.js , , . , : ASCII . , -ASCII, . :
$("#WebGL-output").append(renderer.domElement);
:
var effect = new THREE.AsciiEffect( renderer ); effect.setSize(window.innerWidth, window.innerHeight ); $("#WebGL-output").append(effect.domElement);
. renderer.render(scene, camera), effect.render(scene,camera). :
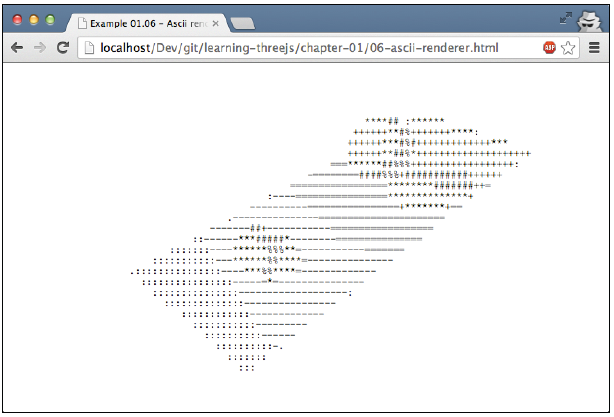
.
, , , Three.js .
. , Three.js .
, . , Three.js.
, : GitHub
:
<script type="text/javascript" src="../libs/stats.js"></script>
,
<div id="Stats-output"></div>
, β , :
function initStats() { var stats = new Stats(); stats.setMode(0); stats.domElement.style.position = 'absolute'; stats.domElement.style.left = '0px'; stats.domElement.style.top = '0px'; $("#Stats-output").append(stats.domElement ); return stats; }
. setMode
(). 0, FPS, 1, . FPS, 0. jQuery :
$(function () { var stats = initStats(); β¦ }
render():
function render() { stats.update(); ... requestAnimationFrame(render); renderer.render(scene, camera); }
, , :
requestAnimationFrame
() . render
() , . :
function render() { ... cube.rotation.x += 0.02; cube.rotation.y += 0.02; cube.rotation.z += 0.02; ... requestAnimationFrame(render); renderer.render(scene, camera); }
, ? β rotation
0,02 render
(), . , , .
render
() :
var step=0; function render() { ... step+=0.04; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... requestAnimationFrame(render); renderer.render(scene, camera); }
rotation
; position
. , , . . Math.cos()
Math.sin()
step
. . , step+=0.04
. :
.
, . 3D-, , , . , , . , .
dat.GUI
Google dat.GUI( ), . , , :
, dat.GUI , :
<script type="text/javascript" src="../libs/dat.gui.js"></script>
β JavaScript , , , dat.GUI. JavaScript :
var controls = new function() { this.rotationSpeed = 0.02; this.bouncingSpeed = 0.03; }
: this.rotationSpeed
this.bouncingSpeed
. dat.GUI , :
var gui = new dat.GUI(); gui.add(controls, 'rotationSpeed',0,0.5); gui.add(controls, 'bouncingSpeed',0,0.5);
rotationSpeed
bouncingSpeed
0 0,5. , β , render
, , dat.GUI, . :
function render() { ... cube.rotation.x += controls.rotationSpeed; cube.rotation.y += controls.rotationSpeed; cube.rotation.z += controls.rotationSpeed; step+=controls.bouncingSpeed; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... }
, , , :
.
ASCII
3D- . Three.js , , . , : ASCII . , -ASCII, . :
$("#WebGL-output").append(renderer.domElement);
:
var effect = new THREE.AsciiEffect( renderer ); effect.setSize(window.innerWidth, window.innerHeight ); $("#WebGL-output").append(effect.domElement);
. renderer.render(scene, camera), effect.render(scene,camera). :
.
, , , Three.js .
. , Three.js .
, . , Three.js.
, : GitHub
:
<script type="text/javascript" src="../libs/stats.js"></script>
,
<div id="Stats-output"></div>
, β , :
function initStats() { var stats = new Stats(); stats.setMode(0); stats.domElement.style.position = 'absolute'; stats.domElement.style.left = '0px'; stats.domElement.style.top = '0px'; $("#Stats-output").append(stats.domElement ); return stats; }
. setMode
(). 0, FPS, 1, . FPS, 0. jQuery :
$(function () { var stats = initStats(); β¦ }
render():
function render() { stats.update(); ... requestAnimationFrame(render); renderer.render(scene, camera); }
, , :

requestAnimationFrame
() . render
() , . :
function render() { ... cube.rotation.x += 0.02; cube.rotation.y += 0.02; cube.rotation.z += 0.02; ... requestAnimationFrame(render); renderer.render(scene, camera); }
, ? β rotation
0,02 render
(), . , , .
render
() :
var step=0; function render() { ... step+=0.04; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... requestAnimationFrame(render); renderer.render(scene, camera); }
rotation
; position
. , , . . Math.cos()
Math.sin()
step
. . , step+=0.04
. :
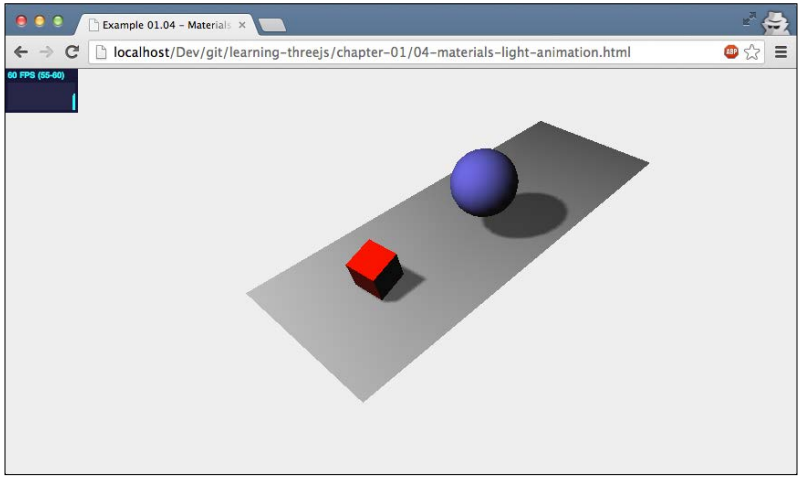
.
, . 3D-, , , . , , . , .
dat.GUI
Google dat.GUI( ), . , , :
, dat.GUI , :
<script type="text/javascript" src="../libs/dat.gui.js"></script>
β JavaScript , , , dat.GUI. JavaScript :
var controls = new function() { this.rotationSpeed = 0.02; this.bouncingSpeed = 0.03; }
: this.rotationSpeed
this.bouncingSpeed
. dat.GUI , :
var gui = new dat.GUI(); gui.add(controls, 'rotationSpeed',0,0.5); gui.add(controls, 'bouncingSpeed',0,0.5);
rotationSpeed
bouncingSpeed
0 0,5. , β , render
, , dat.GUI, . :
function render() { ... cube.rotation.x += controls.rotationSpeed; cube.rotation.y += controls.rotationSpeed; cube.rotation.z += controls.rotationSpeed; step+=controls.bouncingSpeed; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... }
, , , :

.
ASCII
3D- . Three.js , , . , : ASCII . , -ASCII, . :
$("#WebGL-output").append(renderer.domElement);
:
var effect = new THREE.AsciiEffect( renderer ); effect.setSize(window.innerWidth, window.innerHeight ); $("#WebGL-output").append(effect.domElement);
. renderer.render(scene, camera), effect.render(scene,camera). :
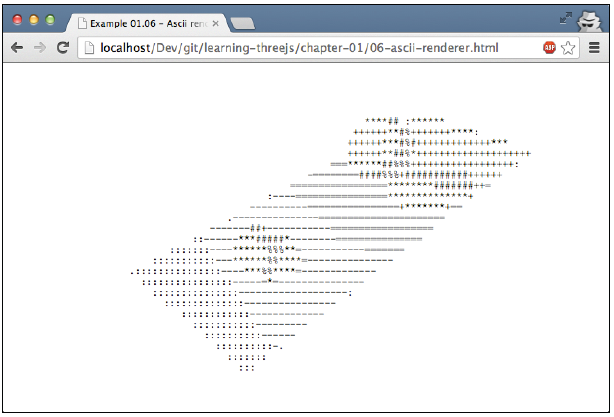
.
, , , Three.js .
. , Three.js .
, . , Three.js.
, : GitHub
:
<script type="text/javascript" src="../libs/stats.js"></script>
,
<div id="Stats-output"></div>
, β , :
function initStats() { var stats = new Stats(); stats.setMode(0); stats.domElement.style.position = 'absolute'; stats.domElement.style.left = '0px'; stats.domElement.style.top = '0px'; $("#Stats-output").append(stats.domElement ); return stats; }
. setMode
(). 0, FPS, 1, . FPS, 0. jQuery :
$(function () { var stats = initStats(); β¦ }
render():
function render() { stats.update(); ... requestAnimationFrame(render); renderer.render(scene, camera); }
, , :

requestAnimationFrame
() . render
() , . :
function render() { ... cube.rotation.x += 0.02; cube.rotation.y += 0.02; cube.rotation.z += 0.02; ... requestAnimationFrame(render); renderer.render(scene, camera); }
, ? β rotation
0,02 render
(), . , , .
render
() :
var step=0; function render() { ... step+=0.04; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... requestAnimationFrame(render); renderer.render(scene, camera); }
rotation
; position
. , , . . Math.cos()
Math.sin()
step
. . , step+=0.04
. :
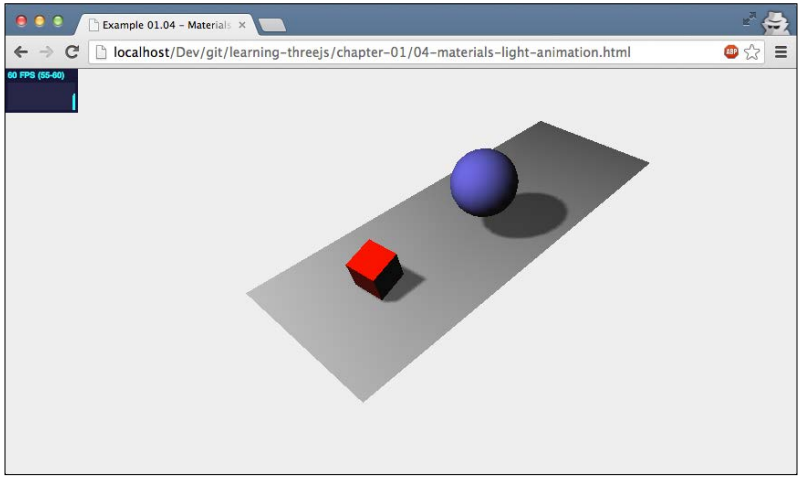
.
, . 3D-, , , . , , . , .
dat.GUI
Google dat.GUI( ), . , , :
, dat.GUI , :
<script type="text/javascript" src="../libs/dat.gui.js"></script>
β JavaScript , , , dat.GUI. JavaScript :
var controls = new function() { this.rotationSpeed = 0.02; this.bouncingSpeed = 0.03; }
: this.rotationSpeed
this.bouncingSpeed
. dat.GUI , :
var gui = new dat.GUI(); gui.add(controls, 'rotationSpeed',0,0.5); gui.add(controls, 'bouncingSpeed',0,0.5);
rotationSpeed
bouncingSpeed
0 0,5. , β , render
, , dat.GUI, . :
function render() { ... cube.rotation.x += controls.rotationSpeed; cube.rotation.y += controls.rotationSpeed; cube.rotation.z += controls.rotationSpeed; step+=controls.bouncingSpeed; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... }
, , , :

