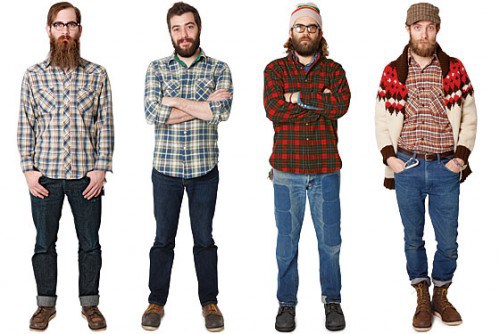
JavaScript is an interesting programming language.
Constant fears to make mistakes in the code make you think all the time. Programming skills are improved due to the fact that you have to constantly execute code in your mind, there is no other way.
That is why it is important that the code is neat, compact and elegant. One that you can fall in love with. Otherwise, javascript can scare away.
')
I picked up some interesting snippets for you that please me and which I use instead of boring code that takes up a lot of space. Some of them make the code shorter, others are clearer and clearer. A couple more are hacks for debugging.
I learned all this while studying projects with source code, but here I am writing as if I had invented them.
Hipster hack # 1 - function call
I really hate the if / else block, a useful trick will help us to get rid of it. It allows us to make a function call based on a boolean value.
Hipster hack # 2 - string connection
A well-known fact - strings are friendly with other strings. Sooner or later you will need to concatenate several of them. I don't really like using "+", so join comes to the rescue:
['first', 'name'].join(' ');
Hipster hack # 3 - default operator ||
In JavaScript, you never know exactly what is in the object. Sometimes you get it as a function argument, sometimes it is passed to you over the network, or you read it in the config file. In any case, you can use the convenient operator || which returns the second value if the first one is false.
Hipster hack # 4 - security operator &&
Like the default operator, it is extremely useful. It allows you to get rid of almost all IF and makes the code much more pleasant.
Hipster hack # 5 - operator XXX
This operator is fully subject to copyright law. When I write code and for some reason I need to go to the web or edit another piece of code, I add a line with the
xxx operator. The code in this place will be interrupted and you can finish what you started later. Line
xxx is perfectly looked up, since it is not found in normal code, and you don’t have to bother with a comment in TODO at all.
var z = 15; doSomeMath(z, 10); xxx
Hipster hack # 6 - time measurement
It is interesting for you to find out which is faster: a loop with i ++ or with i--. I personally do not. Those who are interested can use the output of the measurement time in the console. This is useful if you need to know the speed of operations that block the event cycle.
var a = [1,2,3,4,5,6,7,8,9,10]; console.time('testing_forward'); for (var i = 0; i < a.length; i++); console.timeEnd('testing_forward');
Hipster Hack # 7 - Debugging
I learned this trick from a Java developer. Absolutely can not imagine why he knew about him, but I did not. But, be that as it may, since then I use it constantly. Just enter the
debugger in the code and the debugger will stop at this line.
var x = 1; debugger;
Hipster Hack # 8 - Debugging Old School
I always liked to display the values of variables in some places of the code, instead of line-by-line debugging. If you are like me, you may need to bring some private variables to the global scope. The main thing is not to forget to clean up the code before transferring to production.
var deeplyNestedFunction = function() { var private_object = { year: '2013' };
Hipster hack # 9 - ultra-light template engine
Are you still using "+" for string concatenation? There is a better way to connect data strings. It is called templating, and here you have a great 2.5 lines of code framework.
var firstName = 'Tal'; var screenName = 'ketacode'
Did you all know that already?
And even the operator XXX invented by me personally? Yes, you're just a real hipster hacker!