As part of a series of master classes from IT gurus that Luxoft Training organizes, we invite you to get acquainted with the translation of Adam Bien’s article “Integration Testing for Java EE”
about the authorAdam Bien, a consultant and author of books, is a member of the Java EE 6 and 7 Expert Group, EJB 3.X, JAX-RS, and JPA 2.X JSRs. Works with Java technology, starting with JDK 1.0, and with Servlets / EJB 1.0. He is currently an architect and developer in Java SE and Java EE projects. He edited several books on JavaFX, J2EE and Java EE. He is the author of the books Real World Java EE Patterns — Rethinking Best Practices and Real World Java EE Night Hacks — Dissecting the Business Tier. Adam has a Java Champion award, Top Java Ambassador 2012, JavaOne 2009, 2011 and 2012 Rock Star. Periodically he organizes Java EE workshops in the building of the Munich Airport (http://airhacks.com).
With lambda expressions and asynchronous communication support, JavaFX introduces new integration capabilities for server services.
')
You rarely see isolated applications in your organization. A corporate desktop application displays and manipulates data from one or more server services provided by the application server. At the time of Swing and J2EE, the message was unidirectional and synchronous. JavaFX and Java EE 6 and 7 introduced a variety of new synchronous, asynchronous, push and pull integration strategies. This article focuses on integrating Java EE services with Java FX applications.
JavaFX is Java
JavaFX is Java. Therefore, best practices that apply to Swing applications can also be applied to JavaFX. The integration of server services is independent of the protocols and technologies used.
Services have a variety of configurations, such as IP addresses, ports, and properties files. And API methods often throw protocol-specific exceptions such as java.rmi.RemoteException and, therefore, clutter the presentation logic with unnecessary details. A thin packer around its own service encapsulates implementation details and presents more significant interface details. This is the classic GoF Pattern Adapter.
Revival Business DelegateJ2EE clients relied heavily on Remote Method Invocation technology, based on the Internet Inter-ORB Protocol (RMI-IIOP), and later on the Java API for XML-based RPC (JAX-RPC) and the Java API for XML Web Services (JAX-WS) with server services. Both APIs heavily use controlled exceptions and are tied to a specific technology. The Business Delegate pattern was needed to separate the presentation logic and protocol:
“Use the Business Delegate to reduce the link between the presentation layer clients and the business services. The Business Delegate hides the implementation details of the business service, such as searching for and accessing the EJB architecture. ”
The Business Delegate was often extended by the factory method, which created real proxies in the default version and mock objects in the test environment. With modern mock-libraries, such as Mockito, Business Delegate can be simulated directly. A Business Delegate in the context of JavaFX and JavaEE can be implemented as a Plain Old Java Object (POJO) adapter, which encapsulates implementation details and provides a JavaFX friendly interface.

Picture 1
First the request, then the answer
Sending a blocking request to the application server and waiting for data to be received is the simplest possible integration with the server part. The Business Delegate becomes a service that communicates with the server part, as shown in Listing 1:
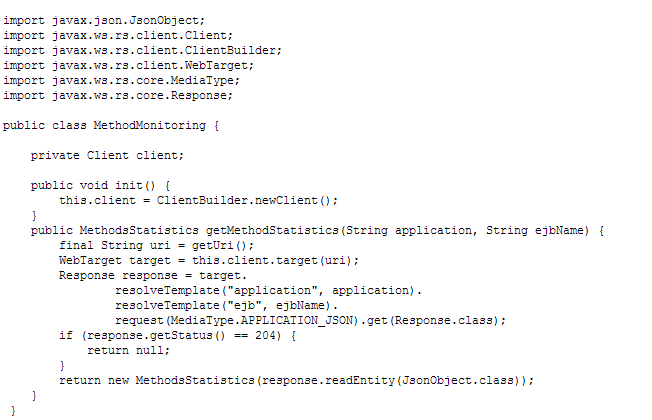
Listing 1
The MethodMonitoring class is easy to implement, test, and integrate with the presenter. Since the getMethodStatistics method can potentially block for an unlimited amount of time a synchronous call from the UI listener method, using it will make the UI unresponsive.