.
ASCII
3D- . Three.js , , . , : ASCII . , -ASCII, . :
$("#WebGL-output").append(renderer.domElement);
:
var effect = new THREE.AsciiEffect( renderer ); effect.setSize(window.innerWidth, window.innerHeight ); $("#WebGL-output").append(effect.domElement);
. renderer.render(scene, camera), effect.render(scene,camera). :
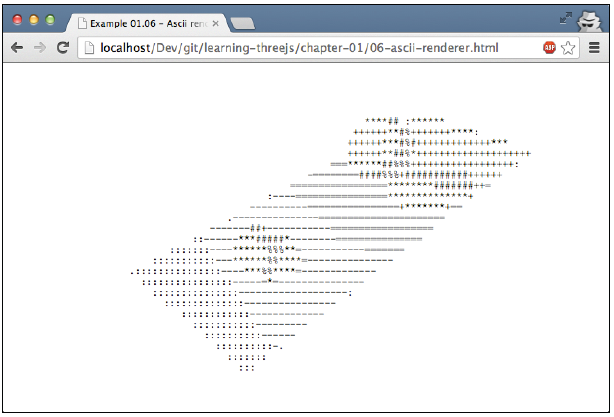
.
, , , Three.js .
. , Three.js .
, . , Three.js.
, : GitHub
:
<script type="text/javascript" src="../libs/stats.js"></script>
,
<div id="Stats-output"></div>
, β , :
function initStats() { var stats = new Stats(); stats.setMode(0); stats.domElement.style.position = 'absolute'; stats.domElement.style.left = '0px'; stats.domElement.style.top = '0px'; $("#Stats-output").append(stats.domElement ); return stats; }
. setMode
(). 0, FPS, 1, . FPS, 0. jQuery :
$(function () { var stats = initStats(); β¦ }
render():
function render() { stats.update(); ... requestAnimationFrame(render); renderer.render(scene, camera); }
, , :

requestAnimationFrame
() . render
() , . :
function render() { ... cube.rotation.x += 0.02; cube.rotation.y += 0.02; cube.rotation.z += 0.02; ... requestAnimationFrame(render); renderer.render(scene, camera); }
, ? β rotation
0,02 render
(), . , , .
render
() :
var step=0; function render() { ... step+=0.04; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... requestAnimationFrame(render); renderer.render(scene, camera); }
rotation
; position
. , , . . Math.cos()
Math.sin()
step
. . , step+=0.04
. :
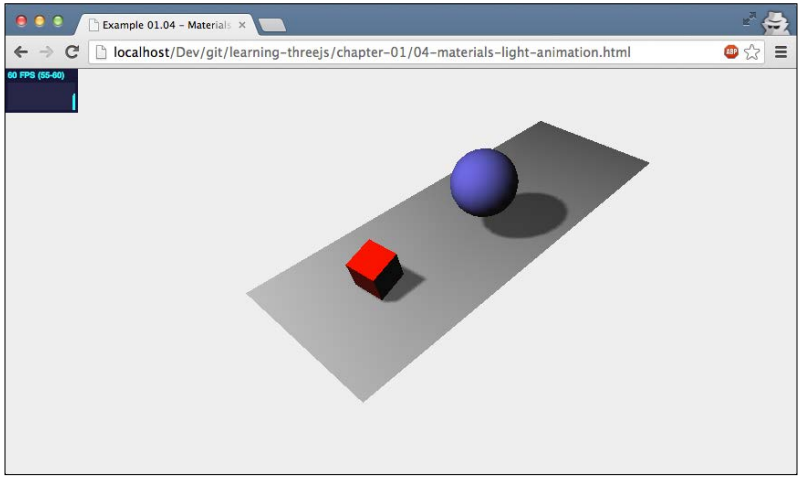
.
, . 3D-, , , . , , . , .
dat.GUI
Google dat.GUI( ), . , , :
, dat.GUI , :
<script type="text/javascript" src="../libs/dat.gui.js"></script>
β JavaScript , , , dat.GUI. JavaScript :
var controls = new function() { this.rotationSpeed = 0.02; this.bouncingSpeed = 0.03; }
: this.rotationSpeed
this.bouncingSpeed
. dat.GUI , :
var gui = new dat.GUI(); gui.add(controls, 'rotationSpeed',0,0.5); gui.add(controls, 'bouncingSpeed',0,0.5);
rotationSpeed
bouncingSpeed
0 0,5. , β , render
, , dat.GUI, . :
function render() { ... cube.rotation.x += controls.rotationSpeed; cube.rotation.y += controls.rotationSpeed; cube.rotation.z += controls.rotationSpeed; step+=controls.bouncingSpeed; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... }
, , , :

.
ASCII
3D- . Three.js , , . , : ASCII . , -ASCII, . :
$("#WebGL-output").append(renderer.domElement);
:
var effect = new THREE.AsciiEffect( renderer ); effect.setSize(window.innerWidth, window.innerHeight ); $("#WebGL-output").append(effect.domElement);
. renderer.render(scene, camera), effect.render(scene,camera). :
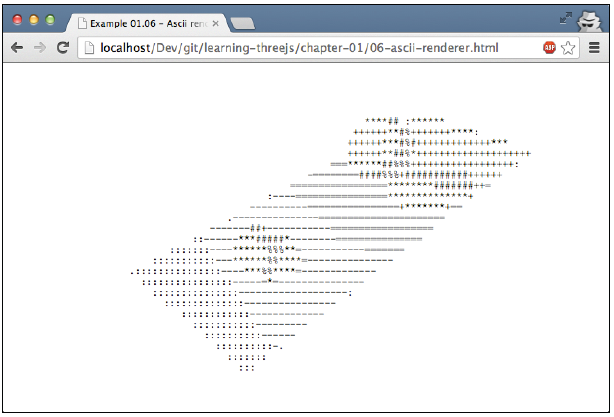
.
, , , Three.js .
. , Three.js .
, . , Three.js.
, : GitHub
:
<script type="text/javascript" src="../libs/stats.js"></script>
,
<div id="Stats-output"></div>
, β , :
function initStats() { var stats = new Stats(); stats.setMode(0); stats.domElement.style.position = 'absolute'; stats.domElement.style.left = '0px'; stats.domElement.style.top = '0px'; $("#Stats-output").append(stats.domElement ); return stats; }
. setMode
(). 0, FPS, 1, . FPS, 0. jQuery :
$(function () { var stats = initStats(); β¦ }
render():
function render() { stats.update(); ... requestAnimationFrame(render); renderer.render(scene, camera); }
, , :
requestAnimationFrame
() . render
() , . :
function render() { ... cube.rotation.x += 0.02; cube.rotation.y += 0.02; cube.rotation.z += 0.02; ... requestAnimationFrame(render); renderer.render(scene, camera); }
, ? β rotation
0,02 render
(), . , , .
render
() :
var step=0; function render() { ... step+=0.04; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... requestAnimationFrame(render); renderer.render(scene, camera); }
rotation
; position
. , , . . Math.cos()
Math.sin()
step
. . , step+=0.04
. :
.
, . 3D-, , , . , , . , .
dat.GUI
Google dat.GUI( ), . , , :
, dat.GUI , :
<script type="text/javascript" src="../libs/dat.gui.js"></script>
β JavaScript , , , dat.GUI. JavaScript :
var controls = new function() { this.rotationSpeed = 0.02; this.bouncingSpeed = 0.03; }
: this.rotationSpeed
this.bouncingSpeed
. dat.GUI , :
var gui = new dat.GUI(); gui.add(controls, 'rotationSpeed',0,0.5); gui.add(controls, 'bouncingSpeed',0,0.5);
rotationSpeed
bouncingSpeed
0 0,5. , β , render
, , dat.GUI, . :
function render() { ... cube.rotation.x += controls.rotationSpeed; cube.rotation.y += controls.rotationSpeed; cube.rotation.z += controls.rotationSpeed; step+=controls.bouncingSpeed; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... }
, , , :
.
ASCII
3D- . Three.js , , . , : ASCII . , -ASCII, . :
$("#WebGL-output").append(renderer.domElement);
:
var effect = new THREE.AsciiEffect( renderer ); effect.setSize(window.innerWidth, window.innerHeight ); $("#WebGL-output").append(effect.domElement);
. renderer.render(scene, camera), effect.render(scene,camera). :
.
, , , Three.js .
. , Three.js .
, . , Three.js.
, : GitHub
:
<script type="text/javascript" src="../libs/stats.js"></script>
,
<div id="Stats-output"></div>
, β , :
function initStats() { var stats = new Stats(); stats.setMode(0); stats.domElement.style.position = 'absolute'; stats.domElement.style.left = '0px'; stats.domElement.style.top = '0px'; $("#Stats-output").append(stats.domElement ); return stats; }
. setMode
(). 0, FPS, 1, . FPS, 0. jQuery :
$(function () { var stats = initStats(); β¦ }
render():
function render() { stats.update(); ... requestAnimationFrame(render); renderer.render(scene, camera); }
, , :

requestAnimationFrame
() . render
() , . :
function render() { ... cube.rotation.x += 0.02; cube.rotation.y += 0.02; cube.rotation.z += 0.02; ... requestAnimationFrame(render); renderer.render(scene, camera); }
, ? β rotation
0,02 render
(), . , , .
render
() :
var step=0; function render() { ... step+=0.04; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... requestAnimationFrame(render); renderer.render(scene, camera); }
rotation
; position
. , , . . Math.cos()
Math.sin()
step
. . , step+=0.04
. :
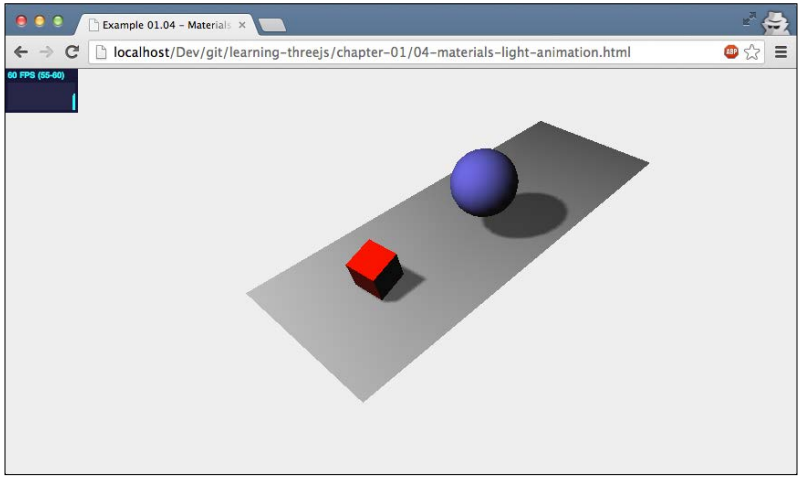
.
, . 3D-, , , . , , . , .
dat.GUI
Google dat.GUI( ), . , , :
, dat.GUI , :
<script type="text/javascript" src="../libs/dat.gui.js"></script>
β JavaScript , , , dat.GUI. JavaScript :
var controls = new function() { this.rotationSpeed = 0.02; this.bouncingSpeed = 0.03; }
: this.rotationSpeed
this.bouncingSpeed
. dat.GUI , :
var gui = new dat.GUI(); gui.add(controls, 'rotationSpeed',0,0.5); gui.add(controls, 'bouncingSpeed',0,0.5);
rotationSpeed
bouncingSpeed
0 0,5. , β , render
, , dat.GUI, . :
function render() { ... cube.rotation.x += controls.rotationSpeed; cube.rotation.y += controls.rotationSpeed; cube.rotation.z += controls.rotationSpeed; step+=controls.bouncingSpeed; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... }
, , , :

.
ASCII
3D- . Three.js , , . , : ASCII . , -ASCII, . :
$("#WebGL-output").append(renderer.domElement);
:
var effect = new THREE.AsciiEffect( renderer ); effect.setSize(window.innerWidth, window.innerHeight ); $("#WebGL-output").append(effect.domElement);
. renderer.render(scene, camera), effect.render(scene,camera). :
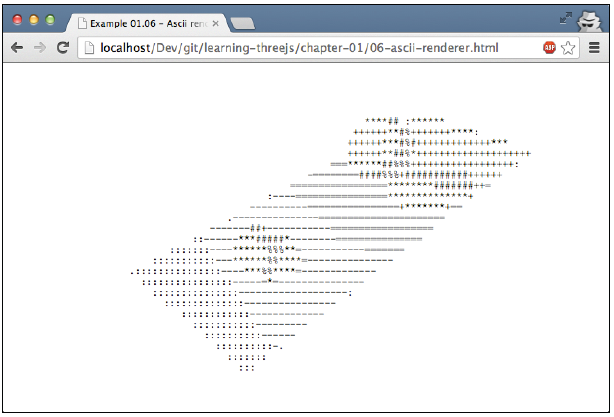
.
, , , Three.js .
. , Three.js .
, . , Three.js.
, : GitHub
:
<script type="text/javascript" src="../libs/stats.js"></script>
,
<div id="Stats-output"></div>
, β , :
function initStats() { var stats = new Stats(); stats.setMode(0); stats.domElement.style.position = 'absolute'; stats.domElement.style.left = '0px'; stats.domElement.style.top = '0px'; $("#Stats-output").append(stats.domElement ); return stats; }
. setMode
(). 0, FPS, 1, . FPS, 0. jQuery :
$(function () { var stats = initStats(); β¦ }
render():
function render() { stats.update(); ... requestAnimationFrame(render); renderer.render(scene, camera); }
, , :

requestAnimationFrame
() . render
() , . :
function render() { ... cube.rotation.x += 0.02; cube.rotation.y += 0.02; cube.rotation.z += 0.02; ... requestAnimationFrame(render); renderer.render(scene, camera); }
, ? β rotation
0,02 render
(), . , , .
render
() :
var step=0; function render() { ... step+=0.04; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... requestAnimationFrame(render); renderer.render(scene, camera); }
rotation
; position
. , , . . Math.cos()
Math.sin()
step
. . , step+=0.04
. :
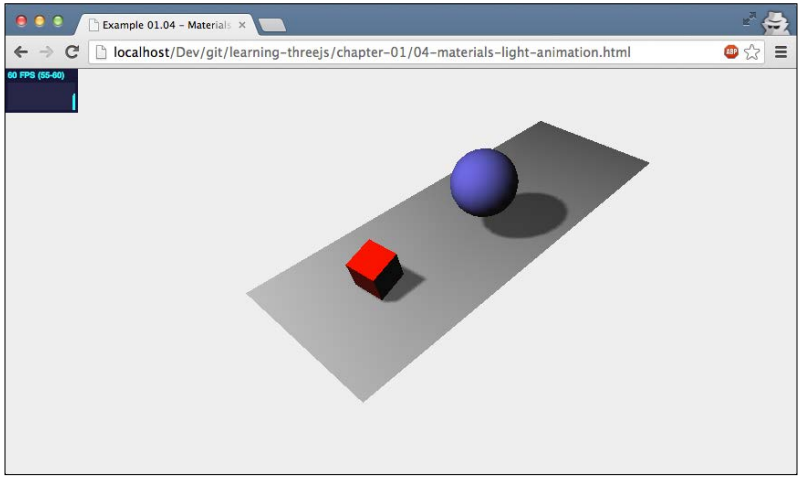
.
, . 3D-, , , . , , . , .
dat.GUI
Google dat.GUI( ), . , , :
, dat.GUI , :
<script type="text/javascript" src="../libs/dat.gui.js"></script>
β JavaScript , , , dat.GUI. JavaScript :
var controls = new function() { this.rotationSpeed = 0.02; this.bouncingSpeed = 0.03; }
: this.rotationSpeed
this.bouncingSpeed
. dat.GUI , :
var gui = new dat.GUI(); gui.add(controls, 'rotationSpeed',0,0.5); gui.add(controls, 'bouncingSpeed',0,0.5);
rotationSpeed
bouncingSpeed
0 0,5. , β , render
, , dat.GUI, . :
function render() { ... cube.rotation.x += controls.rotationSpeed; cube.rotation.y += controls.rotationSpeed; cube.rotation.z += controls.rotationSpeed; step+=controls.bouncingSpeed; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... }
, , , :

