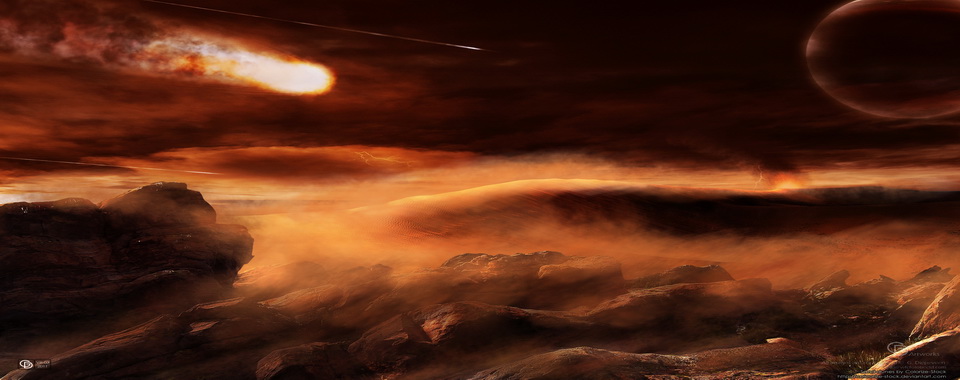
If you are interested in client programming, then, like me, you could probably hear about Meteor, no longer a new web framework that is gaining in popularity, so-called “full stack” frameworks. About him already wrote on Habré, so as not to duplicate information, you can read
here or
here . I had heard about a meteor for a long time, but still could not decide to touch it. And so, the next weekend came, and I stumbled upon it again. This time I decided to honestly try what it is. Maybe this post will give someone a slight impetus to the beginning of the study, or at least familiarity with this tool. Then it would not be in vain.
Disclaimer: may cause addiction.Let's take the first steps to mastering meteorjs, welcome under cat.
Installation
So, first we need to install the meteor itself. For linux-like OS and OSX, you need to run the command in the terminal
$ curl https://install.meteor.com/ | sh
If
you are unlucky, and you have Windows, download and install the binary from
win.meteor.com , everything should work.
')
Create a project
Creating a project is easier than ever, the framework includes the meteor utility, with which we will create a project. It is enough to execute in the terminal
$ meteor create projectName
and the meteor will create a folder for the project and place there the minimum set of files for our new application.
It is necessary to say a few words about the structure of the project. Initially, the meteor creates for us three files for html, css and js and two more for storing information about installed packages.
projectName.css projectName.html projectName.js smart.json smart.lock
The entire application can be written in these three files. Of course, we will not do that.
Meteor lets you decide how to organize the files in your project. It does not impose rules, such as Ruby on Rails, you can name files and folders as you prefer.
Now you can start the server. It's all too easy. In the project directory execute the
$ meteor
command. Now our application is available on port 3000. So far, it does not contain anything supernatural, but it is already a workable meteor application with magic built-in data reactivity. If you do not know what it is yet, do not worry, you will learn about it a little later.
And here is a small demonstration hiddenTo quickly see the magic of a meteor, you can create an application with a ready-made leaderboard test pattern.
$ meteor create --example leaderboard
This example is a simple list of players and points scored by them with the ability to add points to players.
Simply open the application in two browsers located side by side, and add points to players. All changes will be instantly synchronized in both browsers. And this is without a single line of code for this! Meteor does everything for us inside using sockets.
If you are too lazy to create another project, see the video
www.meteor.com/examples/leaderboard Now you can experiment a bit.
First, let's delete the three files created by meteor, we will not need them.
Next, you need to create two folders - client and server. This is one of the few file sharing conventions. Everything that is inside the server folder will be executed only on the server and, accordingly, that in the client folder it will be executed only on the client. All other files, wherever they are, will be executed in both environments. Immediately a couple of details - the files from the
lib
directory will be connected before the others, and the
main.*
Files after everything else.
Something about connecting files
Meteor cares about the convenience of development, Live Reload works out of the box, which, when changing any files in the project directories, will restart the server and refresh the page in the browser. In addition, you do not need to connect any scripts and styles, the meteor will find them and connect them, and if suddenly you are an adherent of coffeescript or less / scss / stylus, then with installed plug-ins (they will be discussed just a couple of lines below) and compiles.
