As requested in the comments to the
previous article on EmBlocks, today I will show from start to finish how to create a simple project in EmBlocks for flashing a pair of LEDs.
As a debug board, we will use the crumbs on the
STM32F103C8 .
Here is our stand:
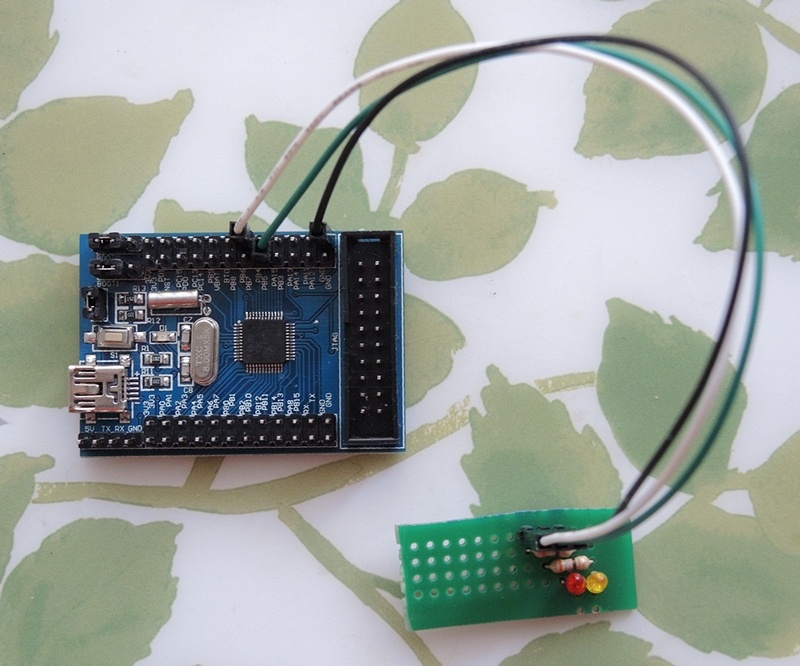
We connect LED anodes to PB5 and PB6 pins, cathodes through resistors of 390 Ohms to ground.
So, if you haven’t downloaded
EmBlocks yet , do it. Unpack to any convenient directory and run.
Create a project by selecting in the menu "File-> New-> Project ..."
In the Projects category, select "STmicro-Arm".
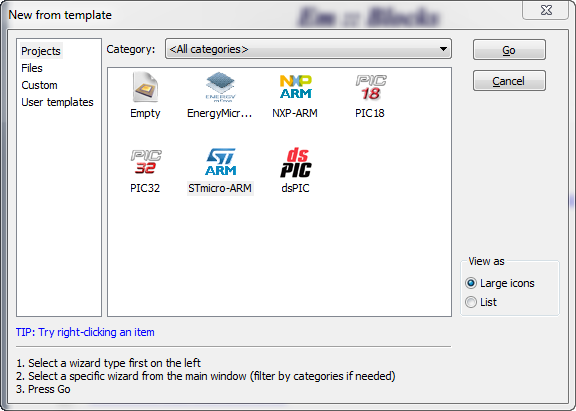
We name the project and select the folder in which it will be created. In the future, new projects will be automatically saved there, if you do not choose another.
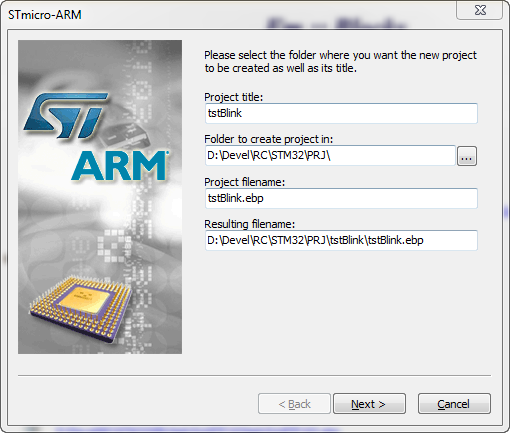
In the compiler selection window, do not touch anything and move on.
In the processor selection window from the first list, select “STM32F10x_md” because STM32F103C8 belongs to the F1 family and contains 64k FLASH, which relates it to medium density devices.
If you use ColinkEx, then select the specific processor name in the next window, this is necessary for the CoFlash utility firmware.
If you use ST-Link, then you can not touch.
The “Create hex file” checkbox is responsible for creating the .hex output instead of the .elf file. ColinkEx accepts both, but ST-Link Utility only .hex
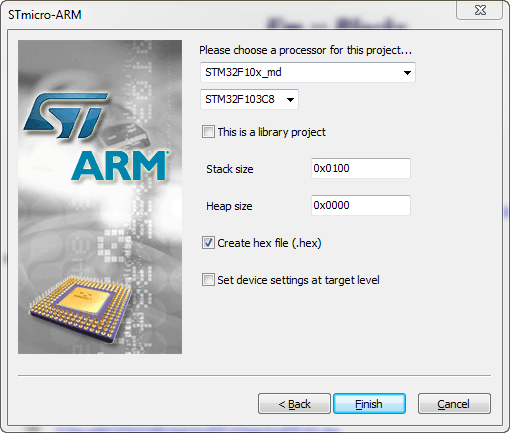
Click Finish. The wizard prompts you to select a debugger and configure it. And twice - for the purpose of Debug, and then for Release ... If you use another, click cancel and select another debugger. I will tell you about other debuggers some other time.
ST-Link is offered by default, it suits us, so just click
Ok twice for each target (Debug, Release)
Everything, the project pig is ready. If you now press F7, the project will be compiled and ready for the firmware with a reminder, which is already taken into account in the Release settings of the target.
Let's analyze the project structure:

All project files are automatically decomposed into
Sources ,
Headers, and
ASM Sources folders for
.c ,
.h, and
.S files, respectively.
In the
Sources folder, we have a cmsis_boot subfolder with the CMSIS library file.
In the
stm_lib \ src subfolder , we already have a couple of files that are needed in almost any project:
stm32f10x_gpio.c
stm32f10x_rcc.c
These are parts of the
StdPeriph Library for working with the GPIO and clocking system.
In the
Src subfolder is the main.c file - the template for our program.
')
In the
Headers folder, headers are laid out in exactly the same folders.
In the future, we will add the necessary parts of the StdPeriph Library to the
stm_lib \ inc and
stm_lib \ src subfolders and include them in the project by right-clicking on the name and selecting “Add files ...” or “Add files recursively ...”. But today we will not need it.
The startup_stm32f10x_md.S file in the
ASM Sources \ cmsis_boot \ startup folder is responsible for launching the microcontroller.
The linker script is in the
Others folder and is called gcc_arm.ld
With the structure of the project, we briefly met each other, it's time to write the code for which we were starting a business.
Open the file
Sources \ Src \ main.c and replace the text in it with this:
#include <stm32f10x.h> #include <stm32f10x_conf.h> #include <stm32f10x_rcc.h> #include <stm32f10x_gpio.h> #define RCC_GPIO RCC_APB2Periph_GPIOB #define LED_PORT GPIOB #define LED1_PIN GPIO_Pin_5 #define LED2_PIN GPIO_Pin_6 void Delay(volatile uint32_t nCount) { for (; nCount != 0; nCount--); } int main(void) { /* SystemInit() startup_stm32f10x_md_vl.S */ GPIO_InitTypeDef GPIO_InitStructure; RCC_APB2PeriphClockCmd(RCC_GPIO, ENABLE); GPIO_InitStructure.GPIO_Pin = LED1_PIN | LED2_PIN; GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP; GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz; GPIO_Init( LED_PORT , &GPIO_InitStructure); LED_PORT->ODR ^= LED2_PIN; while (1) { LED_PORT->ODR ^= LED2_PIN; LED_PORT->ODR ^= LED1_PIN; Delay(0x7FFFF); } return 0; }
We defined the macros RCC_GPIO, LED_PORT, LED_PIN1 and LED_PIN2, by changing which we can connect the LEDs to the pins of another port.
In the main () function, we fill in the GPIO_InitStructure structure, tuning the pins PB5 and PB6 to operate in PushPull mode with a maximum frequency of 50MHz.
Then we invert the LED_PIN2 state so that the LEDs blink and in a cycle we switch them with a small delay.
We press
F7 , we are convinced that the project is assembled without errors

We connect the ST-Link / v2 debugger to the board and supply power to it, for example via USB, removing the P2 jumper so that the PC does not try to identify the board as a USB device, but simply supplies power. Press
F6 to flash using ST-Link / V2 or select “Tools-> Flash w ST-Link / V2” and wait a few seconds and if everything is done correctly, the LEDs will start blinking alternately, which is what we wanted:
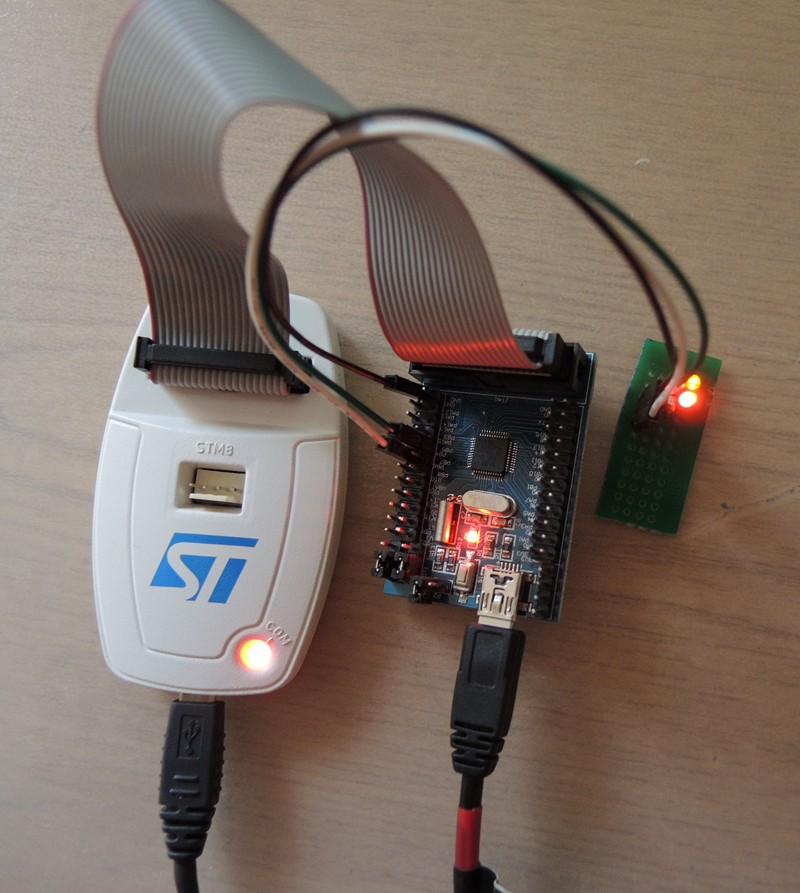
Pretty simple, isn't it?
The whole process takes about a minute. If you still failed,
download the project and compare it with what you did.
For another board, only the choice of processor from the list will change if it is different and the LED definitions, if it is connected to a different port.
For example, for STM32VLDiscovery:
#define RCC_GPIO RCC_APB2Periph_GPIOC #define LED_PORT GPIOC #define LED1_PIN GPIO_Pin_8 #define LED2_PIN GPIO_Pin_9