Asynchronous integrationFortunately, the JAX-RS 2.0 API also supports the asynchronous callback model. Instead of blocking, the getMethodStatistics method initiates a request and registers a callback (see Listing 2).

Listing 2
The callback transforms the incoming JsonObject into a domain object and passes it to the implementation of the java.util.function.Consumer function. The implementation of the Business Delegate is still independent of the JavaFX API and uses Java 8 java.util.function.Consumer as a callback. With Java 7, any customizable interface or class could be used as a callback. However, with Java 8, the implementation of the JavaFX presenter can be greatly simplified, as shown in Listing 3.

Listing 3
java.util.function.Consumer can be implemented as a lambda expression, which significantly reduces the amount of code. JavaFX is a single-threaded user interface development toolkit, so it cannot be accessed from multiple asynchronous threads.
The lambda implementation of the java.lang.Runnable interface is passed to the Platform.runLater method and added to the event queue for execution. As stated in Javadoc, “Run a specific Runnable on a stream of JavaFX applications at an arbitrary point in time in the future. This method, which can be called from any thread, puts Runnable in the event queue, and then immediately returns to the program (function, method) that calls it. All Runnables are executed in the order in which they are queued. The runnable passed to the runLater method will be executed before any runnable is passed to the subsequent runLater call. ”
The Platform # runLater method is not suitable for performing lengthy tasks; rather, it serves to update JavaFX UI components from an asynchronous stream.
Tasks for real workPlatform.runLater is not designed to perform difficult tasks, but is used to quickly update JavaFX nodes. An asynchronous call to long-term methods requires the creation of threads and is initially supported by the JavaFX javafx.concurrent.Task class. The Task class implements the Worker and EventTarget interfaces, inherits from the java.util.concurrent.FutureTask class, and can be seen as a bridge between Java threading and the JavaFX event mechanism. Task can either directly use Thread as a normal Runnable or be passed to ExecutorService as Callable. In any case, synchronous Java EE APIs without the possibility of asynchronous execution, for example, IIOP, can first be packaged into a Business Delegate:
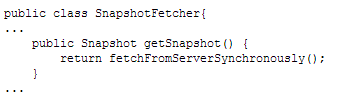
Listing 4
In the next step, the blocking Business Delegate method is packaged into a Task and becomes capable of asynchronous execution.
In the next step, the blocking Business Delegate method is packaged into a Task and becomes capable of asynchronous execution.

Listing 5
The Task class is Runnable and Future, so it can either directly execute Thread or be passed to the Executor. JavaFX comes with a javafx.concurrent.Service class that seamlessly integrates threading with the UI using the bindable bind property. Service is essentially a factory Task method:
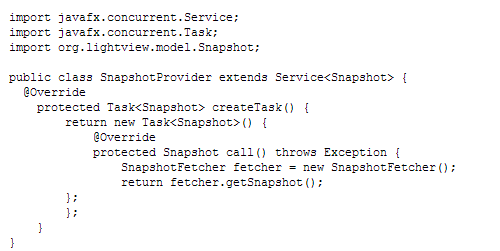
Listing 6
The service state, as well as the results of Task execution, becomes available as JavaFX bindable properties:
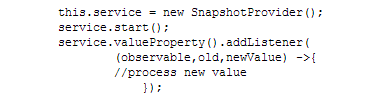
Listing 7
The Task class is a convenient tool for asynchronous integration of synchronous inherited resources or for executing lengthy processes on the client side.
Asynchronous blockingModel Comet and long polling (long survey) are not the best ways to simulate push HTTP communication with browsers. HTTP is the request-response protocol, so the response can only be transmitted in response to a request. Pushing data into the browser via HTTP without an initial request is thus not possible. Communication in the style of long-polling is easy to implement: the browser initiates a connection that is blocked by the server The server uses a blocking connection to transfer data to the browser, which immediately terminates the connection. The browser processes the data and re-initiates a subsequent blocking connection to the server. If there is no more information, the server sends a request 204 to the browser.
