Dear habravchane!
I would like to tell you in more detail about one of the most interesting innovations in my opinion of the
recently held Build 2014 conference . It will be about the simultaneous development of applications for Windows 8 and Windows Phone, i.e. about
universal applications for the Windows platform .
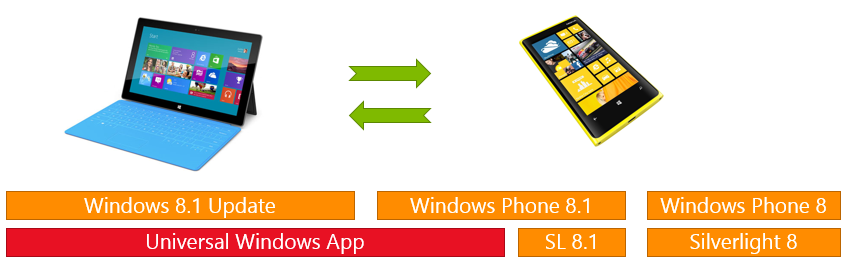
')
The Microsoft platform covers a wide range of devices - from smartphones and tablets to desktops and Xbox One gaming consoles, and it’s quite natural that the developer wants to minimize efforts when creating applications for all form factors. On competing platforms, there is a huge difference between desktop and mobile applications (since they are running different operating systems), while mobile applications designed for smartphones can work on tablet devices, which often leads to user dissatisfaction interface.
At the moment, Microsoft has come close to unifying all platforms (Windows Phone, Windows 8, Xbox One) from an API point of view, and allowing the programmer to maximize the use of common code when creating applications, while retaining the ability to use different designs for different forms. factors. Read more about how it is implemented at the moment - read below.
As previously created applications Windows + Phone
Until today, to create applications with common code for Windows and Windows Phone, we had to use a shared portable library to isolate the common code responsible for data access and business logic, and various projects for the UI. More such an approach is described in a
special course on Microsoft Virtual Academy , or
in this post on Habré . Also, due to the difference in the Windows 8 and Windows Phone APIs, we had to make part of the code platform-dependent.
Universal Windows Applications
At the build conference, the following innovations were announced:
- The new version of Windows Phone 8.1 will use the Windows RT API. This means that about 90% of the system calls between Windows 8.1 and Windows Phone 8.1 will be common. In addition, the XAML markup language was also unified between the platforms. In other words, the new Windows Phone 8.1 applications will use Windows XAML, not Silverlight. If you need compatibility, Windows Phone can still be developed using Silverlight, incl. using new features, but this is a topic for a separate article.
- In Visual Studio 2013 Update 2, a new project template for unified Windows applications will appear . This template creates various projects for Windows and Phone, and a third “shared” project in which all common code is placed. In this case, the shared project
may contain not only code, but also XAML markup, shared resources, images, etc. This project is not compiled into a separate library, but is divided between two platform projects at the level of text inclusion at the compilation stage. Such a template can be used for development in C # / XAML, C ++ / XAML or HTML / JS. - If you want to isolate part of the platform-independent code into a separate library shared between several applications, you can still use a portable library, which can now also include XAML markup . Portable libraries can be used to develop in C # or Visual Basic.
- There is no binary compatibility between the platforms , i.e. Windows 8 and Windows Phone applications will continue to be distributed through the respective stores, and the developer will need to create and download application packages into each store (although now Windows Phone 8.1 will use the same .appx format as Windows 8. However, in stores Windows and Windows Phone will use common application identifiers , which will allow implementing a single application purchase scenario for use on all platforms .
- Xbox One apps in the current version of Visual Studio Update 2 do not fit into the overall story as well, although the plenary report showed the Khan Academy universal app using Kinect running on Xbox and Windows (yes, Kinect v2 will be supported in Windows store apps but this is again a topic for a separate article). Development for Xbox One is currently assumed to be HTML / JS / CSS and C ++
Thus, now there is a convenient opportunity for developers to create applications for Windows and Windows Phone platforms, which contain a significant amount of common code, with the ability to customize the design for different platforms to maximize user satisfaction!
Universal hello world
Consider a small example of creating a universal application. The structure of projects in Visual Studio 2013 Update 2 has been changed, and now in the Window Store section are available both applications for Windows and Windows Phone, and universal applications and libraries.