Meteorite and Atmosphere
Actually about plugins. As you may already know, the meteor uses its packet system. There are several types of packages.
Core packages
The most basic packages that are already included when creating a project. These include, for example, jQuery and underscore. You can use these libraries right out of the box.
Meteor smart packages
A group of about 40 plugins that are installed using the meteor utility. Their list can be viewed by running the command in the terminal
$ meteor list
You can install the plugin with the command
$ meteor add packageName
without even turning off the server.
But even 40 plug-ins - you can say very little, and you will be absolutely right.
Atmosphere smart packages
This is where the
Atmosphere comes to the rescue - a kind of storage for third-party plug-ins. At the time of this writing, there were as many as 859, you can choose something to your liking. To install plug-ins from the atmosphere, you need to install the nte meteorite package. He will be engaged in the installation of plug-ins.
$ sudo npm install -g meteorite
After installation, you can replace the use of the meteor command with mrt, which completely duplicates the functionality of the standard (for example, just starts the server when you call
$ mrt
), or adds specific functionality, say, when calling
$ mrt add foundation-5
in this case adds the foundation-5 plugin from the list atmosphere.
It turns out pretty nice plugin installation system.
Npm packages
Well, and of course npm packages. After all, meteor is still nodejs. You can safely install the usual npm packages and use them.
For the cause
Let's do something with our little application. For example, we make a simple conclusion to-do tasks. To make it look more beautiful, add to the bootstrap project.
$ mrt add bootstrap
Let's start with the template. Meteor uses the
Handlebars engine for Templates. Create a main template in the client folder -
main.html
<head> <title>Simple To-Do list</title> </head> <body> <div class="container"> <header class="navbar"> <div class="navbar-inner"> <a class="brand" href="/">To-Do</a> </div> </header> <div id="main" class="row-fluid"> {{> todosList}} </div> </div> </body>
todosList is a partial template. Remember, I said that we can place files as we like? This is still true, but let's be organized, and create in the
client
directory the
views
, into which we place templates and js files, which will be something like controllers. Usually each template will correspond to a js file.
Create templates for the to-do list and for the individual item.
/client/views/todosList.html: <template name="todosList"> <div class="todos"> {{#each todos}} {{> todo}} {{/each}} </div> </template>
/client/views/todo.html: <template name="todo"> <div class="todo"> <h3>{{task}}</h3> </div> </template>
So, templates are ready. Everything is simple here - a list of all todos - a collection of tasks is displayed in todosList. Let's define this collection. It should be both on the server and on the client, which means that neither the
server
nor the
client
are suitable for hosting the collection file. I suggest creating
collections
directory in the root of the project just for such cases. And add the file itself
/collections/todos.js: Todos = new Meteor.Collection('todos');
For the beginning of this code will be quite enough, it will create the collection itself.
The final touch that needs to be made is to show the template which data to use for display. Create another file.
/client/views/todosList.js: Template.todosList.helpers({ todos: Todos.find() });
Remember, in the template list, we used the expression {{#each todos}}? .. Now we just set the source for this “variable”.
Now you can open the browser console and add the task to the collection.
Todos.insert({task: ' meteorJS'});
The result will not take long to wait - the first to-do task will be drawn immediately. By the way, if the same page is opened in another browser, then the added task will also appear there.
It seems to be more than enough for a start. We looked at how to make the simplest elements using meteor. Of course, this is not enough, it is worth mentioning more about routing, security (did you notice that we have full access to the database from the browser console?) And many other things, but it doesn’t fit in just one article. A little later, I am in the process of researching meteor, I will describe the transition from simply adding data to the console to implementing this feature in the project interface, also working with routing and clever access control, and maybe some other interesting things.
Finally leave a few useful links:
Actually the site meteor - here we watch a couple of introductory videos about the framework, and read the documentation.
A whole collection of links to useful materials.Thank you for reading, I hope you at least a little interested in this, of course, an interesting framework.