Part 1Part 2Setting up the environment and creating a new mobile application.
In the previous part of the cycle, we decided on the task and created the simplest FM application that displays a list of recipes. At the same time, we used the
FireDAC data access library and
LiveBinding object binding technology. We also agreed that, as part of the task, two applications will be created using a single code base. And now, the time has come for perhaps the most interesting part -
creating the first Android application .
')
First of all, you need to configure the environment. If you didn’t install Android development tools (JDK / SDK / NDK) when installing
RAD Studio , you should do it manually. You also need to install a USB driver for the Android device with which the application will be developed. It is worth noting that not all Android devices can find a USB driver. In this case, you can take advantage of the debugging via Wi-Fi.
When creating a new project, we are invited to use one of several mobile application templates. Select the template Header / Footer, which is a mobile form with two toolbars (Toolbar). After selecting a template, you must save the project. It is most convenient to create a new project using Project Manafer (right top panel of the IDE), as shown in the figure. Thus, we will be able to work simultaneously with two projects in the group.
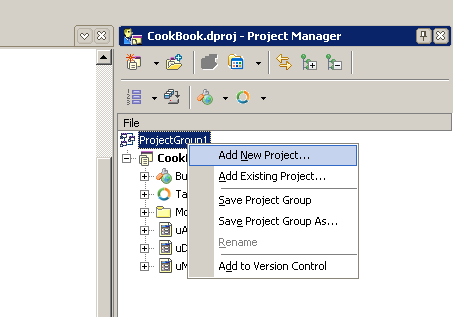
The main form of the application for the template we have chosen is by default adapted to the Google Nexus 4 device. Using the drop-down list in the upper right part of the form designer, you can select another device. For our application, we will choose one of the most popular tablet devices, Google Nexus 7. Immediately change the title text on the main form (Text property of the HeaderLabel component).
First launch of the application
As you already understood, we will use a physical device connected via USB to debug the application. Although other options are possible (connecting via Wi-Fi or using an emulator). If you connected your tablet or phone in accordance with the instructions and activated the developer mode, then the name of your “device” will appear in the list of target devices (Targets). Activate it.

Once again, make sure that the Android SDK is configured for your device, and so on. “Developer Mode” is activated. The entire process of setting up an environment for developing an Android application is
described here .
It starts the mobile application in the same way as the usual "desktop" (by pressing F9). If you did everything correctly, then after compiling you will see the main form of the application on your tablet.
Now we will try to implement the same functionality that we implemented in the previous part. To do this, add to the current project an existing data module from the previous project (menu
Project |
Add to Project ... ). As in the first project, the module should be included in the Uses section of the main form.
implementation {$R *.fmx} uses uDM;
Actually, now nothing prevents us from using the same connection setup procedure that we used in the “desktop” application:
function TDM.ConnectToDB: Boolean; begin try FDConnection1.Connected := True; FDTRecipe.Open; except end; Result:= FDConnection1.Connected; end; procedure TDM.DataModuleCreate(Sender: TObject); begin DM.ConnectToDB; end;
Thus, we have eliminated the need to re-write the connection code.
Main application form
In principle, the development of the user interface of a mobile application is a separate, very extensive topic. Often, it is an incorrectly designed GUI that can cause failure. And sometimes programmers try to transfer their experience in creating interfaces for desktop applications to mobile development. This is not entirely correct. Before you start writing a mobile application, you should carefully study the
basic principles of interface design . And, of course, it is worth exploring the interfaces of popular programs.
In our case, we can easily organize the interface of the main form of the mobile application in the image of the “desktop” (in fact, we built the Windows interface of the application taking into account the fact that we will have to make a mobile application). Place the components TPanel, TSplitter and TCalloutPanel on the form. In Object Inspector, we change their properties as follows:
Panel1: TPanel Align = alLeft Splitter1: TSplitter Align = alLeft Width = 16 CalloutPanel1: TCalloutPanel Align = alClient Width = 630 TabOrder = 4 CalloutPosition = cpLeft
To display a list of recipes, we also use
TListBox and
TBindNavigator , which we will place on the left panel.
