What is the reason that the java program stops all its activity and terminates its execution? The main reasons may be 2 (
JLS Section 12.8 ):
- All threads that are not demons are executed;
- Which thread calls the exit () method of the Runtime class or the System class and
SecurityManager gives the go-ahead to exit ().
In both cases, during the shutdown process, the JVM passes to its parent process (the process that launched the JVM) the exit code - an int value, based on which the parent process can draw conclusions about the success or failure of the tasks assigned to the JVM.
Based on common practice and established standards, an exit code equal to 0 indicates that the task has been completed successfully.
public class Example1 { public static void main(String args[]) { System.out.println("Example 1"); } }
It is the value 0 that returns the JVM in the first, above-described case upon its completion.
')
How can this be verified?
Here we come to the aid of the command line parameter
ERRORLEVEL , which allows you to get the exit code of the program that we ran last.
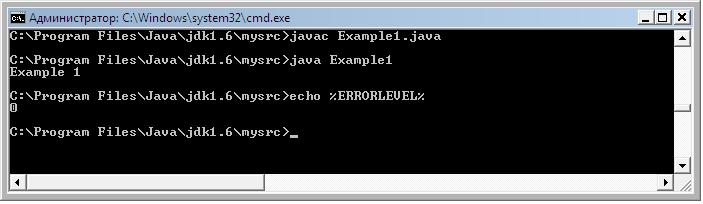
As you can see from the screenshot, the exit code is 0.
We proceed to the second case, using the exit () method.
What values can be passed to the exit method? Based on the
documentation :
The argument serves as a status code; by convention, a nonzero status code indicates abnormal termination.
Yeah, option two: either 0 or any non-zero value. The second variant can be divided into two more variants: any negative number and any positive integer type.
So, try the option with 0:
public class Example2 { public static void main(String args[]) { System.out.println("Example 2 - Start"); System.exit(0); System.out.println("Example 2 - End"); } }
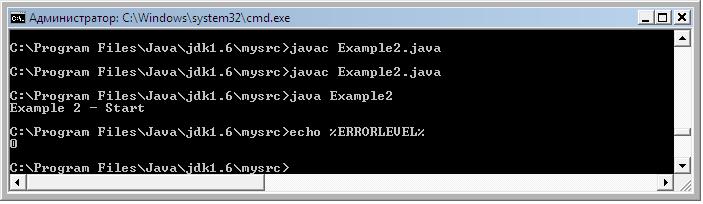
As you can see, the parent process again received information about the successful completion of the JVM.
And what about nonzero values? What, when and in what case to transfer?
Everything is simple - there are no requirements for nonzero values and one should follow only one general rule:
- We return an exit code with a value> 0 in case we expected something bad to happen and it did happen, for example: incorrect data in args [], or it was not possible to find some important file for the application, or could not connect to server and so on.
- We return an exit code with the value <0, in the case when something happened that we could not even predict and did not expect at all (some kind of system errors).
We can also be guided by the following approach - to return such an exit code value that we ourselves desire. This option can be used if we know that no parent process (in our case, cmd) does any additional processing of the exit code, in which case we are free to return any value we want, this will absolutely
not affect anything .
And the last option: if we know that the exit code is additionally processed and in order not to invent values by ourselves, we can borrow the values that are used to the operating system levels.
For example, for the OS of the Windows family there is a whole list of 15999 codes:
System Error Codes , for the Linux family your list:
sysexits.h