.
ASCII
3D- . Three.js , , . , : ASCII . , -ASCII, . :
$("#WebGL-output").append(renderer.domElement);
:
var effect = new THREE.AsciiEffect( renderer ); effect.setSize(window.innerWidth, window.innerHeight ); $("#WebGL-output").append(effect.domElement);
. renderer.render(scene, camera), effect.render(scene,camera). :
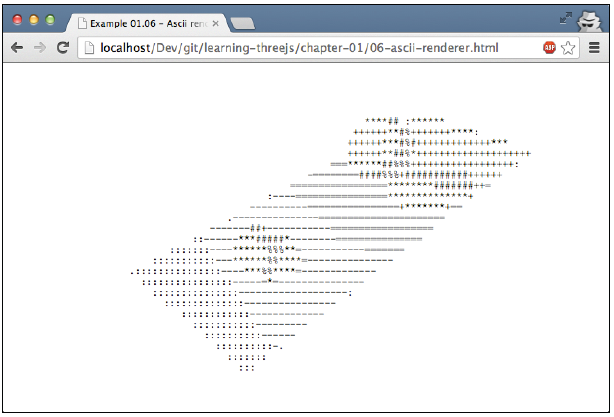
.
, , , Three.js .
. , Three.js .
, . , Three.js.
, : GitHub
:
<script type="text/javascript" src="../libs/stats.js"></script>
,
<div id="Stats-output"></div>
, β , :
function initStats() { var stats = new Stats(); stats.setMode(0); stats.domElement.style.position = 'absolute'; stats.domElement.style.left = '0px'; stats.domElement.style.top = '0px'; $("#Stats-output").append(stats.domElement ); return stats; }
. setMode
(). 0, FPS, 1, . FPS, 0. jQuery :
$(function () { var stats = initStats(); β¦ }
render():
function render() { stats.update(); ... requestAnimationFrame(render); renderer.render(scene, camera); }
, , :

requestAnimationFrame
() . render
() , . :
function render() { ... cube.rotation.x += 0.02; cube.rotation.y += 0.02; cube.rotation.z += 0.02; ... requestAnimationFrame(render); renderer.render(scene, camera); }
, ? β rotation
0,02 render
(), . , , .
render
() :
var step=0; function render() { ... step+=0.04; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... requestAnimationFrame(render); renderer.render(scene, camera); }
rotation
; position
. , , . . Math.cos()
Math.sin()
step
. . , step+=0.04
. :
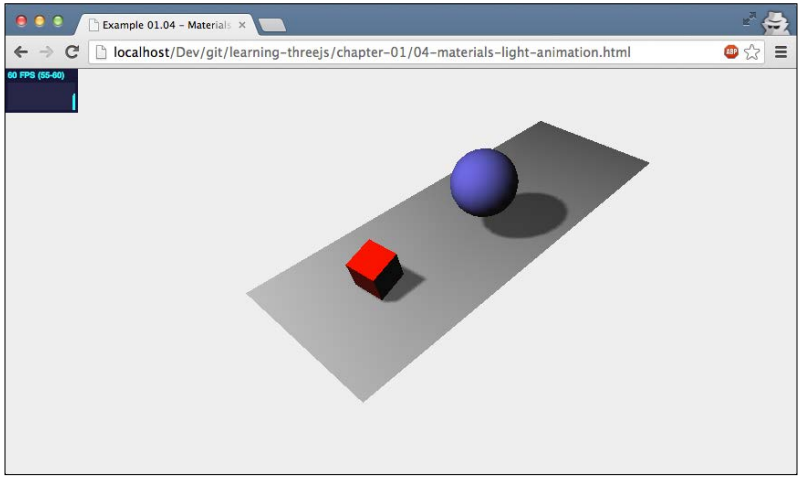
.
, . 3D-, , , . , , . , .
dat.GUI
Google dat.GUI( ), . , , :
, dat.GUI , :
<script type="text/javascript" src="../libs/dat.gui.js"></script>
β JavaScript , , , dat.GUI. JavaScript :
var controls = new function() { this.rotationSpeed = 0.02; this.bouncingSpeed = 0.03; }
: this.rotationSpeed
this.bouncingSpeed
. dat.GUI , :
var gui = new dat.GUI(); gui.add(controls, 'rotationSpeed',0,0.5); gui.add(controls, 'bouncingSpeed',0,0.5);
rotationSpeed
bouncingSpeed
0 0,5. , β , render
, , dat.GUI, . :
function render() { ... cube.rotation.x += controls.rotationSpeed; cube.rotation.y += controls.rotationSpeed; cube.rotation.z += controls.rotationSpeed; step+=controls.bouncingSpeed; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... }
, , , :

.
ASCII
3D- . Three.js , , . , : ASCII . , -ASCII, . :
$("#WebGL-output").append(renderer.domElement);
:
var effect = new THREE.AsciiEffect( renderer ); effect.setSize(window.innerWidth, window.innerHeight ); $("#WebGL-output").append(effect.domElement);
. renderer.render(scene, camera), effect.render(scene,camera). :
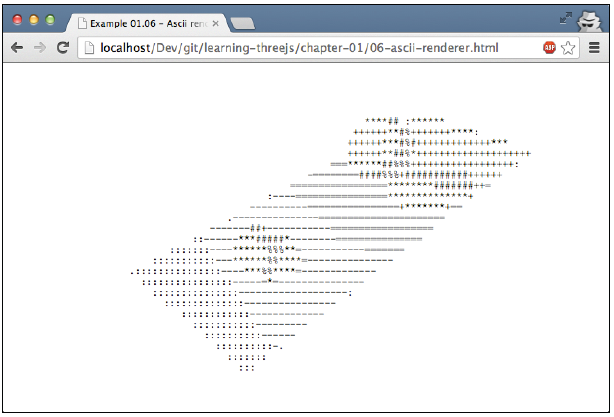
.
, , , Three.js .
. , Three.js .
, . , Three.js.
, : GitHub
:
<script type="text/javascript" src="../libs/stats.js"></script>
,
<div id="Stats-output"></div>
, β , :
function initStats() { var stats = new Stats(); stats.setMode(0); stats.domElement.style.position = 'absolute'; stats.domElement.style.left = '0px'; stats.domElement.style.top = '0px'; $("#Stats-output").append(stats.domElement ); return stats; }
. setMode
(). 0, FPS, 1, . FPS, 0. jQuery :
$(function () { var stats = initStats(); β¦ }
render():
function render() { stats.update(); ... requestAnimationFrame(render); renderer.render(scene, camera); }
, , :

requestAnimationFrame
() . render
() , . :
function render() { ... cube.rotation.x += 0.02; cube.rotation.y += 0.02; cube.rotation.z += 0.02; ... requestAnimationFrame(render); renderer.render(scene, camera); }
, ? β rotation
0,02 render
(), . , , .
render
() :
var step=0; function render() { ... step+=0.04; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... requestAnimationFrame(render); renderer.render(scene, camera); }
rotation
; position
. , , . . Math.cos()
Math.sin()
step
. . , step+=0.04
. :
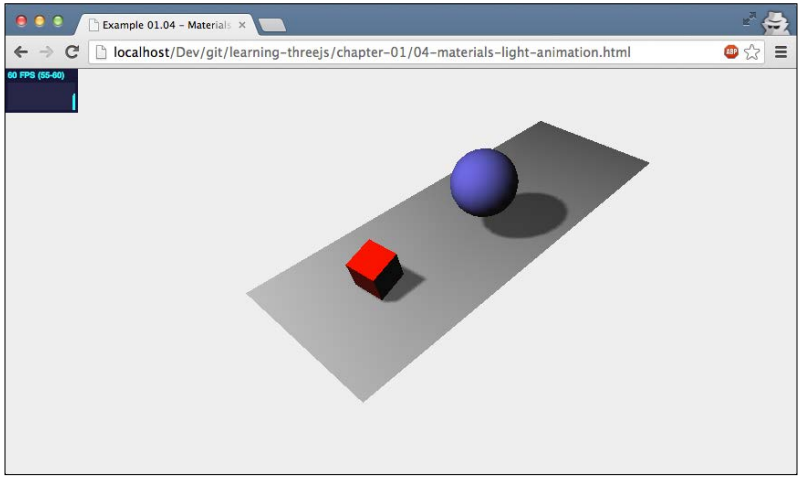
.
, . 3D-, , , . , , . , .
dat.GUI
Google dat.GUI( ), . , , :
, dat.GUI , :
<script type="text/javascript" src="../libs/dat.gui.js"></script>
β JavaScript , , , dat.GUI. JavaScript :
var controls = new function() { this.rotationSpeed = 0.02; this.bouncingSpeed = 0.03; }
: this.rotationSpeed
this.bouncingSpeed
. dat.GUI , :
var gui = new dat.GUI(); gui.add(controls, 'rotationSpeed',0,0.5); gui.add(controls, 'bouncingSpeed',0,0.5);
rotationSpeed
bouncingSpeed
0 0,5. , β , render
, , dat.GUI, . :
function render() { ... cube.rotation.x += controls.rotationSpeed; cube.rotation.y += controls.rotationSpeed; cube.rotation.z += controls.rotationSpeed; step+=controls.bouncingSpeed; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... }
, , , :

.
ASCII
3D- . Three.js , , . , : ASCII . , -ASCII, . :
$("#WebGL-output").append(renderer.domElement);
:
var effect = new THREE.AsciiEffect( renderer ); effect.setSize(window.innerWidth, window.innerHeight ); $("#WebGL-output").append(effect.domElement);
. renderer.render(scene, camera), effect.render(scene,camera). :
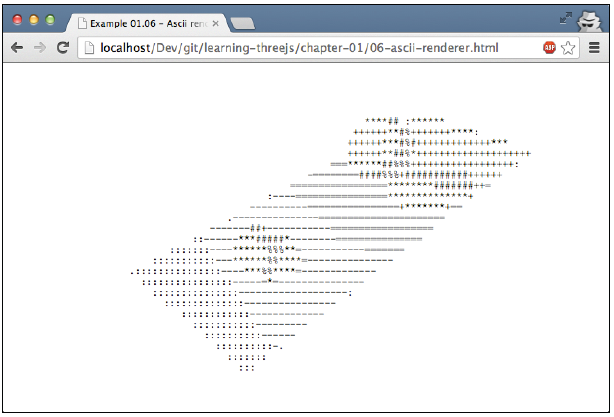
.
, , , Three.js .
. , Three.js .
, . , Three.js.
, : GitHub
:
<script type="text/javascript" src="../libs/stats.js"></script>
,
<div id="Stats-output"></div>
, β , :
function initStats() { var stats = new Stats(); stats.setMode(0); stats.domElement.style.position = 'absolute'; stats.domElement.style.left = '0px'; stats.domElement.style.top = '0px'; $("#Stats-output").append(stats.domElement ); return stats; }
. setMode
(). 0, FPS, 1, . FPS, 0. jQuery :
$(function () { var stats = initStats(); β¦ }
render():
function render() { stats.update(); ... requestAnimationFrame(render); renderer.render(scene, camera); }
, , :

requestAnimationFrame
() . render
() , . :
function render() { ... cube.rotation.x += 0.02; cube.rotation.y += 0.02; cube.rotation.z += 0.02; ... requestAnimationFrame(render); renderer.render(scene, camera); }
, ? β rotation
0,02 render
(), . , , .
render
() :
var step=0; function render() { ... step+=0.04; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... requestAnimationFrame(render); renderer.render(scene, camera); }
rotation
; position
. , , . . Math.cos()
Math.sin()
step
. . , step+=0.04
. :
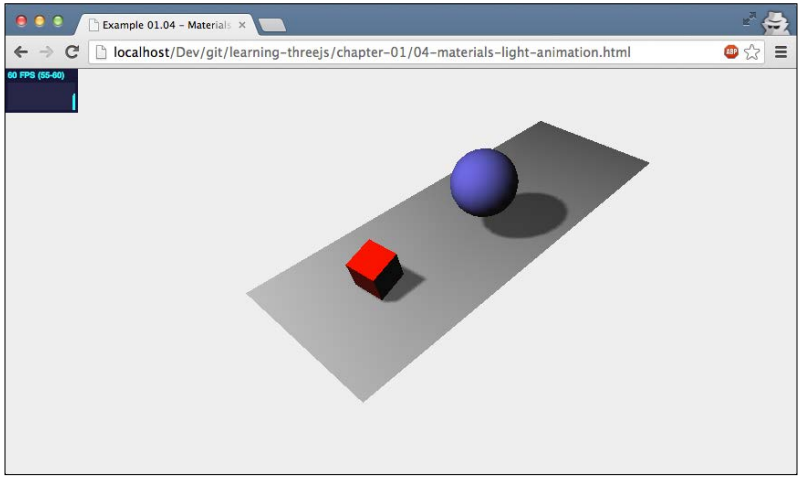
.
, . 3D-, , , . , , . , .
dat.GUI
Google dat.GUI( ), . , , :
, dat.GUI , :
<script type="text/javascript" src="../libs/dat.gui.js"></script>
β JavaScript , , , dat.GUI. JavaScript :
var controls = new function() { this.rotationSpeed = 0.02; this.bouncingSpeed = 0.03; }
: this.rotationSpeed
this.bouncingSpeed
. dat.GUI , :
var gui = new dat.GUI(); gui.add(controls, 'rotationSpeed',0,0.5); gui.add(controls, 'bouncingSpeed',0,0.5);
rotationSpeed
bouncingSpeed
0 0,5. , β , render
, , dat.GUI, . :
function render() { ... cube.rotation.x += controls.rotationSpeed; cube.rotation.y += controls.rotationSpeed; cube.rotation.z += controls.rotationSpeed; step+=controls.bouncingSpeed; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... }
, , , :

