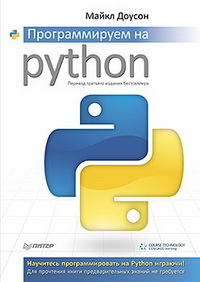
Periodically, we receive letters to the editor asking whether there will be a new edition of the book βWe Program in Pythonβ by M. Lawson. Despite the fact that the original βPython Programming for the Absolute Beginner, 3rd Editionβ was released in 2010, it is still at the top on
amazon.com . The content of the book is based on practical examples of programming simple games. We are very interested to know the opinion of professionals, which will make it possible to decide whether to publish a book.
Full table of contents can be viewed
here .
')
Excerpt from Chapter 5. Lists and dictionaries. Game "Hangman"
Tuples are a good way to manipulate elements of different types in the same sequence. But from the fact that the tuple is immutable, sometimes there are inconveniences. Fortunately, there are sequences of another kind, so-called lists (lists), which can do the same thing as tuples, and even more - simply because the list is modified. Its elements can be deleted as well as add new ones. You can even sort the list. I will introduce you to another kind of sequence - dictionaries. If the list is organized as a set of values, then the dictionary is like a set of pairs of values. Like its namesake from the bookshelf, the dictionary allows you to find the value that corresponds to some other. In more detail, in this chapter you will learn how to do the following:
β’ create lists, index them and make cuts;
β’ add and remove list items;
β’ apply list methods that allow you to sort the list and add a value to the end;
β’ apply nested sequences, which can provide data of any complexity;
β’ use dictionaries for working with value pairs;
β’ add and remove dictionary items.
Getting to know the game "Hangman"
The focus of this chapter is on the Hangman project. Secretly from the user, the computer chooses a word, and the player must try to guess it, expressing his assumptions by letter. Every time a player makes a mistake, the computer draws an image of a figure under the gallows on the screen. If the player fails to guess the word in the allotted number of attempts, the βhangedβ person dies. In fig. 5.1-5.3 shows the gameplay in all its terrible magnificence.
The game is interesting not only in itself. It is also remarkable that by the end of the study of this chapter you will learn how to create your own version of the game. You can, for example, acquire your personal list of "secret" words or replace my primitive drawings with more impressive ones.
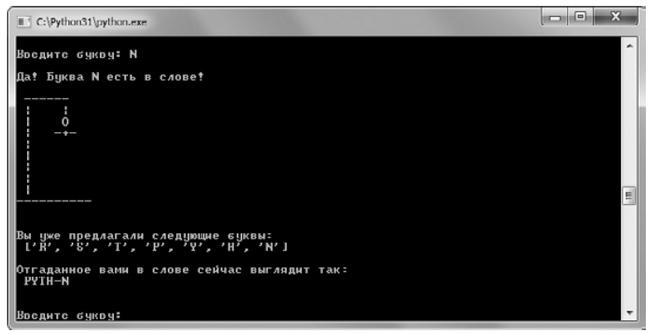
Fig. 5.1. We play "Hangman". Um ... What kind of word did he make?
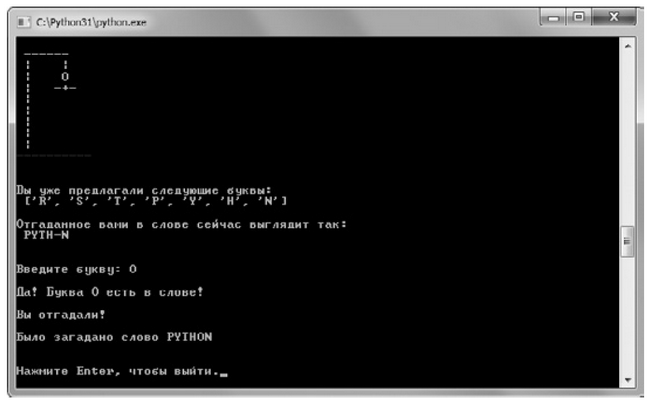
Fig. 5.2. In this game I won!

Fig. 5.3. And this game did not end well, especially for the pseudo graphic man.
Using Lists
Lists, like tuples, are sequences. But the lists are changeable. Their elements are amenable to modification. This is why lists have the same functionality as tuples, and can do something else. What you already know about working with tuples also applies to lists, which means it will not be difficult for you to learn how to use them.
