I share my experience gained in studying the task in the title. The article discusses two options for work that can be used in Python programs that access the Raspberry Pi GPIO ports.
The GPIO Raspberry Pi ports look like this:
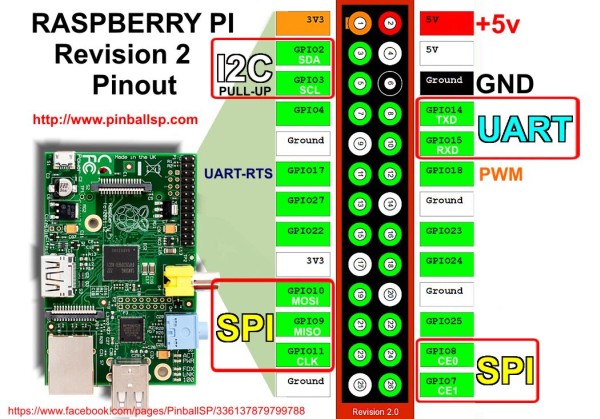
')
One of the options for working with GPIO is the
Sysfs interface. Learn more about Sysfs GPIO interface
here . In this case, read and write files are used to access the GPIO ports. To use a specific output, you need to reserve it and set the direction to the input or output. After you finish working with the port, you need to release it so that other processes can use it.
Port reservation (XX - required port number):
$ echo XX > /sys/class/gpio/export
If the backup is successful, a new folder appears at
/sys/class/gpio/gpioXX/
. Setting the direction is as follows:
$ echo "out" > /sys/class/gpio/gpioXX/direction
Or so:
$ echo "in" > /sys/class/gpio/gpioXX/direction
Setting the high and low levels for the port configured for output is as follows:
$ echo 1 > /sys/class/gpio/gpioXX/value $ echo 0 > /sys/class/gpio/gpioXX/value
Determining the status of the port configured for input is done like this:
$ cat /sys/class/gpio/gpioXX/value
Release of the port after its use ends:
$ echo XX > /sys/class/gpio/unexport
To simplify working with GPIO through the Sysfs interface, I encountered two utilities. One of them is the
WiringPi GPIO utility , the other is
quick2wire-gpio-admin .
The main task solved by these utilities is to provide the ability to work with GPIO on behalf of an unprivileged user. At the same time, the
WiringPi GPIO utility is more functional.
To work with GPIO, I stopped at the
RPIO package (at the time of writing, the latest version was 0.10.0). This package works with GPIO ports directly, referring to the BCM2708 registers via a special device
/dev/mem
. What is RPIO:
Advanced GPIO for the Raspberry Pi. Extends RPi.GPIO with PWM, GPIO interrups, TCP socket interrupts, command line tools and more
RPIO is positioned as an improved and expanded version of another module, RPi.GPIO. The documentation directly on the RPIO discusses in detail only the features that are missing in RPi.GPIO; therefore, for a description of the functions common to these modules, refer to the RPi.GPIO documentation available
here .
Install the package with the following command:
$ sudo pip install RPIO
In addition to the python modules, I install two programs: rpio-curses and rpio. With their help, you can, for example, get the current mode and state of the GPIO and, if desired, change them. This is how working with rpio-curses looks like:

So you can get information about the system:
$ rpio --sysinfo 000f: Model B, Revision 2.0, RAM: 512 MB, Maker: Qisda
An example of a python program using RPIO:
import RPIO import time NRF_CE = 24