.
ASCII
3D- . Three.js , , . , : ASCII . , -ASCII, . :
$("#WebGL-output").append(renderer.domElement);
:
var effect = new THREE.AsciiEffect( renderer ); effect.setSize(window.innerWidth, window.innerHeight ); $("#WebGL-output").append(effect.domElement);
. renderer.render(scene, camera), effect.render(scene,camera). :
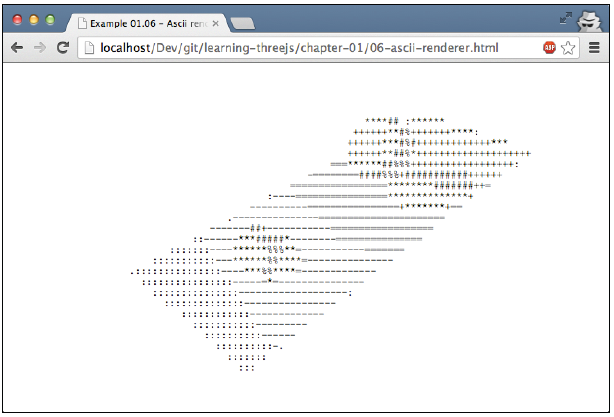
.
, , , Three.js .
. , Three.js .
, . , Three.js.
, : GitHub
:
<script type="text/javascript" src="../libs/stats.js"></script>
,
<div id="Stats-output"></div>
, β , :
function initStats() { var stats = new Stats(); stats.setMode(0); stats.domElement.style.position = 'absolute'; stats.domElement.style.left = '0px'; stats.domElement.style.top = '0px'; $("#Stats-output").append(stats.domElement ); return stats; }
. setMode
(). 0, FPS, 1, . FPS, 0. jQuery :
$(function () { var stats = initStats(); β¦ }
render():
function render() { stats.update(); ... requestAnimationFrame(render); renderer.render(scene, camera); }
, , :

requestAnimationFrame
() . render
() , . :
function render() { ... cube.rotation.x += 0.02; cube.rotation.y += 0.02; cube.rotation.z += 0.02; ... requestAnimationFrame(render); renderer.render(scene, camera); }
, ? β rotation
0,02 render
(), . , , .
render
() :
var step=0; function render() { ... step+=0.04; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... requestAnimationFrame(render); renderer.render(scene, camera); }
rotation
; position
. , , . . Math.cos()
Math.sin()
step
. . , step+=0.04
. :
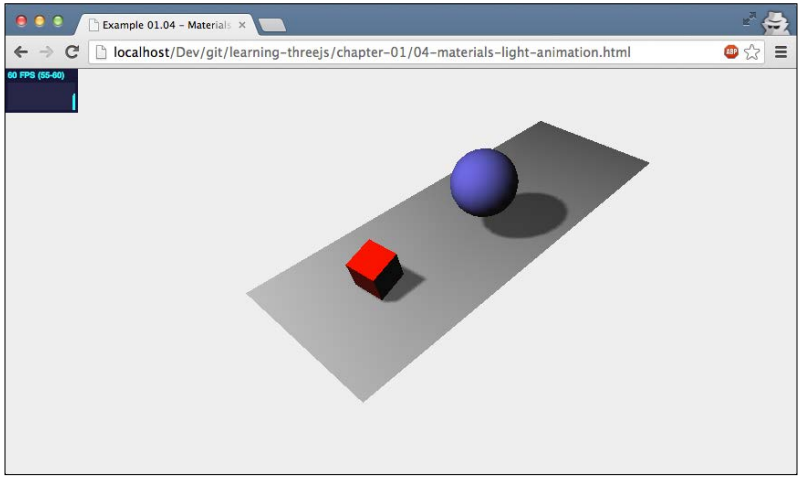
.
, . 3D-, , , . , , . , .
dat.GUI
Google dat.GUI( ), . , , :
, dat.GUI , :
<script type="text/javascript" src="../libs/dat.gui.js"></script>
β JavaScript , , , dat.GUI. JavaScript :
var controls = new function() { this.rotationSpeed = 0.02; this.bouncingSpeed = 0.03; }
: this.rotationSpeed
this.bouncingSpeed
. dat.GUI , :
var gui = new dat.GUI(); gui.add(controls, 'rotationSpeed',0,0.5); gui.add(controls, 'bouncingSpeed',0,0.5);
rotationSpeed
bouncingSpeed
0 0,5. , β , render
, , dat.GUI, . :
function render() { ... cube.rotation.x += controls.rotationSpeed; cube.rotation.y += controls.rotationSpeed; cube.rotation.z += controls.rotationSpeed; step+=controls.bouncingSpeed; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... }
, , , :

.
ASCII
3D- . Three.js , , . , : ASCII . , -ASCII, . :
$("#WebGL-output").append(renderer.domElement);
:
var effect = new THREE.AsciiEffect( renderer ); effect.setSize(window.innerWidth, window.innerHeight ); $("#WebGL-output").append(effect.domElement);
. renderer.render(scene, camera), effect.render(scene,camera). :
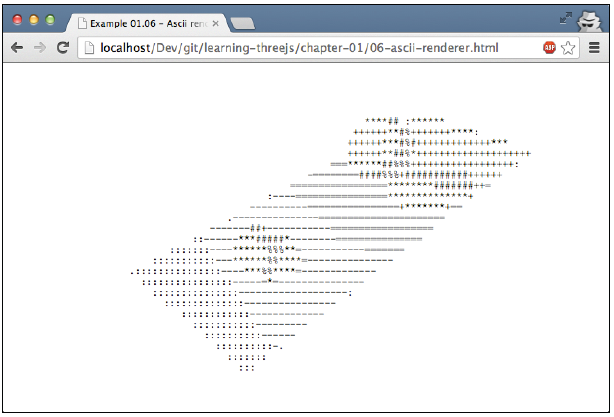
.
, , , Three.js .
. , Three.js .
, . , Three.js.
, : GitHub
:
<script type="text/javascript" src="../libs/stats.js"></script>
,
<div id="Stats-output"></div>
, β , :
function initStats() { var stats = new Stats(); stats.setMode(0); stats.domElement.style.position = 'absolute'; stats.domElement.style.left = '0px'; stats.domElement.style.top = '0px'; $("#Stats-output").append(stats.domElement ); return stats; }
. setMode
(). 0, FPS, 1, . FPS, 0. jQuery :
$(function () { var stats = initStats(); β¦ }
render():
function render() { stats.update(); ... requestAnimationFrame(render); renderer.render(scene, camera); }
, , :
requestAnimationFrame
() . render
() , . :
function render() { ... cube.rotation.x += 0.02; cube.rotation.y += 0.02; cube.rotation.z += 0.02; ... requestAnimationFrame(render); renderer.render(scene, camera); }
, ? β rotation
0,02 render
(), . , , .
render
() :
var step=0; function render() { ... step+=0.04; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... requestAnimationFrame(render); renderer.render(scene, camera); }
rotation
; position
. , , . . Math.cos()
Math.sin()
step
. . , step+=0.04
. :
.
, . 3D-, , , . , , . , .
dat.GUI
Google dat.GUI( ), . , , :
, dat.GUI , :
<script type="text/javascript" src="../libs/dat.gui.js"></script>
β JavaScript , , , dat.GUI. JavaScript :
var controls = new function() { this.rotationSpeed = 0.02; this.bouncingSpeed = 0.03; }
: this.rotationSpeed
this.bouncingSpeed
. dat.GUI , :
var gui = new dat.GUI(); gui.add(controls, 'rotationSpeed',0,0.5); gui.add(controls, 'bouncingSpeed',0,0.5);
rotationSpeed
bouncingSpeed
0 0,5. , β , render
, , dat.GUI, . :
function render() { ... cube.rotation.x += controls.rotationSpeed; cube.rotation.y += controls.rotationSpeed; cube.rotation.z += controls.rotationSpeed; step+=controls.bouncingSpeed; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... }
, , , :
.
ASCII
3D- . Three.js , , . , : ASCII . , -ASCII, . :
$("#WebGL-output").append(renderer.domElement);
:
var effect = new THREE.AsciiEffect( renderer ); effect.setSize(window.innerWidth, window.innerHeight ); $("#WebGL-output").append(effect.domElement);
. renderer.render(scene, camera), effect.render(scene,camera). :
.
, , , Three.js .
. , Three.js .
, . , Three.js.
, : GitHub
:
<script type="text/javascript" src="../libs/stats.js"></script>
,
<div id="Stats-output"></div>
, β , :
function initStats() { var stats = new Stats(); stats.setMode(0); stats.domElement.style.position = 'absolute'; stats.domElement.style.left = '0px'; stats.domElement.style.top = '0px'; $("#Stats-output").append(stats.domElement ); return stats; }
. setMode
(). 0, FPS, 1, . FPS, 0. jQuery :
$(function () { var stats = initStats(); β¦ }
render():
function render() { stats.update(); ... requestAnimationFrame(render); renderer.render(scene, camera); }
, , :

requestAnimationFrame
() . render
() , . :
function render() { ... cube.rotation.x += 0.02; cube.rotation.y += 0.02; cube.rotation.z += 0.02; ... requestAnimationFrame(render); renderer.render(scene, camera); }
, ? β rotation
0,02 render
(), . , , .
render
() :
var step=0; function render() { ... step+=0.04; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... requestAnimationFrame(render); renderer.render(scene, camera); }
rotation
; position
. , , . . Math.cos()
Math.sin()
step
. . , step+=0.04
. :
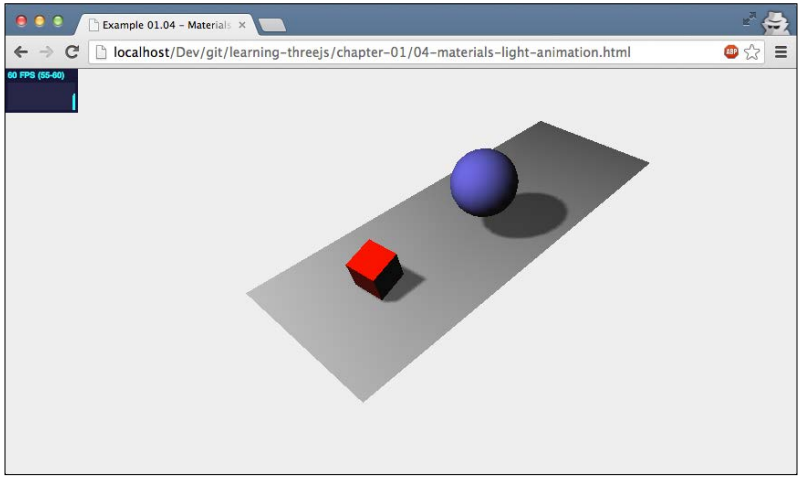
.
, . 3D-, , , . , , . , .
dat.GUI
Google dat.GUI( ), . , , :
, dat.GUI , :
<script type="text/javascript" src="../libs/dat.gui.js"></script>
β JavaScript , , , dat.GUI. JavaScript :
var controls = new function() { this.rotationSpeed = 0.02; this.bouncingSpeed = 0.03; }
: this.rotationSpeed
this.bouncingSpeed
. dat.GUI , :
var gui = new dat.GUI(); gui.add(controls, 'rotationSpeed',0,0.5); gui.add(controls, 'bouncingSpeed',0,0.5);
rotationSpeed
bouncingSpeed
0 0,5. , β , render
, , dat.GUI, . :
function render() { ... cube.rotation.x += controls.rotationSpeed; cube.rotation.y += controls.rotationSpeed; cube.rotation.z += controls.rotationSpeed; step+=controls.bouncingSpeed; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... }
, , , :

