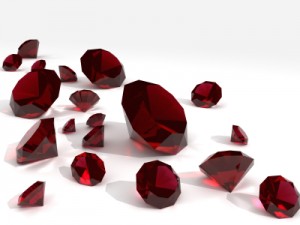
This article describes some interesting tricks on how to effectively use and work with arrays in Ruby. Of course, there is RubiDoc and many other resources, where all the available methods are described in detail, but here I want to share ways of use.
1. How to check or array contains all elements of another array
For example, you want to check or all the imported e-mails are already in the contact list:
imported_emails = [ 'john@doe.com', 'janet@doe.com' ] existing_emails = [ 'john@doe.com', 'janet@doe.com' , 'fred@mercury.com' ] puts 'already imported' if (imported_emails - existing_emails).empty?
Run in IRB :
already imported
=> nil')
2. How to find common elements in two arrays
For example, if you need to find common tags in two different posts:
tags_post1 = [ 'ruby', 'rails', 'test' ] tags_post2 = [ 'test', 'rspec' ] common_tags = tags_post1 & tags_post2
Run in IRB :
=> ["Test"]3. How to connect two arrays without duplicating duplicate elements
followeds1 = [ 1, 2, 3 ] followeds2 = [ 2, 4, 5 ] all_followeds = followeds1 | followeds2
Run in IRB :
=> [1, 2, 3, 4, 5]4. How to sort an array of hashes
For example, you get data from some API, in the form of an array of hashes:
data = [ { name: 'Christophe', location: 'Belgium' }, { name: 'John', location: 'United States of America' }, { name: 'Piet', location: 'Belgium' }, { name: 'François', location: 'France' } ]
When displaying, you want to sort by the location field, then do this:
data.sort_by { |hsh| hsh[:location] }
Run in IRB :
=> [
{: name => "Christophe",: location => "Belgium"},
{: name => "Piet",: location => "Belgium"},
{: name => "François",: location => "France"},
{: name => "John",: location => "United States of America"}
]5. How to get unique elements of an array on a certain basis
For example, you want to show random products on the main page, but that each product is taken from only one category:
Product = Struct.new(:id, :category_id) products = [ Product.new(1, 1), Product.new(2, 2), Product.new(3, 3), Product.new(4, 1), Product.new(5, 3), Product.new(6, 5), ] products = products.uniq &:category_id
Run in IRB :
=> [
# <struct Product id = 1, category_id = 1>,
# <struct Product id = 2, category_id = 2>,
# <struct Product id = 3, category_id = 3>,
# <struct Product id = 6, category_id = 5>
]
6. How to filter string elements of an array
For example, you may have an array with the names of books that you want to filter for some keyword:
books = [ 'The Ruby Programming Language', 'Programming Ruby 1.9 & 2.0: The Pragmatic Programmers\' Guide (The Facets of Ruby)', 'Practical Object-Oriented Design in Ruby: An Agile Primer', 'Eloquent Ruby', 'Ruby on Rails Tutorial: Learn Web Development with Rails' ] books = books.grep(/[Rr]ails/)
Run in IRB :
=> ["Ruby on Rails Tutorial: Learn Web Development with Rails"]7. As always return an array
For example, you have a method that can return a list of products or a single product. To ensure that the return value is always an array, you can use Array () or [*]:
def method