Foreword
Hi, Habr! Just recently, they took me to a circle on robotics. Of course, I gladly agreed, this is a new experience and all that ... Especially since I'm just a freshman. My teacher, explaining to me the general concept, offered to take up work with the Raspberry Pi. It was necessary to figure out how to work with it, install the JDK on it and write a program that would display the readings from the 3-axis accelerometer. Taking everything you need, I went home to understand. When I finished everything (it took about a week), I decided to write a guide, addressed the same as me, in order to collect everything I dug in one place. Well, enjoy reading!
First thing
The first thing you need to access our device. We will connect using the SSH protocol. By default, the SSH server is disabled, and to enable it, connect the Raspberry to the monitor or TV, connect the keyboard and mouse. Of course, you can simply scan the network environment, identify our device and connect using the found IP, but still I recommend doing it in my version. After loading the startup terminal and enter the command
sudo raspi-config . In the menu that opens, go to advanced options-> SSH and select Enable. After these manipulations, the following window should appear:

Fine! Now we can touch our “Malinka” at any time. Let's write out the network settings right away. The file we need is in the
/etc/network/ directory. We go there using the
cd / etc / network command . Next, open the desired file by entering
sudo nano interfaces . Here we enter our data in the corresponding lines, thus we set a static IP address. You should have something like this:
')

After press CTRL + X, Y, Enter. We will connect to this address. Next, download the client
SSH - PuTTY . Running the program, you will see the following window:

Here in the Host Name field we write the same ip, which we registered in interfaces. Here you can save the settings by entering the name in the Save Session field and clicking on Save. Click Open. As soon as PuTTY connects to the SSH server, a window will open and you will be prompted for your credentials (by default, user: pi, password: raspberry):
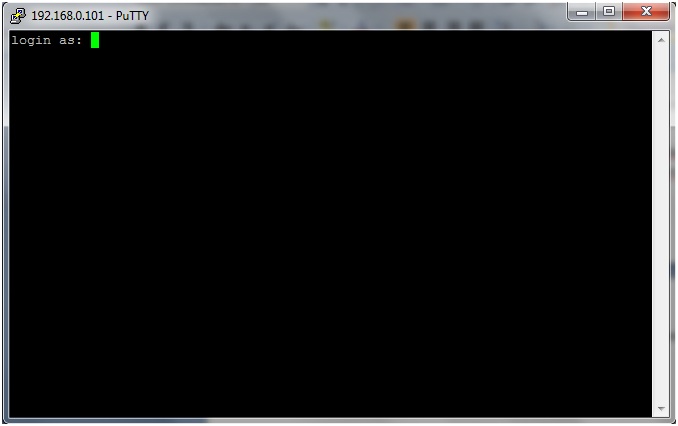
Do not worry that when you type you do not see the password, in Linux like systems the password is simply not visible, although the input occurs. Press Enter. If everything went well, you will see the following window:
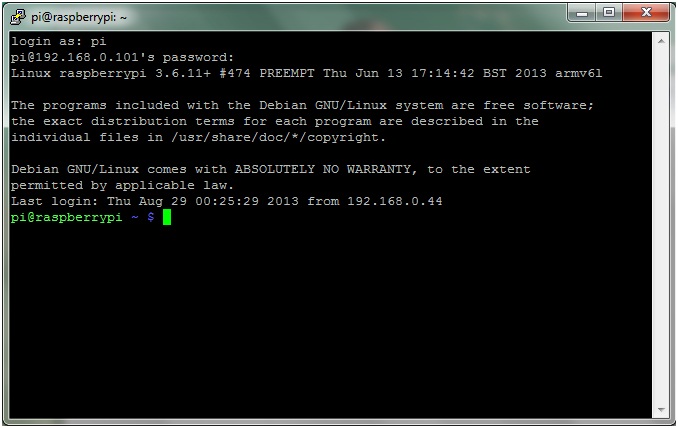
In this console, you can execute any commands, and they will be executed on the device itself. Is it not convenient?
JDK installation
Everything is pretty simple here. We
update the list of packages by entering
sudo apt-get update in the terminal.

