ForewordEternal thrust to the new pushed for the study of such a wonderful programming language like Python. As is often the case, the absence of an idea, the implementation of which is not a pity to spend your time, greatly slowed down the process.
By the will of fate, I came across a remarkable series of articles on the creation of a platform game on Python
here and
here .
I decided to take on one old project. For the simulator of the movement of bodies under the action of gravitational forces.
What came of this, read on.
')
Part one. TheoreticalTo solve a problem, you must first clearly imagine it.
Suppose, by hook or by crook, we managed to get a two-dimensional piece of airless space with the bodies in it. All bodies move by gravity. There is no external impact.
It is necessary to build the process of their movement relative to each other. The simplicity of implementation and the brilliance of the final result will serve as an incentive and reward. Mastering Python will be a good investment in the future.
We introduce a coordinate system.
Let our system consists of two bodies:
1. massive star of mass M and center (x0, y0)
2. light planet of mass m, with center at point (x, y), speed v = (vx, vy) and acceleration a = (ax, ay).
When we manage to disassemble this case, we can easily move on to complex systems with the mutual influence of the stars and planets on each other. Now we will talk about the simplest.
After simple manipulations with Newton's second law, the law of world wideness, and similar triangles, I found that:
ax = G * M * (x0-x) / r ^ 3
ay = G * M * (y0-y) / r ^ 3
This allows you to create an algorithm for moving the planet in the gravitational field of a star:
1. Before the start we set the initial position of the planet (x, y) and the initial velocity (vx, vy)
2. At each step, we calculate the new acceleration using the formula above, then recalculate the speed and coordinates:
vx: = vx + T * ax
vy: = vy + t * ax
x: = x + T * vx
y: = y + T * yx
It remains to deal with the constants G and T. Put G = 1. For our problem, this is not so important. The parameter T affects the accuracy and speed of the calculations. Also put 1 to start.
Part two. PracticalSo, my first program on Python. At the same time once again I want to thank
Velese for the practical guidance.
import pygame, math from pygame import * from math import * WIN_WIDTH = 800 WIN_HEIGHT = 640 PLANET_WIDTH = 20 PLANET_HEIGHT = 20 DISPLAY = (WIN_WIDTH, WIN_HEIGHT) SPACE_COLOR = "#000022" SUN_COLOR = "yellow" PLANET_COLOR = "blue"
This is how our system looks after some simulation time.

While this note was being written, the simulator expanded with new functionality: the number of objects in the star system is not limited, their mutual influence on each other is taken into account, the calculated part is moved to its class, the system configuration is set in a separate file and the system selection option is added.
Now I’m looking for interesting system scripts and minor interface improvements.
Here is an example of what is currently in development:
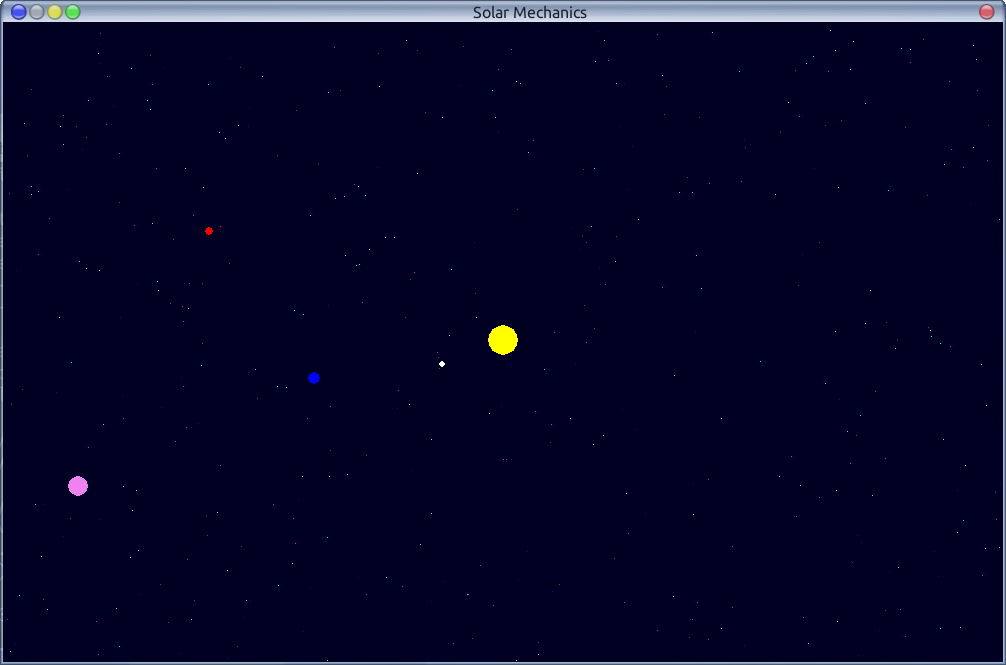
If this article meets with positive reviews, I promise to continue the story of a newer version.
Update:
1. I am grateful to all commentators for critical comments. They give great food for thought.
2. The project has grown. All bodies are already independent, they affect each pipe in accordance with the law of the world. N ^ 2 interactions are counted.
Now it is possible to store the star system configurations in external files and select at the start.
Code hereRun like this: python3.3 main.py -f <configuration name> .ini
Different configurations are there.
3. Thanks to the comments, it was possible to find and eliminate the main flaw - the method of calculating coordinates.
The Runge-Kutta method is now used. As I read Non-Rigid Tasks, I will learn new methods.