In this article we will use ZXing (Zebra Crossing) to decode the barcode in our Android application.
Using ZXing, we do not need to think that the user does not have a barcode scanner, since the classes provided by the library will take care of this. By integrating ZXing into our application, we can provide the user with an easier way to scan the barcode, and this will also allow us to focus on developing the main part of the application.
Creating a new project
Step 1
In Eclipse, create a new Android project. Enter the application name, project and package name.

')
Step 2
Open the main template. Eclipse should have created a standard template. Inside it, replace the existing content with a button.
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" > <Button android:id="@+id/scan_button" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_centerHorizontal="true" android:text="@string/scan" /> </RelativeLayout>
After the button, add two text fields that will display the results of the scanned information.
<TextView android:id="@+id/scan_format" android:layout_width="wrap_content" android:layout_height="wrap_content" android:textIsSelectable="true" android:layout_centerHorizontal="true" android:layout_below="@id/scan_button" /> <TextView android:id="@+id/scan_content" android:layout_width="wrap_content" android:layout_height="wrap_content" android:textIsSelectable="true" android:layout_centerHorizontal="true" android:layout_below="@id/scan_format" />
Add text to the button. Open
res / values ​​/ strings file
<string name="scan">Scan</string>
To scan the user must click on the button. When an application receives a recognition result, it displays it in text fields.
Integrating ZXing
Step 1
ZXing is an open source library providing the ability to recognize barcodes on Android. Some users already have an installed ZXing application, so just pass the code to it and wait for the results. In this application, we consider the method that calls the corresponding function of another application. If the user does not have this application, you will be prompted to download it.
In Eclipse, add a new package to your project. To do this, right-click on the
src folder and select “New” -> “Package”, and then enter
com.google.zxing.integration.android as the name of the package.

Step 2
Eclipse offers several ways to import existing code into a project. In this article, the simplest method will be the creation of two classes containing the code from ZXing. Right click on your project, select “New” -> “Class” and enter “IntentIntegrator” as the class name. The remaining parameters you can not change. Once you have created a class, do the same, but name the class “IntentResult”.
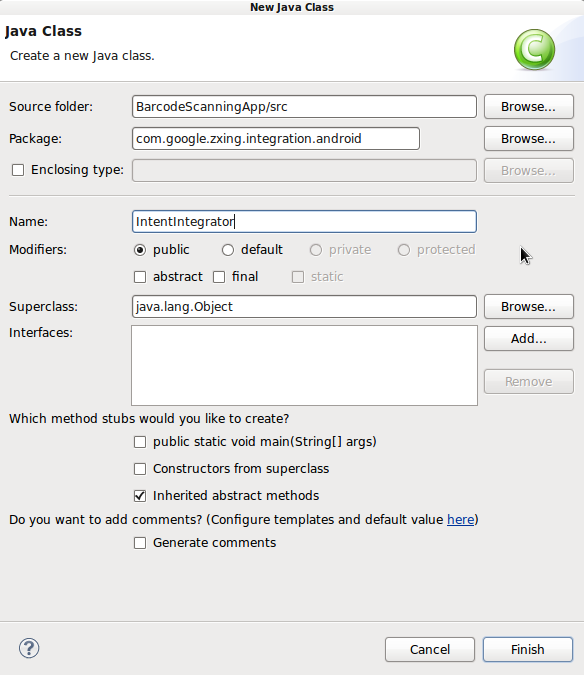
Copy the code from both library classes, and then paste it into the generated classes.
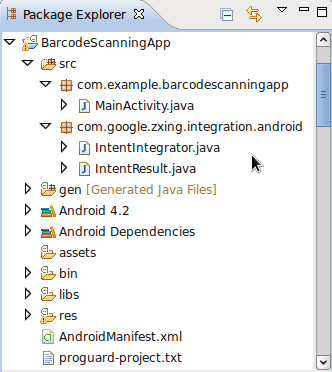
Now you can connect files to the main class
import com.google.zxing.integration.android.IntentIntegrator; import com.google.zxing.integration.android.IntentResult;
Let's go back for a moment and also include the following files. Please note that Eclipse could already connect them for you.
import android.os.Bundle; import android.app.Activity; import android.content.Intent; import android.view.View; import android.view.View.OnClickListener; import android.widget.Button; import android.widget.TextView; import android.widget.Toast;
Examine the contents of the two classes. After examining them you will find that they do not read the code. These two classes are simply interfaces that provide access to scanning functionality.