The newly created universal application will be designed for the Windows Phone 8.1 platform and Windows 8.1 Update. At the same time, in the Windows Phone applications section, there are Silverlight-based Windows Phone project templates that allow you to create applications for earlier versions of the platform — but you cannot use the capabilities of universal applications.
After creating an empty universal application, we get the following structure consisting of three projects: one project for each platform and a common shared project:

Note:
- The default page design (XAML) for platforms is separated by different projects. However, in simple cases, you can use common XAML files for all platforms, if you are confident that your design will adapt well enough to different resolutions, from smartphone to desktop. However, many built-in controls (for example, GridView) are able to adapt and change their appearance depending on the platform.
- If you have a ready-made Windows or Windows Phone project, you can create a universal application based on it by selecting the corresponding item in the context menu of the project. At the same time, the project will be transformed into the same three-project structure, and you will be able to transfer application files to a common project for their sharing.
- References to libraries can be included in a distributed project, and these links will be added to both projects (we see that the Shared-link is in the links of each of the platform projects). If some libraries are available only for one of the platforms, then we can still use the corresponding functionality in the common code, surrounding it with conditional compilation directives #ifdef. Visual Studio is so convenient that Intellisense will work in this case, warning us that the link is only available in one of the platforms.
- If we bring the XAML code into a common project, then in the XAML editor a drop-down is available to switch the platform, and we can visually edit the page design both in the phone mode and in the tablet / desktop mode.

In most cases, you will want to share as much code between platforms as possible, transferring all that is possible to the shared project. In our case, we can transfer MainPage.xaml from one of the projects to the shared project, and delete it in the platform projects, since in our case the page design will not differ from platform to platform:
<Grid Background="{ThemeResource ApplicationPageBackgroundThemeBrush}"> <TextBlock Style="{StaticResource HeaderTextBlockStyle}" HorizontalAlignment="Center" VerticalAlignment="Center"> Hello, world! </TextBlock> </Grid>
Thus, we got a universal application, the code and design of which are completely in a shared project.
On the way to the real application - Photo Viewer
Let's try to turn our Hello World application into something useful - for example, into the best flickr photo viewer. Flickr provides an RSS feed for photos, so it’s relatively easy to determine the appropriate data source (for the sake of simplicity, RSS downloads are non-asynchronous, in real projects you don’t need to do this):
Flickr Image Code public class Flickr { List<BitmapImage> list = new List<BitmapImage>(); public Flickr() { var xdoc = XDocument.Load("http://api.flickr.com/services/feeds/photos_public.gne"); XNamespace xn = "http://www.w3.org/2005/Atom"; var res = from z in xdoc.Descendants(xn + "entry") let l = (from x in z.Descendants(xn + "link") where x.Attribute("rel").Value == "enclosure" select x.Attribute("href").Value).FirstOrDefault() where (l!=null) && (l!="") select l; foreach (var x in res) { list.Add(new BitmapImage(new Uri(x))); } } public List<BitmapImage> Images { get { return list; } }
On the main page we use GridView, attached to this data source. In order for the photos on different platforms to be of different sizes, we use the key from the resource file, which determines the required size of the photo.
XAML-design of the main page of the application <Grid Background="{ThemeResource ApplicationPageBackgroundThemeBrush}"> <GridView ItemsSource="{Binding Images}"> <GridView.DataContext> <local:Flickr/> </GridView.DataContext> <GridView.ItemTemplate> <DataTemplate> <Image Height="{StaticResource ImageSize}" Width="{StaticResource ImageSize}" Source="{Binding}"/> </DataTemplate> </GridView.ItemTemplate> </GridView> </Grid>
To set different parameters in the resource file, create in each of the platform projects your own resource file Resource.xaml with the following content:
<ResourceDictionary xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation" xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml" xmlns:local="using:UniversalHello"> <x:Double x:Key="ImageSize">150</x:Double> </ResourceDictionary>
And finally, we need to include this resource file in App.xaml (which is in the shared project):
App.xaml <Application ...> <Application.Resources> <ResourceDictionary Source="Resources.xaml"/> </Application.Resources> </Application>
As a result, we get a couple of applications for Windows 8 and Windows Phone, which correctly display the image gallery taking into account the specifics of the platform.

The full source code of the application can be
obtained at github .
Morality
To create new applications on the Windows 8 platform, the best solution now will be to use universal applications. If you have an existing Windows 8 application, then it makes sense to slowly convert it into a universal application and port it to Windows Phone 8.1. It is more difficult to convert an existing Windows Phone 8 application into a universal application (since other API sets are used for a number of operations), we'll talk about this later. Finally, universal applications for Windows Phone require a version of Windows Phone 8.1, so at the current moment, in order to have a fairly wide install base, it makes sense to use Silvelight 8.0 applications.
Materials
Build reports on:
Download Visual Studio 2013 Update 2 RC