If you are working on a Node.js application, then there is every chance that it will have some kind of API that will be used by you or someone else. Surely it will be a REST API and you will have a dilemma - what tools and approaches to use. After all, the choice is so wide ...
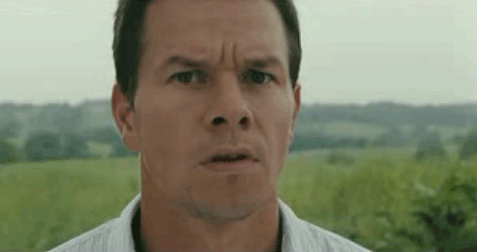
Thanks to the incredibly active community of Node.js, the number of results on NPM at the request of "rest" is off scale. Everyone has their own implementations and approaches, but some have something in common in creating a REST API on Node.js.
')
The most common approach is to give access to resources using Express. This will give an easy start, but later it will become more and more difficult.
ExampleUsing the latest version of Express 4.x Router, the resource will look something like this:
var express = require('express'); var Item = require('models').Item; var app = express(); var itemRoute = express.Router(); itemRoute.param('itemId', function(req, res, next, id) { Item.findById(req.params.itemId, function(err, item) { req.item = item; next(); }); }); itemRoute.route('/:itemId') .get(function(req, res, next) { res.json(req.item); }) .put(function(req, res, next) { req.item.set(req.body); req.item.save(function(err, item) { res.json(item); }); }) .post(function(req, res, next) { var item = new Item(req.body); item.save(function(err, item) { res.json(item); }); }) .delete(function(req, res, next) { req.item.remove(function(err) { res.json({}); }); }) ; app.use('/api/items', itemRoute); app.listen(8080);
pros | Minuses |
---|
- The low threshold of entry, Express, is practically the standard of Node.js applications.
- Full customization.
| - All resources must be created manually and, as a result, a lot of repetitive code will appear or worse - own library.
- Each resource requires testing or a simple check for the 500th error.
- Refactoring will become painful, as it will be necessary to edit everything and everywhere.
- There is no standard approach, you need to look for your own.
|
Express is a great start, but in the end you will feel the pain of “your own” approach.
Restify is a relatively old player on the Node.js API field, but very stable and actively developed. It is designed to build the correct REST services and is intentionally similar to Express.
ExampleSince it is similar to Express, the syntax is almost the same:
var restify = require('restify'); var Item = require('models').Item; var app = restify.createServer() app.use(function(req, res, next) { if (req.params.itemId) { Item.findById(req.params.itemId, function(err, item) { req.item = item; next(); }); } else { next(); } }); app.get('/api/items/:itemId', function(req, res, next) { res.send(200, req.item); }); app.put('/api/items/:itemId', function(req, res, next) { req.item.set(req.body); req.item.save(function(err, item) { res.send(204, item); }); }); app.post('/api/items/:itemId', function(req, res, next) { var item = new Item(req.body); item.save(function(err, item) { res.send(201, item); }); }); app.delete('/api/items/:itemId', function(req, res, next) { req.item.remove(function(err) { res.send(204, {}); }); }); app.listen(8080);
pros | Minuses |
---|
- DTrace support if the API runs on a platform that supports it.
- There is no such extra functionality as templates and rendering.
- Limit the frequency of requests (throttling).
- SPDY support
| The disadvantages are the same as for Express - a lot of extra work.
|
hapi is a lesser-known framework developed by the Walmart Labs team. Unlike Express and Restify, it has a slightly different approach, providing more functionality right out of the box.
Example var Hapi = require('hapi'); var Item = require('models').Item; var server = Hapi.createServer('0.0.0.0', 8080); server.ext('onPreHandler', function(req, next) { if (req.params.itemId) { Item.findById(req.params.itemId, function(err, item) { req.item = item; next(); }); } else { next(); } }); server.route([ { path: '/api/items/{itemId}', method: 'GET', config: { handler: function(req, reply) { reply(req.item); } } }, { path: '/api/items', method: 'PUT', config: { handler: function(req, reply) { req.item.set(req.body); req.item.save(function(err, item) { res.send(204, item); }); } } }, { path: '/api/items', method: 'POST', config: { handler: function(req, reply) { var item = new Item(req.body); item.save(function(err, item) { res.send(201, item); }); } } }, { path: '/api/items/{itemId}', method: 'DELETE', config: { handler: function(req, reply) { req.item.remove(function(err) { res.send(204, {}); }); } } } ]); server.start();
pros | Minuses |
---|
- Full control over the reception of requests.
- Detailed help with the generation of documentation.
| hapi, as well as Express and Restify, gives you great opportunities, but you have to understand how to use them.
|
Express, Restify and hapi are great solutions to start with, but if you plan to develop an API, then they can be a bad choice.
StrongLoop
LoopBack is a complete Node.js framework for connecting applications with data through the REST API. He adopts the mantra "
agreement in configuration ", which has become popular in Ruby on Rails.
Example
var loopback = require('loopback'); var Item = require('./models').Item; var app = module.exports = loopback(); app.model(Item); app.use('/api', loopback.rest()); app.listen(8080);
A lot of magic happens behind the scenes, but only six lines of code will create these resources for you:
GET /items GET /items/count GET /items/findOne GET /items/{id} GET /items/{id}/exists POST /items PUT /items PUT /items/{id} DELETE /items/{id}

To easily explore your API, just connect the built-in
explorer module:
var explorer = require('loopback-explorer'); app.use('/explorer', explorer(app, {basePath: '/api'}));
Now open
localhost : 8080 / explorer and get this cool documentation:

The example on LoopBack is quite simple, but what about the RPC resource?
var loopback = require('loopback'); var explorer = require('loopback-explorer'); var remoting = require('strong-remoting'); var Item = require('./models').Item; var app = module.exports = loopback(); var rpc = remoting.create(); function echo(ping, callback) { callback(null, ping); } echo.shared = true; echo.accepts = {arg: 'ping'}; echo.returns = {arg: 'echo'}; rpc.exports.system = { echo: echo }; app.model(Item); app.use('/api', loopback.rest()); app.use('/explorer', explorer(app, {basePath: '/api'})); app.use('/rpc', rpc.handler('rest')); app.listen(8080);
Now you can do this:
$ curl "http://localhost:8080/rpc/system/echo?ping=hello" { "echo": "hello" }
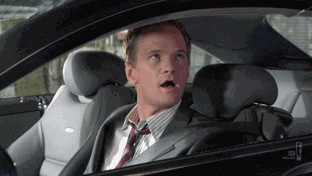
pros | Minuses |
---|
- Very fast REST API development.
- Arrangement in configuration.
- Built-in ready-made models.
- RPC support.
- Fully customizable.
- Extensive documentation.
- The team working on the project on an ongoing basis and is the main contributing company in Node.js.
- Commercial support.
| The threshold of entry is a bit high, as the framework consists of many details.
|
What's next