We scan
Step 1
Let's implement a scan when a user clicks our button. In the main application file, there is an onCreate method that should look something like this.
protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); }
Before this function, create the following variables that will store our button and two text fields created in the template
private Button scanBtn; private TextView formatTxt, contentTxt;
After the existing code in onCreate, add lines that will initialize the variables
scanBtn = (Button)findViewById(R.id.scan_button); formatTxt = (TextView)findViewById(R.id.scan_format); contentTxt = (TextView)findViewById(R.id.scan_content);
Now, add a click handler.
scanBtn.setOnClickListener(this);
Extend the class to declare the OnClickListener interface.
public class MainActivity extends Activity implements OnClickListener
Step 2
Now, we can respond to pressing the button to start the scanning process. Add onClick method
public void onClick(View v){
Check if the scan button was pressed
if(v.getId()==R.id.scan_button){
Inside the condition block, create an instance of the IntentIntegrator class that we imported
IntentIntegrator scanIntegrator = new IntentIntegrator(this);
Now, let's call the procedure that starts the scan.
scanIntegrator.initiateScan();
At the moment, recognition should start, but only if the user has the necessary application installed. If not, then you will be prompted to start the download. The result of the scan will be returned to the application.
Processing scan results
Step 1
The scanner will start when the button is pressed. Then the result will be returned to the onActivityResult method. Add it to our code.
public void onActivityResult(int requestCode, int resultCode, Intent intent) {
Inside the function we will try to process the result.
IntentResult scanningResult = IntentIntegrator.parseActivityResult(requestCode, resultCode, intent);
Step 2
Like any other data received from another application, it would not be bad to check that the result is not empty. We will continue only if we have the correct result.
if (scanningResult != null) {
If we have not received the result of the scan (for example, the user canceled the scan), then we will simply display a message
else{ Toast toast = Toast.makeText(getApplicationContext(), "No scan data received!", Toast.LENGTH_SHORT); toast.show(); }
Let's return to the block with the condition, let's look at what the library has returned to us. The Intent Result object has a method that provides the result of the scan. Get the scan result as a string.
String scanContent = scanningResult.getContents();
Also, we get the barcode view.
String scanFormat = scanningResult.getFormatName();
Step 3
Now, our application has all the necessary information to display. In our article we will simply display it to the user.
formatTxt.setText("FORMAT: " + scanFormat); contentTxt.setText("CONTENT: " + scanContent);
Run our application on a real device instead of an emulator to see how the barcode recognition functionality works. Try scanning a barcode from any book or any other product.
Scan results
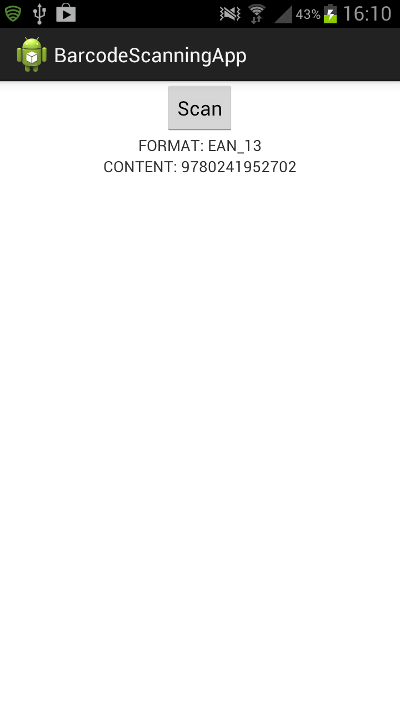
Conclusion
In this article we learned how to scan barcode using ZXing. In your own applications, after extracting the information, you probably want to process it, for example, if the barcode contains a URL, then go to it, etc.