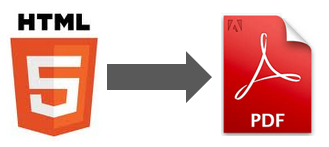
PDF - the format that has already become the standard. It was originally created by Adobe to represent text and images in a fixed structure document. It's not uncommon for web applications that support data download, such as invoices or reports, to give them in PDF format. So in this article we will go through a simple generation of PDF documents using PHP.
Dompdf is an excellent library that can generate PDFs from HTML markup and CSS styles (in most cases, they are CSS 2.1 compatible with some CSS3 properties). We can determine how our content should look using these familiar technologies, and then easily convert it into a fixed document. This library also has other useful and interesting features.
Getting Started
Dompdf is available on GitHub and can be installed using Composer. Installing through Composer without any errors often causes difficulties, so I recommend simply using Git to install Dompdf.
The library requires PHP> = 5.0 with activated mbstring and DOM extensions. It also requires several fonts, which are usually available on most computers.
')
Go to the directory where you want to install the library and run the command line:
git clone https://github.com/dompdf/dompdf.git git submodule init git submodule update
Once we have downloaded the Dompdf, let's write a short example that will generate a simple PDF document:
<?php set_include_path(get_include_path() . PATH_SEPARATOR . "/path/to/dompdf"); require_once "dompdf_config.inc.php"; $dompdf = new DOMPDF(); $html = <<<'ENDHTML' <html> <body> <h1>Hello Dompdf</h1> </body> </html> ENDHTML; $dompdf->load_html($html); $dompdf->render(); $dompdf->stream("hello.pdf");
In order to use the library in a project, we first pull up the
dompdf_config.inc.php file, which contains most of the Dompdf configuration. It also loads the autoloader and a custom configuration file in which we can override the default settings.
HTML markup is passed as a string to the
load_html () method. Alternatively, we can load markup from a file or URL using the
load_html_file () method. It takes the file name or URL of a webpage as an argument.
The
render () method displays HTML to PDF, and we are ready to upload the file. The
stream () method sends the resulting PDF as an attachment to the browser. This method has an optional second parameter, an array of options:
- Accept-Ranges - boolean , sends the “Accept-Ranges” header ( false by default).
- Attachment - boolean , sends the header “Content-Disposition: attachment” forcing the browser to display the save request ( true by default).
- compress - boolean , turns on content compression ( true by default).
We have just generated a very simple PDF, but this is not very practical. In reality, we often have requirements for page size, page orientation, character encoding, etc. There are a number of options that we can set to make Dompdf more suitable for our actual needs. All of them are listed and explained in the file
dompdf_config.inc.php , which sets them default values. You can change these values ​​by updating the user configuration file
dompdf_config.custom.inc.php . Here are some of the important settings:
- DOMPDF_DEFAULT_PAPER_SIZE - sets the default sheet size for a PDF document. Supported sheet sizes can be found in the include / cpdf_adapter.cls.php file (the default is “letter” ).
- DOMPDF_TEMP_DIR - indicates the temporary folder used by Dompdf. Make sure that this directory is writable according to the settings of your web server.
- DOMPDF_UNICODE_ENABLED — Sets whether PDF will use Unicode fonts ( true by default).
- DOMPDF_ENABLE_REMOTE - activates the inclusion of images or CSS styles from remote sites ( false by default).
- DEBUG_LAYOUT - sets whether the border will be displayed around each HTML block in a PDF file. Very handy for debugging the layout ( false by default).
Advanced use
Now let's talk a little about advanced usage of Dompdf. Perhaps we want to save the generated PDF document to disk, instead of sending it to the browser. Here is how it is done:
<?php $dompdf = new DOMPDF(); $dompdf->load_html($html); $dompdf->render(); $output = $dompdf->output(); file_put_contents("/path/to/file.pdf", $output);
Instead of calling
stream () , as in the previous example, we use
output () , which returns the PDF as a string. This method also accepts an array of options, but only one is available -
compress (
true by default).
Dompdf also allows us to add a header and footer to the generated PDF by embedding the PHP script directly in the HTML that it displays. But due to the fact that the processing of arbitrary code may pose a security risk, the configuration value, which is responsible for this functionality, is disabled by default. We need to first set the
DOMPDF_ENABLE_PHP option to
true .
As soon as we enable the execution of the embedded PHP, the PDF object will be available inside the script and we can use it to manipulate the page. We can add text, lines, images, rectangles, etc.
$html = <<<'ENDHTML' <html> <body> <script type="text/php"> if (isset($pdf)) {
The script is embedded directly in the HTML markup and first opens the object so that we can influence the display. All drawing will be written to this object and we will be able to add it to all selected pages (although there are limitations).
Then we get the actual width and height of the page to calculate the coordinates of the footer that we are going to add. We also need to provide a font object as we add text content.
Font_Metrics :: get_font () allows you to create an object that we need. We also take the height of this font from its size using
get_font_height () to calculate the positioning of the contents of the footer. The
get_font_width () method returns the width of our text for a given font and size, which we also use in our calculations.
The
line () method draws a line from point (X1, Y1) to point (X2, Y2). Note that the color value is not completely substituted in RGB. The main PDF class requires values ​​between 0 and 1, so we convert the RGB values ​​into these new values. To get the best approximation, you can divide them by 255.
We add a number for each page using the
page_text () method, which accepts the X and Y coordinates, as well as the text to be added, the font object, font size and color. Dompdf automatically replaces the values ​​for
{PAGE_NUM} and
{PAGE_COUNT} on each page, and makes
$ pdf available to us.
We can also not use the built-in PHP and achieve the same effect directly from PHP, like this:
<?php $dompdf = new DOMPDF(); $dompdf->set_paper("A4");
Note that we place the code after calling
$ dompdf-> render () because we essentially edit the already created PDF.
Let's sum up
In this article, we discussed an easy way to convert HTML to PDF using Dompdf. Although Dompdf is an excellent library, it is not a fully universal solution for generating PDF documents; it still has certain limitations and problems. Dompdf is not very tolerant of poorly formed HTML and large tables can easily lead to memory overflow. Some basic CSS features such as
float are not fully supported. And in general, CSS3 support is very limited. If you need features that are not supported in Dompdf, wkhtmltopdf can help you for example. Nevertheless, Dompdf is a very simple and convenient tool for solving most tasks on exporting PDF.
In fact, it is quite difficult to explain all the functions of a library in an article like this, so be sure to
review the documentation and source code , as well as learn useful features such as adding callbacks, using your own fonts, etc.
Original - phpmaster.com/convert-html-to-pdf-with-dompdf