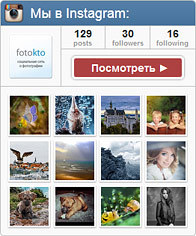
Recently, I needed to integrate an widget into an Instagram site. For users to see the latest published photos. Maybe even subscribed.
Immediately it turned out that Instagram has no official widget. Moreover, you can interact with Instagram only through API requests. No JavaScript libraries, code and design generators. Everything needs to be done by hand.
Immediately there were many third-party services of varying degrees of payment and gratuity. They were united by one thing - the client receives only the code for calling the widget, everything else is drawn from the service. I personally did not like it. Why there is no free standalone open source solution? Maybe I was looking bad? In general, I decided to deposit my five kopecks.
')
Instructions for creating a widget and ready-made solution under the cut.
A small note. Seven months have passed since the writing of this article. During this time, the widget was installed on about 80 resources, a repository was created on GitHub, a kind person gave me an invite, the widget changed the caching mechanism, got its own website, good people based on it made a plug-in for CMS Bitrix, network.
1. Requirements for the widget:
To begin with, I will formulate what widget I wanted to do.
So, the widget should have:
- Instagram icon;
- Title;
- Profile photo;
- Profile statistics;
- Button to go to the profile page;
- Clickable photos;
- Configurable number of photos (total number and how much to display in a line);
- Rubber design and avtomashtab photos depending on the desired width of the widget;
- Output photos by hash tag (added in the process);
- Insert the widget in one line in the HTML-code.
That's what happened in the end:
Demonstration of work>
Example in pictures:
This is how it is inserted into HTML:
<iframe src='/inwidget/index.php' scrolling='no' frameborder='no'></iframe>
If you are interested, then I invite you to get acquainted with the details of the implementation.
2. Registration of the site in Instagram:
The Instagram site has API documentation. Here is the direct link:
http://instagram.com/developer/
First, we are interested in the section “Manage software”. It does not need to register a new application (our site), on behalf of which the widget will work. So go to this section. Press the button “Registration of a new program” and fill in the form:
- Application Name - The name of our application. You can write the name of the site;
- Description - Description of the application;
- Website - the URL of our site;
- OAuth redirect_uri - URL to which the user will go after authorization. Because we just have a widget and we will not authorize anyone, you can simply duplicate the address of our site.
Next, click the button "Register".
After registration, we get two keys. We are interested in CLIENT ID. We will work with him in the future.
3. Getting data through the API:
In order to receive data through the API, you need to use the so-called. "Endpoints" described in the documentation. This is just a list of URLs. Each address is responsible for issuing certain data. The data is given in JSON format. Since I wanted to broadcast photos and profile statistics, I immediately went to the “users” subsection. Authorization is not necessary for this.
Here it turns out that to get any data on the account, you need to know the ID of this account. Users only know their username, but not their ID. Where do you get it?
The identifier can be seen in the HTML code of the profile page, or you can send a request to this URL:
https://api.instagram.com/v1/users/search?q=LOGIN&client_id=CLIENT_ID
Where LOGIN is the desired login on Instagram, and CLIENT_ID is the key that we received during the registration of the application. As a result, we will get a JSON array, which, alongside the identifier, will also contain the user's avatar URL.
Next, to get a list of new photos from our profile, you need to send a GET request to this URL:
https://api.instagram.com/v1/users/USER_ID/media/recent/?client_id=CLIENT_ID
Where USER_ID is the identifier that we received from the previous request. You can also add additional arguments to the query. The list can be found on the documentation page.
Well, the last request for obtaining profile statistics:
https://api.instagram.com/v1/users/USER_ID/?client_id=CLIENT_ID
With data acquisition sorted out. We start implementation.
4. Widget implementation
I'll start with the bad news. The Instagram API is clearly focused on full-fledged applications, not simple widgets for sites. From here two problems follow:
- Only 5,000 requests per hour can be sent to the API from one CLIENT_ID or authorized user;
- It is undesirable for CLIENT_ID to be freely available, because Anyone can make requests to receive data on behalf of your application.