JavaFX applications are deployed in the enterprise as separate Java applications and, therefore, are not limited to HTTP communication. However, often REST endpoints are accessible to HTML5 clients and can be reused by JavaFX applications. REST and JSON are becoming a new low-grade way of communicating with HTML5 clients, Java applications and even low-level devices.
JavaFX applications can directly participate in long polling and receive notifications in the same way as HTML5 clients. The only difference between synchronous communication and long polling is the re-initiation of the blocking call. Repeated polling can be directly implemented via javafx.concurrent.Service. Regardless of whether it succeeds or not, the service will be reset and then restarted:
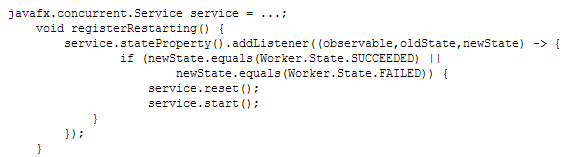
Listing 8
Push integrationPush-communication is a request-response style of communication, only without the request part; The application server can push data at any time. Java Message Service (JMS), WebSockets, and memory grids have a notification mechanism that works like a fire-and-forget principle, and can easily integrate with JavaFX.
JSR 356 implements the WebSocket protocol, is part of Java EE 7, and comes with a Java client API. The WebSocket specification introduces a bi-directional binary protocol and is ideal for integrating UI clients. WebSocket messages can have a binary or textual character and are obtained with a Endpoint subclass, as shown in Listing 9:
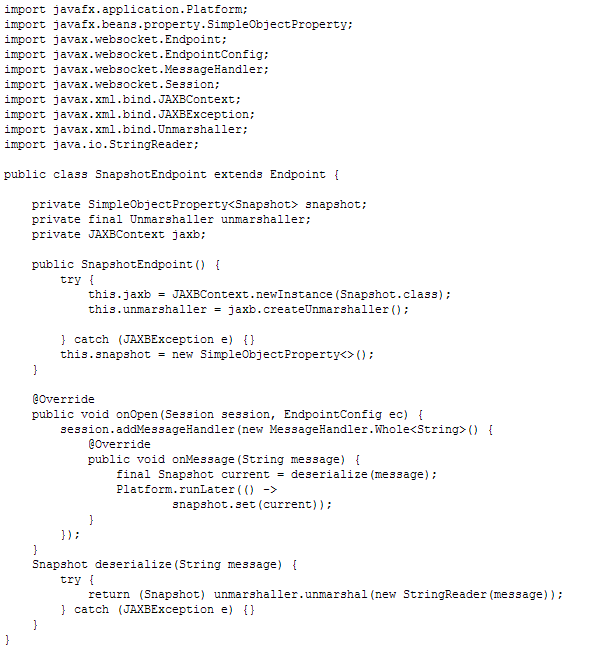
Listing 9
The SnapshotEndpoint class gets a string message and converts it using the Java Archite cture for XML Binding (JAXB) API. The Snapshot domain object is an annotated POJO:
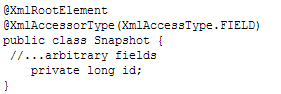
Listing 10
The JSR 356 API supports extensions, so serialization and deserialization can be moved to a dedicated class. We are also not limited to JAXB; You can use any available object presentation, such as JSON or serialization. SnapshotEndpoint runs on the client side with a dedicated WebSocket stream, so the message cannot be used only to update the UI. With the Platform.runLater method, the message is correctly transferred from the WebSocket to the JavaFX stream.
