Recently, I began working in the field of web development, and am still in the Padawan stage. However, recently I discovered a way to organize client javascript code that can be easily integrated into any existing project and is easy to master.
This approach is called “Modular javascript”, and under the cat we will learn how to use it.
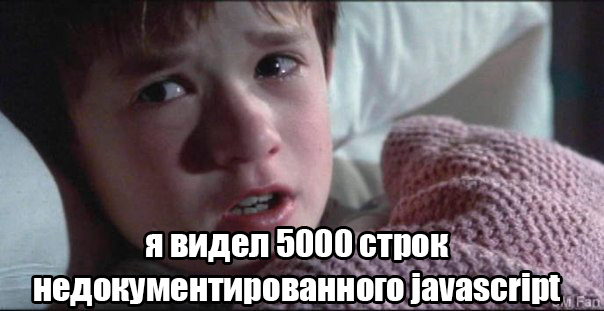
The article is so named because people at the Jedi level already use more advanced techniques and I think they will share them in the comments.
')
I set myself the following task:
"To organize all client js code in a convenient way, so that it would be easy to maintain, look for errors and complement."
The motivation for this was to work with someone else's site, where all the js were in one huge file and the attempt to add something caused a fit of apathy.
The whole essence of the technique comes down to splitting our application into modules. I also call them "widgets", because it's easier to perceive their essence.
Each widget is a separate entity. He does not know about other widgets and does not refer to them directly. A widget can only work with itself and generate events to which other widgets can subscribe.
Schematically, a widget is some part of our site that has specific functionality.

We can mentally break our test site into 3 widgets.
1. Global module - will be responsible for initialization of other modules.
2. Profile - displays the user's avatar (hi Richard :)), and a menu with areas of activity.
3. Portfolio - displays examples of work in the selected direction of this user.
Now let's create our modules.
Each module will be in a separate js file.
We will not consider HTML markup and CSS styles. Let me just note that for the display I usually use the template engine that is part of
underscore.js . And styles are mainly used from the main css file.
Global App.js Module
Profile.js profile module
var Profile = (function(){
Portfolio.js Portfolio Module
var Portfolio = (function(){
What does this give us
- The code is divided by files. Easy to find the right place to change.
- Modules communicate through events. You can remove or replace modules with other ones without touching the code of the rest of the application.
- The process of making new features has become more convenient
For example, we will want to add a new module that does something after the user has selected an item in the profile. It is enough for us to subscribe this module to the 'clickItem' event and perform the necessary actions.
We want to add a pop-up window that appears when you click on the work in the portfolio? No problem. In the event method of the Portfolio module, add something like
Now we need to sign the module that generates pop-up windows, throughout our entire application - to the 'showModal' event and that's it.
I hope this material will be useful to you.
On the topic as I advise you to read
largescalejs.ru .
I use
yepnope.js to upload files with modules.
Thanks for attention.