Because to view the widget it is stupid to ask for authorization from visitors, sending / receiving a request takes time, and our site has 700 thousand views per day (up to 80 000 requests per hour in the evening), you need to implement a data caching mechanism.
As a result, I decided that the widget should be rendered by the server. Therefore, the release will be in PHP + HTML + CSS.
We will connect the widget via iframe.
4.1 Caching:
The first version of the widget wrote the cache to the MySQL database, but then I changed my mind and transferred the cache to a file.
As a result, the speed of work is higher, there are fewer movements with the settings, and not everyone has MySQL.
The cache is stored in JSON format. Relevance is checked by the date of the last modification of the file. If the cache is outdated, then the API is requested to retrieve the actual data.
If an error occurs while sending a request, it is written into the cache as plain text with an explanation. If the cache is plain text, it is displayed instead of the widget. API requests will not be sent until the cache expires again. Thus, the widget will not load the server with regular requests to the API, if something goes wrong. And you can understand why the widget has stopped working.
4.2 Source Code:
We send cURL requests, write the data to the cache, draw the widget using HTML + CSS, write detailed instructions and work on makeovers for a few more sleepless nights. The result is this:
GitHub Repository>
The most interesting thing happens in the inwidget.php file.
config.php - is responsible for the settings, and template.php for the layout.
And, of course, everything is free. All for the people.
4.3 How to connect the widget to the site ?:
Instructions for steps:
- Register your site on Instagram (discussed at the beginning of the article).
- Download the widget source code.
- Upload the folder with the widget to the server.
- Set write permissions for the / inwidget / cache folder.
- Configure the widget settings (config.php file).
- Paste the widget into the site using the following code:
Insert examples with different widget display <iframe src='/inwidget/index.php' scrolling='no' frameborder='no' style='border:none;width:260px;height:330px;overflow:hidden;'></iframe> <iframe src='/inwidget/index.php?toolbar=false' scrolling='no' frameborder='no' style='border:none;width:260px;height:320px;overflow:hidden;'></iframe> <iframe src='/inwidget/index.php?width=100&inline=2&view=12&toolbar=false' scrolling='no' frameborder='no' style='border:none;width:100px;height:320px;overflow:hidden;'></iframe> <iframe src='/inwidget/index.php?width=100&inline=1&view=3&toolbar=false' scrolling='no' frameborder='no' style='border:none;width:100px;height:320px;overflow:hidden;'></iframe> <iframe src='/inwidget/index.php?width=800&inline=7&view=14&toolbar=false' scrolling='no' frameborder='no' style='border:none;width:800px;height:295px;overflow:hidden;'></iframe> <iframe src='/inwidget/index.php?width=800&inline=3&view=9&toolbar=false&preview=large' scrolling='no' frameborder='no' style='border:none;width:800px;height:850px;overflow:hidden;'></iframe>
To your liking, you can customize the insertion parameters, which are passed as GET parameters when accessing the script:
- width - the width of the widget (default 260px).
- inline - the number of photos per line (4 by default).
- view - how many photos to display in the widget (by default 12 pcs., max. 30 pcs., can be fixed in confing.php).
- toolbar - display a toolbar with an avatar and statistics (true / false values, true by default).
- preview - image size and quality (small - small up to 150px, large - large up to 306px, fullsize - full size up to 640px, small by default).
- lang - widget language (ru / en values, default settings from config.php are taken). The priority of this parameter is higher than for settings in config.php.
When changing the width or number of photos, remember to change the size of the iframe.
That's all.
Widget site:
http://inwidget.ru
GitHub repository:
https://github.com/aik27/inwidget
PS: I will be glad to feedback, comments and feedback.
Easter eggs!This is my cat. Each line of the widget is written under its vigilant control. Several times kote made adjustments to the process by running over the keyboard. Spend more time with your pets! Catch funny moments with them. Take more pictures!