.
ASCII
3D- . Three.js , , . , : ASCII . , -ASCII, . :
$("#WebGL-output").append(renderer.domElement);
:
var effect = new THREE.AsciiEffect( renderer ); effect.setSize(window.innerWidth, window.innerHeight ); $("#WebGL-output").append(effect.domElement);
. renderer.render(scene, camera), effect.render(scene,camera). :
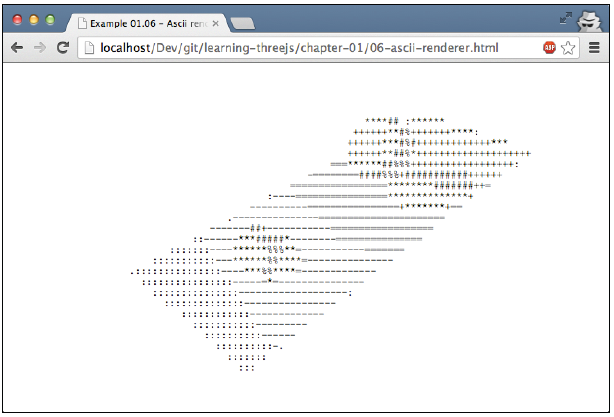
.
, , , Three.js .
. , Three.js .
, . , Three.js.
, : GitHub
:
<script type="text/javascript" src="../libs/stats.js"></script>
,
<div id="Stats-output"></div>
, β , :
function initStats() { var stats = new Stats(); stats.setMode(0); stats.domElement.style.position = 'absolute'; stats.domElement.style.left = '0px'; stats.domElement.style.top = '0px'; $("#Stats-output").append(stats.domElement ); return stats; }
. setMode
(). 0, FPS, 1, . FPS, 0. jQuery :
$(function () { var stats = initStats(); β¦ }
render():
function render() { stats.update(); ... requestAnimationFrame(render); renderer.render(scene, camera); }
, , :
requestAnimationFrame
() . render
() , . :
function render() { ... cube.rotation.x += 0.02; cube.rotation.y += 0.02; cube.rotation.z += 0.02; ... requestAnimationFrame(render); renderer.render(scene, camera); }
, ? β rotation
0,02 render
(), . , , .
render
() :
var step=0; function render() { ... step+=0.04; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... requestAnimationFrame(render); renderer.render(scene, camera); }
rotation
; position
. , , . . Math.cos()
Math.sin()
step
. . , step+=0.04
. :
.
, . 3D-, , , . , , . , .
dat.GUI
Google dat.GUI( ), . , , :
, dat.GUI , :
<script type="text/javascript" src="../libs/dat.gui.js"></script>
β JavaScript , , , dat.GUI. JavaScript :
var controls = new function() { this.rotationSpeed = 0.02; this.bouncingSpeed = 0.03; }
: this.rotationSpeed
this.bouncingSpeed
. dat.GUI , :
var gui = new dat.GUI(); gui.add(controls, 'rotationSpeed',0,0.5); gui.add(controls, 'bouncingSpeed',0,0.5);
rotationSpeed
bouncingSpeed
0 0,5. , β , render
, , dat.GUI, . :
function render() { ... cube.rotation.x += controls.rotationSpeed; cube.rotation.y += controls.rotationSpeed; cube.rotation.z += controls.rotationSpeed; step+=controls.bouncingSpeed; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... }
, , , :
.
ASCII
3D- . Three.js , , . , : ASCII . , -ASCII, . :
$("#WebGL-output").append(renderer.domElement);
:
var effect = new THREE.AsciiEffect( renderer ); effect.setSize(window.innerWidth, window.innerHeight ); $("#WebGL-output").append(effect.domElement);
. renderer.render(scene, camera), effect.render(scene,camera). :
.
, , , Three.js .
. , Three.js .
, . , Three.js.
, : GitHub
:
<script type="text/javascript" src="../libs/stats.js"></script>
,
<div id="Stats-output"></div>
, β , :
function initStats() { var stats = new Stats(); stats.setMode(0); stats.domElement.style.position = 'absolute'; stats.domElement.style.left = '0px'; stats.domElement.style.top = '0px'; $("#Stats-output").append(stats.domElement ); return stats; }
. setMode
(). 0, FPS, 1, . FPS, 0. jQuery :
$(function () { var stats = initStats(); β¦ }
render():
function render() { stats.update(); ... requestAnimationFrame(render); renderer.render(scene, camera); }
, , :

requestAnimationFrame
() . render
() , . :
function render() { ... cube.rotation.x += 0.02; cube.rotation.y += 0.02; cube.rotation.z += 0.02; ... requestAnimationFrame(render); renderer.render(scene, camera); }
, ? β rotation
0,02 render
(), . , , .
render
() :
var step=0; function render() { ... step+=0.04; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... requestAnimationFrame(render); renderer.render(scene, camera); }
rotation
; position
. , , . . Math.cos()
Math.sin()
step
. . , step+=0.04
. :
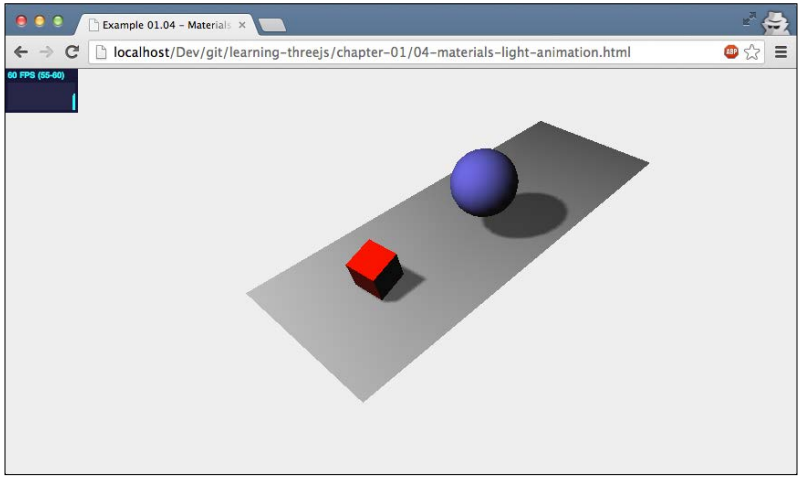
.
, . 3D-, , , . , , . , .
dat.GUI
Google dat.GUI( ), . , , :
, dat.GUI , :
<script type="text/javascript" src="../libs/dat.gui.js"></script>
β JavaScript , , , dat.GUI. JavaScript :
var controls = new function() { this.rotationSpeed = 0.02; this.bouncingSpeed = 0.03; }
: this.rotationSpeed
this.bouncingSpeed
. dat.GUI , :
var gui = new dat.GUI(); gui.add(controls, 'rotationSpeed',0,0.5); gui.add(controls, 'bouncingSpeed',0,0.5);
rotationSpeed
bouncingSpeed
0 0,5. , β , render
, , dat.GUI, . :
function render() { ... cube.rotation.x += controls.rotationSpeed; cube.rotation.y += controls.rotationSpeed; cube.rotation.z += controls.rotationSpeed; step+=controls.bouncingSpeed; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... }
, , , :

.
ASCII
3D- . Three.js , , . , : ASCII . , -ASCII, . :
$("#WebGL-output").append(renderer.domElement);
:
var effect = new THREE.AsciiEffect( renderer ); effect.setSize(window.innerWidth, window.innerHeight ); $("#WebGL-output").append(effect.domElement);
. renderer.render(scene, camera), effect.render(scene,camera). :
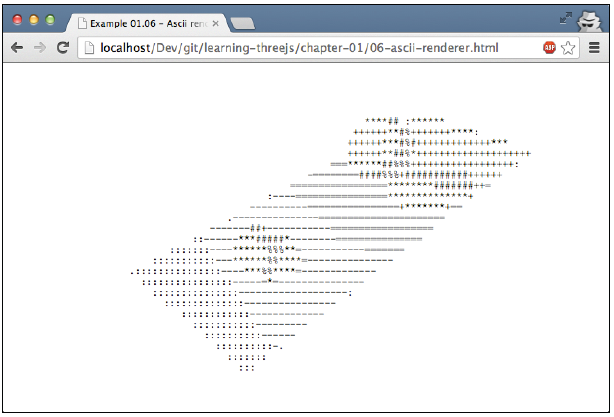
.
, , , Three.js .
. , Three.js .
, . , Three.js.
, : GitHub
:
<script type="text/javascript" src="../libs/stats.js"></script>
,
<div id="Stats-output"></div>
, β , :
function initStats() { var stats = new Stats(); stats.setMode(0); stats.domElement.style.position = 'absolute'; stats.domElement.style.left = '0px'; stats.domElement.style.top = '0px'; $("#Stats-output").append(stats.domElement ); return stats; }
. setMode
(). 0, FPS, 1, . FPS, 0. jQuery :
$(function () { var stats = initStats(); β¦ }
render():
function render() { stats.update(); ... requestAnimationFrame(render); renderer.render(scene, camera); }
, , :
requestAnimationFrame
() . render
() , . :
function render() { ... cube.rotation.x += 0.02; cube.rotation.y += 0.02; cube.rotation.z += 0.02; ... requestAnimationFrame(render); renderer.render(scene, camera); }
, ? β rotation
0,02 render
(), . , , .
render
() :
var step=0; function render() { ... step+=0.04; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... requestAnimationFrame(render); renderer.render(scene, camera); }
rotation
; position
. , , . . Math.cos()
Math.sin()
step
. . , step+=0.04
. :
.
, . 3D-, , , . , , . , .
dat.GUI
Google dat.GUI( ), . , , :
, dat.GUI , :
<script type="text/javascript" src="../libs/dat.gui.js"></script>
β JavaScript , , , dat.GUI. JavaScript :
var controls = new function() { this.rotationSpeed = 0.02; this.bouncingSpeed = 0.03; }
: this.rotationSpeed
this.bouncingSpeed
. dat.GUI , :
var gui = new dat.GUI(); gui.add(controls, 'rotationSpeed',0,0.5); gui.add(controls, 'bouncingSpeed',0,0.5);
rotationSpeed
bouncingSpeed
0 0,5. , β , render
, , dat.GUI, . :
function render() { ... cube.rotation.x += controls.rotationSpeed; cube.rotation.y += controls.rotationSpeed; cube.rotation.z += controls.rotationSpeed; step+=controls.bouncingSpeed; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... }
, , , :
.
ASCII
3D- . Three.js , , . , : ASCII . , -ASCII, . :
$("#WebGL-output").append(renderer.domElement);
:
var effect = new THREE.AsciiEffect( renderer ); effect.setSize(window.innerWidth, window.innerHeight ); $("#WebGL-output").append(effect.domElement);
. renderer.render(scene, camera), effect.render(scene,camera). :
.
, , , Three.js .
. , Three.js .
, . , Three.js.
, : GitHub
:
<script type="text/javascript" src="../libs/stats.js"></script>
,
<div id="Stats-output"></div>
, β , :
function initStats() { var stats = new Stats(); stats.setMode(0); stats.domElement.style.position = 'absolute'; stats.domElement.style.left = '0px'; stats.domElement.style.top = '0px'; $("#Stats-output").append(stats.domElement ); return stats; }
. setMode
(). 0, FPS, 1, . FPS, 0. jQuery :
$(function () { var stats = initStats(); β¦ }
render():
function render() { stats.update(); ... requestAnimationFrame(render); renderer.render(scene, camera); }
, , :

requestAnimationFrame
() . render
() , . :
function render() { ... cube.rotation.x += 0.02; cube.rotation.y += 0.02; cube.rotation.z += 0.02; ... requestAnimationFrame(render); renderer.render(scene, camera); }
, ? β rotation
0,02 render
(), . , , .
render
() :
var step=0; function render() { ... step+=0.04; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... requestAnimationFrame(render); renderer.render(scene, camera); }
rotation
; position
. , , . . Math.cos()
Math.sin()
step
. . , step+=0.04
. :
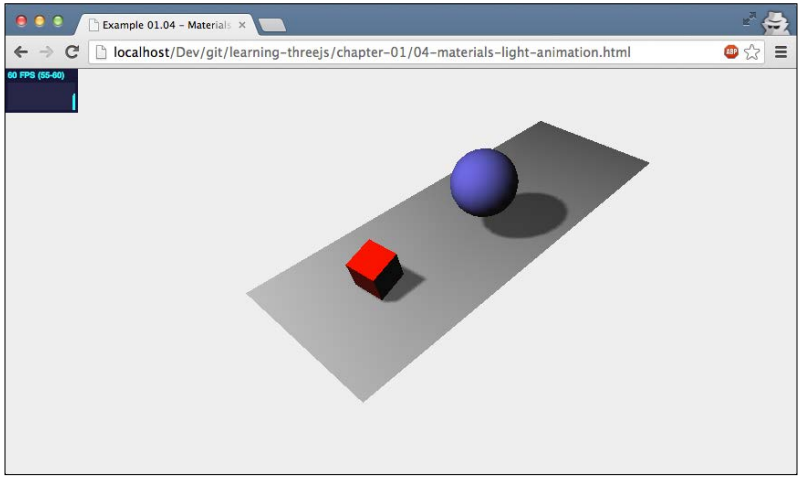
.
, . 3D-, , , . , , . , .
dat.GUI
Google dat.GUI( ), . , , :
, dat.GUI , :
<script type="text/javascript" src="../libs/dat.gui.js"></script>
β JavaScript , , , dat.GUI. JavaScript :
var controls = new function() { this.rotationSpeed = 0.02; this.bouncingSpeed = 0.03; }
: this.rotationSpeed
this.bouncingSpeed
. dat.GUI , :
var gui = new dat.GUI(); gui.add(controls, 'rotationSpeed',0,0.5); gui.add(controls, 'bouncingSpeed',0,0.5);
rotationSpeed
bouncingSpeed
0 0,5. , β , render
, , dat.GUI, . :
function render() { ... cube.rotation.x += controls.rotationSpeed; cube.rotation.y += controls.rotationSpeed; cube.rotation.z += controls.rotationSpeed; step+=controls.bouncingSpeed; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... }
, , , :