Place TTabControl on the
TCalloutPanel component and configure the Align property for each component.
Like last time, we use the
LiveBinding mechanism to connect a dataset. This procedure is completely similar to the one we did in the previous section, creating a “desktop application”.
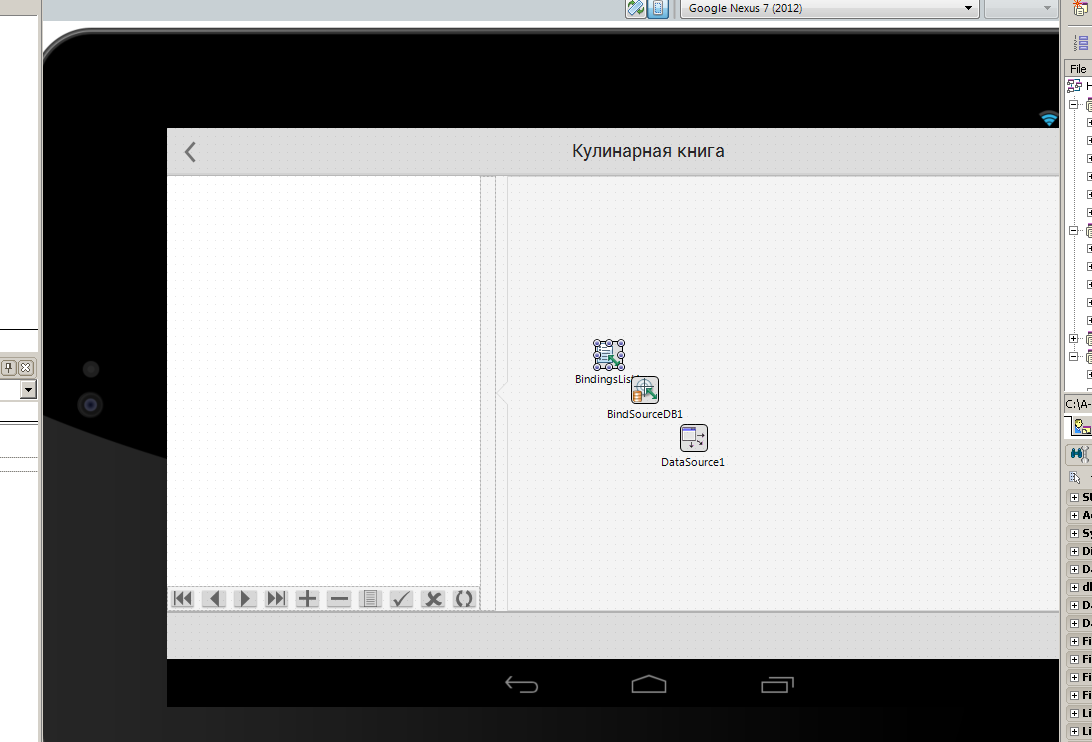
Like last time, we need to create a tool to enter the name of the recipe. If in the desktop application we could use the modal form, then the concept of mobile applications in most cases offers another solution that regulates the user’s action more gently. However, here we use the “dialogue”, which in fact can be regarded as a kind of modal form.
Create a new mobile form. We will place a panel on it, and on the panel we will place those controls that we placed on the recipe addition form last time (TEdit and two buttons). The value of the Align property is alCenter. For buttons, set the value of the ModalResult property (mrOk and mrCancel).

For the form class, create the following class method:
class procedure TfAddRecipe.ShowDialog(DataSet: TDataSet); begin fAddRecipe.ShowModal( procedure(ModalResult: TModalResult) begin if ModalResult= mrOk then begin if DataSet.State in [dsInsert, dsEdit] then DataSet.Post; fAddRecipe.Close; end else begin if DataSet.State in [dsInsert, dsEdit] then DataSet.Cancel; fAddRecipe.Close; end; end ); end;
When an application is launched, we will assign this method as an AfterInsert event handler for the only data set in the program.
procedure THeaderFooterForm.FormShow(Sender: TObject); begin DM.FDTRecipe.AfterInsert:= TfAddRecipe.ShowDialog; end;
This form will be called whenever you add an entry to the FDTRecipe data set.