Endpoint is only responsible for the actual communication. In addition, the WebSocket container requires initial configuration and initialization for what is implemented in a dedicated class:
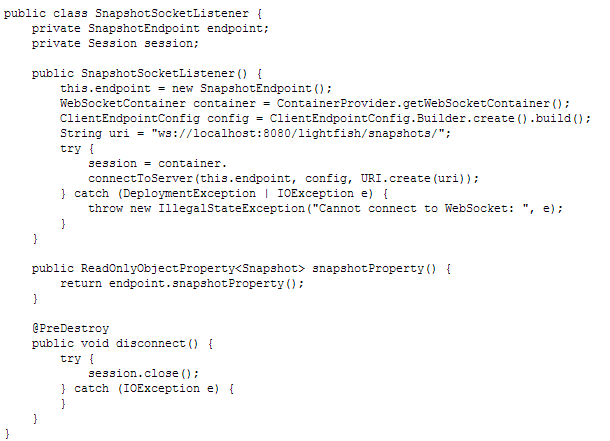
Listing 11
Until now, we practically did not use the integration capabilities of JavaFX, but only focused on various integration styles. However, JavaFX features make synchronous and asynchronous integration especially interesting.
JavaFX integration
The javafx.beans.property.ObjectProperty property wraps an Object instance and is bindable. An interested client can register as a listener or directly bind to the property and receive notifications when replacing a packaged instance. (See the “Binding for Narrow Interfaces” section of the Java Magazine article “JavaFX Data Binding for Enterprise Applications.”) Regardless of the communication protocol and synchronicity, the answer lies with the subject matter object that should be reflected by the UI. With ObjectProperty, the UI can simply bind directly to a value and receive automatic notifications when data is received. Presenter binds directly to ObjectProperty, which makes additional control methods redundant:
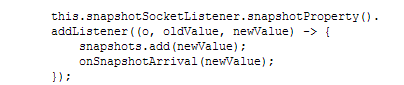
Listing 12
“Binding” ObjectProperty eliminates garbage and makes the interface extremely “narrow”. Binding can also be applied to outgoing communication. Presenter changes the state of the object or domain model, which leads to the notification of the service (Business Delegate). Outbound communication does not require synchronization with UI threads. Asynchronous operations can be directly performed from the UI thread, and long-running operations can either be packaged in a Service or asynchronously executed in a Business Delegate. However, not all UI operations change the state of the domain object. Simple user actions such as “save” or “refresh” can be directly passed to calls to the Business Delegate method.
Further increase responsiveness and simplificationJavaFX UI is event driven and is asynchronous. Also, Java EE 7 APIs, such as JAX-RS 2.0, JMS or WebSockets, have asynchronous capabilities. Using JavaFX in conjunction with the asynchronous Java EE 7 API greatly simplifies the code. All operations of the Business Delegate can be performed asynchronously without blocking the UI or even the Business Delegate itself. The interaction pattern is independent of the communication protocol and can be consistently applied to all asynchronous Java EE 7 APIs.
The request is transmitted to the server in the "started and forgot" mode. Instead of waiting for a response, the callback method is registered to handle the response. The callback receives the data, fills the domain object and replaces the current object using ObjectProperty # set in the Platform.runLater method. Changes in the domain object are translated into a presenter, which transmits a message about changes to all interested recipients.
Complete asynchronous communication significantly reduces the amount of code needed. Using the “launched and forgotten” approach in both directions with data binding eliminates the need to synchronize data between the temporal model and the ground state of the server side. All actions are sent directly to the Business Delegate and all requests from the application server directly update the UI.
Also, the interaction experience (UX) can be significantly improved with fully asynchronous interaction; No matter how expensive the interaction with the server part is, the UI will never be blocked.
ConclusionAt first glance, JavaFX integration with server services is very similar to Swing. Platform # runLater is equivalent to javax.swing.SwingUtilities # invokeLater and the content of javafx.concurrent.Service is very similar to javax.swing.SwingWorker.
Modern Java EE 7 APIs, JavaFX data binding (see “JavaFX Data Binding for Enterprise Applications”) and Inversion control capabilities with FXML and Scene Builder (see “Integrating JavaFX Scene Builder into Enterprise Applications”) allow us to significantly simplify the presentation of logic introduce consistent approaches to the implementation of desktop applications with multiple views.
With Java EE 6 and 7 server parts, you can continue to apply the asynchronous interaction style on the server side.
On June 3-5, Adam Bien’s master-class “Java EE: Architecture, Patterns and Solutions” will be held. Read more about the master class and conditions of participation
here .