Acquaintance with the program "Hero Arsenal 3.0"
This program is based on the earlier game βHero 2.0 Arsenalβ, which we met in Chapter 4. Here, not tuples, but lists are used to store data about the hero's arsenal. The initial part of the Arsenal of Hero 3.0 gives the same results as the earlier version. And the code in it is almost the same; the only difference is that lists are used instead of tuples. In fig. 5.4 shows the program window after the execution of this first part. The code following it demonstrates the useful implications of list volatility and some new techniques for working with sequences. The execution of this part of the program is reflected
in fig. 5.5.

Fig. 5.4. Now the heroβs arsenal is presented in a list. In the program window, everything looks almost the same as it looked in version 2.0, where the tuple was used
Create a list
In the first lines of the code, a new list is created, which becomes the value of the inventory variable; the system displays the elements of the list on the screen. The only difference from the Arsenal of Hero 2.0 is that I put the list items in square brackets rather than in parentheses and got a list, not a tuple. The code for this program can be found on the helper site (courseptr.com/downloads) in the folder Chapter 5. The file is called hero's_inventory3.py.
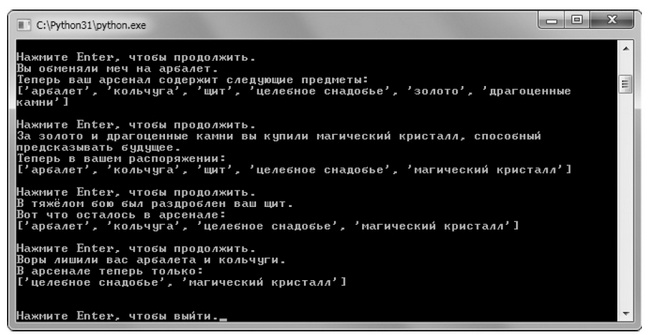
Fig. 5.5. Since the heroβs arsenal is a list, its elements can be added, modified and deleted.
# 3.0
#
# for-
inventory = [ββ, ββ, ββ, β β]
print(β\n, :β)
for item in inventory:
print(item)
input(β\n Enter, .β)
Apply the len () function to lists
The following code literally coincides with the similar code in the Hero 2.0 Arsenal. The len () function works with lists in the same way as with tuples.
#
print(β β, len(inventory), β/-.β)
input(β\n Enter, .β)
Application of the in operator to lists
This code is also unchanged borrowed from the previous version. The in operator does not see the difference between a tuple and a list.
# in
if β β in inventory:
print(β .β)
Indexing lists
Again, nothing new compared to version 2.0. To access the list item, as before, you need to enclose in brackets the position number in which the item is located.
#
index = int(input(β\n : β))
print(β β, index, β β, inventory[index])
List slices
Are you ready to believe that the list slices are the same as the tuple slices? Again, we indicate the starting and ending positions through a colon in square brackets:
#
start = int(input(β\n : β))
finish = int(input(β : β))
print(β inventory[β, start, β:β, finish, β] β β, end=β β)
print(inventory[start:finish])
input(β\n Enter, .β)
Clutch lists
Linking lists is no different from linking tuples. The difference here will only be that I assigned the list to the variable chest, not the tuple. This is a small but significant difference, because only single-type sequences can be concatenated.
#
chest = [ββ, β β]
print(β . :β)
print(chest)
print(β .β)
inventory += chest
print(β :β)
print(inventory)
input(β\n\n Enter, .β)
List Variability
It must be assumed that you have already tired of countless phrases, the general meaning of which is that the list functions in the same way as a tuple. So far, except for the replacement of round brackets with square brackets, the lists have not proved anything new in comparison with the tuples. But the difference is still there, and it is huge. I repeat: the lists are changeable. It follows that the lists can do a lot of tricks for which the tuples are unsuitable.
Assigning a new value to an item selected by index
Since the list is modified, any element can be assigned a new value:
#
print(β .β)
inventory[0] = ββ
print(β :β)
print(inventory)
input(β\n Enter, .β)
inventory, 0,
ββ:
inventory[0] = ββ
This new string value replaces the old (βswordβ). How the list began to look, shows the function print, with which the new version of inventory is displayed.
TRAP
You can assign a new value to an existing list item by selecting it by index, but you cannot create a new item in this way. Attempting to assign any value to an element that did not previously exist will cause an error.
Assigning new values ββto the slice list
It is allowed to assign a new value not only to a separate element of the list, but also to a slice. I assigned inventory [4: 6] the value of a list from one element [βmagic crystalβ]:
#
print(β , .β)
inventory[4:6] = [β β]
print(β :β)
print(inventory)
input(β\n Enter, .β)
Two elements of the list, inventory [4] and inventory [5], are assigned a single string value βmagic crystalβ. Since the singleton list has become the value of a two element slice, the length of the inventory sequence has decreased by one.