I was looking for how to compare C ++ programming and I remembered a 1990 film by director Tim Burton -
"Edward Scissorhands" 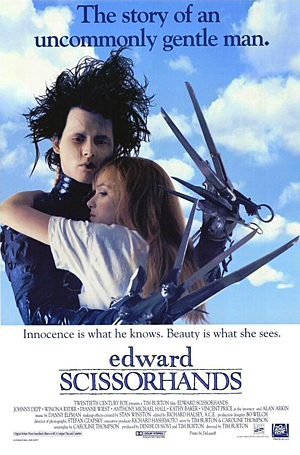
This is a dark version of Pinocchio, shot in a suburban atmosphere. In this film, a creepy guy (Johnny Depp) tries to gently hug Vanona Ryden, but his clumsy scissor arms make it very dangerous for both of them. His face is already covered with deep scars.
If you have scissors instead of hands, then this is not so bad. Edward has a lot of talent: for example, he can cut dogs amazingly!
I am often visited by similar thoughts after attending a C ++ conference: this time it was after
Going Native 2013. Last year, there was enthusiasm and excitement about the new standard - C ++ 11. This year it was a reality check. Don't get me wrong: there were a lot of amazing dog hairstyles (I mean C ++ code, which was simple and elegant), but the main part of the conference was how to avoid injury and provide first aid in case of an unintended amputation.
Little shop of horrors.
There was so much talk about how not to use C ++, that it gave me such an idea: this is not about the problem of incompetent programmers, just using C ++ is generally wrong. So, if you only learn the basics of a language and try to use it, then you are doomed.
C ++ has an excuse: backward compatibility, in particular, compatibility with C. You can treat C as a subset of C ++, really assembly language, which you better not use in everyday programming, except in situations where obviously necessary. If you are blindly immersed in C ++, then you are thinking about clean pointers,
for- cycles - this is all really a silly idea.
A well-known example of how not to do is to use
malloc to dynamically allocate memory and
free to release it.
malloc takes the number of bytes and returns a pointer to void, which you need to cast to something more convenient - to think up the worst API for managing the memory is hard. Here is an example of a really bad (but almost correct, if it were not for the ability to access by the null pointer) code:
struct Pod {
int count;
int * counters;
};
int n = 10;
Pod * pod = (Pod *) malloc (sizeof Pod);
pod->count = n
pod->counters = (int *) malloc (n * sizeof(int));
...
free (pod->counters);
free (pod);
, ++ , , , .
++ «»
malloc free new delete. ++ :
struct Pod {
int count;
int * counters;
};
int n = 10;
Pod * pod = new Pod;
pod->count = n;
pod->counters = new int [n];
...
delete [] pod->counters;
delete pod;
, ,
new , . ,
new ( ? :
n?). , - :
class Snd { // Sophisticated New Data ( POD)
public:
Snd (int n) : _count(n), _counters(new int [n]) {}
~Snd () { delete [] _counters; }
private:
int _count;
int * _counters;
};
Snd * snd = new Snd (10);
...
delete snd;
? ! .
++ ,
delete.
,
malloc new, : , — .
( ), STL- , . , value- . ! Value- - .
shared_ptr shared_ptr? ! , : move- rvalue-.
( !). ? . -, . . , , . ( , , ).
++
, ++.
. , . . , ++, , , , — - .
++ . — , , . , . , , .
, .
, . : , ( , ) . ++, .
, 10 « » ++ ( ). , ++, . ++ : mutation, , , .
, — ++ .
, ( ) .
, , , ++ 10 .
" , , ++. , , .
( , ), . (. ). , , 20 . ++ . , ++.
, , , . , .
? ++ — . , , : , , . , . , ++ , , , ++ . : , ( ++ ).
, , ( , shared-) .
, . , shared- , . , , , , - , , , . , shared-, , , , .
shared_ptr , , . ! , ! : . ++.
8 , :
« !»++ . 2005 . Posix- 1995 . Windows 95, — Windows NT. ++ 2011 .
++ 11 . : , , . , ++ Java ( , Java ). , ++ , . ++ , , .
C++11 , , , . , - , , . — STM (Software Transactional Memory), . STM, , STM . STM , ++ .
task-
future ( , deprecated- ++ 14). task- , . , . . . task- , , futures ( ), task' , , , GPU.
Microsoft PPL Intel TBB (, Microsoft AMP ).
, , , 2015 . , , ++ . ++ , , . : data race. — , D.
++ , , . , . ++, , , . , - .
D, , ( ). D — . D . , . : , , .
++ , - .
, - — , .
: , GPU. ++. , , , . —
«Parallel and Concurrent Programming in Haskell». ++, , .
, ++ . . , GPU - .
:
- Bartosz Milewski, “Resource Management in C++,” Journal of Object Oriented Programming, March/April 1997, Vol. 10, No 1. p. 14-22. unique_ptr, auto_ptr, . auto_vector, auto_ptr.
- C++ Report in September 1998 and February 1999 (auto_ptr ).
- C++ in Action (still auto_ptr), Addison Wesley 2001. , .
- Walking Down Memory Lane, with Andrei Alexandrescu, CUJ October 2005 ( unique_ptr)
- unique_ptr–How Unique is it?, WordPress, 2009