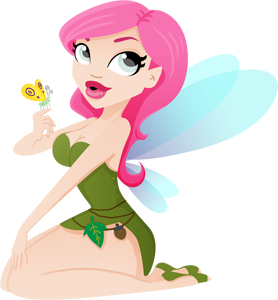
Today I wrote the last test for version 3.0 of the database access module for
PHPixie . When I started it seemed that it would take only a few weeks, but in fact it all took about 2 months because of the huge amount of refactoring and rewriting from the beginning. But now we have an expandable library that can be used even outside the framework itself (that is, if you are forced to write on some other framework or CMS, you can take your favorite library with you).
I will make a release after finishing the ORM module and correcting the existing modules for working with the new API. But if you are interested, you can look into
the 3.0 branch on the githab (however, you should be warned that the documentation is not there yet, it will appear along with the ORM). And now let's look at the new features.
From the point of view of the average user
I’ve already written about advanced query support with logical conditions for MongoDB
here , but if you’re too lazy to click on this link, here’s a brief explanation: MongoDB does not use indices when using queries like “
(A or B) OR (C and (D or E )) ”, So PHPixie itself will lead requests to such forms that will use them.
Several approaches for building queries have become available:
$query ->where('name', 'Trixie') ->or_where('name', 'Tinkerbell') ->where_not('id', '>', 7) ->having('count','<', 5) ->or_having('count', 7);
')
Several approaches to nested logic:
$query ->where('name','Trixie') ->_or(function($builder){ $builder ->_and('id',7) ->_or('id',5) });
Special operators for column comparison:
$query->where('fairies.id','*=','pixies.id');
Ability to use SQL code inserts with separate parameters:
$expr = $this->db->expr('concat(name, ?)', array('test')); $query->where($expr, 'Trixietest');
Search strings by NULL value, not knowing in advance that this is NULL:
$category_id = null; $query->where('category_id', $category_id);
Difficult conditions for JOIN .... ON using the familiar syntax:
$query->join('pixies');
From the perspective of an advanced developer
- Incredibly lightweight, only 57 kilobytes of code
- Query builders do not contain any logic related to their parsing (for example, in SQL code). Instead, each driver uses its Parser service, making the request objects themselves easy and cheap in terms of memory. Using parsers as services makes debugging and expanding them with trivial tasks. By the way, each parser will be created in memory only once for all requests.
- Each parser is divided into parsers of individual conditions and condition groups (Condition Group Parser). This separation makes it easy to add your custom operators.
- The binding of dependencies is rendered into separate classes of drivers, that is, in no case (except throwing exceptions), no class creates objects by itself using the new command. This makes it easy to replace any class with its own implementation.
- Requests to MongoDB are parsed into special Runner objects that are easily debugged in case of an error.
- SQL databases that work through PDO have their own individual adapters and parsers, which makes it easy to add support for any database that PDO works with using minimal code.
The code is fully covered with PHPUnit tests, which gives 166 tests and 1151 comparisons.I hope that this post will convey my joy with the work done and that the final release of the ORM module is not far away.