.
ASCII
3D- . Three.js , , . , : ASCII . , -ASCII, . :
$("#WebGL-output").append(renderer.domElement);
:
var effect = new THREE.AsciiEffect( renderer ); effect.setSize(window.innerWidth, window.innerHeight ); $("#WebGL-output").append(effect.domElement);
. renderer.render(scene, camera), effect.render(scene,camera). :
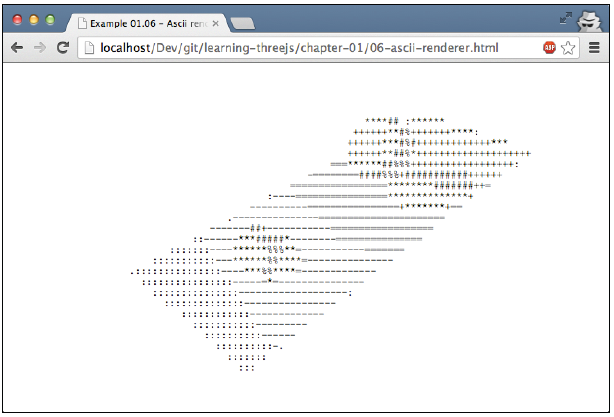
.
, , , Three.js .
. , Three.js .
, . , Three.js.
, : GitHub
:
<script type="text/javascript" src="../libs/stats.js"></script>
,
<div id="Stats-output"></div>
, β , :
function initStats() { var stats = new Stats(); stats.setMode(0); stats.domElement.style.position = 'absolute'; stats.domElement.style.left = '0px'; stats.domElement.style.top = '0px'; $("#Stats-output").append(stats.domElement ); return stats; }
. setMode
(). 0, FPS, 1, . FPS, 0. jQuery :
$(function () { var stats = initStats(); β¦ }
render():
function render() { stats.update(); ... requestAnimationFrame(render); renderer.render(scene, camera); }
, , :
requestAnimationFrame
() . render
() , . :
function render() { ... cube.rotation.x += 0.02; cube.rotation.y += 0.02; cube.rotation.z += 0.02; ... requestAnimationFrame(render); renderer.render(scene, camera); }
, ? β rotation
0,02 render
(), . , , .
render
() :
var step=0; function render() { ... step+=0.04; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... requestAnimationFrame(render); renderer.render(scene, camera); }
rotation
; position
. , , . . Math.cos()
Math.sin()
step
. . , step+=0.04
. :
.
, . 3D-, , , . , , . , .
dat.GUI
Google dat.GUI( ), . , , :
, dat.GUI , :
<script type="text/javascript" src="../libs/dat.gui.js"></script>
β JavaScript , , , dat.GUI. JavaScript :
var controls = new function() { this.rotationSpeed = 0.02; this.bouncingSpeed = 0.03; }
: this.rotationSpeed
this.bouncingSpeed
. dat.GUI , :
var gui = new dat.GUI(); gui.add(controls, 'rotationSpeed',0,0.5); gui.add(controls, 'bouncingSpeed',0,0.5);
rotationSpeed
bouncingSpeed
0 0,5. , β , render
, , dat.GUI, . :
function render() { ... cube.rotation.x += controls.rotationSpeed; cube.rotation.y += controls.rotationSpeed; cube.rotation.z += controls.rotationSpeed; step+=controls.bouncingSpeed; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... }
, , , :
.
ASCII
3D- . Three.js , , . , : ASCII . , -ASCII, . :
$("#WebGL-output").append(renderer.domElement);
:
var effect = new THREE.AsciiEffect( renderer ); effect.setSize(window.innerWidth, window.innerHeight ); $("#WebGL-output").append(effect.domElement);
. renderer.render(scene, camera), effect.render(scene,camera). :
.
, , , Three.js .
. , Three.js .
, . , Three.js.
, : GitHub
:
<script type="text/javascript" src="../libs/stats.js"></script>
,
<div id="Stats-output"></div>
, β , :
function initStats() { var stats = new Stats(); stats.setMode(0); stats.domElement.style.position = 'absolute'; stats.domElement.style.left = '0px'; stats.domElement.style.top = '0px'; $("#Stats-output").append(stats.domElement ); return stats; }
. setMode
(). 0, FPS, 1, . FPS, 0. jQuery :
$(function () { var stats = initStats(); β¦ }
render():
function render() { stats.update(); ... requestAnimationFrame(render); renderer.render(scene, camera); }
, , :

requestAnimationFrame
() . render
() , . :
function render() { ... cube.rotation.x += 0.02; cube.rotation.y += 0.02; cube.rotation.z += 0.02; ... requestAnimationFrame(render); renderer.render(scene, camera); }
, ? β rotation
0,02 render
(), . , , .
render
() :
var step=0; function render() { ... step+=0.04; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... requestAnimationFrame(render); renderer.render(scene, camera); }
rotation
; position
. , , . . Math.cos()
Math.sin()
step
. . , step+=0.04
. :
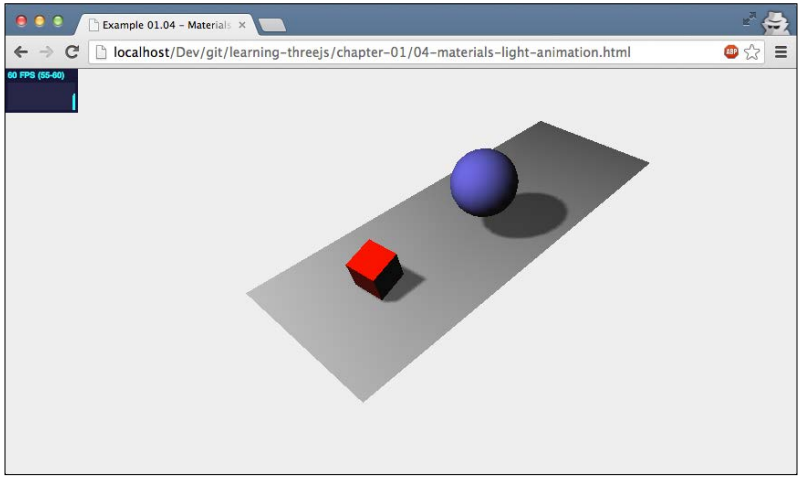
.
, . 3D-, , , . , , . , .
dat.GUI
Google dat.GUI( ), . , , :
, dat.GUI , :
<script type="text/javascript" src="../libs/dat.gui.js"></script>
β JavaScript , , , dat.GUI. JavaScript :
var controls = new function() { this.rotationSpeed = 0.02; this.bouncingSpeed = 0.03; }
: this.rotationSpeed
this.bouncingSpeed
. dat.GUI , :
var gui = new dat.GUI(); gui.add(controls, 'rotationSpeed',0,0.5); gui.add(controls, 'bouncingSpeed',0,0.5);
rotationSpeed
bouncingSpeed
0 0,5. , β , render
, , dat.GUI, . :
function render() { ... cube.rotation.x += controls.rotationSpeed; cube.rotation.y += controls.rotationSpeed; cube.rotation.z += controls.rotationSpeed; step+=controls.bouncingSpeed; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... }
, , , :

.
ASCII
3D- . Three.js , , . , : ASCII . , -ASCII, . :
$("#WebGL-output").append(renderer.domElement);
:
var effect = new THREE.AsciiEffect( renderer ); effect.setSize(window.innerWidth, window.innerHeight ); $("#WebGL-output").append(effect.domElement);
. renderer.render(scene, camera), effect.render(scene,camera). :
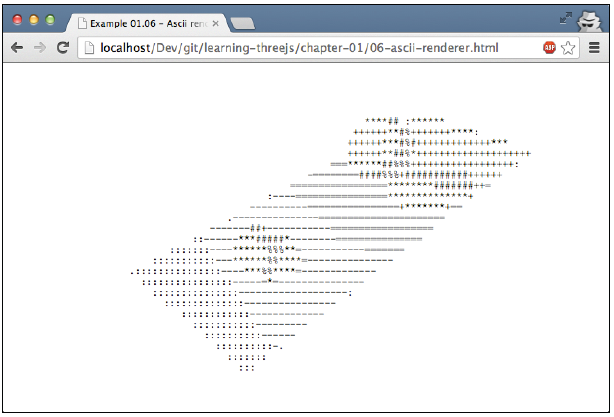
.
, , , Three.js .
. , Three.js .
, . , Three.js.
, : GitHub
:
<script type="text/javascript" src="../libs/stats.js"></script>
,
<div id="Stats-output"></div>
, β , :
function initStats() { var stats = new Stats(); stats.setMode(0); stats.domElement.style.position = 'absolute'; stats.domElement.style.left = '0px'; stats.domElement.style.top = '0px'; $("#Stats-output").append(stats.domElement ); return stats; }
. setMode
(). 0, FPS, 1, . FPS, 0. jQuery :
$(function () { var stats = initStats(); β¦ }
render():
function render() { stats.update(); ... requestAnimationFrame(render); renderer.render(scene, camera); }
, , :

requestAnimationFrame
() . render
() , . :
function render() { ... cube.rotation.x += 0.02; cube.rotation.y += 0.02; cube.rotation.z += 0.02; ... requestAnimationFrame(render); renderer.render(scene, camera); }
, ? β rotation
0,02 render
(), . , , .
render
() :
var step=0; function render() { ... step+=0.04; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... requestAnimationFrame(render); renderer.render(scene, camera); }
rotation
; position
. , , . . Math.cos()
Math.sin()
step
. . , step+=0.04
. :
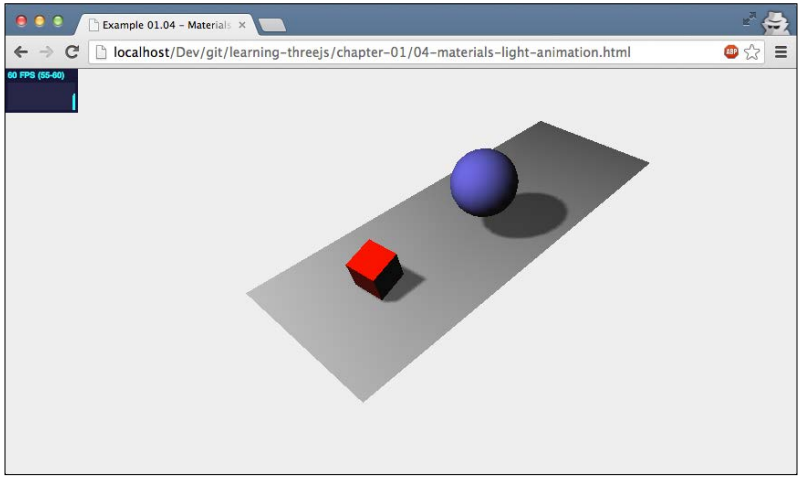
.
, . 3D-, , , . , , . , .
dat.GUI
Google dat.GUI( ), . , , :
, dat.GUI , :
<script type="text/javascript" src="../libs/dat.gui.js"></script>
β JavaScript , , , dat.GUI. JavaScript :
var controls = new function() { this.rotationSpeed = 0.02; this.bouncingSpeed = 0.03; }
: this.rotationSpeed
this.bouncingSpeed
. dat.GUI , :
var gui = new dat.GUI(); gui.add(controls, 'rotationSpeed',0,0.5); gui.add(controls, 'bouncingSpeed',0,0.5);
rotationSpeed
bouncingSpeed
0 0,5. , β , render
, , dat.GUI, . :
function render() { ... cube.rotation.x += controls.rotationSpeed; cube.rotation.y += controls.rotationSpeed; cube.rotation.z += controls.rotationSpeed; step+=controls.bouncingSpeed; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... }
, , , :

.
ASCII
3D- . Three.js , , . , : ASCII . , -ASCII, . :
$("#WebGL-output").append(renderer.domElement);
:
var effect = new THREE.AsciiEffect( renderer ); effect.setSize(window.innerWidth, window.innerHeight ); $("#WebGL-output").append(effect.domElement);
. renderer.render(scene, camera), effect.render(scene,camera). :
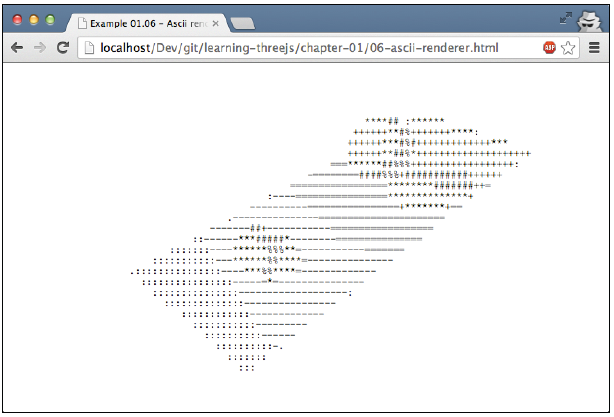
.
, , , Three.js .
. , Three.js .
, . , Three.js.
, : GitHub
:
<script type="text/javascript" src="../libs/stats.js"></script>
,
<div id="Stats-output"></div>
, β , :
function initStats() { var stats = new Stats(); stats.setMode(0); stats.domElement.style.position = 'absolute'; stats.domElement.style.left = '0px'; stats.domElement.style.top = '0px'; $("#Stats-output").append(stats.domElement ); return stats; }
. setMode
(). 0, FPS, 1, . FPS, 0. jQuery :
$(function () { var stats = initStats(); β¦ }
render():
function render() { stats.update(); ... requestAnimationFrame(render); renderer.render(scene, camera); }
, , :

