Tell-Don't-Ask is a principle that helps us remember that object-oriented programming is designed to bundle data and functions for processing it. It reminds us that instead of asking for data from an object, we have to tell the object what to do with it. To do this, all the behavior of the object must be concluded in its methods.
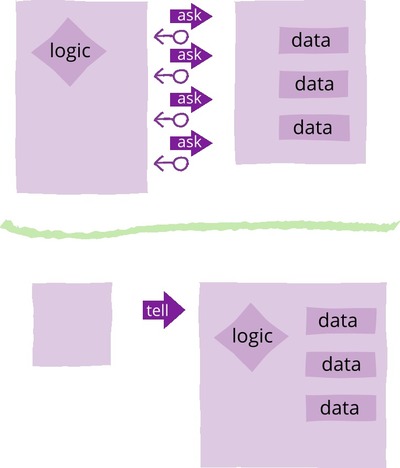
We will understand by example. Let's imagine that we need to control the alarm, which will work if the number rises above a certain bar. If we write it in the “ask” style, we get the data structure ...
class AskMonitor... private int value; private int limit; private boolean isTooHigh; private String name; private Alarm alarm; public AskMonitor (String name, int limit, Alarm alarm) { this.name = name; this.limit = limit; this.alarm = alarm; }
... then compatible with data access methods:
class AskMonitor... public int getValue() {return value;} public void setValue(int arg) {value = arg;} public int getLimit() {return limit;} public String getName() {return name;} public Alarm getAlarm() {return alarm;}
And would use it like this:
AskMonitor am = new AskMonitor("Time Vortex Hocus", 2, alarm); am.setValue(3); if (am.getValue() > am.getLimit()) am.getAlarm().warn(am.getName() + " too high");
“Tell-Don't-Ask” reminds us that the behavior should be described inside the object (using the same fields):
class TellMonitor... public void setValue(int arg) { value = arg; if (value > limit) alarm.warn(name + " too high"); }
And it will work like this:
TellMonitor tm = new TellMonitor("Time Vortex Hocus", 2, alarm); tm.setValue(3);
Many people find the principle of "Tell-Don't-Ask" useful. One of the fundamental principles of object-oriented design is the combination of data and behavior, so the simple elements of the system (objects) combine it in themselves. This is often good because the data and its processing are closely related: changes in one cause changes in others. Things that are closely related should be one component. Remembering this principle will help programmers see how you can combine data and behavior.
But personally, I do not use this principle. I just try to put data and behavior in one place, which often leads to the same results. One of the problems is that this principle encourages people to remove methods that request information. But it happens when objects cooperate effectively by providing information. A good example would be objects that simplify information for other objects. I saw code that is so twisted that suitable requesting methods would solve this problem. For me, the “Tell-Don't-Ask” is a step towards combining behavior and data, but this is not a primary task.
Note translator: I doubt the need to translate the name of the principle, but as one of the options I propose - “ask, but don't ask”