Update the
sudo apt-get upgrade system itself.

All this may take quite a lot of time (I have this update took about 30 minutes), so stock up on tea. Glory to the great power that the JDK package was added to the package lists, and we did not have to dance with a tambourine. To install the package, just type
sudo apt-get install openjdk-7-jdk .
To test the performance, enter java -version. You should see something like this:

PS On some machines, there may be a problem due to the fact that the system does not find the JDK package. It arises from the incompatibility of the Debian “wheezy” kernel with java. Try reinstalling the OS on a Soft-float Debian “wheezy” optimized for working with java. In any case, on my “Malinka” everything fell on the first try. Here is my version:

I2c vs Raspberry PI
So, now the fun begins! For a start, let's see what it is all about? The great and mighty Wikipedia says:
I²C (Rus. Ay-tu-si / u-two-tse / and-two-si) is a serial data bus for interconnecting integrated circuits using two bidirectional communication lines (SDA and SCL). Used to connect low-speed peripheral components to the motherboard, embedded systems and mobile phones. The name is an abbreviation of the words Inter-Integrated Circuit.In my work, I use this
LSM303DLM device, in the future all the examples will be shown on it. Below I have drawn a wiring diagram:

It is quite simple, and I think it does not require clarification. We continue. Oh yeah, let's immediately add a couple of lines to the file responsible for autoloading the modules. We set
sudo nano / etc / modules in the command line and append there:
i2c-bcm2708
i2c-dev
Now install the i2c-tools package. In the terminal, we write:
sudo apt-get install python-smbus and
sudo apt-get install i2c-tools .
Edit the blacklist. To do this, we write sudo nano /etc/modprobe.d/raspi-blacklist.conf. Add the lines:
blacklist spi-bcm2708
blacklist i2c-bcm2708
Everything. We have done everything necessary for working with I2C, now let's check if we can see our device. The i2c-tools utility includes the i2cdetect command, and we need it. But first, check that the drivers have been successfully installed. We write in the terminal
i2cdetect –l . If we see the following:

That's all right, you can move on. Now we write
i2cdetect –y 1 (
i2cdetect –y 0 ). We see the address grid:

In my case, the address of the accelerometer is 0x18 (you can find out, for example, in the documentation). Now let's talk about PI4J.
Wow Wow Wow Palekh ...
To work with I2C using JAVA tools, you need to download and install the additional library PI4J. Read about it
here . Download by writing in the terminal
wget pi4j.googlecode.com/files/pi4j-0.0.5.deb . Install by writing:
sudo dpkg -i pi4j-0.0.5.deb . Check the installed files:

Now, to compile the program, we write:
javac -classpath.: classes: / opt / pi4j / lib / '*' (title)For start:
sudo java -classpath.: classes: / opt / pi4j / lib / '*' (title)Of all the packages we need 3:
import com.pi4j.io.i2c.I2CBus; import com.pi4j.io.i2c.I2CDevice; import com.pi4j.io.i2c.I2CFactory;
We create links to objects of classes I2CDevice and I2CBus.
import java.io.IOException; import com.pi4j.io.i2c.I2CBus; import com.pi4j.io.i2c.I2CDevice; import com.pi4j.io.i2c.I2CFactory; public class accelerometrOne { static I2CDevice device; static I2CBus bus; static byte[] accel; public static void main(String[] args) throws IOException { System.out.println("Starting sensors reading:"); try { bus = I2CFactory.getInstance(I2CBus.BUS_1); System.out.println("Connected to bus OK!"); device = bus.getDevice(0x18); System.out.println("Connected to device OK!"); } catch (IOException e) { System.out.println(e.getMessage()); } } }
Let's sort the code. With the line
bus = I2CFactory.getInstance (I2CBus.BUS_1); we connect to our bus, and with
device = bus.getDevice (0x18); we connect to our device.
Now you need to calibrate the accelerometer. We climb into the
documentation .