Naturally, such code needs optimization. Creating an instance of a form when launching an application is not a good idea. Especially for mobile apps. But this is a topic for a separate discussion, but for now, for reasons of clarity, let's leave everything as it is.
Deploying (deploying) Android applications
Before you start an application running from the database on Android, you need to make certain settings that allow you to transfer the database to a mobile device, and allow the application to "see" the database. To do this, use the Deployment Manager (menu Project | Deployment).
Make sure the debug configuration for the Android platform is selected. In the drop-down list on the top toolbar, the
Debug configuration value should be selected -
Android platform .
In order to transfer the database to the device when deploying the application, you should use the “Add files” button and select a database file.
Enter
assets \ as the Remote Path field value, and set the Platform field value to Android. The assets directory is a special directory
for storing application support resources . In the Platform field, select
Android .
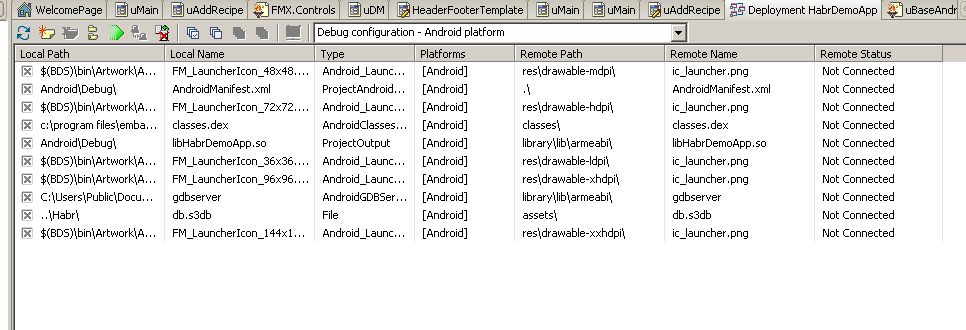
You can click the Deploy button to test the application deployment process.
And finally, in order for the application to work, another important step should be taken - to define the event of the FDConnection1 component as follows:
procedure TDM.FDConnection1BeforeConnect(Sender: TObject); begin {$IFDEF ANDROID} FDConnection1.Params.Values['Database'] := '$(DOC)/db.s3db'; {$ENDIF} end;
The compiler directive {$ IFDEF ANDROID} is transmitted to ensure that this code is executed only on Android devices. Thus, we indicate to the application the relative path to the database.
We start the application, and on the device screen we get an almost complete analogue of the Windows application that we created in the previous part.
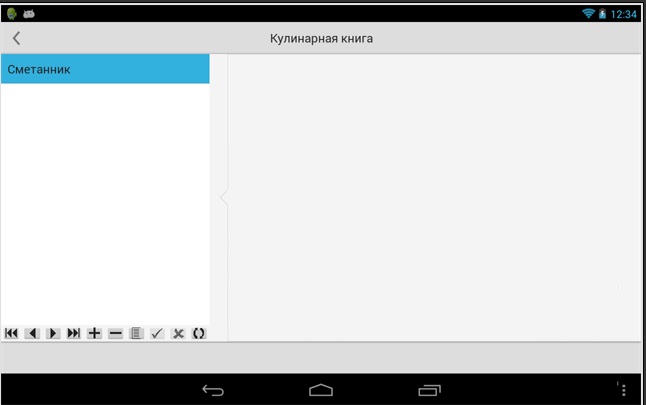
In this part, we built the first mobile application and figured out how the process of creating mobile applications in Delphi is similar and how much it differs from the process of creating a “normal” desktop application. Naturally, while we implemented only the basic functionality. This applies to both the program logic and its interface. But everything has its time. In the next part, we will continue working on the Windows application and learn more about the possibilities and principles of the
LiveBinding mechanism.
Stay with us.