Today we will talk about how to create the simplest applications for VKontakte under Windows 8. First, go to
http://vk.com/developers.php and create a new application,

Next, we select Standalone applications, as it is intended for computers and smartphones. Enter the name of our application and click Connect applications. After that, you will receive messages on your mobile phone with a code that is required to confirm the registration of the application.
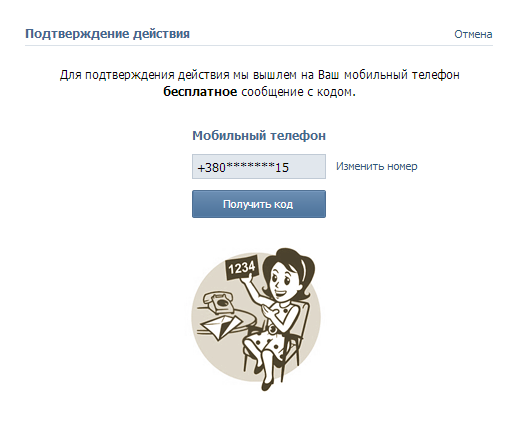
After a successful check, you will be taken to the application editing page, it looks like this:
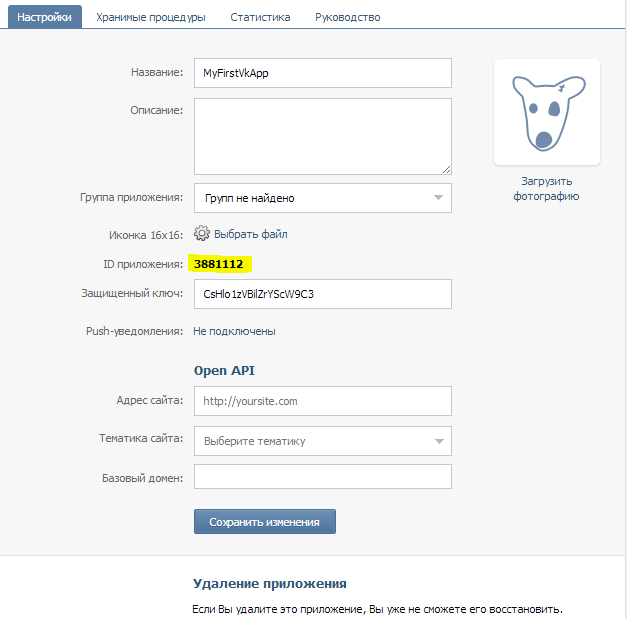
From this page we will need the application ID, you can save it somewhere for further work with it.
So, the floor of the case is done, now go to the authorization.
Go to
this page where it is described how the authorization should take place. Now go directly to the coding, to do this, start Visual Studio and create a new project based on BlankApp.
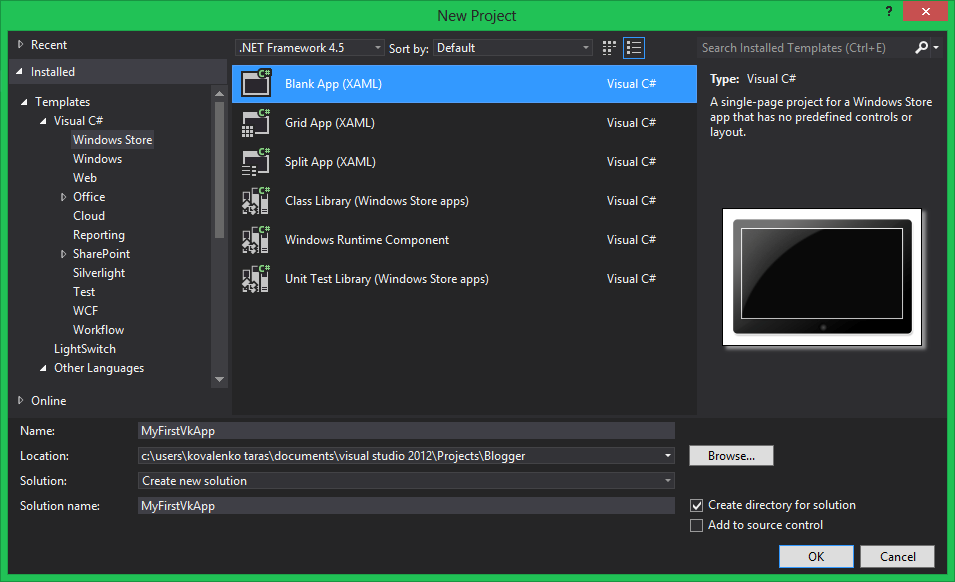
Authorization will be done using the
OAuth 2.0 protocol
. To do this, go to the MainPage.xaml.cs page and create a new OAuthVk () method that will authorize via WebAuthenticationBroker, which is a special class that runs the standard window that opens the link for authorization. After entering the data in the authorization window, the broker completes its work and transmits the result of whether the authorization was successful or not.