requestAnimationFrame
() . render
() , . :
function render() { ... cube.rotation.x += 0.02; cube.rotation.y += 0.02; cube.rotation.z += 0.02; ... requestAnimationFrame(render); renderer.render(scene, camera); }
, ? β rotation
0,02 render
(), . , , .
render
() :
var step=0; function render() { ... step+=0.04; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... requestAnimationFrame(render); renderer.render(scene, camera); }
rotation
; position
. , , . . Math.cos()
Math.sin()
step
. . , step+=0.04
. :
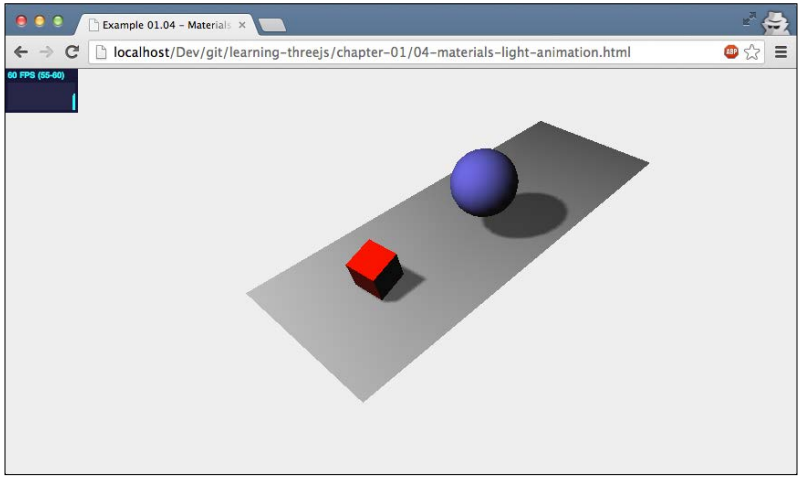
.
, . 3D-, , , . , , . , .
dat.GUI
Google dat.GUI( ), . , , :
, dat.GUI , :
<script type="text/javascript" src="../libs/dat.gui.js"></script>
β JavaScript , , , dat.GUI. JavaScript :
var controls = new function() { this.rotationSpeed = 0.02; this.bouncingSpeed = 0.03; }
: this.rotationSpeed
this.bouncingSpeed
. dat.GUI , :
var gui = new dat.GUI(); gui.add(controls, 'rotationSpeed',0,0.5); gui.add(controls, 'bouncingSpeed',0,0.5);
rotationSpeed
bouncingSpeed
0 0,5. , β , render
, , dat.GUI, . :
function render() { ... cube.rotation.x += controls.rotationSpeed; cube.rotation.y += controls.rotationSpeed; cube.rotation.z += controls.rotationSpeed; step+=controls.bouncingSpeed; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... }
, , , :

.
ASCII
3D- . Three.js , , . , : ASCII . , -ASCII, . :
$("#WebGL-output").append(renderer.domElement);
:
var effect = new THREE.AsciiEffect( renderer ); effect.setSize(window.innerWidth, window.innerHeight ); $("#WebGL-output").append(effect.domElement);
. renderer.render(scene, camera), effect.render(scene,camera). :
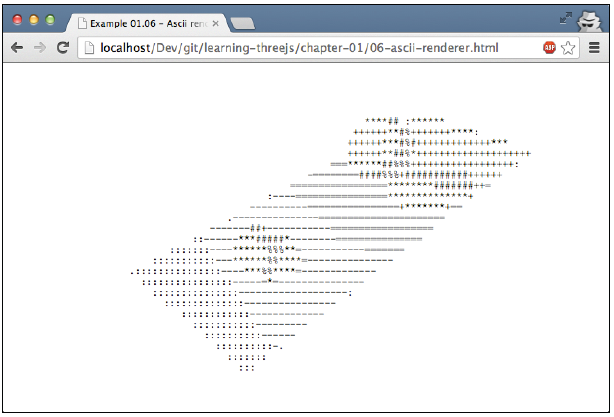
.
, , , Three.js .
. , Three.js .
, . , Three.js.
, : GitHub
:
<script type="text/javascript" src="../libs/stats.js"></script>
,
<div id="Stats-output"></div>
, β , :
function initStats() { var stats = new Stats(); stats.setMode(0); stats.domElement.style.position = 'absolute'; stats.domElement.style.left = '0px'; stats.domElement.style.top = '0px'; $("#Stats-output").append(stats.domElement ); return stats; }
. setMode
(). 0, FPS, 1, . FPS, 0. jQuery :
$(function () { var stats = initStats(); β¦ }
render():
function render() { stats.update(); ... requestAnimationFrame(render); renderer.render(scene, camera); }
, , :
requestAnimationFrame
() . render
() , . :
function render() { ... cube.rotation.x += 0.02; cube.rotation.y += 0.02; cube.rotation.z += 0.02; ... requestAnimationFrame(render); renderer.render(scene, camera); }
, ? β rotation
0,02 render
(), . , , .
render
() :
var step=0; function render() { ... step+=0.04; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... requestAnimationFrame(render); renderer.render(scene, camera); }
rotation
; position
. , , . . Math.cos()
Math.sin()
step
. . , step+=0.04
. :
.
, . 3D-, , , . , , . , .
dat.GUI
Google dat.GUI( ), . , , :
, dat.GUI , :
<script type="text/javascript" src="../libs/dat.gui.js"></script>
β JavaScript , , , dat.GUI. JavaScript :
var controls = new function() { this.rotationSpeed = 0.02; this.bouncingSpeed = 0.03; }
: this.rotationSpeed
this.bouncingSpeed
. dat.GUI , :
var gui = new dat.GUI(); gui.add(controls, 'rotationSpeed',0,0.5); gui.add(controls, 'bouncingSpeed',0,0.5);
rotationSpeed
bouncingSpeed
0 0,5. , β , render
, , dat.GUI, . :
function render() { ... cube.rotation.x += controls.rotationSpeed; cube.rotation.y += controls.rotationSpeed; cube.rotation.z += controls.rotationSpeed; step+=controls.bouncingSpeed; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... }
, , , :
.
ASCII
3D- . Three.js , , . , : ASCII . , -ASCII, . :
$("#WebGL-output").append(renderer.domElement);
:
var effect = new THREE.AsciiEffect( renderer ); effect.setSize(window.innerWidth, window.innerHeight ); $("#WebGL-output").append(effect.domElement);
. renderer.render(scene, camera), effect.render(scene,camera). :
.
, , , Three.js .
. , Three.js .
, . , Three.js.
, : GitHub
:
<script type="text/javascript" src="../libs/stats.js"></script>
,
<div id="Stats-output"></div>
, β , :
function initStats() { var stats = new Stats(); stats.setMode(0); stats.domElement.style.position = 'absolute'; stats.domElement.style.left = '0px'; stats.domElement.style.top = '0px'; $("#Stats-output").append(stats.domElement ); return stats; }
. setMode
(). 0, FPS, 1, . FPS, 0. jQuery :
$(function () { var stats = initStats(); β¦ }
render():
function render() { stats.update(); ... requestAnimationFrame(render); renderer.render(scene, camera); }
, , :

requestAnimationFrame
() . render
() , . :
function render() { ... cube.rotation.x += 0.02; cube.rotation.y += 0.02; cube.rotation.z += 0.02; ... requestAnimationFrame(render); renderer.render(scene, camera); }
, ? β rotation
0,02 render
(), . , , .
render
() :
var step=0; function render() { ... step+=0.04; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... requestAnimationFrame(render); renderer.render(scene, camera); }
rotation
; position
. , , . . Math.cos()
Math.sin()
step
. . , step+=0.04
. :
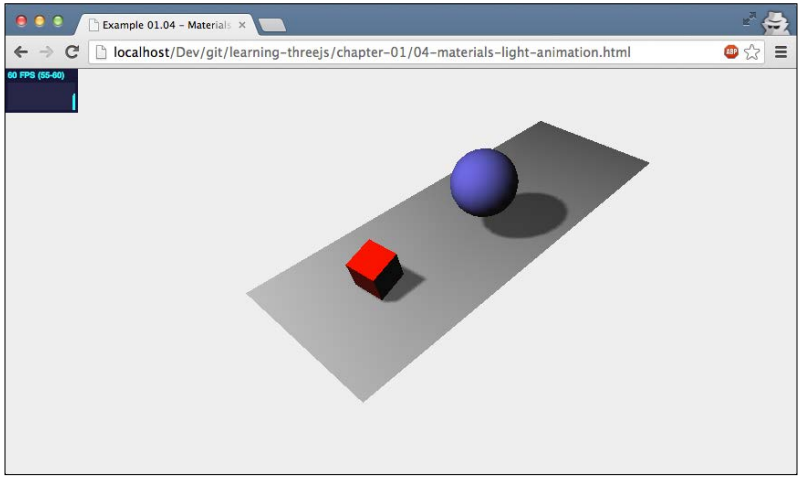
.
, . 3D-, , , . , , . , .
dat.GUI
Google dat.GUI( ), . , , :
, dat.GUI , :
<script type="text/javascript" src="../libs/dat.gui.js"></script>
β JavaScript , , , dat.GUI. JavaScript :
var controls = new function() { this.rotationSpeed = 0.02; this.bouncingSpeed = 0.03; }
: this.rotationSpeed
this.bouncingSpeed
. dat.GUI , :
var gui = new dat.GUI(); gui.add(controls, 'rotationSpeed',0,0.5); gui.add(controls, 'bouncingSpeed',0,0.5);
rotationSpeed
bouncingSpeed
0 0,5. , β , render
, , dat.GUI, . :
function render() { ... cube.rotation.x += controls.rotationSpeed; cube.rotation.y += controls.rotationSpeed; cube.rotation.z += controls.rotationSpeed; step+=controls.bouncingSpeed; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... }
, , , :

.
ASCII
3D- . Three.js , , . , : ASCII . , -ASCII, . :
$("#WebGL-output").append(renderer.domElement);
:
var effect = new THREE.AsciiEffect( renderer ); effect.setSize(window.innerWidth, window.innerHeight ); $("#WebGL-output").append(effect.domElement);
. renderer.render(scene, camera), effect.render(scene,camera). :
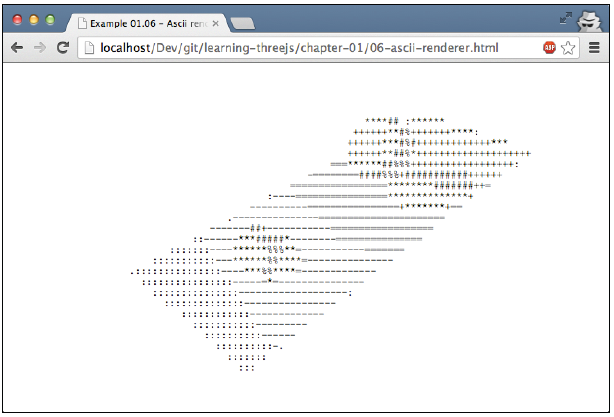
.
, , , Three.js .
. , Three.js .
, . , Three.js.
, : GitHub
:
<script type="text/javascript" src="../libs/stats.js"></script>
,
<div id="Stats-output"></div>
, β , :
function initStats() { var stats = new Stats(); stats.setMode(0); stats.domElement.style.position = 'absolute'; stats.domElement.style.left = '0px'; stats.domElement.style.top = '0px'; $("#Stats-output").append(stats.domElement ); return stats; }
. setMode
(). 0, FPS, 1, . FPS, 0. jQuery :
$(function () { var stats = initStats(); β¦ }
render():
function render() { stats.update(); ... requestAnimationFrame(render); renderer.render(scene, camera); }
, , :
requestAnimationFrame
() . render
() , . :
function render() { ... cube.rotation.x += 0.02; cube.rotation.y += 0.02; cube.rotation.z += 0.02; ... requestAnimationFrame(render); renderer.render(scene, camera); }
, ? β rotation
0,02 render
(), . , , .
render
() :
var step=0; function render() { ... step+=0.04; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... requestAnimationFrame(render); renderer.render(scene, camera); }
rotation
; position
. , , . . Math.cos()
Math.sin()
step
. . , step+=0.04
. :
.
, . 3D-, , , . , , . , .
dat.GUI
Google dat.GUI( ), . , , :
, dat.GUI , :
<script type="text/javascript" src="../libs/dat.gui.js"></script>
β JavaScript , , , dat.GUI. JavaScript :
var controls = new function() { this.rotationSpeed = 0.02; this.bouncingSpeed = 0.03; }
: this.rotationSpeed
this.bouncingSpeed
. dat.GUI , :
var gui = new dat.GUI(); gui.add(controls, 'rotationSpeed',0,0.5); gui.add(controls, 'bouncingSpeed',0,0.5);
rotationSpeed
bouncingSpeed
0 0,5. , β , render
, , dat.GUI, . :
function render() { ... cube.rotation.x += controls.rotationSpeed; cube.rotation.y += controls.rotationSpeed; cube.rotation.z += controls.rotationSpeed; step+=controls.bouncingSpeed; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... }
, , , :
.
ASCII
3D- . Three.js , , . , : ASCII . , -ASCII, . :
$("#WebGL-output").append(renderer.domElement);
:
var effect = new THREE.AsciiEffect( renderer ); effect.setSize(window.innerWidth, window.innerHeight ); $("#WebGL-output").append(effect.domElement);
. renderer.render(scene, camera), effect.render(scene,camera). :
.
, , , Three.js .
. , Three.js .
, . , Three.js.
, : GitHub
:
<script type="text/javascript" src="../libs/stats.js"></script>
,
<div id="Stats-output"></div>
, β , :
function initStats() { var stats = new Stats(); stats.setMode(0); stats.domElement.style.position = 'absolute'; stats.domElement.style.left = '0px'; stats.domElement.style.top = '0px'; $("#Stats-output").append(stats.domElement ); return stats; }
. setMode
(). 0, FPS, 1, . FPS, 0. jQuery :
$(function () { var stats = initStats(); β¦ }
render():
function render() { stats.update(); ... requestAnimationFrame(render); renderer.render(scene, camera); }
, , :

requestAnimationFrame
() . render
() , . :
function render() { ... cube.rotation.x += 0.02; cube.rotation.y += 0.02; cube.rotation.z += 0.02; ... requestAnimationFrame(render); renderer.render(scene, camera); }
, ? β rotation
0,02 render
(), . , , .
render
() :
var step=0; function render() { ... step+=0.04; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... requestAnimationFrame(render); renderer.render(scene, camera); }
rotation
; position
. , , . . Math.cos()
Math.sin()
step
. . , step+=0.04
. :
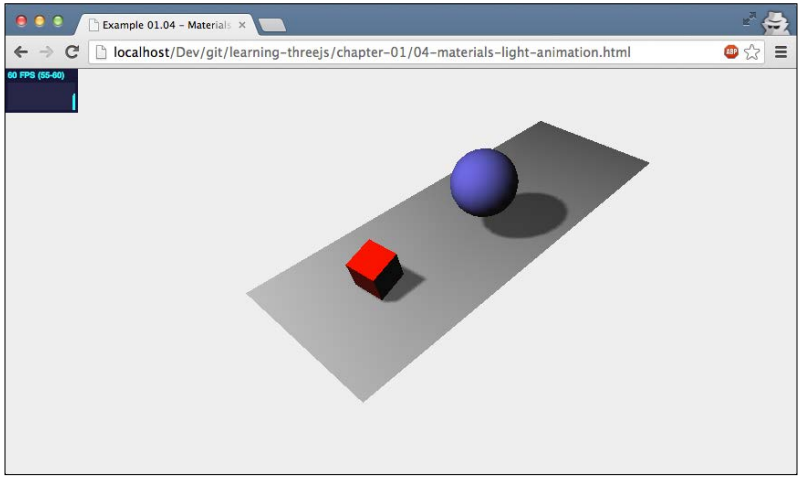
.
, . 3D-, , , . , , . , .
dat.GUI
Google dat.GUI( ), . , , :
, dat.GUI , :
<script type="text/javascript" src="../libs/dat.gui.js"></script>
β JavaScript , , , dat.GUI. JavaScript :
var controls = new function() { this.rotationSpeed = 0.02; this.bouncingSpeed = 0.03; }
: this.rotationSpeed
this.bouncingSpeed
. dat.GUI , :
var gui = new dat.GUI(); gui.add(controls, 'rotationSpeed',0,0.5); gui.add(controls, 'bouncingSpeed',0,0.5);
rotationSpeed
bouncingSpeed
0 0,5. , β , render
, , dat.GUI, . :
function render() { ... cube.rotation.x += controls.rotationSpeed; cube.rotation.y += controls.rotationSpeed; cube.rotation.z += controls.rotationSpeed; step+=controls.bouncingSpeed; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... }
, , , :

.
ASCII
3D- . Three.js , , . , : ASCII . , -ASCII, . :
$("#WebGL-output").append(renderer.domElement);
:
var effect = new THREE.AsciiEffect( renderer ); effect.setSize(window.innerWidth, window.innerHeight ); $("#WebGL-output").append(effect.domElement);
. renderer.render(scene, camera), effect.render(scene,camera). :
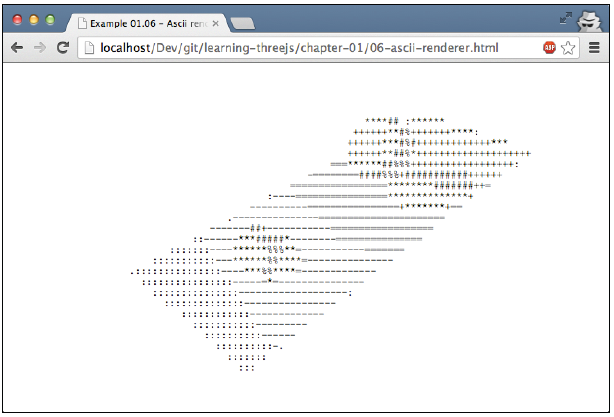
.
, , , Three.js .
. , Three.js .
, . , Three.js.
, : GitHub
:
<script type="text/javascript" src="../libs/stats.js"></script>
,
<div id="Stats-output"></div>
, β , :
function initStats() { var stats = new Stats(); stats.setMode(0); stats.domElement.style.position = 'absolute'; stats.domElement.style.left = '0px'; stats.domElement.style.top = '0px'; $("#Stats-output").append(stats.domElement ); return stats; }
. setMode
(). 0, FPS, 1, . FPS, 0. jQuery :
$(function () { var stats = initStats(); β¦ }
render():
function render() { stats.update(); ... requestAnimationFrame(render); renderer.render(scene, camera); }
, , :

requestAnimationFrame
() . render
() , . :
function render() { ... cube.rotation.x += 0.02; cube.rotation.y += 0.02; cube.rotation.z += 0.02; ... requestAnimationFrame(render); renderer.render(scene, camera); }
, ? β rotation
0,02 render
(), . , , .
render
() :
var step=0; function render() { ... step+=0.04; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... requestAnimationFrame(render); renderer.render(scene, camera); }
rotation
; position
. , , . . Math.cos()
Math.sin()
step
. . , step+=0.04
. :
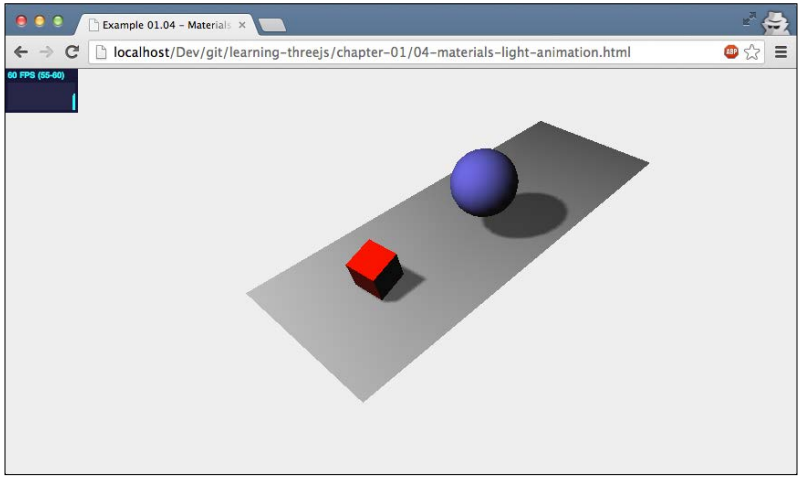
.
, . 3D-, , , . , , . , .
dat.GUI
Google dat.GUI( ), . , , :
, dat.GUI , :
<script type="text/javascript" src="../libs/dat.gui.js"></script>
β JavaScript , , , dat.GUI. JavaScript :
var controls = new function() { this.rotationSpeed = 0.02; this.bouncingSpeed = 0.03; }
: this.rotationSpeed
this.bouncingSpeed
. dat.GUI , :
var gui = new dat.GUI(); gui.add(controls, 'rotationSpeed',0,0.5); gui.add(controls, 'bouncingSpeed',0,0.5);
rotationSpeed
bouncingSpeed
0 0,5. , β , render
, , dat.GUI, . :
function render() { ... cube.rotation.x += controls.rotationSpeed; cube.rotation.y += controls.rotationSpeed; cube.rotation.z += controls.rotationSpeed; step+=controls.bouncingSpeed; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... }
, , , :

.
ASCII
3D- . Three.js , , . , : ASCII . , -ASCII, . :
$("#WebGL-output").append(renderer.domElement);
:
var effect = new THREE.AsciiEffect( renderer ); effect.setSize(window.innerWidth, window.innerHeight ); $("#WebGL-output").append(effect.domElement);
. renderer.render(scene, camera), effect.render(scene,camera). :
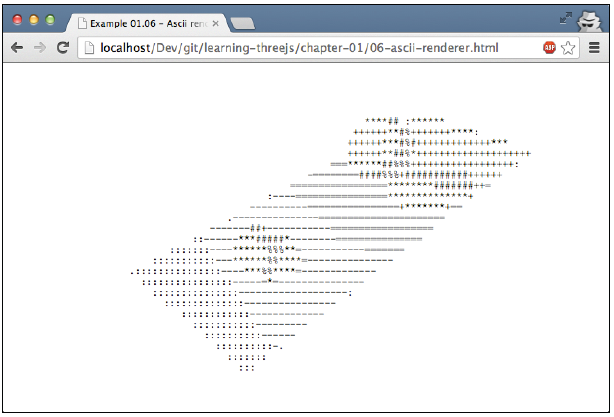
.
, , , Three.js .
. , Three.js .
, . , Three.js.
, : GitHub
:
<script type="text/javascript" src="../libs/stats.js"></script>
,
<div id="Stats-output"></div>
, β , :
function initStats() { var stats = new Stats(); stats.setMode(0); stats.domElement.style.position = 'absolute'; stats.domElement.style.left = '0px'; stats.domElement.style.top = '0px'; $("#Stats-output").append(stats.domElement ); return stats; }
. setMode
(). 0, FPS, 1, . FPS, 0. jQuery :
$(function () { var stats = initStats(); β¦ }
render():
function render() { stats.update(); ... requestAnimationFrame(render); renderer.render(scene, camera); }
, , :

requestAnimationFrame
() . render
() , . :
function render() { ... cube.rotation.x += 0.02; cube.rotation.y += 0.02; cube.rotation.z += 0.02; ... requestAnimationFrame(render); renderer.render(scene, camera); }
, ? β rotation
0,02 render
(), . , , .
render
() :
var step=0; function render() { ... step+=0.04; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... requestAnimationFrame(render); renderer.render(scene, camera); }
rotation
; position
. , , . . Math.cos()
Math.sin()
step
. . , step+=0.04
. :
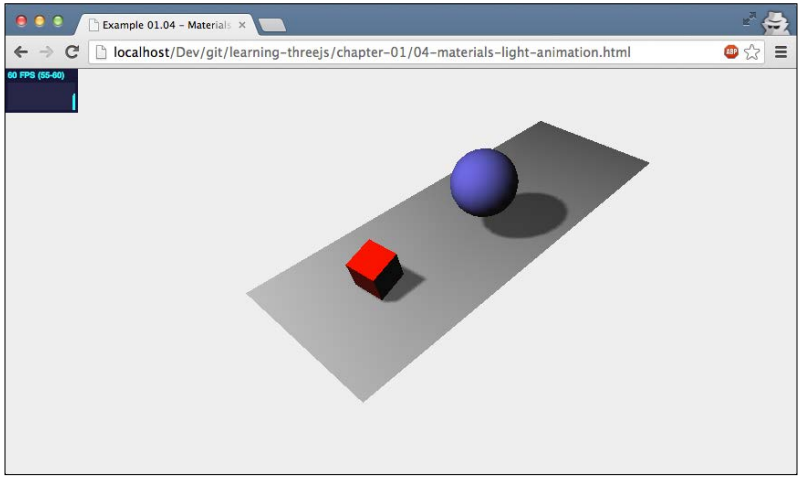
.
, . 3D-, , , . , , . , .
dat.GUI
Google dat.GUI( ), . , , :
, dat.GUI , :
<script type="text/javascript" src="../libs/dat.gui.js"></script>
β JavaScript , , , dat.GUI. JavaScript :
var controls = new function() { this.rotationSpeed = 0.02; this.bouncingSpeed = 0.03; }
: this.rotationSpeed
this.bouncingSpeed
. dat.GUI , :
var gui = new dat.GUI(); gui.add(controls, 'rotationSpeed',0,0.5); gui.add(controls, 'bouncingSpeed',0,0.5);
rotationSpeed
bouncingSpeed
0 0,5. , β , render
, , dat.GUI, . :
function render() { ... cube.rotation.x += controls.rotationSpeed; cube.rotation.y += controls.rotationSpeed; cube.rotation.z += controls.rotationSpeed; step+=controls.bouncingSpeed; sphere.position.x = 20+( 10*(Math.cos(step))); sphere.position.y = 2 +( 10*Math.abs(Math.sin(step))); ... }
, , , :

.
ASCII
3D- . Three.js , , . , : ASCII . , -ASCII, . :
$("#WebGL-output").append(renderer.domElement);
:
var effect = new THREE.AsciiEffect( renderer ); effect.setSize(window.innerWidth, window.innerHeight ); $("#WebGL-output").append(effect.domElement);
. renderer.render(scene, camera), effect.render(scene,camera). :
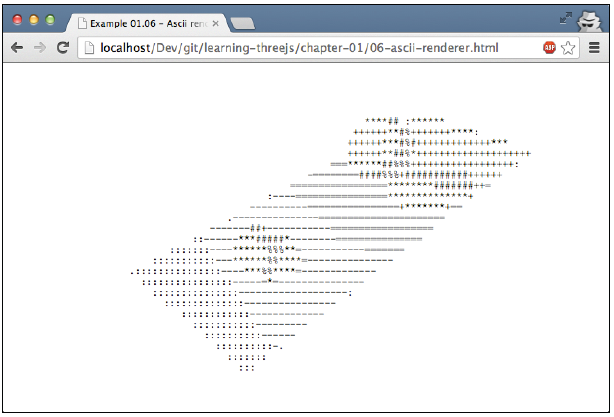
.
, , , Three.js .
. , Three.js .
, . , Three.js.
, : GitHub
Source: https://habr.com/ru/post/224509/
All Articles