This article is a translation of the second part of articles devoted to the use of Java on the Raspberry Pi. The translation of the first part caused great interest in the possibility of using Java on the Raspberry Pi. The original of this article was published in the 16th issue of MagPi .This is the second article about using Raspberry Pi for programming in the Java language. After reading it, you will learn more about the constructions of this language and can try to write more complex programs. It may also be useful to those who want to learn about the C language, since the basic syntax of Java coincides with the syntax of C.
At the beginning of this article I will describe the control structures in more detail. And then I will move on to the description of numeric, string and logical data types, giving examples of their use. Given all this, more complex execution logic will be added to our sample programs. Well then, let's get to work!
More about control structures
')
In the
first article published in
MagPi # 14, we met two control structures:
if and
while . I know that you remember this, but just in case I remind you. The block
if construction will be executed only if the value of the logical expression in parentheses is true. For example:
if (a > 3) { c = c + a; }
We add a and c only when the value of a is greater than 3. The expression in parentheses, called a state, can be
true (true) or
false (false), i.e. data type is
boolean (For more information about the logical data type below, see the article).
The
if construct can include a block that runs when the status is
false . For example:
if (score > 50) { System.out.println("You won! :)"); } else { System.out.println("You lost. :("); }
The next construction we examined was
while . A block of a
while construct can be executed only in cases when the state of the expression in brackets is
true , i.e. it may be one or more times, and maybe not once. For example:
int f = 10; while (f < 20) { System.out.println(f); f++; }
Integers from 10 to 19 will be printed until the variable
f becomes equal to 20 and the result of the expression changes to
false . Also, did you notice the red string? Well, if noticed. Two plus signs after the variable
f increase it by 1. This is a short form of writing:
f = f + 1;
Similarly:
f--;
Minus signs decrease the value assigned to the variable
f . Otherwise it will look like this:
f = f - 1;
Now let's look at the
for construction. The
for construct is similar to
while , but for it in one line you can combine execution criteria and an expression that changes the value of a variable. Therefore, the enumeration of numbers from 10 to 19 can be written using
for :
for (int f = 10; f < 20; f++) { System.out.println(f); }
Yes, this option looks more compact than the example with
while . However,
while , in some cases, it may be more convenient to execute loops.
The section in brackets for the for construction is divided into three parts. The first to initialize the variable at the beginning, the second to check the variable at each iteration of the loop, the third to change the variable at the end of each loop.
Now open your editor and create a new program:
public class Countdown { public static void main(String[] args) throws InterruptedException { for (int t = 10; t >= 0; t--) { System.out.println("-> " + t); Thread.sleep(1000); } System.out.println("-> Fire!"); } }
We compile it with the
javac command and run
java (more details in the
previous article ). This program will count 10 seconds after which it prints "Fire!". How does the program change the variable
t ? Double minus reduces the value of
t from 10 to 0. But then why
did you need the
Thread.sleep (1000) line; ? It creates a delay of 1 second (1000 milliseconds) in the program at each step of the cycle. Using the
Thread.sleep method, you only delay within the
for block. Now let's leave everything as it is, I will tell you about exception handling later.
Complicate task # 3 : Create a new program, Countup.java, for an account from 1 to 20 with a delay of one second. And when the bill reaches 15, we display the message “Five to go ...”.
And finally, take another look at the
switch . The
switch construct checks the values ​​of a variable and, depending on what the variable in parentheses is equal to, executes the code after the colon. Example:
import java.util.Random; public class CardinalDirection { public static void main(String[] args) { Random r = new Random(); int dir = r.nextInt(4); switch (dir) { case 0: System.out.println("North"); break; case 1: System.out.println("West"); break; case 2: System.out.println("South"); break; default: System.out.println("East"); break; } } }
In this example, an integer is randomly generated in the range from 0 to 3. But, interestingly, the value of the number is not shown, it is needed only for reference. Each of the blocks is executed only if the variable value matches the value after the
case . Notice I did not set the
case for the number 3, I used the
default construction. This is a good example of the fact that it is always better to set
default , so this block of commands is selected for any non-matching value.
Well, well, that's all I wanted to tell you about control structures. Of course there are other more exotic constructions, such as
continue or
do-while , but they are not so often used and I will leave them to you, for independent study.
Well, now you can move on to a completely different Java learning topic.
A pair of bits about numbers
Sooner or later, you will need mathematical calculations, in this chapter are given the basics with which you will need to begin to learn. In Java, each numeric variable is one of the basic language types. In Java, four integer types (without decimal places), two floating point (with decimal places), and two high precision. For use in a program, you declare a variable once, then change its values ​​as the program runs. For example:
int trees;
But you can immediately declare a variable to assign a value to it, for this we combine two lines into one:
Have you noticed
// ? Two slashes mark the beginning of a comment, Java ignores all that comes after them. In this way, after them you can leave any notes and reminders on the program execution, so that in a couple of months or even years you can remember how your program should work.
And now the range of available values ​​for the four types of integer variables:
byte: -128 127 short: -32 768 32 767 int: -2 147 483 648 2 147 483 647 long: -9 223 372 036 854 775 808 9 223 372 036 854 775 807
More often you will need a third type (
int ). But if you need decimal (floating point) numbers, there are two types of data in Java:
float: ±1,401298e-45 ±3,402823e+38 double: ±4,94065645841246e-324 ±1,79769313486231e+308
For mathematical calculations, it is usually preferred to type
double (15 decimal places) than
float (7 decimal places).