Here is the code of our method:
public static async void OAuthVk() { const string vkUri = "https://oauth.vk.com/authorize?client_id=3881112&scope=9999999&" + "redirect_uri=http://oauth.vk.com/blank.html&display=touch&response_type=token"; Uri requestUri = new Uri(vkUri); Uri callbackUri = new Uri("http://oauth.vk.com/blank.html"); WebAuthenticationResult result = await WebAuthenticationBroker.AuthenticateAsync( WebAuthenticationOptions.None, requestUri, callbackUri); switch (result.ResponseStatus) { case WebAuthenticationStatus.ErrorHttp: MessageDialog dialogError = new MessageDialog(" \n" + " !", ""); dialogError.ShowAsync(); break; case WebAuthenticationStatus.Success: string responseString = result.ResponseData; MessageDialog dialogSuccess = new MessageDialog(responseString); dialogSuccess.ShowAsync(); break; } }
Now a little about what I wrote. The first line of vkUri is the opening address of the OAuth 2.0 dialog box; we take it
from here . In the line it writes client_id - we pass the id of our application, scope - the application access rights that come
from here , I wrote 9999999 this is access to everything where access_token does not change its value, this is in order not to pass authorization every time, but to immediately save access_token and user_id, and use them to call the API, but more on that later in a separate post. After that, we call the AuthenticateAsync method to perform authentication, and write the result to the WebAuthenticationResult. After that, if you start the application, we will see an authorization window:

Further, through the switch statement, we check the result of the authorization and perform the corresponding actions. If the network fails, we display an error message; upon successful authorization, we display the messages in which access_token and user_id will be specified. The server response will be something like this:
REDIRECT_URI # access_token = 533bacf01e11f55b536a565b57531ad114461ae8736d6506a3 & expires_in = 86400 & user_id = 8492
All we need to do now is get it from the access_token and user_id lines for their further use. To do this, instead of a message about successful authorization, we will write the following code:
char[] separators = { '=', '&' }; string[] responseContent = responseString.Split(separators); string accessToken = responseContent[1]; int userId = Int32.Parse(responseContent[5]); MessageDialog dialogSuccess = new MessageDialog("access_token = "+accessToken+ "\nuser_id = "+userId); dialogSuccess.ShowAsync();
Now, upon successful authorization, we will display messages in which only access_tokeb and user_id will be displayed.
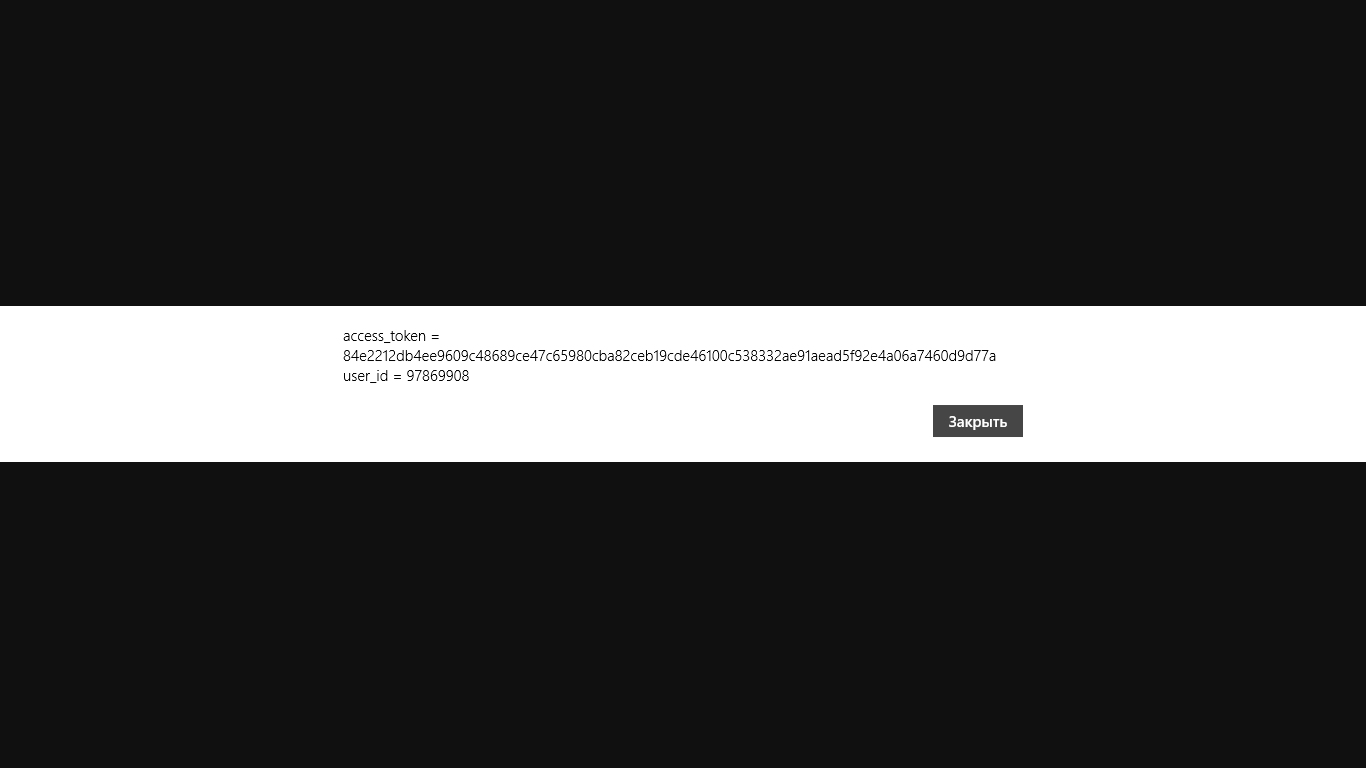
That's all, the token and id we got, you can call any API.
PS If you are interested in the following posts, I will consider the most interesting and complex API functions, because most of them are called the same.
Good luck in PROGRAMMING.