Instead of entering
At the dawn of programming and until very recently, the program was something complete, completely ready for use by an independent unit that performed its functions and only them.
However, with the advent of mobile devices, web sites with rich logic and social networks, everything began to change. Now programs that do not go online, do not know how to upload something to Facebook and generally work on their own, practically have no right to life. Even professional tools, such as
Microsoft Office 2013 , began to support cloud storage for exchanging documents.
The world is changing. Now, in order to make money selling software, it is not necessary to write your own
operating system or antivirus, spending a lot of time and resources. Simply ask your wife and work together to develop a
world hit . Therefore, many today dream of creating their own evil birds or cat-ze-roup, studying the development for
iOS ,
Android ,
Windows Phone .
')
Suppose you have written your application and published it in some of the stores. Everything is fine, you make a profit, but you want more. You understand that you need to write applications for other platforms in order to expand the user base. This is where the first ambush lies - how to minimize the amount of code you write, if applications run more equally and differ only in appearance (and not fact) and in programming language?
The answer to this question will be the PLO old man, dressed in fashionable clothes and changed his name. If you take out the common logic from the application code to a certain common service and locate this service on the Internet so that all applications can connect to it, then to implement the mobile application on a specific platform, you just need to write the data mapping code from the server. Sounds familiar, doesn't it? This is very similar to the
MVC pattern. Here, Model is a service on the Internet that receives and sends data, and View and Controller are implemented on a mobile device and can be as simple as possible. Today, the so-called
RESTful API , a software interface that can be accessed through standard HTTP methods, has increasingly been used as a model for such a service.
And everything seems to be looking good and it already seems that a solution has been found. However, problems begin when you begin to deploy the backend service on the server. I am a programmer, and when it comes to installing and configuring a server, I immediately feel uneasy. First of all, this is absolutely new knowledge that needs to be learned so that everything works as it should. Secondly, in order for everything to work as it should and not fall with increased load or attack, it is necessary to smoke mana even more and more persistently. You can try to find such a hoster that will save you from messing with the servers and pre-install PHP and / or something else. But then the problem remains that you will need to independently implement all the necessary server event handlers to implement a full-fledged REST API. And this again takes away your useful time and spends it on unnecessary things.
Why am I all this?
I myself have long been tormented by the question, how can I organize my server backend for an application? At first I wrote something special using WCF services. Then came the
ASP.NET Web API , which pretty well simplified life. But today I want to talk about something else. I, as a fan of easy-to-use things, could not pass by the relative new service that appeared in the
Windows Azure cloud platform. The name of this service is Windows Azure Mobile Services Custom API.
This service, along with other useful features of Windows Azure Mobile Services, is a PaaS solution and provides the ability to quickly deploy the cloud-based RESTful API that the program can access in any language and platform. The basis of this decision is no longer a new, but quite popular
Node.js technology. Custom API is a fully functional Node.js application with all the ensuing consequences - it is a complete solution without compromise. And taking into account the fact that native SDKs for all three popular mobile platforms were written to work with it, this solution becomes even more interesting.
Further in this part, I want to talk about how to create and start using the cloud backend in Windows Azure and access it from a mobile device. Do not switch!
Creating cloud backend
Creating a cloud backend, like any other service in Windows Azure, comes from
the cloud
management portal . First you need to create a mobile service, inventing some meaningful name for it:

Next, you need to select the SQL database in which the mobile service data will be stored. There is an option to create a free copy of 20Mb. To test the possibilities - enough for the eyes. And if you like it, you can always upgrade to more serious decisions.
After clicking on the arrow further and entering the database server parameters (create a new or use an existing administrator login / password and other dullness), a new mobile service will be created in the cloud. This usually happens extremely quickly, in less than a minute. When the service is created and you enter it, you will see something like this window:

Remember it, it is still useful to us further.
API creation
To create your first cloud-based API, just go to the API tab and click the Create a Custom API button:
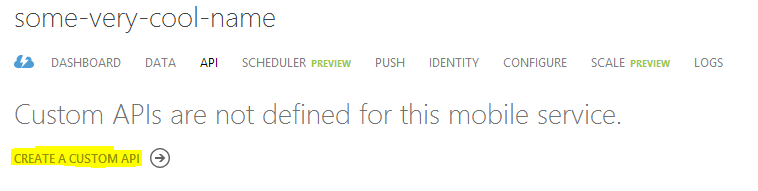
If you have previously worked with Windows Azure Mobile Services, then the following window will be familiar to you. It is necessary to specify the name of the future API, as well as one of the four levels of access to its various methods. Leave everything by default, then only those clients that have an authorization parameter can connect to our API:

Once the new API has been created, we can proceed to editing it. Initially, you should see a script similar to this:

For educational / test purposes, I suggest changing it slightly to make it like this:
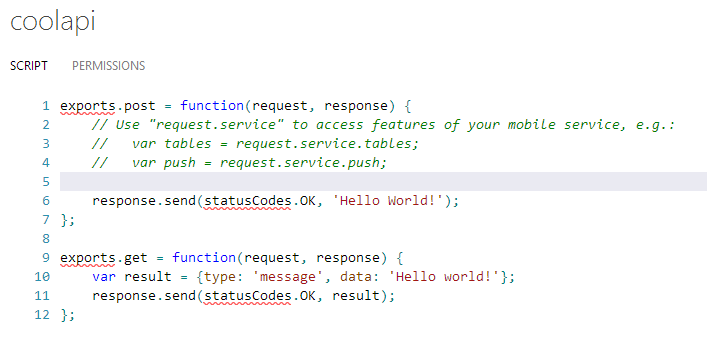
The main change in this case was that the POST request handler began returning data as a simple string, and a variable was added to GET, and its object has more than one field. I did so for clarity, to illustrate the various possibilities of working with data.
API usage
For this article, we will use a test application that Windows Azure Mobile Services kindly provides us. To do this, go back to the Quick Create page (this is the same one with a cloud and a lightning bolt on the icon), select Windows Phone 8 (although pay attention to a wide choice) and click Create A New Windows Phone App:
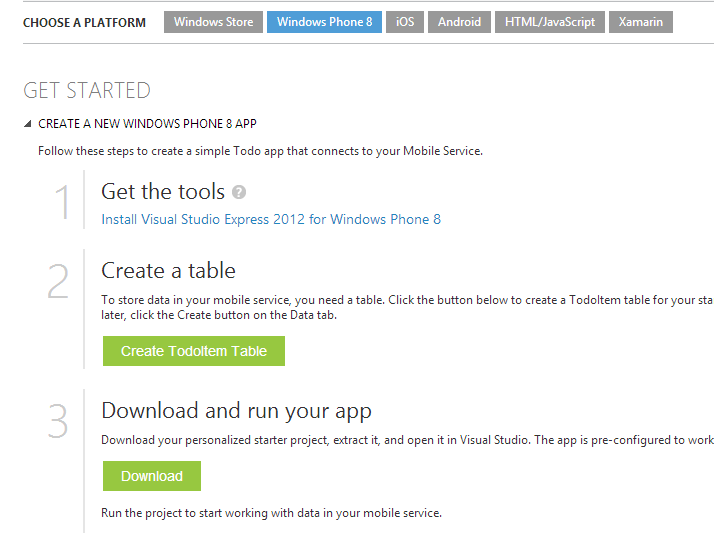
After creating the desired label (TodoItem) and downloading the application using the Download button, open it in Visual Studio.
First of all, we are interested in two things. The file App.xaml.cs has a line like this:
public static MobileServiceClient MobileService = new MobileServiceClient( "https://mva-test-api.azure-mobile.net/", "___" );
With this field, we will communicate with our new mobile service. The set of incomprehensible symbols is the ApplicationKey, the individual key of your service, which you can use and honor.
Let's run the application and see at least how it looks:
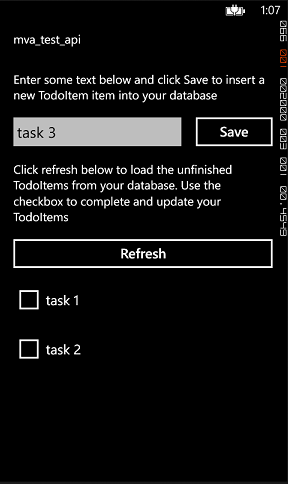
Well, not hit upstor, but not bad for a start. Let's endow it with additional logic and call our API methods. Go to the file MainPage.xaml.cs. From him, I want to ensure that when you click on the Save button, the services are accessed, after which the result is simply written to the debug console. To do this, add the following code to the end of the InsertTodoItem method:
// POST var result = await App.MobileService.InvokeApiAsync("CoolAPI", null, HttpMethod.Post, null, null); Debug.WriteLine(result.StatusCode); var stringData = await result.Content.ReadAsStringAsync(); Debug.WriteLine(stringData); // GET var resultJson = await App.MobileService.InvokeApiAsync("CoolAPI", HttpMethod.Get, null); Debug.WriteLine(resultJson);
The main method in this code can be called InvokeApiAsync. He is responsible for calling one or another API method. This method is overloaded and endowed with a different set of parameters. In the example, it is clear that in the case of POST we pass as many as 5 parameters, and in the case of GET there are only 3. This is due to the fact that the method for POST is calculated on the fact that the result will be a regular line (remember the implementation of the script on the backend) and the variant with GET is for working with a JSON object (the result will be
Newtonsoft.Json ).
If you now start the application and press the Save button, then in the Debug console of the application you will see something like this:
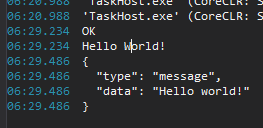
How are they
I didn’t just ask to pay attention to the possibility of choosing the type of application on the Quick Create page in the Windows Azure Control Panel. The fact is that the native SDK for working with mobile services is written not only for Windows devices. Their libraries are released for both iOS and Android, so all the same can be used on these platforms. Here is an example of ObjectiveC code that will do roughly the same as in the example:
[self.client invokeAPI:@"CoolAPI" data:nil HTTPMethod:"POST" parameters:nil headers:nil completion:^(NSData *result, NSHTTPURLResponse *response, NSError *error) { NSLog(@"%i", response.statusCode); NSString *stringData = [[NSSatring alloc] initWithData:result encoding:NSUTF8StringEncoding]; }];
As you can see - “pure” ObjectiveC, without flies. Similarly for Android, WinRT and even for the web version (in HTML and JavaScript).
What's next
I originally planned to write only one article at once about all the capabilities of the server code in Windows Azure Mobile Services, but there is too much material. So now we’ll finish, and in the next part I’ll talk about how you can work on a cloud backend in a team using Git. And how to extend the functionality with common scripts and NPM packages.