Who is completely bad with English. In my free translation: “After switching on the LSM303DLH, it is necessary to configure the device in 2 registers CTRL_REG1_A (20h) and CTRL_REG2_A (23h). To do this, in the first register we write 0x27 for normal operation with ODR 50Hz.
We write 0 * 40 to the second register in order to preserve the full measurement range + - 2 gauss in the continuous update of the database and change the byte order from low to high by an order of magnitude, from high to low. ”
To write data to the device, we use the
write method from the
I2CDevice class. We will add the program:
import java.io.IOException; import com.pi4j.io.i2c.I2CBus; import com.pi4j.io.i2c.I2CDevice; import com.pi4j.io.i2c.I2CFactory; public class accelerometrOne { static I2CDevice device; static I2CBus bus; static byte[] accel; public static void main(String[] args) throws IOException { System.out.println("Starting sensors reading:"); try { bus = I2CFactory.getInstance(I2CBus.BUS_1); System.out.println("Connected to bus OK!"); device = bus.getDevice(0x18); System.out.println("Connected to device OK!"); device.write(0x20, (byte) 0x27); device.write(0x23, (byte) 0x40); System.out.println("Configuring sensors OK!"); } catch (IOException e) { System.out.println(e.getMessage()); } }
Fine!!! It remains to read the data from the device. Again we climb into the documentation and find out that the data is given out by 2 bytes for each axis:
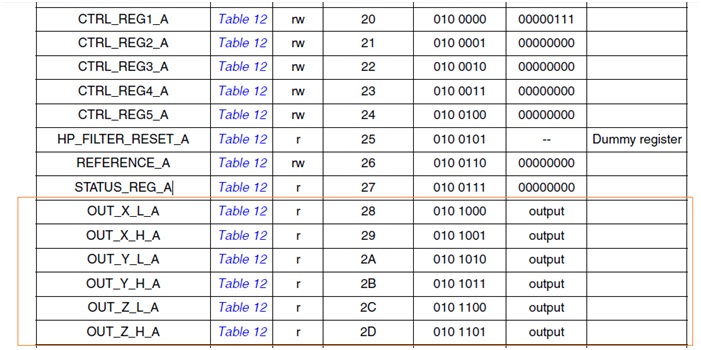
Well, that's it. Write the resulting bytes to the array and display the data on the screen:
import java.io.IOException; import com.pi4j.io.i2c.I2CBus; import com.pi4j.io.i2c.I2CDevice; import com.pi4j.io.i2c.I2CFactory; public class accelerometrOne { static I2CDevice device; static I2CBus bus; static byte[] accel; public static void main(String[] args) throws IOException { System.out.println("Starting sensors reading:"); try { bus = I2CFactory.getInstance(I2CBus.BUS_1); System.out.println("Connected to bus OK!"); device = bus.getDevice(0x18); System.out.println("Connected to device OK!"); device.write(0x20, (byte) 0x27); device.write(0x23, (byte) 0x40); System.out.println("Configuring sensors OK!"); readingsensors(); } catch (IOException e) { System.out.println(e.getMessage()); } } private static void readingsensors() throws IOException { while (true) { accel = new byte[6]; accel[0] = (byte) device.read(0x28); accel[1] = (byte) device.read(0x29); accel[2] = (byte) device.read(0x2a); accel[3] = (byte) device.read(0x2b); accel[4] = (byte) device.read(0x2c); accel[5] = (byte) device.read(0x2d); int accelx = asint(accel[0]) * 256 + asint(accel[1]); int accely = asint(accel[2]) * 256 + asint(accel[3]); int accelz = asint(accel[4]) * 256 + asint(accel[5]); System.out.println("accelx: " + accelx + ", accely: " + accely + ", accelz: " + accelz); } } private static int asint(byte b) { int i = b; if (i < 0) { i = i + 256; } return i; } }
As a result, after the launch, we will see something like the following:
