With the advent of ASP.NET MVC 4 and WebMatrix, the mvc team seeks to make things easier for the developer. Based on reviews, one of the areas for improvement was the security of asp.net.
An ASP.NET MVC 4 Internet project template has added several new very useful features that are built using SimpleMembership. SimpleMembership brought a simple setting of roles and users, and also added support for OAuth. However, the new provider is not compatible with the existing ASP.NET Membership Provider.
In this post I will talk about what SimpleMembership is and how to use it in an ASP.NET MVC 4 project.
')
What is SimpleMembership
- SimpleMembership is designed to replace the previous version of the ASP.NET Membership Provider (ASP.NET Role)
- SimpleMembership solves the same tasks, but makes them easier for the developer and adapts to modern security requirements.
- The
AccountController
controller in the ASP.NET MVC 4 Interner project template requires SimpleMembership and is not compatible with older versions. - The sad news is that the Web Site Administration Tool (WSAT) is not compatible with SimpleMembership.
The ASP.NET Membership System was introduced in 2005. It was designed to solve common tasks, such as registering on a site using a login and password bundle, storing a profile in a SQL Server database. Extension mechanisms have also been added that allow overriding the standard logic of the work of MembershipProvider and RoleProvider. 8 years ago, this mechanism did its job, but today it is an inconvenient tool. If the user profile requires additional fields, all of them are stored in one column and require access to the corresponding provider API calls for access.
In ASP.NET WebPages and WebMatrix many things are presented in a new way, for example, the Razor and SimpleMembership submissions engine.
Why using ASP.NET Membership is not recommended
The standard provider works fine if the following conditions are met: all information will be stored in the full version of the SQL Server database and that all the necessary data are presented as a set of attributes (UserName, Password, IsApproved, CreationDate ...) and other information will be provided using the profile provider.
Full SQL Server Default Required
Most full-featured ASP.NET providers require the full version of SQL Server (because they rely on the work of stored procedures, the SQL Server cache, and other server features). In addition, the default providers will not work on SQL Azure.
Difficulties working with another database
To work with a database other than SQL Server, it is necessary to override the set of provider methods that are strongly oriented towards storing data in a relational database. Firstly, it’s a lot of work to override these methods, and secondly, there’s likely to be a lot of undefined methods containing a
System.NotImplementedException
that doesn’t paint the code.
Model orientation User> Role
Existing data providers are strictly focused on this model, in which the user has a login and password. Of course, additional information can be added through the API, but this model is not suitable for OAuth (there the user does not have a password).
The system is role-oriented will not always be appropriate, it is more convenient to use the model of access rights to individual objects or actions (Claims)
Also requires a hard database scheme with a large number of blob columns.
SimpleMembership improved user system
SimpleMembership was designed just to solve the problems voiced above.
Matthew Osborn in his entry
Using SimpleMembership With ASP.NET WebPages explains that SimpleMembership is designed to easily integrate with your database.
SimpleMembership requires that there are 2 columns in the user table: “ID” and “UserName”. The important part here is that these columns can have any names.

Now you need to tell about this SimpleMembership: add a string to connect to the database.
<connectionStrings> <add name="DefaultConnection" connectionString="Data Source=ARCTURUS\SQLEXPRESS;Initial Catalog=MembershipDemoDB;Integrated Security=True;Pooling=False" providerName="System.Data.SqlClient" /> </connectionStrings>
And define initialization:
WebSecurity.InitializeDatabaseConnection("DefaultConnection", "Users", "Id", "Name", autoCreateTables: true);
After launching the site and processing the initialization attribute, SimpleMembership itself creates the necessary tables for it to work. Our users table will be used as the user table.

SimpleMembership works with the entire line of SQL Server (SQL Azure, SQL Server CE, SQL Server Express and LocalDB). Everything is implemented as SQL calls, which is much better than using stored procedures.
Using EntityFramework with Code First
The problem with ASP.NET Membership is that it stores additional information about the account itself. This means that you cannot directly access profile data. While SimpleMembership doesn't care what table and how user data is stored. You can easily change the table with users, for example, add an address.
[Table("Users")] public class UserProfile { [Key] [DatabaseGeneratedAttribute(DatabaseGeneratedOption.Identity)] public int Id { get; set; } public string Name { get; set; } public string Address { get; set; } }
Now you can easily access this field without using the provider, but directly from the database. This allows you to accept SimpleMembership as a layer between the database and the ASP.NET Membership system.
As it is implemented, I will omit, you can see in the original record
SimpleMembership . It is important to know that the SimpleMembership is inherited from ExtendedMembershipProvider.
ASP.NET MVC 4 Internet template
The default template implements the following mechanism for working with SimpleMembership:

- AccountModel.cs describes the user's base account and includes attributes for the database.
- InitializeSimpleMembershipAttribute.cs just contains information for initializing the provider: which database to use, which fields and other settings.
- AccountController.cs contains calls to the WebSecurity class of the WebMatrix library.
WebSecurity works with any ExtendedMembershipProvider. The default is SimpleMembershipProvider, but you can implement your own.
Configure SimpleMembership
Adding SimpleMembership to an existing project
Although this provider works by default in a project with an ASP.NET MVC 4 Internet template, some (including me) create a project with an empty template. You need to add 2 links: WebMatrix.Data and WebMatrix.WebData. Or install these libraries via NuGet with the same identifiers).
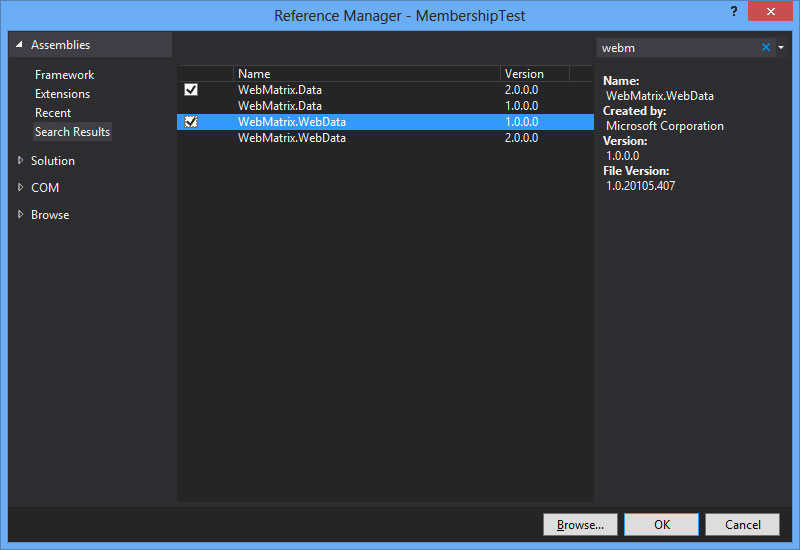
Now you need to add a provider and indicate the use of WebMatrix
<roleManager enabled="true" defaultProvider="SimpleRoleProvider"> <providers> <clear /> <add name="SimpleRoleProvider" type="WebMatrix.WebData.SimpleRoleProvider,WebMatrix.WebData" /> </providers> </roleManager> <membership defaultProvider="SimpleMembershipProvider"> <providers> <clear /> <add name="SimpleMembershipProvider" type="WebMatrix.WebData.SimpleMembershipProvider, WebMatrix.WebData" /> </providers> </membership>
Since WSAT cannot be used, there are 2 ways to create users and roles. If the EntityFramework Code First model is used, it will be convenient to add Micragion with the creation of users by default:
public partial class AddDefaultUser : DbMigration { public override void Up() { if (!WebSecurity.Initialized) { WebSecurity.InitializeDatabaseConnection("DefaultConnection", "Users", "Id", "UserName", autoCreateTables: true); } var roles = (SimpleRoleProvider)Roles.Provider; var membership = (SimpleMembershipProvider)Membership.Provider; if (!roles.RoleExists("Admin")) { roles.CreateRole("Admin"); } if (membership.GetUser("Admin", false) == null) { membership.CreateUserAndAccount("Admin", "SuperAdminPassword"); } if (!roles.GetRolesForUser("Admin").Contains("Admin")) { roles.AddUsersToRoles(new[] { "Admin" }, new[] { "Admin" }); } } public override void Down() { throw new NotImplementedException(); } }
Alternatively, you can directly create a user role in the database editor and assign a role to the user.
Links