#FlappyBird - And that says it all!
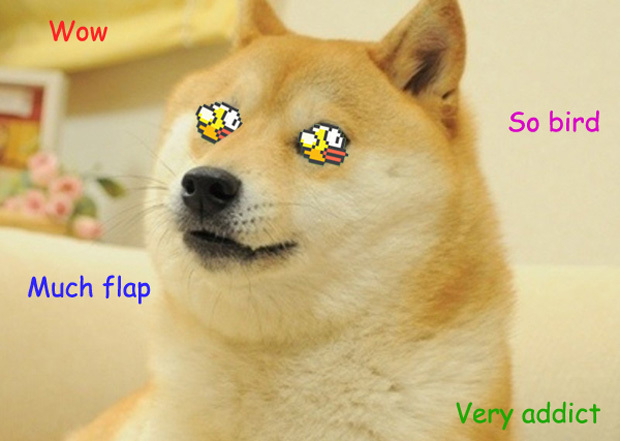
This toy is incredibly simple, but at the same time contains a good mix of entertainment and resentment inherent in the games of the 90s, such as Double Dragon 3, and Teenage Mutant Ninja Turtles. After
Dong Nguyen announced that he was going to remove the game from the app store, I wondered how difficult it was to recreate physics and interaction with the help of the new iOS7 physics engine. I wrote this article because many developers do not even know that iOS 7 has a built-in physics engine - UIKit Dynamics.
1. Wings
The implementation contains three steps. First, you need to create a recognition of the touch gesture:
')
UITapGestureRecognizer *singleTapGestureRecognizer = [[UITapGestureRecognizer alloc] initWithTarget:self action:@selector(handleSingleTapGesture:)]; [self.view addGestureRecognizer:singleTapGestureRecognizer]; [singleTapGestureRecognizer setNumberOfTapsRequired:1];
The next stage we need to create a wave of "birds". Create a constant vector
flapUp
(up):
flapUp = [[UIPushBehavior alloc] initWithItems:@[self.block] mode:UIPushBehaviorModeInstantaneous]; flapUp.pushDirection = CGVectorMake(0, -1.1); flapUp.active = NO;
And finally, add gravity to the “bird”.
gravity = [[UIGravityBehavior alloc] initWithItems:@[self.block]]; gravity.magnitude = 1.1;
This is how our “bird” will move:

Moving tubes
The implementation of the pipes is also quite simple. We need to create them outside the screen and start moving from the right to the left with negative force X. Create pipes and give them density.
pipesDynamicProperties= [[UIDynamicItemBehavior alloc] initWithItems:@[topPipe, bottomPipe]]; pipesDynamicProperties.allowsRotation = NO; pipesDynamicProperties.density = 1000;
The pipes are created, and now let them run around the screen.
movePipes = [[UIPushBehavior alloc] initWithItems:@[topPipe, bottomPipe] mode:UIPushBehaviorModeInstantaneous]; movePipes.pushDirection = CGVectorMake(-2800, 0); movePipes.active = YES;
And it will look something like this:
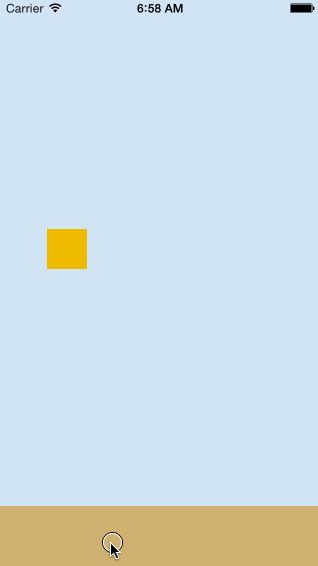
Dying at collisions
The final step will be to add a loss moment when colliding with objects. It is worth paying attention to the left border on the screen. In the event of a collision, it removes the pipes and also causes the code to generate new ones.
blockCollision = [[UICollisionBehavior alloc] initWithItems:@[self.block]]; [blockCollision addBoundaryWithIdentifier:@"LEFT_WALL" fromPoint:CGPointMake(-1*PIPE_WIDTH, 0) toPoint:CGPointMake(-1*PIPE_WIDTH, self.view.bounds.size.height)]; blockCollision.collisionDelegate = self;
Add a collision between the “bird” and the ground.
groundCollision = [[UICollisionBehavior alloc] initWithItems:@[self.block, self.ground]]; groundCollision.collisionDelegate = self;
And in the end, after the pipes are created, we have to add a collision between the pipes and the “bird”.
[blockCollision addItem:topPipe]; [blockCollision addItem:bottomPipe];
Since the pipes and the ground are one and the same
self
delegate, all that concerns the “bird” causes “Game Over”. Now collisions are added between the “bird” and the ground, as well as the “bird” and dynamically created pipes. Here's what it looks like:
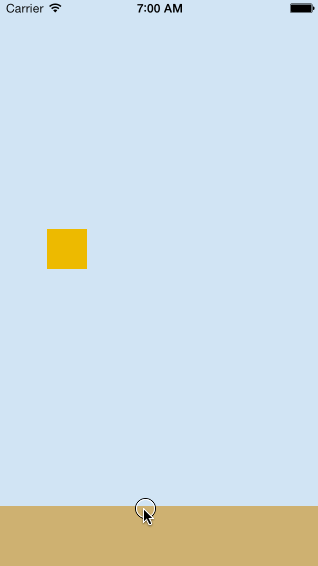
Source codes are available on GitHub under the MIT license for those who want to learn how to use the iOS7 physics engine. You can see the source here:
github.com/joeblau/FlappyBlock