
In the
first part, I explained how to create a RESTful API in the Windows Azure cloud and how to start using it in my mobile application. I recommend reading this article, since this text and examples largely rely on it.
In the second part of the article on the development of cloud backend for mobile applications, I will talk about how to maximize the benefits of team development API, how to write once, and use a lot, and how to use the help of the community. Let's get started
')
Windows Azure and Git
As you have already heard wrong, Microsoft, to its credit, has recently increasingly turned its attention to Open Source technology. For example, in the latest version of
Visual Studio 2013 , support for the
Git version control system was introduced out of the box. The Windows Azure cloud also does not stand aside, and now it allows you to work with the code not only through TFS, but also with the help of Git and other systems (SVN, Dropbox, Bitbucket).
Windows Azure Mobile Services decided to go ahead and leave only Git to work. Perhaps this is so only for the time of the Preview-version of this solution, but, given the tendencies of the corporation, this option may move to the release.
What gives us the use of Git in your project? Yes, everything is exactly the same as always. Namely - the joint work on the code, local repositories for each team member, support for versioning. But what is more important is the observance of the principle of continuous integration - continuous deployment of the solution with each new code in the repository. Every time someone from a team member sends their changes to the server, they are deployed in the cloud. All this works on
Kudu (by the way, another open source project), and quite successfully.
Enabling Git in your service
In order to start using Git to work with Mobile Services, you need to go to the control panel of this service and select the appropriate item:

You will be asked if you are sure of your action and will warn you that while the function is in the Preview stage, some malfunctions are possible. We agree and continue.
After the creation of the repository is completed, you can see the parameters for accessing it on the Configure page in the service control panel. In the Source Control section there will be two parameters: the reporter access URL and the URL that will launch the deployment of your solution:

After completing the creation operation, your cloud Git is ready for work Let's get the files that are stored on the server in your local repository. To do this, you can use the standard Git console (for example,
Git Bash for Windows ).
The server repository is cloned locally using the command
git clone <URL_>

You must specify the URL specified in the
GIT URL parameter in the cloud service config. You will also need to enter the username and password of the Git user (if you did not have it, then when you first create a Git repository, WIndows Azure will suggest to come up with this pair). As a result, it should turn out approximately as in the picture below:

Repository structure
As soon as the command is completed, a directory with the name of the mobile service will appear on your hard disk. Inside it will be the
service folder and the
.deployment file. The file is not of interest, but inside the
service folder you should look.
$ ls api extensions package.json scheduler shared table
If you pay attention to the names of the folders that are inside the service, you can trace the pattern that all of them are named the same as the Windows Azure mobile services subsystems (API, scheduler and tables), plus two directories called
extensions and
shared .
API Directory
The API directory is responsible for storing files that describe the Custom API code (what we created in the
first part ). If you go to the directory and see its contents, then there should be three files:
$ ls coolapi.js coolapi.json readme.md
Apart from the readme.md file, which is in each folder and simply describes its contents, js-scripts are stored here, one for each API that you have. Also, for each script with code, there is one .json file that describes the access rights to a specific API. Let's look at the contents of these files. Below is the contents of the
coolapi.js file:
exports.post = function(request, response) {
As you can see, the file is exactly the same as the code we wrote in the previous article directly in the Windows Azure Control Panel. And this is understandable, because behind each API there is a simple js-script that works in the Node.js application.
The coolapi.json file is a bit more interesting, since we have previously only seen it indirectly and not in this form:
{ "routes": { "*": { "get": {"permission":"application"}, "post": {"permission":"application"}, "put": {"permission":"application"}, "patch": {"permission":"application"}, "delete": {"permission":"application"} } } }
In fact, this is nothing more than a description of the access rights to a specific API, which we asked at the very beginning when it was created (though we did this with the help of a wizard in the management portal). Here for each HTTP method these or those restrictions are registered. In our case, the
application type is set for all methods, which means that only those users who have an application key have access to these methods. Valid values are also:
- public - access is allowed for everyone (analogue Everyone on the portal)
- user - access is allowed only to registered users (equivalent to Only Authenticated Users)
- admin - access is allowed only to administrators (equivalent to Only Administrators)
If you now change anything in the text of any of these files, and then save it to the repository, then all changes will immediately appear in the management portal (and therefore will be available to all your users). Let's, for example, change the
Hello World string to
Hello from Git in one of the methods, and make the GET method available to everyone (public), and send corrections to the cloud:
git commit . -m "My first Git commit" git push
The result will look something like this:

If you look at the logs of the operation, in addition to simply copying files to the server, work is done to deploy the solution using Kudu.
Now, if you go to the mobile services management portal and see the code for this API, you can see the changes made:

And if you access the API through a browser (which became possible after we relaxed the restrictions for the GET method), make sure that the changes are accessible from the outside:
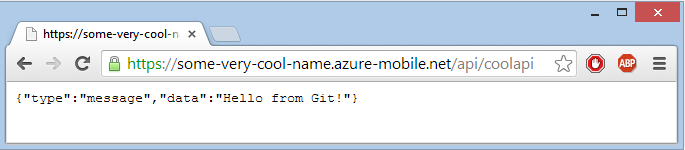
TABLE directory
In this folder, as you can guess from its name, should be kept something that relates to the tables that are in Mobile Services. But it is not the data of the tables themselves that are stored here (which is logical for Git), but the code that controls the insert / delete / change events of the records (for more information about working with tables, you can read
here and
here ). I created a table with the name MyTable in advance and slightly corrected the script that works during the insert operation. And this is what I ended up with in the table folder:
$ ls MyTable.json mytable.insert.js readme.md
Following the logic of naming files in mobile services, you can come to the logical conclusion that the json file stores information about access rights to the table (and this is so, this file is similar to what we saw in the API), and the js-file stores code. Moreover, the file name is formed from the name of the table to which it belongs, and the operation for which it is defined. Thus, if you create a similar file directly on your local computer (call it, for example, mytable.update.js), write code in it and commit it to the server, these changes will be immediately available in the management portal, without the need to manually create a handler update operations.
SHARED directory
Scripts that do not belong to a particular part of mobile services, but, on the contrary, can be used everywhere can be stored here. Something like library code storage within a single service. To use this code, you must, first, write it (and you can only do it through Git, since there is no direct access to these scripts from the control panel), and second, connect a new module in the existing code.
Here is a simple example. Create a file in the shared folder with the name
myshared.js and the following contents:
exports.someSharedMethod = function () { return 'Hello from Shared!'; }
Now in the API code we use this module by adding the following lines of code:
exports.get = function(request, response) { var myshared = require('../shared/myshared.js'); response.send(statusCodes.OK, myshared.someSharedMethod); };
If you have already worked with Node.js, this code will be familiar to you. This is the standard mechanism for connecting external modules to your application. By and large, the
shared folder is just a directory on the hard disk where it is recommended to store common modules. No magic, everything is fair.
Other directories
About the remaining two directories, you can say about the same as about the previous ones: the code is stored in them. The
scheduler folder will contain scripts for managing the corresponding service, and the extensions folder contains code that can be connected to the entire Node.js mobile services application and that will run exactly once when it starts. At the same time it is guaranteed that all other operations in the application will be suspended until the extension code is completed. This can be useful if you want to make some special settings in the database every time the mobile services are restarted.
NPM packages
If we now understand that behind the scenes of mobile services the application is running on Node.js, then it is logical to ask - is there NPM? For those who don't know,
NPM is a package manager for Node (something like NuGet or Linux stuff). This is a very useful thing when developing applications, because it allows in a matter of seconds to connect tons of third-party libraries developed for various purposes (error handling, logging, template types, etc.) to your project.
Fortunately, you can work with NPM in Windows Azure Mobile Services. To do this, you need to edit the package.json file (which is on the same level of the file structure as the api, table directories, etc.). By adding the necessary modules to this file (in the manner standard for Node.js, in the
dependencies section) and committing this file back to the server, we will have Windows Azure download them for our application. It only remains to connect them through require and you can get all the benefits from the creations of the community.
Conclusion
The PaaS platform, which is in Windows Azure, provides very rich opportunities for those developers who need a fast and high-quality solution and who do not really want to spend a lot of effort on infrastructure deployment and administration. Windows Azure Mobile Services provide rich functionality of a wide purpose. And the Custom API, in particular, helps to avoid code duplication problems when you need to develop some common solutions for various platforms.
What is additionally good and honorable for Microsoft is that the company decided to meet current trends and partially abandoned the use of proprietary technologies in favor of Open Source and cross-platform. If you notice, all the things that I showed in this article can be done absolutely on any computer, be it PC or Mac, Windows or Linux. And the presence of native cross-platform libraries for all three key mobile devices (and REST API support for most of their services) make it possible to use this cloud from almost anywhere. In my opinion, this is an excellent approach to doing business and a great reserve for the future.
PS Finally, I would like to note that since the work with Git repositories in mobile services is still in the preview stage, something may be unstable, but something may change with time.
PPS The material in this article is also available as a
YouTube video tutorial or a course on the
Microsoft Virtual Academy .