In Java, there are four arithmetic operators
+ ,
- ,
*, and
/ , and still parentheses for prioritizing calculations. The following calculations with these operators:
public class Numbers { public static void main(String[] args) { int x = 5; System.out.println(7 + x * 3);
Multiply and divide operations take precedence over addition and subtraction. Pay attention to the action of the operators
/ and
% when dividing integers, if in the first case (operator
/ ) after dividing only an integer part remains, then in the second case (operator), the whole part was rejected, and the result is 1 7 on 3.
Do you need more math functions? Well, call the
Math class. An example of this formula:
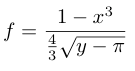
In Java, it will look like this:
double f = Math.abs(1.0 - Math.pow(x, 3.0)) / (4.0 / 3.0 * Math.sqrt(y - Math.PI)));
When we write integers and fractional numbers, we mark it with a full stop. For example, 3 is integer, and 3.0 is fractional.
The following program displays all integers from 1 to 10 that are not divisible by 3:
public class Sum { public static void main(String[] args) { int total = 0; for (int n = 1; n <= 10; n++) { if (n % 3 != 0) { total = total + n; System.out.println(total); } } System.out.println("Total: " + total); } }
As a result, we obtain 1, 2, 4, 5, 7, 8, 10 and their sum is 37.
Complicate task # 4 : Change the last program and display the numbers that are not divisible by 4.
If you need more details, what other functions are available in the
Math class:
docs.oracle.com/javase/7/docs/api/java/lang/Math.htmlBut what if 15 decimal places are not enough? Then you need the
BigInteger and
BigDecimal types, these are classes for performing calculations with very high accuracy. If necessary, one hundred, one thousand or one million decimal places. Example:
import java.math.BigDecimal; public class HighPrecision { public static void main(String[] args) { BigDecimal a = new BigDecimal("2.7182818284" + "590452353602874713526624977572" + "4709369995");
Run the program and you will see everything yourself.
A couple of bits about strings
You already had the opportunity in each of our examples to become familiar with the use of strings. Type String is a sequence of letters, numbers, and characters. The simplest example is “Hi John!”. But most often the lines are used to search for parts of the text or for arranging large messages from several parts. To do this, you can use the
+ sign, it will merge several lines into one:
String qty = "50"; String message = "I found " + qty + " books.";
If we need to get part of a string, we use the
substring method. Each character in the string has a position. The numbering is 0. In the following example, you need to get the word "house", its characters are from 4 to 8 positions:
String line = "The house is blue."; String word = line.substring(4, 8 + 1);
Well, if you need to find out exactly where the beginning of the word “house” is, then we apply
indexOf :
String line = "The house is blue."; int position = line.indexOf("house");
Please note
indexOf is spelled with a capital "O". In the last line of the example, we are trying to find the words “red”, but it does not exist and the method returned us -1.
You can find the length of a string using the
length () method. To get individual characters,
we use
charAt () , the following example shows how to decompose a string into characters and display them on a letter by letter.
public class LetterByLetter { public static void main(String[] args) { if (args.length == 0) { System.out.println("Please type" + " a word."); } else { String word = args[0]; for (int i = 0; i < word.length(); i++){ System.out.println(word.charAt(i)); } } } }
After compiling
javac , run the program by typing the command line after the name of our class.
java LetterByLetter telephone
As I said in the last article, the string entered through the args variable is transferred to your program (in this example, it is “telephone”). Try not to enter this word and the result will be different. The control structures ensure the work of the program so that from the beginning we get the size of the line and only then we select letters in order.
There are many methods for working with strings. This is described in more detail in the
docs.oracle.com/javase/7/docs/api/java/lang/String.html documentation
.Complicate task # 5 : Change the previous example and capitalize each letter. Hint: use the method that makes all the letters in a line capitalized at once, this method can be found in the documentation for the link above.
A couple of bits about logic
The
boolean boolean type can have only two options for the value of a variable:
true or
false . It is most often used as an indicator or as a switch for
if ,
while, or
for control constructs. Example of declaring a boolean variable:
boolean painted = false;
And so we change its value:
painted = true;
Or use for
while :
while (painted) {
You can also use operators for logical variables
! (NOT),
&& (AND) and
|| (OR). Operator
! returns the inverse of the variable. The
&& operator returns
true if both parts of the expression are
true , and the operator
|| returns
true if at least one of them is
true . Example:
boolean a = true; boolean b = false; boolean c = !a;
In the future, you may encounter the operators
& and
| . These are also AND and OR operators, but for numeric variables. But the version of the operators
&& and
|| more effective, since there is no difference in the location of the operands. In the following example, you can see that the result does not depend on the location of the operands:
boolean g = a || b;
Numeric and string variables can also return a
boolean result. This is a comparison result for control structures. Example:
int total = 156; if (total > 100) {
The expression in parentheses compares the variable
total , if
total is more than 100, the result will be
true , otherwise
false . To compare two numbers, you can use the following six operators:
== != > < >= <=
All returned values ​​will be of type
boolean , and you can combine them with other operators of type
boolean , for example:
int sides = 6; boolean painted = false; if (sides >= 4 && !painted) {
Conclusion
This article included several topics, and it turned out to be more complex than the first, but I tried to make sure that you read the basics of Java more quickly.
In the next article, we will introduce Java methods, classes, and objects, with some of the class libraries in Java. Then you can make a program generating a card.